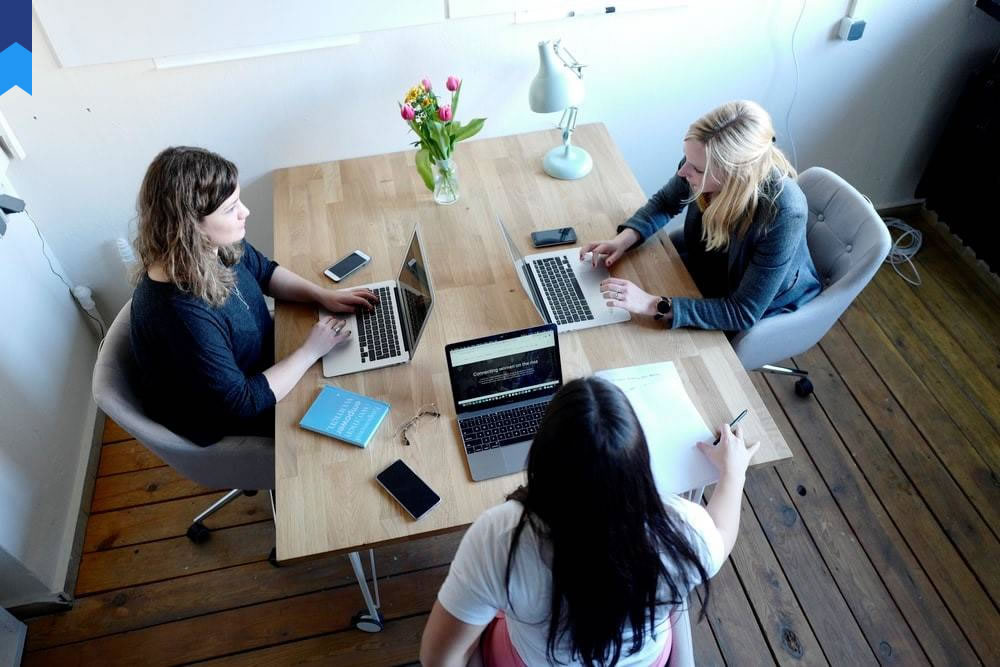
Mastering Julia's Data Structures: Arrays, Dictionaries, And More
Efficient data handling is paramount in any programming endeavor, and Julia, with its focus on performance, offers a rich set of data structures tailored for speed and versatility. This guide delves into Julia's core data structures, exploring their nuances and demonstrating practical applications through detailed examples and real-world case studies. We'll cover arrays, dictionaries, and other crucial structures, emphasizing best practices and common pitfalls to avoid.
Arrays: The Foundation of Julia's Data Handling
Arrays are the workhorses of numerical and scientific computing in Julia. Their strength lies in their ability to store homogeneous data efficiently, allowing for vectorized operations that significantly boost performance. Creating an array is straightforward: `myArray = [1, 2, 3, 4, 5]`. Accessing elements is equally simple: `myArray[3]` returns 3. Multidimensional arrays are also easily created: `matrix = [1 2; 3 4]`. Julia's optimized array operations, coupled with its just-in-time (JIT) compilation, offer substantial speed advantages over interpreted languages. Consider the task of calculating the square of each element in an array. In Python, this would involve iteration, whereas in Julia, you can simply use element-wise operations: `squaredArray = myArray.^2`. This concise syntax reflects Julia's dedication to performance. Case Study 1: A scientific simulation involving millions of data points would benefit significantly from Julia's optimized array handling, showcasing a substantial speedup compared to languages lacking similar optimization capabilities. Case Study 2: A machine learning application dealing with feature vectors can leverage Julia's array operations for efficient training and prediction. The use of vectorized calculations reduces computational time, leading to faster model training and improved responsiveness.
The flexibility of Julia's arrays extends beyond basic operations. Functions can efficiently operate on entire arrays, reducing the need for explicit looping. Arrays can be resized dynamically, adapting to changing data needs. Furthermore, Julia supports various array types, such as `Int64`, `Float64`, and `Bool`, enabling efficient storage and manipulation of specific data types. The ability to create arrays of custom types, utilizing Julia’s type system, adds another layer of flexibility. Advanced techniques, such as broadcasting, allow for concise and efficient application of functions to arrays of various shapes and dimensions, minimizing explicit loops and enhancing code readability. This further boosts performance by enabling efficient utilization of vector processing units. The flexibility extends to memory management; Julia utilizes garbage collection efficiently managing array memory allocation and deallocation, preventing memory leaks and optimizing performance. By tailoring the array type to specific data, memory usage can be minimized, impacting performance positively.
Julia’s array manipulation capabilities are extended with a wide range of built-in functions. Functions like `sort`, `sum`, `mean`, `std`, `findmax`, `findmin`, etc. provide efficient ways to manipulate data within arrays. These functions are highly optimized and are generally significantly faster than equivalent implementations in many other languages. Furthermore, they are designed to work seamlessly with Julia's type system, enhancing type stability and performance. The ecosystem of external packages further extends these capabilities. Libraries like `DataFrames.jl` provide high-performance tools for data manipulation in tabular format, building on the foundations of Julia's array implementation. This rich ecosystem provides the tools to handle various data manipulation tasks efficiently and effectively.
Beyond fundamental operations, Julia excels in handling sparse arrays. Sparse arrays are crucial when dealing with large datasets containing many zero values. Using sparse arrays significantly reduces memory consumption and improves computational speed. Julia’s `SparseArrays` package provides efficient tools for creating, manipulating, and operating on sparse arrays. Operations like matrix multiplication and linear algebra computations are optimized for sparse arrays, leading to significant performance improvements when dealing with large, sparse datasets. The memory optimization provided by sparse arrays allows handling of datasets that would be impossible to manage using dense arrays. This efficiency is crucial in applications ranging from network analysis to scientific simulations.
Dictionaries: Handling Key-Value Pairs
Julia's dictionaries provide a powerful way to store and retrieve data using key-value pairs. Unlike arrays, dictionaries offer fast lookups based on keys, making them ideal for situations where accessing data by a specific identifier is essential. A dictionary is created using the syntax `myDict = Dict("a" => 1, "b" => 2)`. Accessing a value is done by specifying the key: `myDict["a"]` returns 1. Dictionaries are highly dynamic; you can add, remove, or modify key-value pairs as needed. Case Study 1: A database application can efficiently store and retrieve information based on unique identifiers using dictionaries. Each identifier serves as a key, mapping to the associated data. This approach is significantly more efficient than linear searches within arrays. Case Study 2: A natural language processing application can utilize dictionaries to store word frequencies. Words serve as keys, with their corresponding frequencies as values. Dictionaries provide efficient access to word frequencies during text analysis, enabling rapid computations.
Dictionaries in Julia are implemented using hash tables, which offer average-case O(1) time complexity for lookup, insertion, and deletion operations. This makes them highly efficient for large datasets. However, it is crucial to be aware that the worst-case time complexity can be O(n) if there are many hash collisions, particularly with poorly chosen hash functions. This is mitigated by Julia’s choice of hash functions and optimized hash table implementation. Choosing appropriate key types also plays a role in efficiency. Using immutable key types, such as strings or numbers, ensures consistency and avoids potential performance issues. Mutable keys can lead to unexpected behavior and decreased performance. A key consideration when designing applications that use dictionaries is to ensure that the keys chosen for the dictionaries are well-suited for the hash function used by Julia. Careful attention to this aspect can significantly impact performance.
Beyond basic usage, Julia provides advanced features for dictionary manipulation. Functions like `keys`, `values`, and `pairs` allow you to efficiently access the keys, values, or key-value pairs of a dictionary. These functions are highly optimized for speed and allow for convenient iteration over the dictionary's contents. Julia’s built-in `haskey` function allows efficient checking for the existence of a key without the risk of throwing an error. This is crucial for robust error handling in applications that use dictionaries extensively. Additionally, comprehensions provide a concise syntax for creating dictionaries from existing data structures. This feature enhances code readability and can improve efficiency when creating dictionaries from arrays or other data sources. The flexibility to define custom key and value types allows integrating dictionaries seamlessly into complex data structures. This flexibility makes Julia’s dictionaries suitable for diverse data structures and application designs.
The efficiency of dictionaries extends beyond basic operations to include more complex scenarios. Dictionaries can be nested, forming tree-like structures to represent hierarchical data. Julia’s support for custom types enables creating dictionaries where both keys and values can be complex data structures. This is important in applications requiring efficient storage and retrieval of large, nested datasets. Such applications can benefit significantly from the structure offered by dictionaries, allowing for efficient navigation and querying of intricate data. For example, representing hierarchical organizational structures or complex document databases.
Sets: Efficient Membership Testing
Sets in Julia offer a powerful way to manage collections of unique elements. Sets provide efficient membership testing, making them ideal for scenarios where determining whether an element exists within a collection is crucial. A set is created using the syntax `mySet = Set(["a", "b", "c"])`. Membership testing is performed using the `in` operator: `("a" in mySet)` returns `true`. Sets automatically handle duplicate elements, ensuring only unique values are stored. Case Study 1: A network application using sets can efficiently track unique IP addresses without worrying about duplicates. Case Study 2: A spell checker can use sets to store valid words, allowing rapid verification of word existence.
Julia’s implementation of sets leverages hash tables, providing average-case O(1) time complexity for membership testing, insertion, and deletion. This efficiency is particularly valuable for large datasets. While the worst-case scenario is O(n) due to potential hash collisions, the probability of this occurring is minimized with Julia’s optimized hash table implementation and the choice of hash functions. The use of sets significantly enhances the efficiency of membership checking compared to using arrays or lists, where linear searches are required. The elimination of redundant elements also leads to more efficient memory management.
Sets in Julia offer a wide array of operations beyond basic membership testing. Set union, intersection, difference, and symmetric difference operations are readily available, allowing for efficient manipulation and analysis of sets. These operations are highly optimized, providing performance benefits compared to manual implementations. Julia's set operations work seamlessly with other data structures. For instance, set operations can be readily combined with arrays to filter data efficiently, or they can be used with dictionaries to perform complex analyses. This flexibility expands the applicability of sets to diverse data analysis tasks.
Beyond fundamental set operations, Julia’s sets support a rich set of functionalities, including efficient iteration, conversion to other data structures like arrays, and integration with other Julia packages for advanced data analysis. These functionalities further enhance the practicality of using sets for diverse applications. The ability to create sets of custom types enhances the flexibility of this data structure. Combining Julia’s type system with sets allows the efficient management of sets of objects, such as structs or custom classes. This is crucial for modeling complex data structures and applications where the elements of the set are not limited to basic data types like numbers or strings.
Tuples: Immutable Collections
Tuples in Julia are immutable collections of elements. Unlike arrays, tuples cannot be modified after creation. This immutability provides several advantages, such as thread safety and increased code predictability. A tuple is created using parentheses: `myTuple = (1, 2, 3)`. Access to elements is similar to arrays: `myTuple[2]` returns 2. However, attempting to modify a tuple results in an error. Case Study 1: In concurrent programming, tuples are ideal for representing data that should not be changed by multiple threads simultaneously, enhancing program stability and preventing data races. Case Study 2: In a function that receives multiple parameters, using a tuple can ensure that the parameters remain unchanged within the function’s scope.
The immutability of tuples leads to several performance benefits. Because tuples cannot be modified, the Julia compiler can perform various optimizations, leading to faster execution and more efficient memory management. The compiler can make assumptions about the data within a tuple, streamlining computations and reducing overhead. The compiler can also effectively leverage this immutability for efficient caching, leading to faster repeated accesses. This predictability allows the just-in-time compiler to generate more efficient machine code, resulting in improved overall performance. This contrasts with mutable data structures, where the compiler needs to handle dynamic modifications, potentially increasing the complexity of code optimization.
Beyond basic operations, Julia’s tuples support advanced features that leverage their immutability. Tuple unpacking allows for assigning the elements of a tuple directly to multiple variables, improving code readability and conciseness. Tuples can be used as keys in dictionaries, particularly useful when representing complex keys that should remain unchanged. This is not possible with mutable data structures such as arrays. Tuples can be nested, creating complex hierarchical structures that are both immutable and highly structured, enhancing data integrity and improving predictability.
The immutability of tuples extends beyond basic functionality. Their usage in function arguments ensures parameter preservation throughout the function's execution, making them especially useful when dealing with large data sets. The predictable nature of tuples allows for efficient memory allocation and deallocation, minimizing runtime overhead. They are also useful in representing configurations or settings that should remain constant throughout the program's execution. This is beneficial in applications requiring high stability and reduced risk of unintentional data modification, such as critical system components or embedded systems.
Conclusion
Julia's data structures are meticulously designed for performance and versatility. Arrays provide efficient handling of numerical data, dictionaries offer fast key-based access, sets ensure uniqueness, and tuples enforce immutability. Mastering these structures is crucial for writing efficient and maintainable Julia code. Understanding their strengths and limitations, along with best practices for usage, will enable developers to harness Julia’s power for tackling computationally intensive tasks and building robust, high-performance applications. Choosing the right data structure depends critically on the specific application and the nature of the data being handled. Careful consideration of the characteristics of the data and the type of operations to be performed is essential in order to optimize the performance and efficiency of the program. The combination of optimized data structures and a high-performance language leads to substantial improvements in computational efficiency and overall application performance. The ability of Julia's compiler to effectively utilize the immutability and other characteristics of these data structures ensures that programs written using these data structures are faster and more efficient.