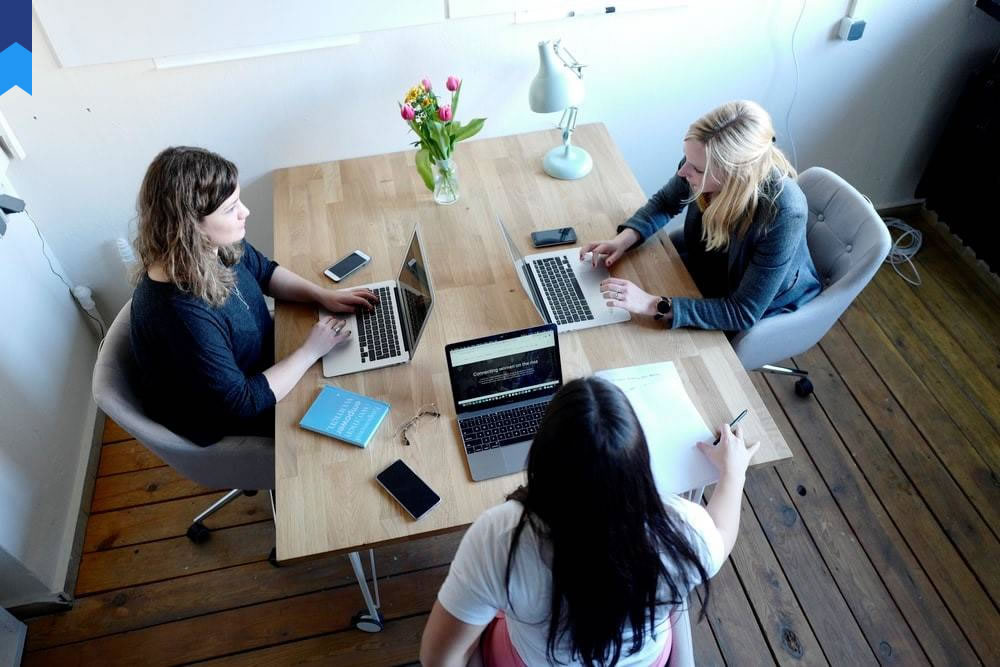
Unlocking the Secrets of C# Asynchronous Programming
C# asynchronous programming is a powerful tool that can significantly improve the performance and responsiveness of your applications. This article delves into the intricacies of asynchronous operations, exploring practical techniques and innovative approaches that go beyond the basics. We'll uncover the secrets to building efficient and scalable applications using asynchronous programming in C#.
Understanding Asynchronous Operations
Asynchronous programming allows your application to perform long-running operations without blocking the main thread. This prevents the user interface from freezing or becoming unresponsive while waiting for the operation to complete. A common example is downloading a large file; using asynchronous methods prevents the application from hanging while the download is in progress. The `async` and `await` keywords are central to this paradigm in C#. Consider a scenario where you're fetching data from a remote server. A synchronous approach would halt execution until the data arrives, impacting user experience. Asynchronous programming, however, allows the application to continue responding to user input while the data is fetched in the background.
Case Study 1: A web application that retrieves user profiles from a database. A synchronous approach would cause a significant delay, potentially making the application unusable. An asynchronous approach would maintain responsiveness, allowing users to interact with other parts of the application while their profile is loading. Case Study 2: A game that loads assets like textures and models. Asynchronous loading prevents the game from freezing while it loads these resources, leading to a smoother and more enjoyable experience.
Furthermore, the Task Parallel Library (TPL) in C# provides powerful tools for managing parallel operations. The `Parallel.ForEach` method allows you to efficiently process large collections of data across multiple threads, which is crucial for achieving optimal performance. The use of `async` and `await` along with TPL allows for both concurrency and responsiveness. Effective asynchronous programming requires careful consideration of resource management. While it enhances responsiveness, it also introduces the complexity of managing threads and potentially increasing resource usage if not implemented correctly. Therefore, understanding the underlying mechanisms is crucial for avoiding performance bottlenecks. Properly handling exceptions is also vital, using `try-catch` blocks around asynchronous operations to prevent unexpected application termination.
The benefits of asynchronous programming extend beyond responsiveness. It can lead to significant improvements in overall application performance, especially when dealing with I/O-bound operations like network requests or database interactions. By freeing up the main thread, asynchronous operations allow the application to handle more requests concurrently, increasing throughput and reducing response times. Modern applications often deal with multiple data sources and complex interactions. Effective asynchronous programming allows these applications to handle these complexities without sacrificing performance or responsiveness. Properly designed asynchronous code facilitates better resource utilization, reducing the need for excessive threads and ultimately improving the stability and reliability of the application.
Mastering Async and Await
The `async` and `await` keywords are fundamental to asynchronous programming in C#. The `async` keyword modifies a method, indicating that it's asynchronous and can use the `await` keyword. The `await` keyword pauses execution of the method until an awaited task completes. Consider this example: `async Task
Case Study 1: An e-commerce application asynchronously updates the inventory after each purchase. The `async` and `await` keywords ensure that the application remains responsive to new orders even while the inventory is being updated in the background. Case Study 2: A social media application that asynchronously fetches and displays new posts from various users. Using `async` and `await`, the application remains responsive while fetching posts, improving user experience and overall application performance.
Efficient use of `async` and `await` requires careful planning. Avoid nesting `await` calls excessively, as this can lead to a complex and difficult-to-debug codebase. Prefer using `Task.WhenAll` or `Task.WhenAny` to coordinate multiple asynchronous operations. These methods allow you to wait for multiple tasks to complete concurrently, or for the first one to finish, respectively. Understanding the differences and applications of these methods is essential for efficient asynchronous programming. Proper error handling is paramount in asynchronous operations. While exceptions can be caught using standard `try-catch` blocks, asynchronous exceptions may not propagate as straightforwardly as their synchronous counterparts. Therefore, using techniques like `try-catch` within the asynchronous function and handling potential exceptions through the `Task.ContinueWith` method or `Task.Exception` property are important strategies for robust asynchronous code.
Another critical aspect is cancellation. Long-running asynchronous operations might need to be cancelled. The `CancellationToken` provides this functionality. By incorporating `CancellationToken` into your asynchronous methods, you can gracefully stop them when necessary without causing unintended effects. This adds a layer of responsiveness to your application, enabling dynamic responses to user interactions or changing circumstances. Asynchronous operations, when not properly managed, can lead to performance bottlenecks. Avoid overuse of `async` and `await` where it’s not strictly necessary. Overly complex asynchronous operations, especially when dealing with nested awaits, can sometimes introduce performance overhead compared to a well-optimized synchronous approach. Therefore, the choice between synchronous and asynchronous operations should be determined based on the specific needs and characteristics of each application.
Handling Asynchronous Exceptions
Asynchronous exceptions require a different approach to handling compared to synchronous ones. Because asynchronous operations often occur on different threads, exceptions may not immediately propagate to the caller. Therefore, a common strategy is to use the `try-catch` block within the asynchronous method itself, to handle exceptions as locally as possible within the context of the asynchronous operation. In some cases, exceptions are aggregated to the `Task` object itself, and these can be retrieved using the `Task.Exception` property. Checking this property after awaiting a task allows for centralized exception handling.
Case Study 1: A file upload operation that might encounter network errors. Using `try-catch` blocks within the asynchronous upload method allows for specific error handling and prevents the entire application from crashing. Case Study 2: A database query that might encounter a connection timeout. Retrieving the `Task.Exception` property allows the application to gracefully handle the error and display a user-friendly message instead of crashing.
Another important technique is using `Task.ContinueWith`. This allows you to specify a continuation action to be executed when the preceding task completes, regardless of whether it succeeded or failed. This can be useful for cleanup operations or to perform post-processing actions in both successful and failed cases. Furthermore, proper logging of asynchronous exceptions is crucial for debugging and monitoring. Logging libraries can be integrated with asynchronous operations to capture relevant information regarding the exceptions, timestamps, and context. This helps developers in identifying and resolving errors effectively. This logging process should be tailored to the application's needs and reporting preferences, ensuring that the logging method doesn't impact the performance of the application significantly.
Advanced techniques involve creating custom exception handling strategies. For instance, a custom exception handler might be implemented to consolidate exception reporting and automate recovery procedures in cases of recoverable errors. This requires a deeper understanding of the application’s error handling mechanisms and the desired level of resilience. Asynchronous programming enhances application responsiveness, but it requires a different mindset when it comes to exception handling. By utilizing these approaches, you can create robust, responsive, and reliable asynchronous applications that gracefully handle errors and continue operating without disruptions or unexpected crashes.
Advanced Asynchronous Patterns
Beyond basic `async` and `await`, C# offers advanced asynchronous patterns that enhance efficiency and code organization. One such pattern is the use of asynchronous streams. These enable the processing of large datasets in a non-blocking way, where data is yielded as it becomes available. This contrasts with traditional approaches that load the entire dataset into memory at once. This pattern is especially beneficial when dealing with large files, streaming data from network sources, or handling continuous data feeds.
Case Study 1: An application processing large log files. An asynchronous stream would process each log entry as it's read, preventing memory exhaustion and improving responsiveness. Case Study 2: A real-time data visualization application receiving data from multiple sensors. Asynchronous streams allow the application to display data as it arrives, making the visualization more dynamic and responsive.
Another powerful technique is using Reactive Extensions (Rx). Rx provides a framework for composing asynchronous operations using observable sequences. This allows for creating complex asynchronous pipelines and handling events in a declarative way. Rx simplifies the handling of asynchronous events and provides sophisticated operators for transforming and filtering data streams. This approach improves code readability and maintainability, especially in scenarios with complex event flows and data processing requirements. Furthermore, understanding asynchronous cancellation is key. Long-running asynchronous operations often need to be cancelled, and the `CancellationToken` plays a critical role. Incorrect handling of cancellation can lead to resource leaks or unexpected behavior.
Advanced scenarios often involve asynchronous operations spanning multiple services or components. Proper coordination and management of these operations are critical to ensure consistent behavior and avoid deadlocks. Sophisticated techniques, such as distributed tracing and distributed logging, may be needed to debug and monitor such complex systems. Furthermore, performance optimization in advanced asynchronous scenarios requires careful consideration of thread pool usage, I/O operations, and resource allocation. Profiling tools and performance analysis techniques can provide valuable insights for identifying and resolving performance bottlenecks.
Optimizing Asynchronous Code
Optimizing asynchronous code focuses on maximizing performance and responsiveness without compromising resource utilization. One key aspect is to avoid overusing asynchronous operations when synchronous ones suffice. Unnecessarily introducing asynchronous operations can sometimes introduce overhead, outweighing the benefits. Profiling tools can help identify areas where optimization is needed, pinpointing the bottlenecks in the asynchronous pipeline.
Case Study 1: An application performing a series of CPU-bound calculations. Asynchronous operations might not improve performance in this case, as the calculations still need to be performed on a single thread, but will introduce overhead. Case Study 2: An application heavily reliant on network requests. Optimizing network requests using techniques such as connection pooling and efficient data serialization is crucial to maximizing asynchronous operation efficiency.
Another important aspect is efficient use of the thread pool. The thread pool manages threads used for asynchronous operations, and improper use can lead to resource exhaustion or starvation. Monitoring thread pool usage and adjusting the configuration can improve overall performance. Moreover, minimizing context switching is important for improved performance. Context switching involves switching between different threads, and excessive context switching can significantly reduce throughput. Strategies like using asynchronous streams or efficient task coordination can reduce this overhead. Careful consideration should also be given to memory management. Asynchronous operations can potentially increase memory usage due to the creation of tasks and intermediate results. Techniques such as efficient data structures and proper resource disposal are crucial for minimizing memory footprint.
Finally, continuous monitoring and performance analysis are key to maintaining optimal performance. Regular monitoring of key metrics like request latency, throughput, and resource utilization can help identify potential issues and guide optimization efforts. Employing monitoring and logging tools to track these metrics is vital for preventing performance degradation and ensuring the responsiveness and efficiency of the application over time. Continuous testing and optimization are essential to maintain the effectiveness of asynchronous operations, ensuring that the application remains responsive, efficient, and reliable.
Conclusion
Mastering asynchronous programming in C# is crucial for building responsive, efficient, and scalable applications. This journey involves understanding the fundamental concepts of `async` and `await`, handling exceptions effectively, leveraging advanced patterns, and optimizing for performance. By embracing these principles and incorporating best practices, developers can unlock the full potential of asynchronous programming in C#, creating applications that excel in both performance and user experience. The techniques presented here, from basic usage of `async` and `await` to sophisticated handling of asynchronous streams and reactive programming, provide a comprehensive approach to building robust and efficient applications. Continuously monitoring and optimizing asynchronous code is essential for ensuring that the application remains responsive and efficient, particularly as the application scales and handles increasing workloads. The combination of sound principles and effective optimization strategies is key to creating high-performance C# applications that are capable of handling the demands of the modern development landscape.