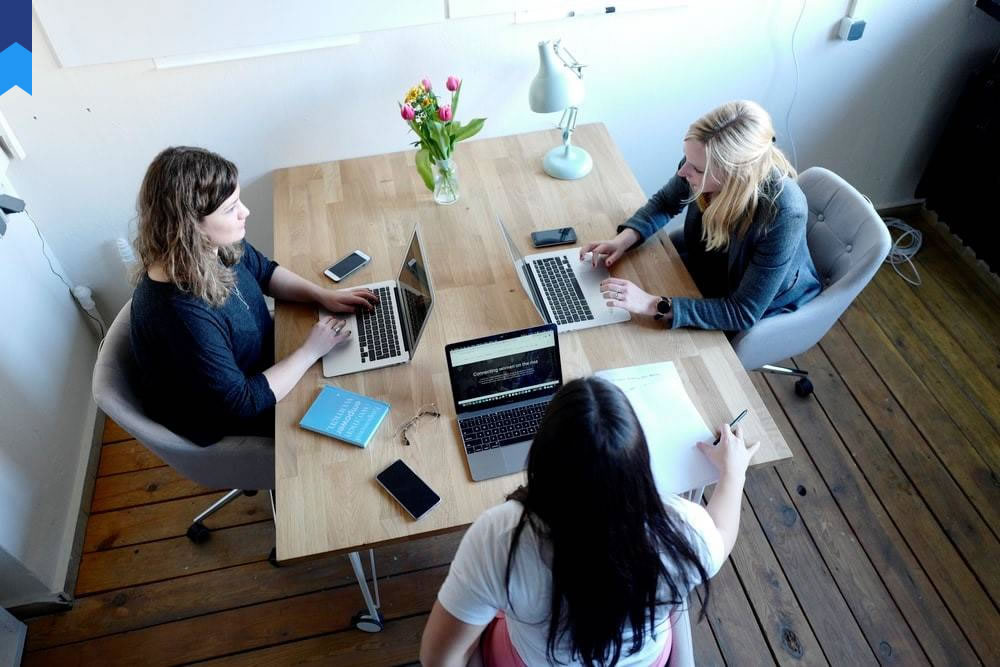
How Effectively Can You Structure BackboneJS Applications?
Efficiently managing complexity is paramount when building robust and scalable applications. Backbone.js, despite its age, remains a powerful framework for structuring JavaScript applications, offering a clear MVC architecture. However, effectively leveraging its features requires a nuanced understanding of its strengths and limitations. This article delves into practical strategies and innovative approaches to master Backbone.js application structuring, exploring techniques beyond the rudimentary.
Understanding Backbone.js's MVC Paradigm
Backbone.js’s Model-View-Controller (MVC) architecture provides a fundamental structure for separating concerns within an application. Models represent data, Views handle presentation, and Controllers mediate interactions between Models and Views. However, effectively using this architecture goes beyond simply creating these components. Careful consideration of data relationships, event handling, and the overall application flow is crucial.
Consider a case study involving a task management application. The model might represent a single task with attributes like title, description, and due date. The view could be a simple list item displaying task details. The controller would handle user interactions, such as adding, deleting, or marking tasks as complete. A more complex application could involve nested models and collections for efficient data management. For instance, a project model might contain a collection of task models, improving organization and data integrity.
Another example involves an e-commerce platform. Product models hold details like name, price, and description, while order models track purchases. Views could display product catalogs, individual product pages, and shopping carts. Controllers would handle adding items to the cart, processing payments, and managing user accounts. This layered approach keeps the codebase clean and maintainable, especially as the application scales. Efficient data synchronization across various views is essential, often involving custom events and Backbone's event system.
Effective use of Backbone.js's events is critical. Custom events allow components to communicate effectively without tightly coupling them. This loose coupling enhances modularity and simplifies testing. Careful design of event names and event handlers is important to avoid confusion and maintain a clear flow of information. Proper error handling within event handlers is also essential for a robust application. A real-world example is a social media application; user updates trigger events that update multiple views, such as a newsfeed and a profile page. The event system ensures that these views are updated consistently and efficiently.
Furthermore, Backbone.js offers excellent extensibility through custom events. This allows developers to create their own custom events and handlers tailored to specific application needs. This feature enhances the framework's adaptability and makes it suitable for a wide array of applications. By leveraging custom events effectively, developers can create more modular and maintainable code. This also fosters collaboration within development teams. A social networking app, for example, could use custom events for friend requests, notifications, and other interactions.
Optimizing Collections and Models
Backbone.js Collections and Models are fundamental building blocks. However, their efficient use is crucial. Models should be designed with appropriate attributes and methods. Overly complex models should be refactored into smaller, more manageable units to improve maintainability. This approach ensures that the code remains organized and easy to understand. Careful consideration of relationships between models is crucial for data integrity. Using Backbone.js's relational capabilities effectively is key to avoiding redundant data and maintaining consistency.
One case study example involves a customer relationship management (CRM) application. A customer model might have attributes like name, address, and contact information. A related order model could link to the customer. This relational structure prevents data duplication and ensures consistency across different parts of the application. It's also essential to use appropriate validation rules on models to prevent inconsistent data from entering the system. Proper validation reduces the risk of errors and improves data quality. The CRM could also involve models for sales representatives and products, further showcasing relational efficiency.
Another example is a blogging platform. A blog post model could have attributes like title, content, and author. A user model could represent the author. The relationship between the blog post and the author is crucial for proper attribution and data management. Careful design of model relationships prevents issues with data integrity and simplifies data access. Similarly, comments on blog posts could be modeled as a separate collection linked to the blog post, forming another crucial relational aspect.
Efficient use of Backbone.js collections is just as important. Collections provide a structured way to manage lists of models. Proper use of collection methods, such as `fetch`, `add`, and `remove`, is crucial for maintaining data integrity and performance. Overusing collection methods can impact performance. Understanding collection methods' implications is crucial. Effective use of filters and sorting methods within collections improves application efficiency. This ensures that only necessary data is fetched and processed, reducing application load times.
For instance, a news aggregator application could use collections to manage news articles. Collections provide methods for efficiently filtering, sorting, and displaying news articles based on various criteria. The application could use different collections for different news categories, enhancing organization and efficient data access. This organization also allows for the implementation of specific sorting and filtering rules per category.
Implementing Router and Navigation
Backbone.js’s Router facilitates client-side routing, enabling seamless navigation within the application. However, effectively structuring routes is crucial for maintainability and scalability. Routes should be designed with a clear and consistent structure, reflecting the application's navigation flow. The router should be well-organized to reflect the application's structure. Proper organization ensures that the routing logic remains clear and understandable. Using named routes enhances readability and maintains a consistent naming scheme across the routes.
One example is a single-page application (SPA) for a blog. The router could handle routes like `/posts`, `/posts/:id`, and `/about`. The route `/posts` could display a list of blog posts, while `/posts/:id` could display a single blog post. The route `/about` could display an "about" page. This structure makes the routing logic intuitive and easy to maintain. Additionally, handling routes for different content types (e.g., blogs, images, videos) showcases organized routing.
Another example involves an e-commerce website. Routes could include `/products`, `/products/:id`, `/cart`, and `/checkout`. The route `/products` could display a catalog of products, while `/products/:id` could display a specific product's details. The routes `/cart` and `/checkout` would handle the shopping cart and checkout process. This organization streamlines user experience and enables straightforward code management, enhancing maintainability.
Effective use of Backbone.js events within the router is essential. Events allow the router to communicate with other components, such as views and models. This communication ensures that the application state is updated consistently. The router should also implement proper error handling to gracefully manage navigation errors. Thorough error handling ensures the application remains stable even in unexpected situations. This robust error management ensures a consistent user experience.
Furthermore, for complex applications, consider using a dedicated routing library in conjunction with Backbone.js. Libraries like React Router or similar can provide advanced features like nested routing and dynamic route generation. This enhances flexibility and scalability for large applications. Integration with other frameworks extends functionality and improves performance significantly.
Leveraging Advanced Techniques
Beyond the basics, several advanced techniques enhance Backbone.js application development. These include utilizing Marionette.js, a comprehensive framework built upon Backbone.js, which provides additional structure and features. Marionette.js offers advanced components like Regions and Layouts, enabling more complex application architectures. It also provides tools for managing application state and handling complex interactions. Integrating Marionette.js introduces a significant layer of organization.
One case study involves a large-scale enterprise application. Marionette.js's Regions and Layouts provide a more organized approach to managing the application's UI components compared to using only basic Backbone.js views. This improved organization is crucial for maintaining a consistent and scalable architecture. This structure also facilitates easier testing and debugging of individual components.
Another example is a complex dashboard application. Marionette.js facilitates the creation of reusable UI components that can be easily integrated into different sections of the dashboard. This reusability saves development time and improves the application's overall consistency. The structured layout provided by Marionette.js simplifies the complexity of handling multiple interacting components within the dashboard.
Another advanced technique is to employ a state management library. Libraries like Redux or MobX can manage the application's state effectively, especially in larger applications. These libraries enhance the application's predictability and testability. Efficient state management simplifies interactions between components and enhances overall application robustness.
Furthermore, consider using a build system like Webpack or Parcel to manage dependencies and optimize the application's performance. A build system streamlines the development workflow and enables code splitting for improved loading times. These systems also facilitate modular code organization.
Conclusion
Backbone.js, while a mature framework, continues to be a powerful tool for building well-structured JavaScript applications. Mastering its intricacies, however, requires more than just understanding the basics. By carefully considering model relationships, event handling, routing strategies, and leveraging advanced techniques like Marionette.js or state management libraries, developers can unlock Backbone.js's full potential. Effective application of these principles leads to cleaner, more maintainable, and scalable applications. This attention to detail contributes to a smoother development process and superior user experiences. Choosing the right tools and techniques based on project needs is crucial for successful implementation.