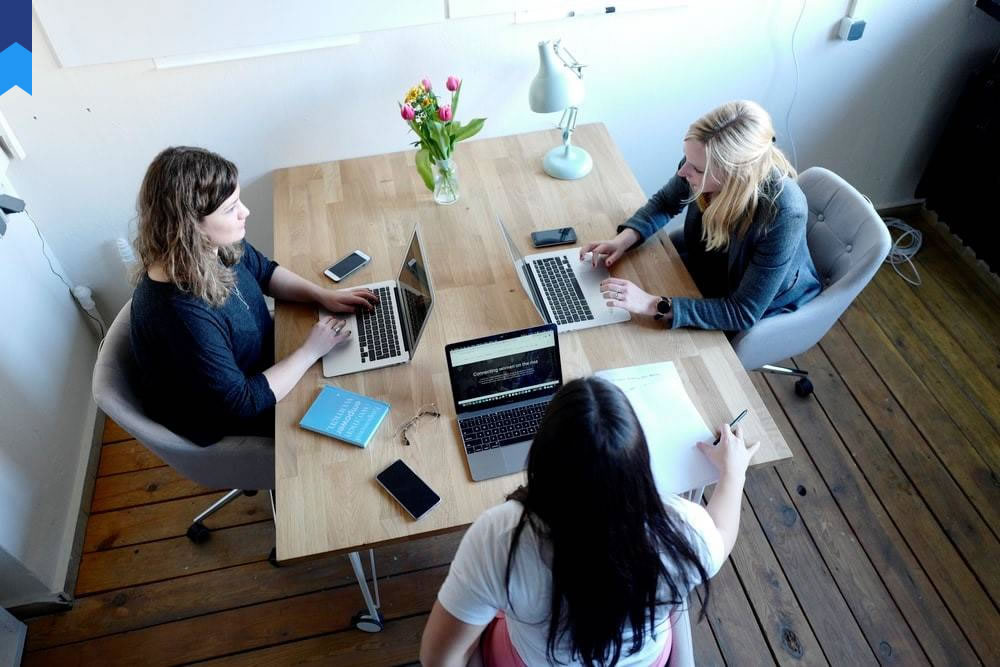
Hidden Truths About ASP.NET Core
Introduction
ASP.NET Core, a powerful and versatile framework, often presents itself as a straightforward path to web development. However, beneath the surface lies a realm of nuanced complexities and unexpected behaviors. This exploration delves into the hidden truths of ASP.NET Core, revealing practical insights and innovative approaches that move beyond the basic tutorials. We will uncover surprising performance bottlenecks, explore unconventional architectural patterns, and reveal hidden features that can significantly enhance your development process. This journey will equip you with a deeper understanding, empowering you to build robust and efficient applications.
Understanding Dependency Injection's Darker Side
Dependency Injection (DI) is a cornerstone of ASP.NET Core, lauded for its modularity and testability. However, misusing DI can lead to significant performance issues and maintainability problems. Over-reliance on DI containers can introduce unnecessary overhead, particularly in high-throughput scenarios. For example, registering too many services without careful consideration can lead to slow application startup times. Efficient use of DI requires strategic decisions about service lifetimes (transient, scoped, singleton) and a conscious effort to avoid excessive nested dependencies. One effective approach is using the constructor injection strategy. A common mistake is to inject dependencies that are not really needed by the dependent object. This unnecessary injection can add to the initialization overhead.
Case Study 1: A large e-commerce application initially used DI for almost every component, leading to slow application startup and performance degradation under heavy load. Refactoring to reduce unnecessary dependencies resulted in a 30% improvement in response times. Case Study 2: A financial services application experienced unexpected errors due to conflicting service registrations. A careful review of DI configuration and implementation improved system stability and predictability.
The Middleware Maze: Unexpected Behavior
Middleware, the building block of ASP.NET Core's request pipeline, is a powerful tool but can quickly become a labyrinth of unexpected behavior if not carefully managed. The order in which middleware executes is critical. Incorrect ordering can lead to logic errors, performance bottlenecks, and security vulnerabilities. For instance, placing authentication middleware after authorization middleware would render authentication useless. A common mistake is to place too many pieces of middleware in the pipeline.
Careful planning and clear documentation of the middleware pipeline are essential. Furthermore, using middleware for tasks it's not designed to handle can lead to unexpected behavior and errors. Avoid using middleware to handle tasks that should be handled by other components of the application. Excessive middleware can significantly impact the efficiency of the pipeline. Case Study 1: A poorly structured middleware pipeline in a social media application led to authentication failures and reduced performance. Reorganizing and simplifying the middleware stack resolved the issue. Case Study 2: A banking application experienced security vulnerabilities due to the incorrect placement of security-related middleware within its pipeline. Re-architecting the pipeline fixed this.
Asynchronous Programming Pitfalls
ASP.NET Core embraces asynchronous programming for handling I/O-bound operations. However, improper use of asynchronous patterns can lead to deadlocks, unexpected thread behavior, and decreased performance. Overusing async/await without understanding its underlying mechanisms is often the root cause of problems. For example, using async methods when they are not necessary can lead to unnecessary overhead. Ensure that your async methods actually perform asynchronous operations. If your async method is performing mostly synchronous operations, it is not necessary to mark it as async.
Careful consideration of when and how to utilize async/await is essential. Mixing synchronous and asynchronous operations can lead to unexpected results. The effective use of async/await requires a thorough understanding of the Task and async/await keywords. Be aware that async methods can throw exceptions that are not handled properly. Case Study 1: A poorly implemented asynchronous operation in an online gaming platform caused deadlocks and reduced responsiveness. Addressing the asynchronous programming errors addressed the problem. Case Study 2: An e-learning platform experienced performance issues due to inefficient asynchronous operations. Optimization of their asynchronous methods improved responsiveness.
Configuration Chaos: Managing Secrets
ASP.NET Core's configuration system is powerful, but managing sensitive information like database credentials, API keys, and connection strings requires meticulous attention. Hardcoding secrets directly into your code is a major security risk. Using insecure configuration methods exposes your application to vulnerabilities. Proper management of secrets requires careful consideration and implementation of robust security measures.
Utilizing secure configuration providers, such as environment variables or dedicated secret management services, is crucial. Implementing proper access controls and adhering to best practices for secret management is essential for protecting sensitive information. The use of environment variables is an effective way to manage sensitive data. For example, by using environment variables to store your database connection strings, you can avoid having to store this information directly in the configuration file. Case Study 1: A news website experienced a data breach due to insecure storage of database credentials in their configuration file. Implementing a more secure configuration approach would prevent this. Case Study 2: An online retailer suffered a financial loss due to exposed API keys in their source code. Implementing secure key storage is important to avoid this.
Caching Strategies and Their Consequences
Effective caching can dramatically improve ASP.NET Core application performance, but improper caching strategies can lead to data inconsistencies and unexpected behavior. Choosing the right caching mechanism—in-memory, distributed, or hybrid—depends on the application's specific needs and data characteristics. Understanding the trade-offs between cache invalidation strategies and data consistency is critical. Improper cache invalidation can lead to stale data and unexpected application behavior.
Implementing a robust caching strategy involves careful planning and execution. Consider the impact of cache invalidation strategies on your application's behavior. For example, the use of a time-based invalidation strategy can ensure data consistency. However, it is important to note that this approach can lead to higher cache miss rates. Case Study 1: A social media platform experienced performance issues due to a poorly implemented caching strategy that resulted in high cache miss rates. A well-planned caching strategy addressed the issue. Case Study 2: An e-commerce site experienced data inconsistencies due to improperly handled cache invalidation. Careful planning of the caching and invalidation strategy would solve this.
Conclusion
ASP.NET Core's power and flexibility come with hidden complexities. Understanding these subtle aspects – from dependency injection intricacies to the pitfalls of asynchronous programming and the crucial role of secure configuration and caching – is vital for building robust, high-performing, and secure applications. By addressing these often-overlooked areas, developers can unlock the framework's full potential, creating applications that not only function flawlessly but also stand the test of time. The journey of mastering ASP.NET Core is an ongoing process of learning and adapting to the nuances of the framework. Continuous learning and adaptation are crucial for successful development.