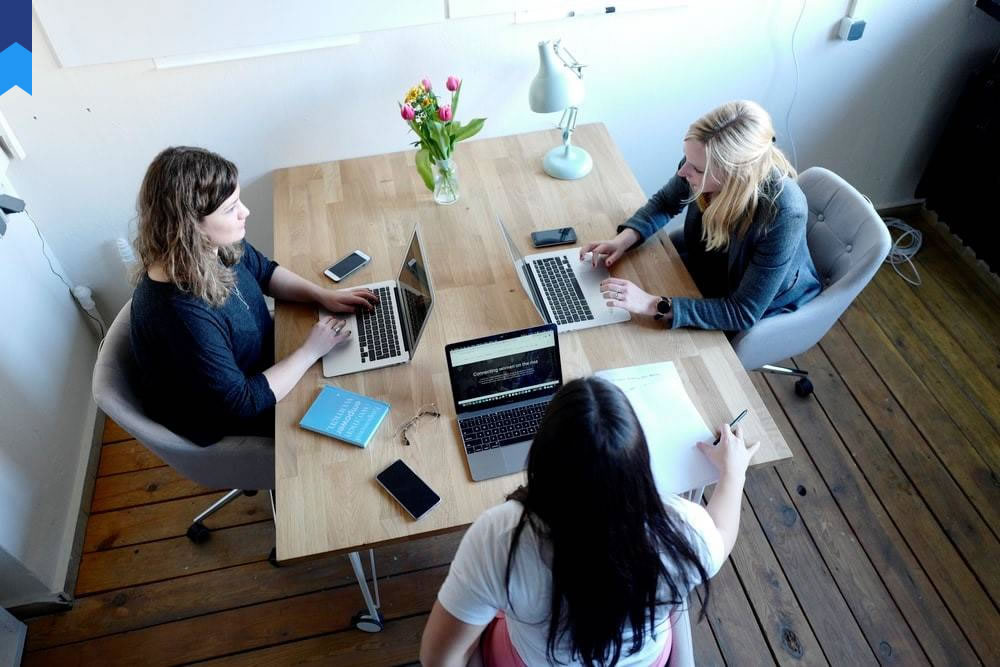
Optimizing Your Java Performance: Unlocking Hidden Potential
Java, a stalwart in the programming world, often finds itself burdened by performance bottlenecks. This article delves beyond superficial optimizations, exploring unconventional strategies to unlock previously untapped potential within your Java applications. We'll dissect common performance pitfalls and unveil techniques to dramatically improve efficiency, scalability, and responsiveness.
Unveiling the Mysteries of JVM Tuning
The Java Virtual Machine (JVM) is the heart of any Java application. Understanding its inner workings is crucial for performance optimization. Many developers overlook the power of JVM flags, critical parameters that can significantly influence garbage collection, memory allocation, and overall throughput. For instance, setting the correct heap size using the `-Xmx` and `-Xms` flags is paramount. A heap that's too small leads to frequent garbage collections, impacting responsiveness, while one that's too large wastes memory. Careful analysis of your application's memory usage using tools like JProfiler or YourKit is essential for accurate tuning.
Case Study 1: A large e-commerce platform experienced a dramatic 30% reduction in latency by meticulously tuning its JVM parameters, focusing on the garbage collection strategy and adjusting the heap size based on peak load patterns. The platform transitioned from a "stop-the-world" garbage collector to a concurrent collector, minimizing application pauses during garbage collection cycles. Case Study 2: A financial institution significantly improved transaction processing speeds by fine-tuning the JVM's JIT compiler settings, optimizing code compilation for specific hardware architectures. This resulted in a noticeable increase in application responsiveness and throughput.
Beyond heap size, exploring different garbage collection algorithms is key. The choice between Serial, Parallel, Concurrent Mark Sweep (CMS), G1GC, and ZGC depends on the application's characteristics. Applications with short-lived objects might benefit from the speed of Parallel GC, while long-running applications might prefer the lower latency of G1GC or ZGC. Experimentation and profiling are crucial to determining the optimal strategy for your specific environment.
Furthermore, understanding the JVM's internal memory management is essential. The permanent generation (Metaspace in newer JVMs) holds class metadata, and its size can influence performance. By monitoring memory usage and tuning the Metaspace size, developers can prevent OutOfMemoryErrors and improve overall stability.
Effective JVM tuning requires a deep understanding of your application's workload, memory patterns, and the intricacies of the garbage collection process. Continual monitoring and adjustment are necessary to maintain optimal performance over time.
Mastering the Art of Efficient Data Structures
Choosing the right data structures significantly impacts performance. Using an inappropriate data structure can lead to unnecessary overhead, impacting search, insertion, and deletion operations. For instance, using an ArrayList for frequent insertions and deletions is inefficient; a LinkedList would be a better choice. Similarly, using a HashMap for fast lookups is preferable over iterating through an ArrayList.
Case Study 1: A social media platform shifted from using an ArrayList to a more efficient data structure for storing user connections, reducing the time complexity of friend requests and notifications dramatically. This optimization significantly improved the overall responsiveness of the platform. Case Study 2: A logistics company used a Trie data structure to optimize the searching and autocompletion of addresses, resulting in a speed increase of more than 40% in their address lookup processes.
Understanding the time and space complexities of different data structures – arrays, linked lists, hash tables, trees, graphs – is crucial for making informed decisions. This knowledge enables developers to choose the structure that aligns with the specific needs of the application, minimizing time complexity and maximizing efficiency. Utilizing profiling tools can highlight performance bottlenecks caused by inefficient data structures, guiding optimization efforts.
Beyond basic data structures, exploring specialized structures like Bloom filters, tries, and skip lists can offer dramatic performance improvements in specific contexts. For instance, Bloom filters can efficiently check for the existence of an element in a set, making them ideal for tasks like spam filtering or duplicate detection. Tries, designed for efficient string searching, are valuable for applications like autocomplete functionality.
Proper data structure selection requires a careful analysis of the application’s operational demands, considering factors like data access patterns, frequency of updates, and memory constraints. Experimentation and performance testing are essential for validating the effectiveness of chosen data structures.
Concurrency and Parallelism: Unleashing Java's Multicore Potential
Modern processors boast multiple cores, yet many Java applications fail to leverage this parallelism. Properly utilizing threads and concurrent data structures can drastically improve performance, particularly for CPU-bound tasks. However, concurrent programming introduces complexities, requiring careful consideration of thread safety and synchronization mechanisms.
Case Study 1: A scientific simulation application that previously ran on a single core achieved a significant speedup by parallelizing its computation using Java's Fork/Join framework, distributing the workload across multiple cores. Case Study 2: A real-time trading platform implemented a concurrent algorithm for processing market data, significantly reducing latency and improving responsiveness in a high-volume environment.
Java's concurrency utilities, including `ExecutorService`, `Future`, and `Callable`, simplify the creation and management of threads. Using these tools, developers can efficiently parallelize tasks without the complexities of manually managing threads. Understanding the implications of thread safety and potential race conditions is crucial for preventing unexpected behavior and ensuring data consistency.
Effective use of concurrent data structures, like `ConcurrentHashMap` and `ConcurrentLinkedQueue`, is essential for efficient thread-safe data sharing. These structures provide built-in synchronization mechanisms, eliminating the need for manual locking, improving performance, and reducing the risk of deadlocks.
However, excessive thread creation can lead to performance degradation due to context switching overhead. Carefully managing the number of threads, using thread pools, and employing efficient synchronization techniques are vital for maximizing performance gains.
Optimizing for concurrency requires a deep understanding of thread management, synchronization primitives, and the potential for race conditions and deadlocks. Thorough testing and profiling are essential to ensure correctness and optimal performance in concurrent environments.
Database Interactions: Minimizing Overhead
Database interactions are often major performance bottlenecks. Poorly written database queries, excessive round trips, and inefficient data retrieval can severely impact application responsiveness. Optimizing database interactions is crucial for improving overall performance.
Case Study 1: An online banking system improved its transaction processing speed by optimizing its SQL queries, reducing the number of database accesses, and implementing caching mechanisms. Case Study 2: An e-commerce platform improved the speed of product searches by implementing efficient indexing strategies in its database.
Properly indexing database tables is crucial for efficient data retrieval. Indexing allows the database to quickly locate relevant rows without scanning the entire table, dramatically reducing query execution time. Choosing the right indexing strategy depends on the query patterns and data characteristics. Using profiling tools to identify slow queries and analyze query execution plans is essential for effective optimization.
Batching database operations can reduce the overhead associated with multiple round trips. Instead of executing many individual queries, batching operations allows for sending multiple updates or queries in a single transaction, minimizing network latency and improving throughput.
Caching frequently accessed data can significantly reduce database load. By storing frequently accessed data in memory, applications can avoid repeated database queries, improving responsiveness and reducing database server load. Implementing an effective caching strategy requires careful consideration of cache size, eviction policies, and data consistency.
Utilizing connection pools can also dramatically improve database interaction performance. Connection pools allow applications to reuse database connections, minimizing the overhead of establishing new connections for every query, leading to better performance and improved resource utilization. Optimizing database interactions requires a holistic approach, incorporating indexing strategies, batching operations, caching, and connection pooling.
Code Optimization Techniques: Refining for Efficiency
Even with optimized infrastructure, inefficient code can negate performance gains. Applying code optimization techniques can further enhance performance. These techniques include using efficient algorithms, reducing object creation, and minimizing unnecessary method calls.
Case Study 1: A game development studio optimized its rendering engine by implementing more efficient algorithms for rendering 3D graphics, resulting in significant improvements in frame rates. Case Study 2: A data processing application reduced its memory footprint and execution time by eliminating unnecessary object creation and reusing objects whenever possible.
Utilizing efficient algorithms is crucial for minimizing computational complexity. Understanding the time and space complexity of algorithms allows developers to choose the most efficient solution for the task at hand. Using profiling tools helps identify performance bottlenecks in the code, guiding optimization efforts.
Reducing object creation minimizes garbage collection overhead. Excessive object creation increases the load on the garbage collector, impacting application responsiveness. Reusing objects whenever possible reduces the number of objects that need to be garbage collected, improving performance.
Minimizing unnecessary method calls reduces the overhead associated with function calls. By avoiding unnecessary calls and optimizing frequently called methods, developers can improve overall performance. Profiling helps identify frequently called methods, guiding optimization efforts.
Code optimization is an iterative process that requires careful attention to detail. Continuous monitoring and profiling are essential for identifying areas for improvement and measuring the impact of optimization efforts.
Conclusion
Optimizing Java performance isn't a one-size-fits-all solution. It demands a multifaceted approach, encompassing JVM tuning, judicious data structure selection, efficient concurrency strategies, database optimization, and meticulous code refinement. By mastering these techniques, developers can unlock hidden potential within their Java applications, leading to improved responsiveness, scalability, and overall efficiency. The journey towards peak performance involves continuous monitoring, profiling, and iterative improvements, consistently challenging conventional wisdom and embracing innovative strategies to push the boundaries of Java's capabilities.