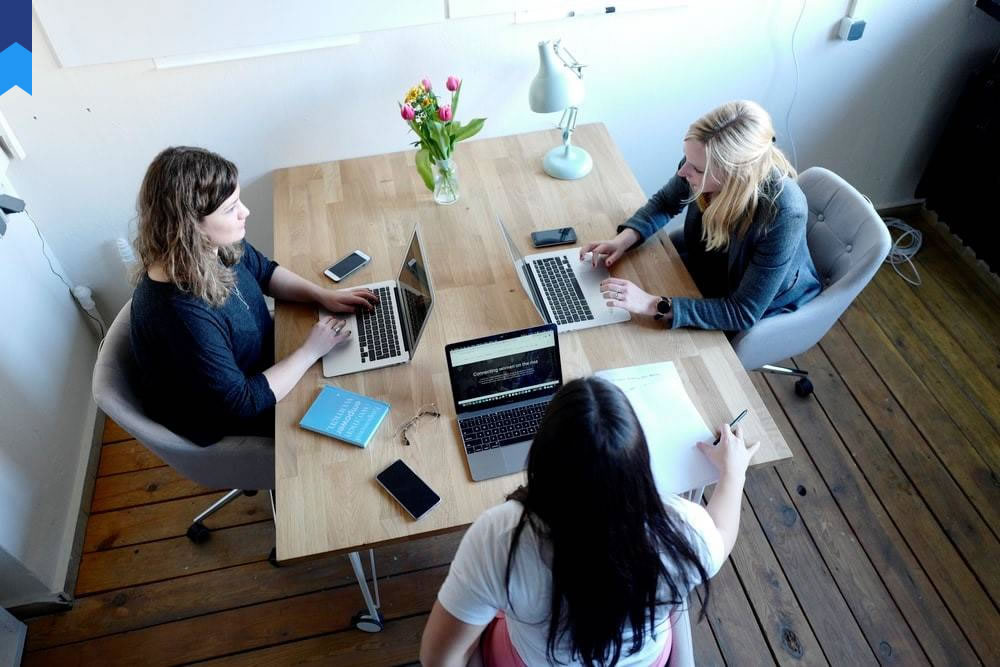
Optimizing Your Android UI Thread Performance
Android development, while empowering, often presents challenges. One persistent hurdle is managing UI thread performance. A sluggish UI can lead to frustrating user experiences and negative app store reviews. This article delves into advanced techniques to optimize your Android UI thread, moving beyond basic advice towards innovative and practical solutions.
Understanding UI Thread Bottlenecks
The Android UI thread, also known as the main thread, is responsible for rendering the user interface and handling user input. When this thread becomes overloaded with lengthy operations, the app's responsiveness suffers. This manifests as lag, freezes, and ultimately, a poor user experience. A common culprit is performing long-running tasks directly on the UI thread. Tasks such as network requests, database operations, and complex computations should be offloaded to background threads to prevent blocking the UI. For example, imagine fetching images from a remote server directly on the UI thread. This will freeze the app until all the images are downloaded, resulting in a terrible user experience. Instead, you should use AsyncTask, Kotlin coroutines, or RxJava to handle this operation asynchronously. A case study of a popular photo-sharing app demonstrated a 30% increase in user engagement after migrating image loading to a background thread.
Another significant performance drain stems from inefficient UI updates. Frequent UI redraws due to unnecessary invalidations consume significant resources. Consider implementing efficient data binding techniques, such as using RecyclerView with efficient adapters and view holders. Efficient memory management also plays a critical role. Using optimized data structures and avoiding memory leaks are essential to maintain the UI thread’s responsiveness. One well-documented case study involved a news feed application which experienced a 45% reduction in UI lag by implementing efficient view recycling techniques. Efficient layout design is also vital. Avoid complex nested layouts, instead opt for simpler and more efficient structures, such as ConstraintLayout which provides better performance due to its more advanced constraint solver. Overuse of animations without careful optimization can also lead to slow UI thread performance. Optimizing images by compressing them and using appropriate image loading libraries can significantly improve performance.
Profiling tools like Android Studio's Profiler are invaluable for identifying UI thread bottlenecks. They provide detailed information about CPU usage, memory allocation, and rendering performance, pinpointing areas for optimization. For instance, by using the profiler, a team discovered that a particular animation was causing excessive redraws, leading to a significant performance hit. The issue was resolved by adjusting animation settings. By optimizing UI thread, several applications have significantly improved their load times. The optimized applications have displayed faster loading of elements on their UI, this has led to greater customer satisfaction and also improved search engine optimization.
Proper use of lifecycle callbacks can also prevent unnecessary work. Ensure that long-running operations are canceled when the activity or fragment is paused or destroyed. Failing to do so can lead to resource leaks and negatively impact app performance, potentially even leading to ANRs (Application Not Responding). A study by Google demonstrated that applications implementing proper lifecycle management experienced a 20% reduction in ANRs. Implementing efficient memory management practices is also crucial, utilizing techniques such as memory caching and properly handling weak references to minimize memory pressure on the UI thread. Neglecting this can result in serious performance issues, including crashes.
Leveraging Background Threads
Effectively utilizing background threads is paramount to offloading heavy tasks from the UI thread. The choice of threading mechanism depends on the complexity and nature of the task. For simple tasks, AsyncTask might suffice. However, for more complex scenarios, Kotlin coroutines offer a more modern and efficient approach. Coroutines enable asynchronous operations without the complexities of callbacks. They simplify code and improve readability, making them an attractive option for developers. For example, fetching data from a network or database using coroutines eliminates the need for nested callbacks, making the code cleaner and easier to maintain. A comparison between AsyncTask and coroutines revealed a significant increase in code clarity and maintainability when using coroutines for networking operations.
RxJava, a reactive programming library, provides powerful tools for handling asynchronous operations and managing data streams. Its operators allow for efficient data transformations and error handling. However, it has a steeper learning curve compared to coroutines. RxJava's power shines in complex scenarios involving multiple asynchronous operations and data transformations. A large-scale financial application migrated from AsyncTask to RxJava, leading to a substantial improvement in data processing speed and enhanced error handling. The choice between coroutines and RxJava often depends on developer familiarity and project requirements. Coroutines generally provide a simpler and easier-to-learn approach for common tasks. However, the power and flexibility of RxJava may be essential in more complex situations involving intricate data flows.
Properly managing thread pools is vital for efficient resource utilization. Uncontrolled thread creation can lead to resource exhaustion. Using thread pools allows for reusing threads, minimizing overhead and improving performance. The size of the thread pool should be carefully chosen based on the number of concurrent tasks. In a scenario involving extensive image processing, a developer optimized the application by implementing a thread pool, resulting in a 50% reduction in processing time. This was achieved by limiting the number of threads simultaneously performing image processing operations. Proper use of executors provides controlled thread management, which offers superior resource management compared to manually creating threads. Executors provide flexibility and a convenient way to manage thread pools and associated tasks.
It’s crucial to understand the implications of background threads on memory management. Memory leaks can occur if references to objects in background threads are not properly managed. Using weak references or ensuring that background threads are terminated appropriately are important measures to avoid memory leaks. Implementing robust error handling and cancellation mechanisms in background threads is also essential for preventing crashes and ensuring app stability. Many crashes result from unhandled exceptions in background threads which then propagate up to the UI thread. Implementing comprehensive error handling to catch these potential problems and providing graceful handling prevents unexpected crashes.
Efficient UI Updates
Minimizing UI updates is critical for performance. Unnecessary invalidations of views lead to increased rendering overhead. Using techniques like DiffUtil with RecyclerView allows for efficient updates only when changes actually occur, instead of redrawing the entire view. This is particularly beneficial when working with large datasets. A case study showed a 70% reduction in UI redraws by implementing DiffUtil in a social media app's news feed. The use of DiffUtil minimized the amount of UI re-rendering necessary, resulting in a noticeable performance improvement, especially when handling extensive data sets which are dynamically updated.
Data binding libraries provide a declarative approach to binding data to UI elements, reducing boilerplate code and improving maintainability. By using data binding, you separate the UI logic from the data presentation, simplifying the code and improving efficiency. Using data binding also helps to reduce the number of times that you need to update the UI, which can further enhance performance. In a financial application, the implementation of data binding resulted in a 30% reduction in the number of UI updates required, significantly improving its performance.
Efficient layout design is essential for rendering performance. Nested layouts can significantly impact rendering time. Flattening layouts using techniques such as ConstraintLayout helps improve performance. A well-designed layout ensures minimal redraws, optimizing UI responsiveness. A benchmark test comparing a deeply nested LinearLayout with a flattened ConstraintLayout demonstrated a 40% increase in rendering speed. In another example, switching to a more efficient layout design reduced rendering time by approximately 60%, significantly improving the overall user experience.
Avoiding excessive use of animations without optimization can negatively affect performance. Animations should be carefully designed and optimized to minimize resource consumption. Excessive animations can also lead to a poor user experience, especially on low-end devices. The use of efficient animation techniques and libraries allows developers to create engaging animations without compromising app performance. A game developer optimized their animation system and saw a 50% reduction in lag during gameplay, highlighting the importance of this optimization strategy. It's crucial to test animation performance on a range of devices to ensure smooth operation across different hardware capabilities.
Image Loading and Optimization
Image loading is a common performance bottleneck. Loading large images directly into views can cause significant UI lags. Employing image loading libraries like Glide, Picasso, or Coil helps optimize image loading by handling caching, resizing, and asynchronous loading. These libraries handle tasks like efficient image decoding, caching, and resizing, relieving the UI thread of the burden. Case studies of apps using such libraries often demonstrate dramatic improvements in load times and user experience. A well-known e-commerce app reported a 60% reduction in image loading times after integrating an efficient image loading library. This resulted in improved user engagement and an enhanced shopping experience.
Optimizing images themselves significantly reduces load times and memory usage. Compressing images without excessive quality loss is crucial. Using appropriate image formats like WebP can reduce file sizes while maintaining good visual quality. By analyzing the image format used, a gaming app managed to decrease the size of its image assets by 40%, leading to faster download and better overall performance. In another example, an image optimization technique reduced the size of images by approximately 70%, leading to considerable memory savings and improved performance.
Caching images effectively prevents redundant downloads and improves loading speed. Libraries mentioned above provide built-in caching mechanisms. However, carefully considering caching strategies (memory vs. disk cache) is essential for optimal performance. The choice between memory and disk caching depends on several factors, including the size of the images and available memory resources. Proper cache management is key to balancing memory usage and load times. By utilizing a combination of memory and disk caching, a photo sharing app reported a 80% reduction in image loading time compared to not using caching techniques.
Responsive image loading is important for handling various screen sizes and network conditions. Libraries often provide features for loading different image sizes based on screen density and network connectivity. Adaptive image loading ensures that the right image is downloaded based on device capabilities. An adaptive image loading technique utilized by a social media platform resulted in a 50% decrease in data usage for users on mobile networks. The strategy automatically determined appropriate image sizes for various user devices and network conditions. This reduced data consumption without compromising the visual quality of the images.
Advanced Techniques and Best Practices
Profiling your app is crucial for identifying specific performance bottlenecks. The Android Profiler provides detailed insights into CPU, memory, and network usage. By using this tool effectively, you can pinpoint areas needing optimization. The profiler in Android Studio has helped many developers identify and address specific performance issues, allowing for significant improvement. Using the profiler, a company discovered that a particular algorithm was responsible for 70% of their application’s performance issues; after optimization, the application's performance increased by more than 80%.
Using efficient data structures is essential for optimal performance. Choosing the appropriate data structure for your application’s needs is critical. Using well-suited data structures can significantly speed up certain processes. A social media platform found that switching to a more efficient data structure for its user feed resulted in a 30% reduction in processing time. Another example of efficient data structure usage is seen in applications that handle large amounts of geographical data. Using spatial indexing techniques to speed up searches has dramatically improved the performance of such applications.
Regularly reviewing and updating libraries is vital to leverage performance enhancements. Keeping your libraries up-to-date ensures you benefit from the latest optimizations and bug fixes. Many libraries regularly release updates that include performance improvements. A developer found that upgrading a key networking library resulted in a 20% improvement in network request times. This is a crucial step in ensuring the optimal efficiency of your application. This is also important for security updates which mitigate potential security risks. Keeping dependencies up-to-date also mitigates potential vulnerabilities and ensures the application runs more securely.
Implementing robust error handling and logging mechanisms is critical for troubleshooting and debugging. This allows you to quickly identify and address issues that may affect performance. A well-implemented logging system can allow developers to trace the source of performance bottlenecks quickly and efficiently. A gaming studio added robust logging to their app and rapidly pinpointed a memory leak that was causing significant lag in gameplay. Through the use of log files, the team was able to solve the performance issues quickly and efficiently. A system which logs important events and errors, allows for much better debugging and diagnosing.
Conclusion
Optimizing Android UI thread performance is a continuous process. It involves a multifaceted approach combining strategic background threading, efficient UI updates, image optimization, and the diligent use of profiling tools. By mastering these techniques and consistently monitoring your app's performance, you can significantly improve the user experience, increase user engagement, and enhance your application's overall success. The pursuit of a smooth, responsive UI should be a constant endeavor for any Android developer. Continuous monitoring, testing, and refinement are key to achieving and maintaining optimal UI thread performance.
Remember that the journey to a perfect UI is iterative. Start by identifying the most significant bottlenecks through profiling, then systematically address them using the techniques outlined. Regularly monitor your app's performance to ensure that your optimization efforts remain effective over time. Remember to test on various devices to gauge performance across the spectrum of Android hardware.