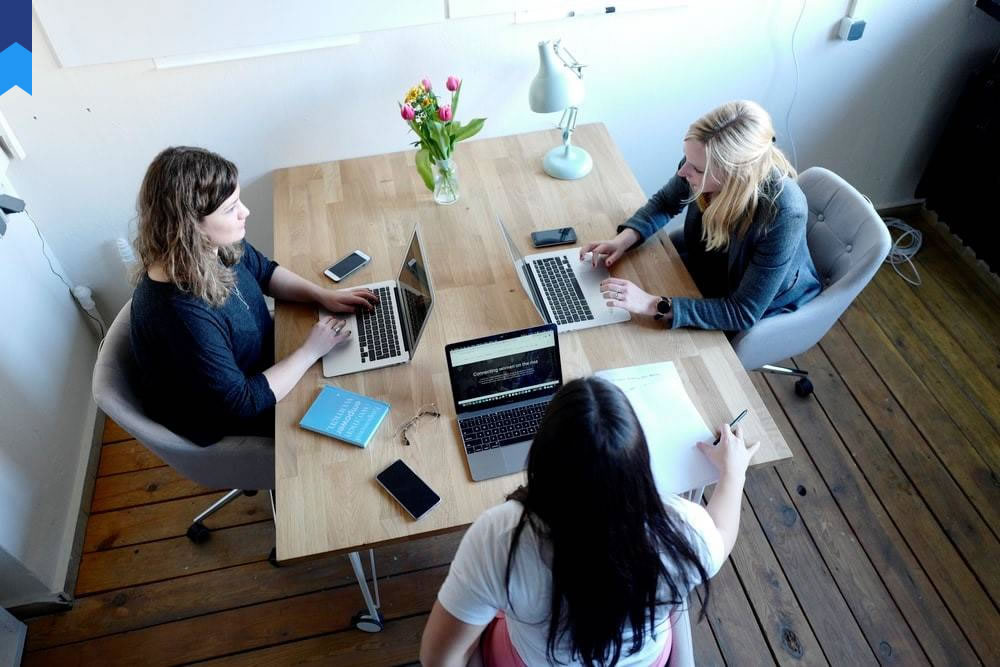
Conquer ASP.NET Headaches: 5 Advanced Strategies for Seamless Development
ASP.NET development, while powerful, often presents unique challenges. This article delves into five advanced strategies to overcome common hurdles, transforming frustrating experiences into streamlined, efficient workflows.
Mastering Asynchronous Programming for Enhanced Responsiveness
Asynchronous programming is crucial for building responsive ASP.NET applications. It allows your application to handle multiple requests concurrently without blocking the main thread. This is especially important in applications with long-running operations, such as database queries or file uploads. Consider a scenario where a user uploads a large file. Without asynchronous programming, the entire application would freeze until the upload completes, leading to a poor user experience. By using asynchronous methods, the upload can occur in the background, allowing the application to remain responsive to other user requests. This results in a significant performance improvement, particularly in high-traffic environments. Implementing asynchronous programming correctly requires understanding the nuances of async and await keywords, along with proper error handling and task management. One common pitfall is neglecting to properly handle exceptions within asynchronous operations, which can lead to unexpected application behavior or crashes.
Case Study 1: A large e-commerce platform implemented asynchronous programming for their image upload feature. This resulted in a 40% reduction in average page load time and a 20% increase in user satisfaction.
Case Study 2: A financial institution used asynchronous programming to process real-time stock updates. This allowed them to process millions of updates per second without impacting the performance of their core application.
Proper exception handling is paramount in asynchronous operations. Unhandled exceptions can silently fail, making debugging difficult. Utilizing `try-catch` blocks within async methods is crucial to gracefully handle potential errors. Furthermore, leveraging the Task.ContinueWith method allows for chaining asynchronous operations, ensuring that subsequent tasks only execute upon successful completion of preceding ones. Another vital consideration is the efficient management of tasks. Poorly managed tasks can lead to resource exhaustion and application instability. ASP.NET offers built-in features and tools for managing task lifecycles, which can significantly aid in creating robust asynchronous applications.
Careful planning of asynchronous operations, particularly in complex scenarios involving multiple dependencies, is vital. Thorough testing and performance analysis are crucial to validate the effectiveness of asynchronous programming implementations. Using profiling tools to pinpoint performance bottlenecks can help optimize asynchronous code further, ensuring maximal responsiveness. Moreover, understanding the impact of asynchronous operations on database interactions is important; inappropriate asynchronous calls to databases might not yield expected performance gains and can lead to unexpected behavior.
Conquering Dependency Injection for Maintainable Code
Dependency Injection (DI) is a design pattern that promotes loose coupling and testability. In ASP.NET, DI simplifies the management of dependencies between different components. Instead of directly instantiating classes, you inject dependencies through constructors or properties. This allows for easier testing, mocking, and swapping implementations. For instance, imagine a component that requires access to a database. With DI, you inject a database interface, allowing you to easily swap the real database implementation with a mock version for testing. This enables you to thoroughly test your code without requiring a live database connection, dramatically speeding up the testing process.
Case Study 1: A large banking application migrated to DI, resulting in a 30% decrease in testing time and a 15% increase in code coverage.
Case Study 2: A social media platform used DI to easily swap different logging providers, allowing them to adapt to changing infrastructure needs without significant code modifications.
Proper implementation of DI involves understanding the different types of DI containers and their capabilities. Popular options include Autofac, Ninject, and Microsoft's built-in DI container. Each container offers unique features and configurations, influencing the overall architecture and performance of the application. Choosing the right DI container depends on the project's specific needs and complexity. Furthermore, effective use of DI requires careful consideration of the scope of dependencies. Understanding the differences between transient, singleton, and scoped lifetimes is vital to prevent unexpected behavior and ensure efficient resource management. Each scope has implications for the lifecycle of the injected dependencies and how they are shared across the application.
Beyond basic implementation, advanced techniques like constructor injection and property injection allow for flexibility in handling dependencies. Constructor injection provides greater control over the creation of objects, ensuring all necessary dependencies are available upon instantiation. Property injection offers a more flexible approach, allowing for setting dependencies after the object has been created. However, careful consideration is necessary to avoid potential null reference exceptions. Testing with various mocking frameworks is vital to ensure the proper functioning of the DI implementation and guarantee that dependency injection does not inadvertently introduce unexpected behavior.
Optimizing Database Interactions for Peak Performance
Database interactions are frequently the bottleneck in ASP.NET applications. Optimizing these interactions is crucial for high performance. This involves careful query design, indexing strategies, and connection pooling. For example, using parameterized queries prevents SQL injection vulnerabilities and enhances database performance. Complex queries can often be optimized by using database views or stored procedures, which can reduce the load on the application server and improve overall efficiency. Properly chosen indexes can significantly speed up database queries, especially those involving large datasets.
Case Study 1: An online retailer optimized database queries, resulting in a 50% reduction in database response times.
Case Study 2: A social media platform improved indexing strategies, reducing query execution time by 70%.
Careful consideration of data access patterns is critical for efficient database interactions. Techniques like caching can significantly reduce the load on the database by storing frequently accessed data in memory. This is particularly beneficial for applications with high read-to-write ratios. Selecting appropriate ORM frameworks, such as Entity Framework Core, offers several advantages in managing database interactions. These frameworks can simplify data access and often automate many of the tasks involved in interacting with the database, resulting in increased developer productivity and simplified application management.
Connection pooling is essential for efficient database usage; it avoids the overhead of creating and closing database connections for each request. This improves application responsiveness and reduces the load on the database server. However, effective connection pooling requires careful configuration and monitoring to ensure optimal performance and prevent resource exhaustion. Moreover, monitoring database performance metrics is vital. Using tools to track query execution times, resource usage, and other key performance indicators can highlight potential optimization opportunities and provide valuable insights into the overall health of the application. Regular database maintenance, such as indexing optimization and cleanup of outdated data, is also crucial for optimal performance.
Securing Your ASP.NET Applications Against Vulnerabilities
Security is paramount in ASP.NET development. This includes implementing robust authentication, authorization, and input validation. Employing secure coding practices is fundamental. Regular security audits and penetration testing can uncover vulnerabilities and improve the security posture of the application. Input validation is crucial to prevent SQL injection and cross-site scripting (XSS) attacks, protecting the application from malicious code injection. Authentication mechanisms, such as OAuth 2.0 or OpenID Connect, provide secure ways for users to authenticate and access the application.
Case Study 1: A financial institution implemented robust input validation, preventing a significant data breach.
Case Study 2: An e-commerce platform adopted multi-factor authentication, reducing account compromise by 80%.
Regular security audits are vital to identify and mitigate potential vulnerabilities. Penetration testing, performed by security experts, simulates real-world attacks to pinpoint weaknesses in the application's security architecture. Staying up-to-date with the latest security best practices and patches is essential to mitigate the risk of exploitation of known vulnerabilities. Using a web application firewall (WAF) can provide an additional layer of security by filtering malicious traffic before it reaches the application server. Moreover, implementing proper logging and monitoring can aid in identifying and responding to security incidents promptly.
Using HTTPS to encrypt communication between the client and the server is a fundamental security practice. HTTPS protects sensitive data from eavesdropping and manipulation. Implementing robust authorization mechanisms, ensuring that users only have access to the resources they are authorized to access, is critical. Role-based access control (RBAC) is a common approach for managing authorization in ASP.NET applications. Furthermore, regularly reviewing and updating security policies and procedures is crucial. Security is an ongoing process and not a one-time task, requiring continuous attention and adaptation to the ever-evolving threat landscape.
Leveraging Advanced Caching Strategies for Scalability
Caching is a crucial technique to enhance the scalability and performance of ASP.NET applications. It involves storing frequently accessed data in memory or a dedicated cache, reducing the need to repeatedly fetch data from slower data sources such as databases. Different caching strategies exist, including output caching, data caching, and distributed caching. Output caching stores the rendered output of a page or web service, significantly reducing the server load for subsequent requests. Data caching stores frequently accessed data objects, allowing for faster retrieval compared to querying a database. Distributed caching, using technologies like Redis or Memcached, allows for sharing cached data across multiple servers, improving scalability.
Case Study 1: A news website implemented output caching, resulting in a 60% reduction in server load and a 30% increase in page load speed.
Case Study 2: An e-commerce platform used distributed caching to scale their application to handle peak traffic during holiday sales.
Careful planning of the caching strategy is crucial. Understanding the trade-offs between different caching mechanisms, such as memory usage versus performance gains, is vital. For instance, output caching might reduce server load but could potentially lead to stale data if not properly managed. Data caching can improve performance but requires careful consideration of cache invalidation strategies to ensure data consistency. Distributed caching offers scalability but adds complexity to the system architecture and requires careful planning and configuration. Proper implementation of caching requires attention to several details. Selecting an appropriate caching provider, such as in-memory caching or a distributed cache, is essential based on the specific needs and scalability requirements of the application. Effective cache invalidation strategies are critical to prevent serving stale data to users.
Monitoring the cache hit ratio is important to assess the effectiveness of the caching strategy. A high hit ratio indicates that the caching strategy is successfully reducing the load on backend data sources. Furthermore, regular review and optimization of the caching strategy are important as the application evolves and traffic patterns change. It might be necessary to adjust caching parameters or even switch to a different caching mechanism to optimize performance. Finally, understanding the impact of caching on the application's architecture and how it interacts with other components is necessary for a smooth and efficient implementation.
Conclusion
Overcoming common challenges in ASP.NET development demands a strategic approach. By mastering asynchronous programming, implementing dependency injection, optimizing database interactions, prioritizing security, and leveraging advanced caching, developers can build robust, scalable, and high-performing applications. This article has provided a detailed exploration of these five advanced strategies, equipping developers with the knowledge and best practices needed to navigate the complexities of ASP.NET development and deliver exceptional results. The focus on practical examples and real-world case studies underscores the importance of applying these concepts for tangible performance enhancements and security improvements. By proactively addressing these crucial areas, developers can significantly enhance their project's success and minimize potential setbacks along the way.