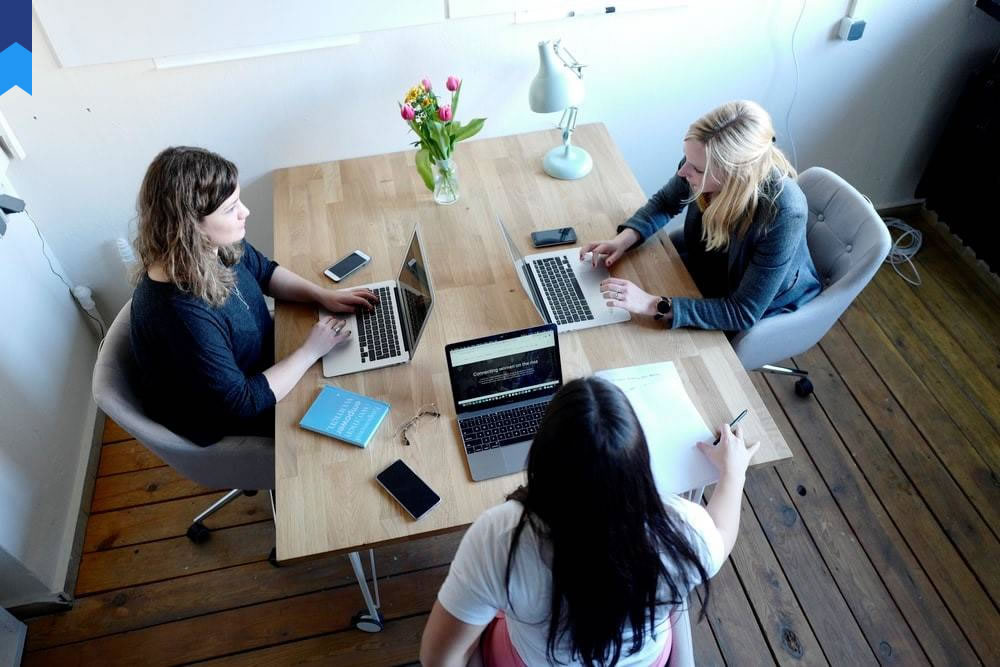
Mastering COBOL File Handling: Techniques And Best Practices
COBOL, despite its age, remains a vital language in many legacy systems. Understanding its file handling capabilities is crucial for maintaining and modernizing these applications. This guide delves into the intricacies of COBOL file handling, providing practical techniques and best practices for efficient data management. We will explore different file types, access methods, and error handling strategies to ensure robust and reliable data processing.
Understanding COBOL File Structures
COBOL supports various file structures, each with its own strengths and weaknesses. Sequential files offer simplicity but lack random access capabilities. Indexed files provide efficient random access through indexing, enabling faster data retrieval. Relative files offer direct access based on record numbers, suitable for applications requiring rapid data access by position. Choosing the right file structure depends on the specific application requirements and performance needs. For instance, a transaction processing system might benefit from indexed files for fast record updates, while a batch processing system might use sequential files for their simplicity. Consider a large insurance company processing millions of policy records: indexed files allow for quick retrieval of specific policy information. In contrast, a payroll system processing a fixed number of employee records might be perfectly suited to sequential files.
Case Study 1: A large banking institution utilized indexed files to manage customer account information, enabling rapid account balance retrieval and transaction processing. The indexed structure significantly improved the system's responsiveness compared to its previous sequential file-based system. Case Study 2: A manufacturing company opted for relative files to track inventory items using the item ID as the record number. This approach enabled quick access to inventory information for real-time production updates and order fulfillment. Understanding the nuances of each file type is critical in selecting the most appropriate method.
Data organization is paramount. Consider the impact of poorly structured data on searching, sorting, updating and even reporting. This can affect overall efficiency and can lead to data inconsistencies or potential errors. Data integrity is crucial; carefully designed data structures minimize these risks. The COBOL language offers options for defining records within files, including structures of varying complexity. Properly defined records facilitate efficient processing and data management.
Effective file handling practices also involve error detection and correction. COBOL provides robust error handling mechanisms, enabling the graceful handling of file-related exceptions, such as attempting to open a non-existent file or encountering a file I/O error. Implementing thorough error handling prevents program crashes and data loss, ensuring reliable operation. Appropriate error messages inform users of problems, allowing for prompt resolution.
Moreover, the efficient use of file buffers can significantly improve the performance of file I/O operations. Understanding how buffers work and optimizing their size and usage can reduce the number of disk accesses, speeding up processing. This is especially crucial when dealing with large files or frequent file access.
Mastering File Access Methods
COBOL offers various file access methods, including sequential, random, and dynamic access. Sequential access reads or writes records in the order they are stored in the file, suitable for batch processing. Random access allows direct access to records based on a key, ideal for applications requiring quick record retrieval or updates. Dynamic access combines elements of sequential and random access, providing flexibility in handling records. Choosing the right access method is crucial for application performance. A system processing daily transactions might use random access to quickly update account balances, whereas a system generating end-of-month reports might utilize sequential access to process records in order.
Case Study 1: A retail company uses random access to update inventory levels in real-time as sales transactions occur. This ensures accurate inventory data is available immediately. Case Study 2: A government agency uses sequential access to process large batches of tax returns, ensuring all returns are processed in order according to their submission date. Careful consideration of these methods leads to a robust and efficient system.
Understanding the implications of each method extends to memory management. The way data is stored and accessed affects system memory. Sequential access, for example, requires less memory in comparison to random access, which relies on indexing mechanisms that can consume more memory. Programmers should carefully consider these factors during system design.
Efficiency in accessing files can be greatly enhanced by employing techniques such as record blocking and buffering. Record blocking groups multiple records together, reducing the number of disk reads and writes, thereby improving performance. Buffering allows temporary storage of records in memory, further reducing disk I/O operations and boosting efficiency. Effective use of these features is crucial for optimal performance.
Furthermore, understanding the various COBOL file-handling verbs, such as OPEN, READ, WRITE, CLOSE, and REWRITE, is critical for program development. Proper usage of these verbs is essential for ensuring correct file handling operations and avoiding common errors. Documentation and clear commenting within code contributes greatly to maintainability.
Implementing Robust Error Handling
COBOL provides numerous ways to handle errors during file operations. The FILE STATUS clause is a critical tool for identifying file-related errors. By checking the file status after each file I/O operation, a program can detect and handle problems such as file not found, end-of-file, or I/O errors. This allows for graceful error recovery and prevents program crashes. Error handling should be comprehensive, encompassing all possible scenarios to avoid unexpected program termination.
Case Study 1: An airline reservation system uses file status checks to ensure that passenger data is correctly read from the file. If an error occurs, a message is displayed and the program attempts to recover gracefully. Case Study 2: A banking application checks file status after each transaction to ensure that all updates are successfully written to the file. If an error occurs, the transaction is rolled back, preventing data corruption.
Beyond the FILE STATUS clause, exception handling routines are critical components of robust error management. These routines provide mechanisms to intercept file-handling exceptions and respond appropriately, which prevents unintended behavior and data inconsistencies. Error logging is essential for tracking incidents and facilitating debugging.
Error handling isn't merely about preventing crashes; it's about building resilience. Effective error handling means anticipating potential problems and designing solutions to minimize disruption to the system. This includes logging and reporting mechanisms to aid in identification and resolution of issues, ensuring the system’s continuity.
Moreover, designing for recoverability is a key aspect of robust error handling. This involves incorporating mechanisms to restore the system to a consistent state in the event of errors, minimizing data loss and ensuring the integrity of the information. Recovery strategies vary depending on the criticality of the data and the application's needs, and should be planned in advance.
Optimizing File I/O Performance
Optimizing file I/O performance is crucial for COBOL applications, particularly those handling large datasets. Techniques such as blocking records, using appropriate buffer sizes, and optimizing file access methods significantly impact efficiency. Understanding how the operating system interacts with files is key to improving performance. Excessive disk I/O can severely hinder application responsiveness. Careful planning of file structure and access methods are critical for performance optimization.
Case Study 1: A logistics company optimized its warehouse management system by carefully selecting file access methods, achieving a 30% improvement in transaction processing speed. Case Study 2: A large retailer improved the performance of its point-of-sale system by implementing record blocking and adjusting buffer sizes, reducing database access times by 20%.
Database indexes are essential when retrieving data from indexed files; they should be properly designed to expedite search operations. Selecting optimal index structures and managing index updates properly greatly influences overall performance and database efficiency.
Memory management plays a crucial role in file I/O efficiency. Efficient use of system memory through effective programming techniques reduces the number of disk accesses, improving system responsiveness and minimizing resource contention. Techniques like buffering reduce the frequency of disk accesses.
Beyond the technical aspects, regular system maintenance is crucial for maintaining peak performance. This includes regular defragmentation of hard drives and timely upgrades to hardware and software, to ensure the system is operating optimally and that it maintains a high level of performance.
Modernizing COBOL File Handling
While COBOL remains relevant, integrating it with modern technologies can significantly enhance its capabilities. Connecting COBOL applications to relational databases through APIs and middleware allows for more flexible data management and integration with other systems. This modernization approach not only improves efficiency but also enables easier data integration with newer technologies.
Case Study 1: A financial institution modernized its COBOL-based core banking system by integrating it with a relational database and modern APIs, significantly improving its scalability and maintainability. Case Study 2: A manufacturing company improved its supply chain management by integrating its COBOL-based inventory system with a cloud-based data analytics platform, enabling real-time data analysis and improved decision-making.
Modernizing doesn’t always mean replacing everything. Refactoring COBOL code to improve readability and maintainability is a crucial aspect of modernization. Refactoring allows for easier future maintenance and reduces the risk of errors. Well-structured code is not only easier to maintain but also allows for simpler integration with other systems.
Modern development practices, such as Agile methodologies and DevOps, can be applied to the maintenance and enhancement of COBOL applications. These practices improve development efficiency and accelerate delivery of improvements. Adopting such methodologies makes the maintenance process far more efficient.
Security is paramount in any system, especially those dealing with sensitive data. Integrating modern security practices, such as data encryption, access controls, and regular security audits, protects data and ensures compliance with regulations. Security measures add significant value to these existing systems.
In conclusion, mastering COBOL file handling involves understanding file structures, access methods, and error handling techniques. Optimizing performance and embracing modernization strategies extend the lifespan and effectiveness of COBOL applications, even as technology continues to evolve. By implementing the best practices outlined in this guide, organizations can ensure the reliability, efficiency, and security of their COBOL systems for years to come.