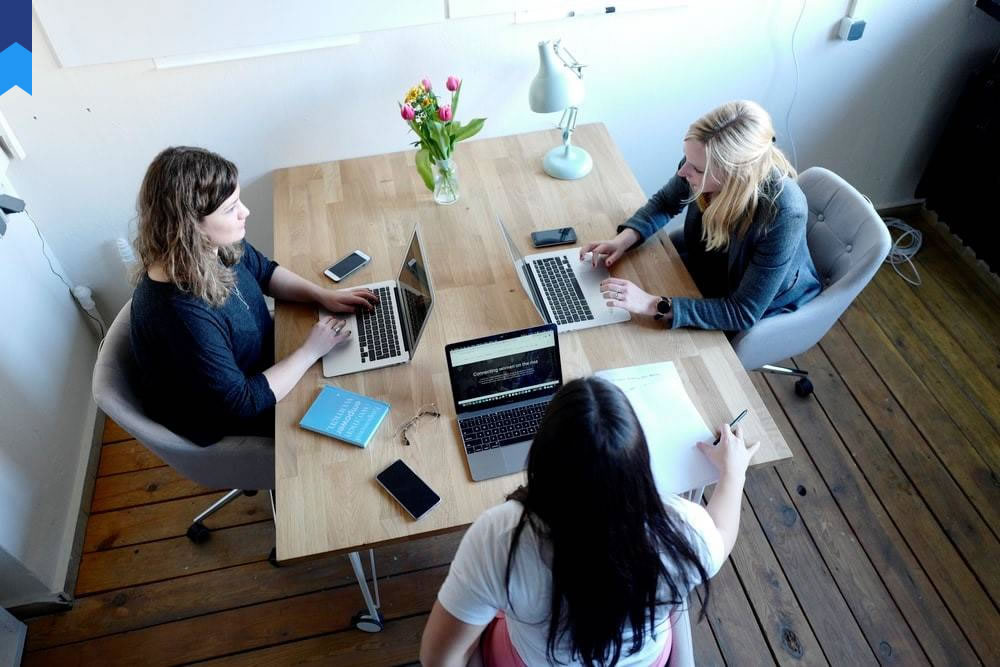
How to design and implement algorithms for efficient computation
The backbone of computer science, and designing efficient algorithms is crucial for solving complex computational problems. An efficient algorithm is one that uses minimal computational resources (such as time and memory) while still producing the desired output. In this article, we will explore the principles and techniques for designing and implementing efficient algorithms.
1. Understand the Problem
Before designing an algorithm, it is essential to understand the problem you are trying to solve. This involves:
- Defining the problem statement: Clearly articulate the problem you are trying to solve, including the inputs, outputs, and any constraints.
- Identifying the requirements: Determine what is required to solve the problem, including any specific properties or conditions that must be satisfied.
- Analyzing the problem: Break down the problem into smaller components and identify any patterns, relationships, or constraints that can help inform your algorithm design.
2. Choose an Algorithm Design Paradigm
There are several algorithm design paradigms, each with its strengths and weaknesses. Some common paradigms include:
- Brute Force: A brute force algorithm involves trying all possible solutions until the correct one is found. This approach can be effective for small problems but becomes impractical for larger problems.
- Divide and Conquer: This approach involves breaking down a problem into smaller sub-problems and solving each sub-problem recursively until the solution is found.
- Greedy Algorithm: A greedy algorithm makes the locally optimal choice at each step, hoping that these local choices will lead to a global optimum.
- Dynamic Programming: Dynamic programming involves breaking down a problem into smaller sub-problems and solving each sub-problem only once, storing the solutions to sub-problems in a table for later reuse.
3. Analyze Time and Space Complexity
Once you have chosen an algorithm design paradigm, it's essential to analyze the time and space complexity of your algorithm. Time complexity refers to how long an algorithm takes to complete, measured in terms of input size. Space complexity refers to how much memory an algorithm requires.
- Big O Notation: Big O notation is a way of expressing time and space complexity using upper bounds (e.g., O(n), O(n log n), etc.). This notation helps us compare the efficiency of different algorithms.
- Asymptotic Analysis: Asymptotic analysis involves analyzing an algorithm's performance as the input size approaches infinity. This helps us understand how an algorithm's performance will scale as the input size increases.
4. Implement the Algorithm
Once you have designed and analyzed your algorithm, it's time to implement it. This involves:
- Choosing a programming language: Select a programming language that is suitable for your algorithm, taking into account factors such as syntax, performance, and ease of use.
- Writing pseudocode: Write pseudocode for your algorithm using a high-level language, focusing on the logic and control flow.
- Implementing in code: Translate your pseudocode into actual code using a programming language.
- Testing and debugging: Test your implementation thoroughly to ensure it produces the correct output and handles edge cases correctly.
5. Optimize and Refine
After implementing your algorithm, you may need to optimize and refine it to improve its performance.
- Profile and benchmark: Use profiling tools to identify bottlenecks in your algorithm's performance.
- Optimize hotspots: Focus on optimizing sections of code that have the greatest impact on performance.
- Refine data structures: Choose data structures that are well-suited for your algorithm's requirements.
- Consider parallelism: Explore opportunities for parallelism to improve performance on multi-core processors.
Examples of Efficient Algorithms
- Binary Search: Binary search is an efficient algorithm for finding an element in a sorted array by repeatedly dividing the search space in half until the element is found.
- Fibonacci Series: The Fibonacci series is an example of a dynamic programming algorithm that solves a problem by breaking it down into smaller sub-problems.
- QuickSort: QuickSort is an efficient sorting algorithm that uses divide-and-conquer approach to sort an array of elements.
Challenges in Designing Efficient Algorithms
- Scalability: Designing algorithms that scale well with large inputs is crucial for solving complex problems.
- Trade-offs: Algorithms often require trade-offs between time and space complexity, making it essential to carefully consider these trade-offs during design.
- Computational Complexity Theory: Understanding computational complexity theory is essential for designing algorithms with good time and space complexity.
Designing efficient algorithms requires a deep understanding of computer science principles, including analysis of time and space complexity, asymptotic analysis, and optimization techniques. By following a structured approach to designing algorithms, developers can create solutions that are both efficient and effective.
Additional Resources
- Introduction to Algorithms by Thomas H. Cormen
- Algorithms by Robert Sedgewick
- Computational Complexity Theory by Michael Sipser
By studying these resources and practicing with real-world examples, developers can gain a deeper understanding of efficient algorithm design principles and improve their skills in designing algorithms for efficient computation
Related Courses and Certification
Also Online IT Certification Courses & Online Technical Certificate Programs