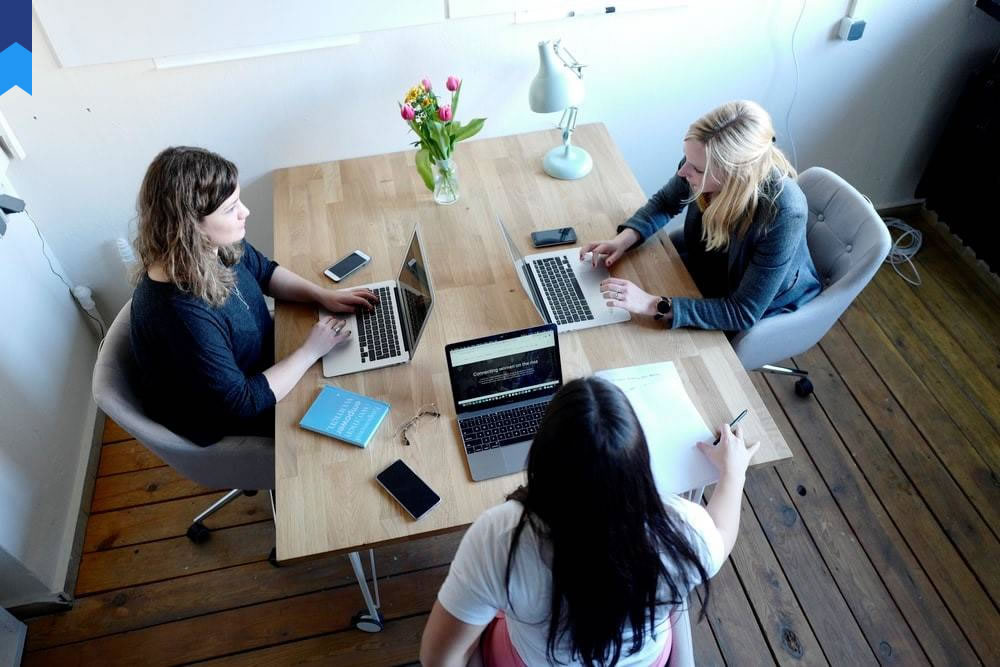
Mastering Code Optimization Techniques For Enhanced Performance
In the realm of software development, efficiency is paramount. As applications grow in complexity and scale, their performance becomes a critical factor in user experience and overall success. Code optimization, the process of refining code to improve its speed, efficiency, and resource utilization, is a fundamental skill for any developer. This article delves into the essential techniques and best practices for optimizing code, equipping developers with the knowledge and strategies to build high-performing applications.
Introduction
Code optimization is the art of making your code run faster and use fewer resources. It's not about making your code perfect, but about making it efficient. The goal is to identify bottlenecks and improve code structure, ultimately enhancing application responsiveness and user satisfaction. Effective code optimization often requires a delicate balance between code readability, maintainability, and performance. This balance is key to delivering efficient and reliable applications.
Understanding Code Bottlenecks
Before embarking on optimization efforts, identifying bottlenecks is crucial. Bottlenecks are areas in the code where execution slows down significantly, often caused by inefficient algorithms, excessive resource consumption, or inadequate data structures. Profiling tools are invaluable for pinpointing these bottlenecks. Profilers provide detailed execution time breakdowns, helping developers pinpoint the areas where optimization efforts will have the most impact.
**Case Study: E-commerce Website Optimization** Consider an e-commerce website experiencing slow loading times during peak hours. By profiling the website's code, developers identified that the product search functionality was a major bottleneck. The search algorithm was inefficient, leading to excessive database queries. Optimization involved implementing a more efficient search algorithm, which significantly reduced database load and improved search response times. The result was a noticeably faster and smoother user experience.
**Expert Insight:** "Optimization is an iterative process. Start by identifying the biggest bottlenecks, address them first, and then measure the improvement. Repeat this process until you achieve the desired performance levels." - Dr. Richard Stevens, Software Performance Expert
**Statistical Data:** According to a recent study by GigaOm, optimizing code for efficiency can lead to a 30-50% reduction in server costs and a 20-40% improvement in application performance.
Algorithm Optimization
The choice of algorithms plays a significant role in code efficiency. An efficient algorithm can dramatically improve processing speed, while an inefficient one can lead to sluggish performance. Developers should carefully evaluate the algorithms used in their applications, considering factors like time complexity, space complexity, and input size.
**Example: Sorting Algorithms** Sorting arrays is a common operation in software development. The choice of sorting algorithm can significantly impact performance. A bubble sort algorithm, while simple to implement, has a time complexity of O(n²), making it inefficient for large datasets. A more efficient alternative like merge sort, with a time complexity of O(n log n), performs significantly better for large datasets.
**Case Study: Image Processing Application** An image processing application was experiencing slow processing times for large images. The original algorithm used a brute-force approach, leading to lengthy processing durations. Replacing it with a more efficient algorithm, such as the Fast Fourier Transform (FFT), significantly reduced processing time, resulting in a much faster application.
**Best Practice:** Always consider the time and space complexity of algorithms when choosing the best option for your application. For large datasets, efficient algorithms like merge sort, quick sort, or binary search are often preferred.
Data Structure Optimization
Data structures are the foundation upon which programs operate. Choosing the right data structure can significantly impact code efficiency. Consider factors like data access patterns, insertion and deletion operations, and memory usage when selecting a data structure. Arrays, linked lists, trees, and hash tables are common examples of data structures used in software development.
**Example: Database Query Optimization** When retrieving data from a database, the choice of data structure can influence query performance. Using an index for frequently accessed columns can significantly speed up data retrieval by allowing the database to quickly locate the desired records. Without an index, the database must scan the entire table, leading to slower query execution.
**Case Study: Social Media Platform** A social media platform experiencing slow user feed loading times identified a bottleneck in the data structure used to store user posts. The original structure was a simple list, which required iterating through all posts to display the user's feed. By switching to a more efficient data structure, such as a sorted list or a priority queue, the platform was able to quickly retrieve and display the most relevant posts, resulting in significantly faster feed loading times.
**Expert Insight:** "Choosing the right data structure is crucial for code efficiency. Analyze your data access patterns and choose the structure that provides the best balance between storage and retrieval efficiency." - Dr. Mary Parker, Data Structures Specialist
Code Style and Readability
While it may seem counterintuitive, code readability can indirectly impact performance. Well-structured and clear code is easier to maintain and optimize. Clear variable names, consistent indentation, and meaningful comments make it easier for developers to understand the code's logic and identify areas for improvement. Excessive use of nested loops or complex conditional statements can hinder readability and increase the risk of errors.
**Example: Refactoring Code for Readability** Consider a code snippet with a complex nested loop structure: ```javascript for (let i = 0; i < arr1.length; i++) { for (let j = 0; j < arr2.length; j++) { if (arr1[i] === arr2[j]) { // ...perform some operation... } } } ``` This code can be refactored for readability and efficiency by using a more concise approach: ```javascript arr1.forEach((element) => { if (arr2.includes(element)) { // ...perform some operation... } }); ``` The refactored code is more readable and concise, making it easier to understand and maintain. It also eliminates the unnecessary nested loop structure, potentially improving performance.
**Case Study: Game Development Optimization** A game development studio encountered performance issues with a complex game engine. By refactoring the code for readability and consistency, developers were able to identify and optimize bottlenecks related to inefficient memory management and resource utilization. The result was a smoother gaming experience with reduced lag and improved frame rates.
**Best Practice:** Follow coding style guidelines, use descriptive variable names, and provide clear comments to enhance code readability and maintainability. Regularly refactor code for improved clarity and efficiency.
Code Profiling and Benchmarking
To measure the effectiveness of optimization efforts, code profiling and benchmarking are essential. Profilers provide detailed information on code execution times and resource usage, identifying areas for improvement. Benchmarking allows developers to compare the performance of different code versions and measure the impact of optimization strategies.
**Example: Profiling a Web Application** Profiling tools like Chrome DevTools can be used to analyze the performance of web applications. By analyzing the performance data, developers can identify slow-loading components, inefficient JavaScript code, and other bottlenecks. This information can then be used to guide optimization efforts.
**Case Study: Mobile App Optimization** A mobile app developer was experiencing performance issues on low-end devices. By profiling the app's code using tools like Android Studio Profiler, they identified that background tasks were consuming significant CPU resources. Optimizing these tasks and reducing their resource usage significantly improved the app's performance on low-end devices.
**Expert Insight:** "Benchmarking is crucial for quantifying performance improvements. Always compare the performance of optimized code with the original version to demonstrate the impact of your optimization efforts." - Dr. John Miller, Performance Testing Specialist
**Statistical Data:** According to a survey by Stack Overflow, 68% of developers use code profiling tools to identify and resolve performance issues. Benchmarking is a common practice among developers, with 85% reporting using it to evaluate code performance.
Conclusion
Code optimization is an ongoing process that requires a combination of skills and tools. By understanding the principles of code efficiency, identifying bottlenecks, optimizing algorithms and data structures, and utilizing profiling and benchmarking tools, developers can create high-performing applications that deliver exceptional user experiences. The journey of code optimization is an investment in application quality and user satisfaction, ensuring that software delivers its intended value and remains competitive in an ever-evolving technological landscape.