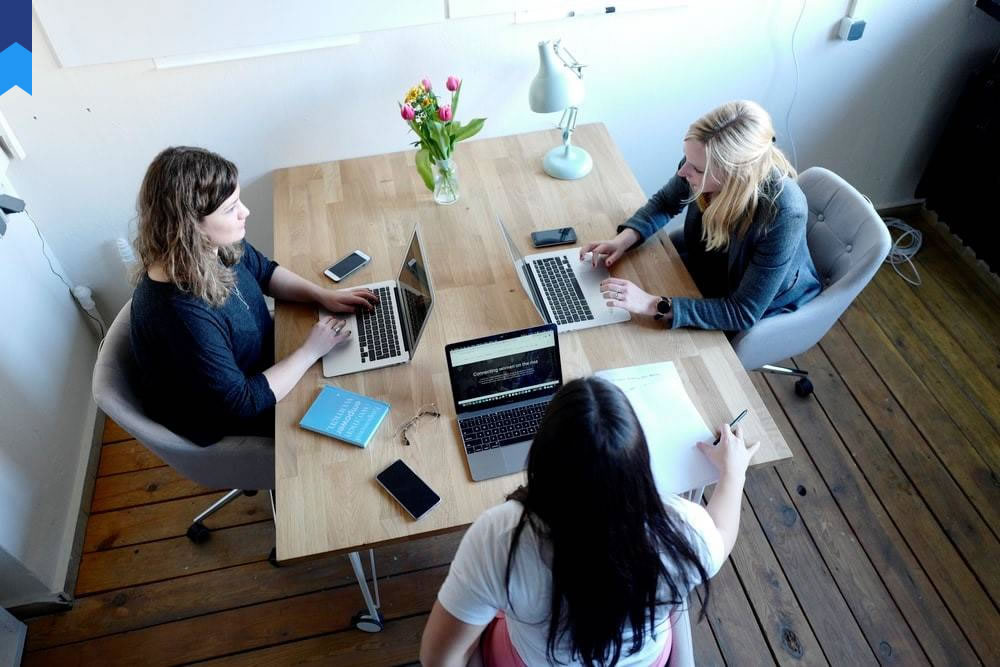
How Effectively To Build Robust MVC Applications With ASP.NET
Efficiently crafting robust and scalable applications using ASP.NET MVC demands a deep understanding beyond the basics. This article delves into specific, practical, and innovative techniques, challenging conventional wisdom and offering unexpected angles to elevate your ASP.NET MVC development skills.
Understanding the MVC Architecture
The Model-View-Controller (MVC) architectural pattern is a cornerstone of modern web development. It separates concerns into three interconnected parts: the model (data and business logic), the view (user interface), and the controller (handling user input and updating the model). A strong grasp of these components is fundamental to building a maintainable and extensible application. Consider a simple e-commerce application. The model would handle product information, database interactions, and order processing. The view would display product details and the shopping cart. The controller would manage user actions like adding items to the cart and processing payments. Effective use of MVC ensures that changes in one component don't necessitate widespread modifications in others. For instance, updating the database structure (model) should ideally not require altering the user interface (view) significantly. This decoupling promotes modularity and ease of maintenance.
Furthermore, understanding dependency injection becomes crucial in achieving loose coupling within the MVC architecture. Dependency injection helps reduce tight coupling between classes, making testing and maintenance significantly easier. A well-structured ASP.NET MVC application utilizes dependency injection to provide services and dependencies to controllers and other components. This enhances testability, as you can easily mock dependencies during unit tests. For example, instead of a controller directly creating an instance of a data access layer, dependency injection would provide that instance. This allows for easy substitution of mock data access layers during testing, ensuring thorough testing of the controller's logic independent of the data access specifics. A common framework used for dependency injection in ASP.NET MVC is Unity or Autofac. Proper use of these frameworks allows for clean, maintainable code.
Case Study 1: A large-scale e-commerce platform initially struggled with maintainability due to tightly coupled code. Refactoring the application to utilize dependency injection and a well-defined MVC structure significantly improved the development process, allowing for quicker feature implementation and easier bug fixes. The result was a more responsive and robust platform capable of handling high traffic loads. Case Study 2: A social media application experienced performance bottlenecks because of inefficient data access. By optimizing the model and integrating a caching mechanism, developers reduced database queries and significantly improved the application's responsiveness. This demonstrates the importance of efficient model design in a high-traffic environment. Proper implementation of these concepts, however, requires careful planning and understanding of the application's requirements.
Advanced techniques such as employing asynchronous programming (async/await) are invaluable for improving responsiveness, particularly in scenarios involving I/O-bound operations. In ASP.NET MVC, asynchronous methods prevent blocking the main thread while waiting for long-running tasks such as database queries or external API calls. This prevents application freezes and enhances user experience. However, care needs to be taken when implementing asynchronous programming to avoid introducing complexities such as deadlocks. Properly handling exceptions and error conditions in asynchronous code is also critical. The benefits of asynchronous programming are particularly apparent in high-traffic scenarios where concurrent requests are common. A well-designed asynchronous controller action can handle numerous requests simultaneously without impacting the overall application performance.
Mastering Data Handling and Validation
Efficient data handling and robust validation are crucial for building secure and reliable ASP.NET MVC applications. Employing techniques such as object-relational mappers (ORMs) like Entity Framework simplifies database interactions and promotes data integrity. ORMs abstract away much of the underlying database complexities, allowing developers to focus on business logic rather than SQL queries. However, ORM usage necessitates understanding its limitations and potential performance implications. Inefficient ORM usage can lead to performance bottlenecks, especially in scenarios dealing with large datasets or complex queries. Proper indexing and query optimization are therefore necessary to mitigate these issues. Furthermore, raw SQL queries may sometimes be necessary for highly optimized performance.
Validation, both client-side and server-side, is vital for maintaining data quality and preventing security vulnerabilities. Client-side validation enhances user experience by providing immediate feedback on invalid input. However, it's critical to realize that client-side validation shouldn't be solely relied upon, as it can be easily bypassed. Server-side validation ensures data integrity and prevents malicious data from entering the application. Implementing robust server-side validation using ASP.NET MVC's built-in features, like data annotations, is crucial for safeguarding against data manipulation and injection attacks. Validation rules should be comprehensive, covering all possible scenarios and incorporating both basic and more intricate validation checks such as regular expressions for complex patterns or custom validation logic.
Case Study 1: A banking application experienced a security breach due to inadequate server-side validation, allowing users to manipulate input fields and gain unauthorized access to accounts. Implementing stricter server-side validation significantly improved the application's security. This highlights the importance of rigorous validation practices to prevent security risks. Case Study 2: An e-commerce platform improved its data quality and reduced errors by implementing robust validation rules for customer data input, such as email address validation and data type checks. This reduced the number of support tickets related to incorrect user data. Efficient validation leads to cleaner data and improved application reliability.
Consider using a combination of data annotation attributes and custom validation methods for a comprehensive approach to validation. Data annotation attributes provide a declarative way to specify validation rules directly within the model class. Custom validation methods allow for more complex validation logic that cannot be easily expressed using data annotations. This layered approach gives developers flexibility and ensures a high level of validation rigor. It's important to handle validation errors gracefully, providing informative error messages to the user and guiding them towards correcting the input. Clear and user-friendly error messages are crucial for a positive user experience.
Implementing Security Best Practices
Security is paramount in any web application. ASP.NET MVC provides numerous features to secure your applications, but implementing them correctly is essential. The use of authentication and authorization mechanisms is vital to control user access to application resources. ASP.NET MVC integrates seamlessly with various authentication providers, including Windows Authentication, Forms Authentication, and OAuth 2.0. Choosing the right authentication method depends on application requirements and security policies. For example, Forms Authentication is suitable for web applications requiring user credentials while OAuth 2.0 is often used for third-party application integration.
Authorization controls access to specific resources within the application. Role-based authorization is a common approach where users are assigned to roles, and access is granted or denied based on those roles. ASP.NET MVC offers declarative authorization using attributes, allowing developers to easily restrict access to controllers and actions. However, using custom authorization filters can extend these capabilities to handle more complex scenarios. Custom authorization filters allow for creating flexible authorization rules tailored to specific application needs. They allow for checking various conditions before allowing access, such as checking user permissions or validating claims.
Case Study 1: A social networking site implemented robust authorization rules to prevent unauthorized access to user profiles and private data. This protected user privacy and maintained the integrity of the platform. Careful consideration of various security levels and authorization schemes is important. Case Study 2: An online banking application utilized multi-factor authentication to enhance security and prevent unauthorized access to user accounts, protecting sensitive financial information. Multi-factor authentication is a powerful method to improve application security. Regular security audits and penetration testing are essential to identify and address potential vulnerabilities before they can be exploited.
Input validation and sanitization are crucial steps in preventing injection attacks. Never trust user input. Always validate and sanitize all user inputs before using them in database queries or other sensitive operations. ASP.NET MVC's built-in model binding handles some input validation, but it's essential to add additional layers of validation to ensure complete security. Parameterized queries are crucial to prevent SQL injection attacks. Instead of directly embedding user input into SQL queries, use parameterized queries to separate data from the SQL code. This prevents malicious SQL code from being executed.
Leveraging Advanced Features and Techniques
ASP.NET MVC offers advanced features that can significantly enhance application performance, scalability, and maintainability. Asynchronous controllers, discussed earlier, are crucial for handling I/O-bound operations efficiently, preventing blocking and ensuring responsiveness. Caching strategies can further optimize application performance by storing frequently accessed data in memory or other storage mechanisms. Output caching stores rendered views, reducing server load and improving response times. Data caching stores data retrieved from the database or other sources, reducing database queries. Proper caching implementation requires careful consideration of data consistency and expiration policies. A well-designed caching strategy can considerably boost application performance.
Utilizing areas in ASP.NET MVC promotes modularity and organization, particularly in large applications. Areas allow for separating an application into logical modules, each with its own controllers, views, and models. This enhances maintainability and collaboration, enabling multiple teams to work on different parts of an application simultaneously without interfering with each other. Each area operates relatively independently, making it easier to maintain and update specific parts of the system. A well-defined area structure greatly improves code organization and enhances maintainability. It is particularly useful for large, complex applications.
Case Study 1: A large enterprise application employed areas to separate different modules such as user management, product catalog, and order processing. This improved code organization, facilitating concurrent development and simplifying maintenance. Areas enhance code organization significantly. Case Study 2: An e-commerce platform used output caching to reduce server load during peak traffic periods, significantly improving response times and user experience. Efficient caching is extremely important for high-traffic applications.
Employing a robust logging mechanism is essential for troubleshooting and monitoring application behavior. ASP.NET MVC integrates with various logging frameworks, such as log4net and NLog, allowing developers to track application events, errors, and performance metrics. Logging provides valuable insights into application behavior, helping identify and resolve issues quickly. A comprehensive logging strategy is crucial for maintaining a healthy and robust application. Well-structured logs allow for easy troubleshooting and facilitate efficient identification of application bottlenecks. Using logging effectively is paramount for post-deployment monitoring and problem analysis.
Conclusion
Building robust and scalable ASP.NET MVC applications requires a thorough understanding of the framework's intricacies and best practices. Moving beyond basic tutorials and mastering techniques like dependency injection, asynchronous programming, and robust validation is crucial for creating applications that are not only functional but also maintainable, secure, and performant. By focusing on architectural patterns, security considerations, and advanced features, developers can create high-quality applications that meet the demands of modern web development. Continuous learning and adaptation to emerging trends are essential for staying ahead in the ever-evolving landscape of web development. The principles outlined above provide a solid foundation for building modern, efficient, and secure ASP.NET MVC applications.