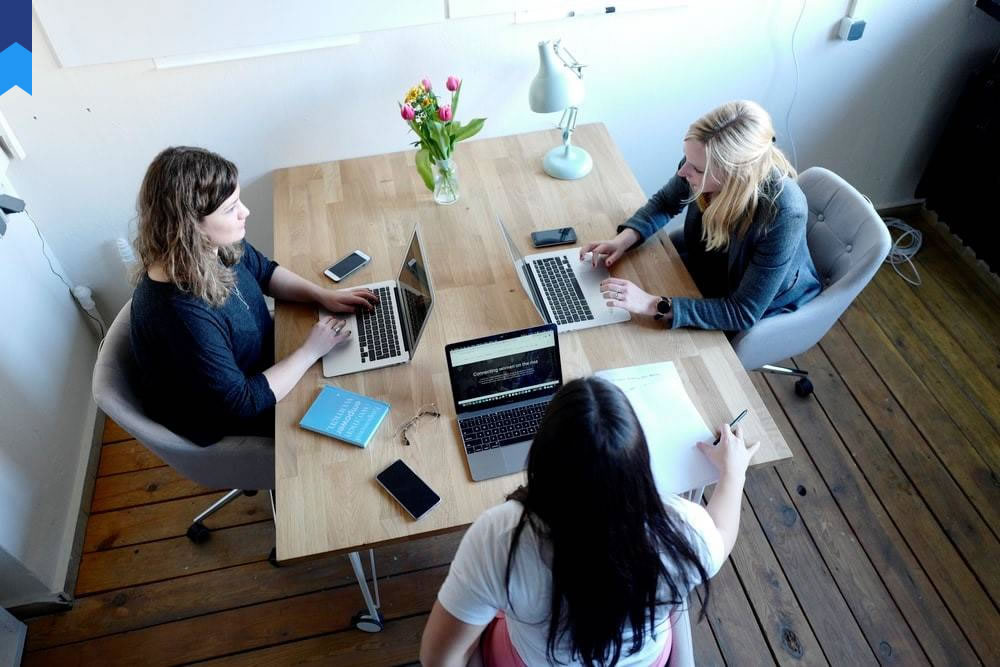
How to use data structures effectively in computer engineering projects
In computer engineering, data structures play a crucial role in the development of efficient and effective software systems. A data structure is a way to organize and store data in a program so that it can be easily accessed, manipulated, and retrieved. Effective use of data structures can significantly improve the performance, scalability, and maintainability of a program.
In this article, we will explore the importance of data structures in computer engineering projects and provide a comprehensive guide on how to use them effectively.
Why Data Structures are Important in Computer Engineering?
Data structures are essential in computer engineering because they help to:
- Improve Performance: Data structures allow for efficient storage and retrieval of data, which can significantly improve the performance of a program. For example, using a hash table instead of a linear search can reduce the time complexity of searching for an element from O(n) to O(1).
- Reduce Memory Usage: Data structures can help reduce memory usage by storing data in a compact and efficient manner. For example, using an array instead of a linked list can reduce memory usage by eliminating the need for pointers.
- Simplify Code: Data structures can simplify code by providing a standardized way of organizing and accessing data. For example, using an array instead of a linked list can simplify code by eliminating the need for manual memory management.
- Improve Scalability: Data structures can improve scalability by allowing for easy addition or removal of elements without affecting the overall structure of the program.
Types of Data Structures
There are several types of data structures that can be used in computer engineering projects, including:
- Arrays: An array is a collection of elements of the same data type stored in contiguous memory locations.
- Linked Lists: A linked list is a collection of elements where each element points to the next element in the list.
- Stacks: A stack is a Last-In-First-Out (LIFO) data structure that uses push and pop operations to add and remove elements.
- Queues: A queue is a First-In-First-Out (FIFO) data structure that uses enqueue and dequeue operations to add and remove elements.
- Trees: A tree is a hierarchical data structure where each node has a value and zero or more child nodes.
- Graphs: A graph is a non-linear data structure that consists of nodes and edges that connect the nodes.
- Hash Tables: A hash table is a data structure that stores key-value pairs in an array using a hash function to map keys to indices.
How to Use Data Structures Effectively
Here are some best practices for using data structures effectively in computer engineering projects:
- Choose the Right Data Structure: Choose the right data structure based on the problem you are trying to solve. For example, if you need to store and retrieve large amounts of data quickly, an array or hash table may be suitable.
- Use Abstract Data Types: Use abstract data types (ADTs) to define the behavior of your data structure, rather than implementing it directly. ADTs provide a contract that specifies how your data structure should behave, making it easier to change or replace it later.
- Minimize Indirection: Minimize indirection by avoiding unnecessary pointers or references between data structures. This can improve performance and simplify code.
- Use Iterators Correctly: Use iterators correctly by iterating over your data structure only when necessary, and avoiding unnecessary copying or mutation of the underlying data.
- Test Your Data Structure: Test your data structure thoroughly to ensure it meets your requirements and behaves as expected.
Best Practices for Specific Data Structures
Here are some best practices for specific data structures:
- Arrays:
- Use arrays when you need fast access to elements by index.
- Use multi-dimensional arrays when you need to store complex relationships between elements.
- Avoid using arrays with dynamic sizes or resizing arrays frequently.
- Linked Lists:
- Use linked lists when you need to frequently insert or delete elements at arbitrary positions.
- Use double-linked lists when you need to traverse the list backwards as well as forwards.
- Avoid using linked lists with large numbers of nodes or complex node structures.
- Stacks:
- Use stacks when you need to implement LIFO behavior.
- Use recursive algorithms with care when using stacks, as they can lead to stack overflow errors.
- Avoid using stacks with large numbers of elements or complex stack operations.
- Queues:
- Use queues when you need to implement FIFO behavior.
- Use priority queues when you need to prioritize elements based on their priority.
- Avoid using queues with large numbers of elements or complex queue operations.
Real-World Applications of Data Structures
Data structures are used extensively in real-world applications, including:
- Database Management Systems: Databases use various data structures such as arrays, linked lists, trees, and graphs to store and retrieve large amounts of data efficiently.
- Web Search Engines: Search engines use hash tables and graphs to index web pages and retrieve search results quickly.
- Compilers: Compilers use parsing trees and symbol tables to analyze source code and generate machine code.
- Operating Systems: Operating systems use various data structures such as arrays, linked lists, and trees to manage system resources such as processes, files, and memory.
Data structures are an essential part of computer engineering projects, providing efficient ways to organize and store data in programs. By choosing the right data structure for your problem, using abstract data types, minimizing indirection, using iterators correctly, testing your data structure thoroughly, and following best practices for specific data structures, you can write efficient, scalable, and maintainable code that meets your requirements.
In conclusion, this article has provided an overview of the importance of data structures in computer engineering projects and best practices for using them effectively. By following these guidelines and staying up-to-date with industry trends and advancements in data structures, you can develop high-quality software systems that meet your needs efficiently and effectively
Related Courses and Certification
Also Online IT Certification Courses & Online Technical Certificate Programs