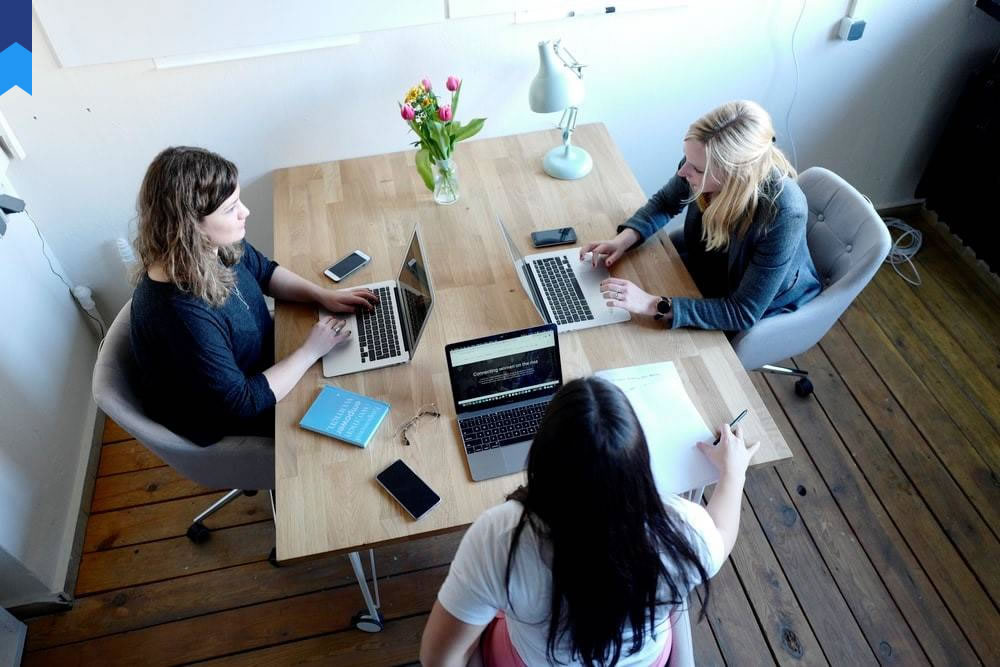
Evidence-Based Flutter Development Strategies
Flutter's popularity continues to surge, making mastering its intricacies crucial for developers. This article delves into specific, practical, and innovative strategies for effective Flutter development, moving beyond basic tutorials and addressing the challenges faced by developers daily.
State Management: Mastering the Art of Data Flow
Effective state management is paramount in Flutter. Choosing the right approach significantly impacts app performance and maintainability. Provider, BLoC, Riverpod, and GetX are popular choices, each with its strengths and weaknesses. Consider the complexity of your application; a simpler app might benefit from Provider's straightforward approach, while a complex application might require the architectural rigor of BLoC or the efficiency of Riverpod. GetX offers a concise syntax attractive to developers seeking faster development cycles.
Case Study 1: A small e-commerce app might find Provider’s simplicity sufficient, managing product details and cart information effectively. The app's smaller scope means the overhead of more complex architectures would be unnecessary. The lightweight nature of Provider also helps minimize app size.
Case Study 2: A large-scale social media application, on the other hand, would benefit from the scalability and testability offered by BLoC. The clear separation of concerns in BLoC aids in maintaining the app's complex state and interactions, particularly crucial for handling extensive user data and numerous UI updates.
Data persistence is another critical aspect of state management. Consider using local databases like SQLite or shared preferences for offline data storage, integrating with cloud databases like Firebase for synchronization and scalability. Choosing the appropriate approach depends on your data size, complexity, and synchronization requirements.
A common mistake is neglecting asynchronous operations during state updates. This can lead to UI freezes and poor user experience. Always employ asynchronous programming techniques, such as `async` and `await`, to prevent blocking the main thread.
Performance optimization is a continuous process. Profiling tools help identify bottlenecks in your app's state management. Careful consideration of the data structures, the use of efficient algorithms, and choosing the appropriate state management solution directly impact the overall performance and user experience.
Effective state management is not a one-size-fits-all solution; careful evaluation is necessary. Choosing the right approach, optimizing data persistence, and managing asynchronous operations are vital for creating a high-performing and maintainable application.
The selection of the correct state management solution is crucial; each framework presents advantages and disadvantages that should be considered in terms of app complexity, team expertise, and long-term maintenance. Improper state management can lead to bugs, performance issues, and decreased developer productivity.
Regularly reviewing and refactoring your state management solution is essential, especially as your application grows. This proactive approach prevents future technical debt and ensures the maintainability of your project. Continuous improvement and adaptation to changing needs are crucial for long-term success.
UI/UX Optimization: Crafting Seamless User Experiences
A visually appealing and intuitive user interface is crucial for user engagement. Leverage Flutter's rich widget library to create a visually appealing and responsive design. Consider utilizing readily available packages to speed up the development process and ensure consistency.
Case Study 1: An application focusing on data visualization can greatly benefit from utilizing custom charts and graphs. This might necessitate using packages for creating interactive elements. The user experience is directly tied to the ease of understanding and interaction with the presented information. Proper usage of visuals enhances the understanding of the data.
Case Study 2: A news app should prioritize clear and concise display of information, utilizing efficient layouts and minimizing visual clutter. News articles should be easy to read, images should load quickly, and navigation should be simple and intuitive.
Accessibility is paramount. Ensure your app adheres to accessibility guidelines, making it usable for everyone regardless of their abilities. This involves providing alternative text for images, using sufficient color contrast, and implementing keyboard navigation.
Animations and transitions can dramatically improve user experience, enhancing engagement and providing visual feedback. However, overuse can lead to performance issues; use them judiciously and with consideration for the overall visual aesthetic. Animations should serve a purpose; they should not be solely for decoration.
Performance profiling is critical for identifying and resolving performance bottlenecks. Tools like the Flutter DevTools help in isolating areas of improvement. Optimizing image loading, using efficient widgets, and minimizing unnecessary rebuilds can significantly enhance performance.
Testing the UI/UX design is essential for identifying usability issues before the app is released. User testing is a valuable tool for gaining insights into how real users interact with your application. Feedback helps refine the design and enhances the overall user experience.
UI/UX design must constantly evolve; regular updates and improvements based on user feedback and new design trends are vital. Following best practices ensures that the app remains current and appealing, thus enhancing long-term user engagement.
Efficient layout management is crucial for responsive design. Using Flutter's layout widgets effectively enables adaptation to different screen sizes and orientations. This is critical for broad accessibility and positive user experience across a wider range of devices.
Testing and Deployment Strategies: Ensuring Quality and Reliability
Thorough testing is crucial for building high-quality Flutter applications. Implement a comprehensive testing strategy encompassing unit tests, widget tests, and integration tests to ensure the reliability and stability of your application.
Case Study 1: A financial application requires rigorous testing to ensure accuracy in calculations and data handling. Unit tests focus on individual functions, widget tests check UI components, and integration tests verify the interaction between different components.
Case Study 2: A gaming application might incorporate performance testing to assess frame rate and responsiveness under various conditions. This helps prevent performance issues that can lead to negative user feedback and low engagement.
Continuous integration and continuous deployment (CI/CD) pipelines streamline the development and deployment process, ensuring regular and efficient releases. This facilitates faster iteration and quicker delivery of features and updates to users.
Choosing the right deployment platform is essential. Consider factors such as platform support, audience reach, and deployment cost. Various platforms offer specific advantages and disadvantages; careful consideration is required.
Security is a critical aspect of app development. Implement robust security measures to protect user data and prevent vulnerabilities. This includes data encryption, secure authentication, and regular security audits.
Monitoring the app’s performance in production is vital for identifying and addressing any post-release issues. Gathering user feedback helps in prioritizing improvements and maintaining application quality.
Testing and deployment strategies evolve alongside the development process, adaptation and improvement are essential. Regular reviews and updates to testing procedures and deployment pipelines ensure the long-term stability and security of the application.
Understanding the tradeoffs between various testing approaches is crucial, tailoring the strategy to the specific needs of the project ensures efficient resource allocation and effective quality control.
API Integration and Data Handling: Working with External Services
Effective integration with APIs is fundamental for most Flutter applications. Leverage packages like `http` or `dio` for making HTTP requests and managing API interactions. Always handle potential errors appropriately and implement retry mechanisms to ensure resilience.
Case Study 1: A weather application retrieves weather data from a weather API. Error handling ensures the app gracefully handles network issues and displays appropriate messages to the user. Proper data formatting and display are crucial for optimal user experience.
Case Study 2: An e-commerce application interacts with multiple APIs for product catalogs, user accounts, and payment processing. Efficient data management ensures optimal performance, even with considerable data volume. Security measures are critical to protect sensitive user information.
Data parsing and serialization are essential steps in working with APIs. JSON encoding and decoding are common techniques. Using libraries that streamline these processes enhances efficiency and maintainability.
Caching mechanisms improve performance by reducing the frequency of API calls. This is especially important for applications that frequently access the same data. Appropriate caching strategies ensure that data is updated as needed without excessive API calls.
Asynchronous programming is critical for handling API interactions without blocking the main thread. Using asynchronous techniques like `async` and `await` prevents UI freezes and keeps the user interface responsive.
Security considerations are paramount when interacting with APIs. Handle sensitive data securely and implement appropriate authentication mechanisms to protect against unauthorized access.
Maintaining data consistency across different parts of the application requires careful coordination of data flow and state management. Choosing the right state management solution significantly impacts the efficiency and reliability of data handling.
Proper error handling and reporting mechanisms are vital. This enhances debugging and provides insights into the root causes of issues. Effective error management is critical for maintaining the stability of the application.
Advanced Techniques and Future Trends: Staying Ahead of the Curve
Exploring advanced Flutter techniques like platform channels, integrating with native code, and employing state-of-the-art architectures are crucial for building high-performance, feature-rich applications. Mastering these skills positions developers for success in the constantly evolving landscape of mobile development.
Case Study 1: A high-performance application requiring access to specific hardware features might necessitate platform channels to interact with native modules. This could include accessing the device's camera, sensors, or other hardware functionalities not directly available through Flutter’s core APIs.
Case Study 2: A complex application needing to integrate with legacy systems or specialized libraries might require integrating native code to leverage existing infrastructure and functionality. This could involve porting existing codebases or utilizing existing third-party libraries not readily available for Flutter.
Understanding the nuances of different architectural patterns and selecting the one best suited for the project’s complexity is critical. The choice influences maintainability, scalability, and development speed. Choosing the wrong architectural approach can lead to difficulties in future maintenance and scaling.
Staying updated with the latest trends in Flutter and mobile development is vital. Following relevant blogs, communities, and official documentation ensures developers are informed about new features, best practices, and potential challenges. Continuous learning is essential to adapt to evolving technology.
Performance optimization techniques such as code splitting, lazy loading, and efficient widget usage, combined with the proper application of architectural patterns and data handling strategies, significantly enhance app performance and user experience. Effective optimization strategies minimize app size and improve the overall responsiveness.
Exploring cutting-edge techniques and integrating emerging technologies, such as machine learning and augmented reality, opens doors to innovative app functionalities and features. This proactive approach ensures that applications remain relevant and appealing in a competitive market.
Continuous learning and development are critical; participation in open-source projects, contribution to communities, and engagement in online forums foster growth and keep developers abreast of best practices.
Staying informed about security vulnerabilities and implementing appropriate countermeasures is vital for the protection of user data and the reputation of the application. Proactive security measures ensure compliance with security standards and regulatory requirements.
Conclusion
Mastering Flutter requires more than just understanding the basics. By embracing evidence-based strategies in state management, UI/UX optimization, testing, API integration, and advanced techniques, developers can build high-quality, performant, and scalable applications. This holistic approach, incorporating best practices and continuous learning, ensures success in the dynamic world of Flutter development. The future of Flutter development lies in continuous adaptation and innovation, embracing new technologies and optimizing existing approaches to create exceptional user experiences.
The journey of becoming a proficient Flutter developer is an ongoing process. Continuous learning, participation in the community, and the adoption of advanced techniques contribute to achieving mastery and consistently delivering high-quality applications. The commitment to improvement and a proactive approach are essential for long-term success in this dynamic field.