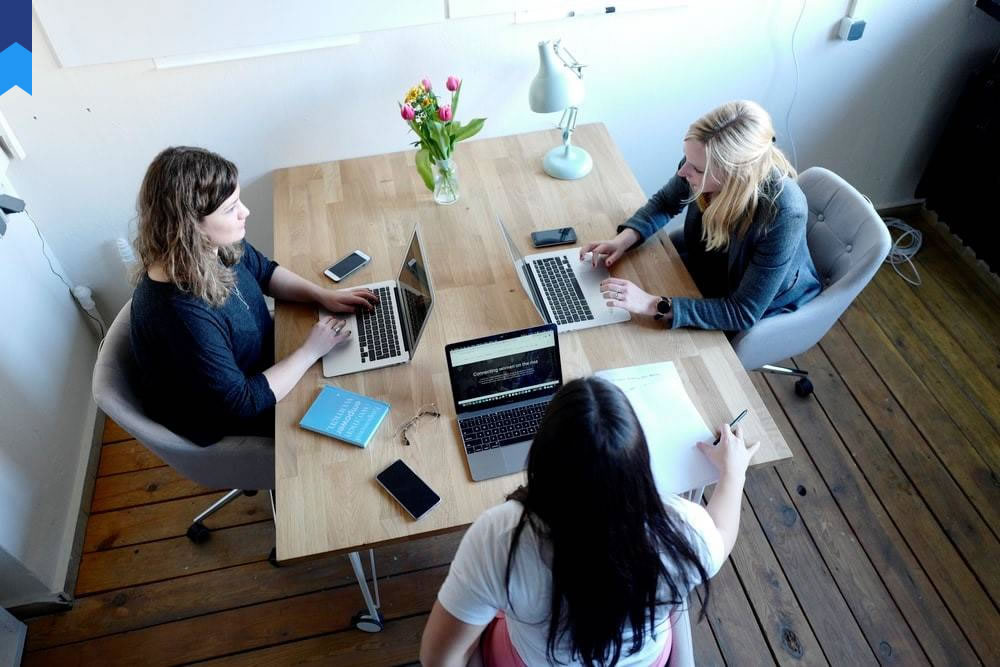
The Counterintuitive Guide to Microprocessor Mastery
Microprocessors: the silent workhorses powering our digital world. Understanding their intricacies, however, often feels like deciphering an ancient code. This guide flips the script, offering counterintuitive approaches to mastering these complex chips. We'll explore techniques that might initially seem contradictory, yet ultimately unlock a deeper understanding and enhance your programming proficiency. This isn't a basic overview; we delve into practical applications and innovative strategies that will transform your microprocessor skills.
Understanding Interrupt Handling: The Art of Controlled Chaos
Interrupt handling is often viewed as a complex, error-prone aspect of microprocessor programming. The counterintuitive approach? Embrace the chaos. Instead of striving for perfectly predictable interrupt sequences, learn to manage the unpredictable nature of interrupts. Implement robust error handling routines that gracefully manage unexpected interruptions. A well-structured interrupt service routine (ISR) should be designed to handle a wide variety of potential errors, allowing the system to recover smoothly from unforeseen events. For example, consider a real-time operating system (RTOS) where interrupts might arrive at any time. Instead of fighting this, structure your code to handle the variations in interrupt timing and prioritize critical interrupts effectively. Case study: Industrial control systems rely heavily on interrupt handling for rapid response to sensor data. Poorly designed interrupt handling can lead to catastrophic failures. A counterintuitive approach here might involve prioritizing interrupts based on the criticality of the sensor data rather than a strict sequential order. Another case study would be the development of embedded systems for automotive applications, where interrupt response speed is crucial for safety features like anti-lock brakes (ABS). Robust interrupt handling, rather than simple prioritization, is essential here to prevent unexpected system behavior.
Furthermore, consider the use of interrupt coalescing, where multiple interrupts are combined into a single interrupt to reduce overhead. This can seem counterintuitive, as it might seem to lead to a loss of precision; however, in many situations, the benefits of reduced processing load outweigh the minor loss of granularity. Another approach is to preemptively handle potential interrupt conflicts through careful system design. By anticipating potential clashes, developers can create a more resilient system that is less prone to unexpected behaviour. This includes techniques such as disabling interrupts during critical sections of code, or using atomic operations to ensure data integrity. The key is to anticipate rather than react to interrupt-related issues.
Advanced techniques like interrupt chaining allow for complex interrupt management, where one interrupt triggers another, creating a cascade of events. Properly implementing this can lead to increased efficiency. A final counterintuitive tip is to thoroughly test your interrupt handling under stress. Intentionally introducing unpredictable interrupt patterns during testing will reveal weaknesses in your system that might otherwise go unnoticed under normal operation. This proactive approach can significantly improve the reliability of your microprocessor applications.
Consider the case of a data acquisition system. The traditional approach might be to process each data point individually upon arrival. A more counterintuitive approach might involve buffering the data and processing it in batches. This could significantly reduce the interrupt overhead and improve overall system performance. Another example involves network communications. Instead of responding to each network packet individually, buffering packets allows for efficient batch processing, improving throughput. Properly implementing this involves a balance between buffer size, processing speed and latency requirements. Understanding these trade-offs is critical.
Memory Management: Thinking Outside the Cache
Efficient memory management is key to high-performance microprocessor applications. The counterintuitive approach involves understanding the limitations of caching mechanisms. Instead of solely relying on the cache for speed improvements, consider strategies that minimize cache misses. This includes data structures designed to promote locality of reference. Case study: In embedded systems development, memory access is often constrained by the limited resources available. A counterintuitive approach is to use memory mapping strategically to access peripheral devices, minimizing memory allocation and deallocation calls. Another case study would be in game development, where efficient texture management within the GPU's cache is vital for smooth frame rates. Focusing on minimizing cache misses can provide significant performance improvements, even more so than focusing solely on cache hits.
The use of memory-mapped I/O is another aspect worth exploring. It offers a flexible but potentially unpredictable method of interfacing with peripherals. While it might initially seem less efficient than other methods, with careful planning it can offer significant advantages in terms of flexibility. Understanding memory alignment and its impact on performance is crucial, often ignored in basic tutorials. Data structures like arrays and structs must be aligned properly to avoid costly memory access penalties. Consider the use of page tables, a sophisticated method of memory management typically used in operating systems. While they appear complex at first glance, understanding how page tables optimize memory access will significantly improve your understanding of sophisticated microprocessor systems.
Optimizing data structures for improved cache utilization is crucial. This means minimizing cache misses by strategically arranging data in memory. The design of efficient data structures such as linked lists versus arrays must take into account the processor's cache architecture. This can involve understanding concepts such as cache lines and their size, in order to improve cache utilization. The choice of data structure should be driven by cache utilization, not simply convenience or familiarity. Another counterintuitive strategy involves using techniques such as pre-fetching to anticipate data needs, bringing data into the cache before it is actually needed. This is a more advanced technique, but it can dramatically improve performance in certain scenarios.
A case study in optimizing memory management could be found in the development of database systems, where efficient storage and retrieval of vast amounts of data are critical. Here, strategies to minimize cache misses and efficiently utilize memory can have a significant impact on overall performance. Another case study would be real-time image processing, where processing large image data efficiently necessitates clever memory management and careful consideration of cache utilization. By understanding and minimizing cache misses, performance can be drastically improved. The seemingly counterintuitive approach of prioritizing cache efficiency over simply increasing cache size can lead to significant performance gains.
Parallel Processing: Embracing Asynchronous Operations
Parallel processing is no longer a niche technique; it's essential for modern microprocessor applications. The counterintuitive approach is to embrace asynchronous operations. Instead of rigidly synchronizing tasks, learn to leverage asynchronous programming models. This allows for concurrent execution of multiple tasks, maximizing the utilization of multiple cores. A case study: In a high-performance computing environment, using asynchronous I/O allows for sustained high throughput. Another case study would involve the development of server-side applications, where handling multiple client requests concurrently significantly improves responsiveness. Asynchronous operations are key to building scalable and responsive systems.
Utilizing multithreading techniques allows multiple threads to execute concurrently, increasing performance. However, it's crucial to handle thread synchronization properly, often a neglected detail that can cause significant issues. Learning to avoid race conditions and deadlocks is crucial for effective multithreading. In contrast to synchronous programming, where tasks are executed sequentially, asynchronous operations allow for the efficient use of resources by allowing multiple tasks to execute concurrently without waiting for each other. This leads to improved utilization of processor cores and, consequently, greater performance. Consider the development of parallel algorithms, where data is broken down into smaller chunks that can be processed independently on different cores. Carefully selecting an appropriate algorithm allows for parallel processing to produce a significant speed improvement over a sequential approach.
Efficiently managing shared resources between threads is another counterintuitive aspect. It requires careful consideration and implementation of thread synchronization mechanisms, such as mutexes and semaphores. These mechanisms provide a robust way to manage access to shared resources, preventing race conditions and ensuring data integrity. Understanding the nuances of these mechanisms is crucial for successfully developing parallel applications. The seemingly counterintuitive need for careful control and management of asynchronous operations is essential to realizing the performance benefits.
Modern processors have multiple cores, and utilizing all of them effectively can often seem counterintuitive, especially given the complexities of multi-core programming. Effectively splitting tasks across multiple cores is essential to reaping the benefits of parallel processing. However, careful consideration must be given to communication overhead and task granularity to minimize the negative impact of task splitting. A case study involves handling massive datasets, where breaking down the data into smaller chunks for parallel processing enables significantly faster analysis. Another case study would be the development of high-frequency trading algorithms, where processing speed is paramount. Utilizing all available cores in a highly efficient manner is key to optimizing the algorithm's performance.
Power Management: The Low-Power Paradox
In many embedded systems, power consumption is a primary concern. The counterintuitive approach is to prioritize low-power design from the outset. This often involves selecting the right hardware components and using power-saving modes effectively. A case study: In the design of wearable devices, maximizing battery life is essential. Using low-power microcontrollers and implementing aggressive power management techniques is crucial for achieving long battery life. Another case study would be in the design of remote sensors, where minimizing power consumption is vital for extending the device’s operational lifetime without requiring frequent battery changes.
Clock gating is a powerful technique to reduce power consumption. By selectively disabling clock signals to inactive components, power consumption can be significantly reduced. This strategy may appear counterintuitive, as it might seem to reduce performance; however, with careful planning and implementation, clock gating can significantly reduce power consumption without significantly impacting performance. Another aspect is the careful selection of operating voltage and frequency, often overlooked in initial designs. Balancing performance requirements with power consumption is key to efficient power management. Reducing the operating voltage can significantly reduce power consumption, but it is important to account for the impact on performance.
Dynamic voltage and frequency scaling (DVFS) is a technique that allows the processor to adjust its voltage and frequency based on the workload. This is crucial for optimizing power consumption without compromising performance. By reducing the voltage and frequency during periods of low activity, power consumption can be significantly reduced without significantly affecting performance. This approach may appear counterintuitive at first, as it might seem to be complicating the system. However, the resulting efficiency often significantly outweighs the additional complexity.
The use of sleep modes can drastically reduce power consumption. However, the seemingly counterintuitive aspect is that carefully selecting which components to power down and the optimal wake-up mechanisms can be crucial for achieving optimal power efficiency. This requires an in-depth understanding of the system’s behavior and power consumption characteristics. The choice of sleep mode needs to balance the power savings with the latency introduced by the wake-up process. A case study involves the development of energy-harvesting sensors, where maximizing energy efficiency is crucial for self-powered operation. Another case study involves designing long-range wireless sensor networks, where extended battery life is paramount for the overall network’s longevity and efficiency.
Debugging Techniques: The Unexpected Paths to Solutions
Debugging is an iterative process, often frustrating. The counterintuitive approach is to embrace systematic debugging methodologies and avoid rushing to solutions. Using a debugger effectively, including setting breakpoints and stepping through the code, will greatly expedite the debugging process. Case study: In embedded systems, where direct access to hardware is often necessary, careful use of debugging tools is crucial for effective troubleshooting. Another case study involves network applications, where network monitoring tools are often necessary for effective debugging.
Utilizing logging effectively is often overlooked. A comprehensive logging system allows for tracing the execution flow of a program and identifying areas that are exhibiting unexpected behavior. This requires thinking ahead and designing the logging system in a way that captures as much relevant information as possible. Understanding the debugging process involves learning to effectively use a debugger, examining memory and register values, and setting breakpoints at critical junctures in the code execution. Effective logging facilitates identifying potential issues during development, enabling efficient problem identification and resolution.
Utilizing simulation and emulation techniques for debugging is often counterintuitive, as it might seem to add additional steps to the process. However, the ability to replicate a system environment before deploying the code to the actual hardware can often save significant time and effort. This allows developers to catch issues early, preventing costly and time-consuming debugging sessions on the physical hardware. In addition to simulation, the use of code analysis tools and static analysis to identify potential errors before runtime can save significant time and effort. These tools can automatically flag potential issues such as memory leaks or undefined behavior.
Systematically isolating the problem is often overlooked. Instead of randomly changing code segments in the hope of fixing an issue, a methodical approach will often lead to a faster resolution. This often involves a careful examination of the program's behavior, including the input, output, and intermediate data to isolate potential sources of error. A case study involves identifying and resolving race conditions in parallel programs, requiring a systematic analysis of thread interactions and shared memory access. Another case study involves troubleshooting hardware issues, requiring a thorough analysis of hardware signals and timing diagrams. The use of advanced debugging techniques often provides the most efficient means to identify and rectify errors, even though these techniques may initially seem counterintuitive.
Conclusion
Mastering microprocessors requires more than just memorizing specifications. It demands a shift in perspective, an embrace of the unexpected. By adopting these counterintuitive approaches – embracing interrupt chaos, thinking outside the cache, leveraging asynchronous operations, prioritizing low-power design, and employing systematic debugging – you'll unlock a deeper understanding and achieve greater success in your microprocessor endeavors. This isn't just about writing code; it's about understanding the underlying architecture and leveraging its potential in unconventional ways. Remember, the most innovative solutions often lie in challenging the status quo and exploring the paths less traveled.