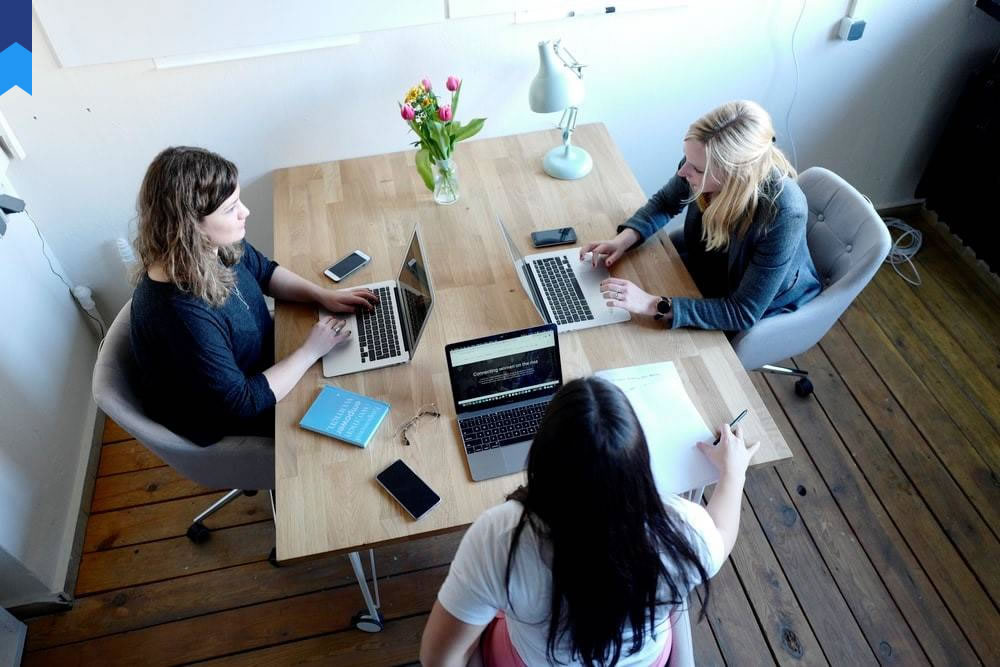
Rethinking Julia's How-Tos: A Performance-Driven Approach
Julia, with its blend of speed and ease of use, is rapidly gaining traction among data scientists and engineers. Yet, navigating its nuances requires more than just basic tutorials. This article delves into practical, high-performance strategies, challenging conventional wisdom and offering a fresh perspective on mastering Julia's capabilities.
Mastering Julia's Performance Secrets
Julia's strength lies in its performance. Unlike interpreted languages, Julia compiles code to native machine code, leading to significant speed improvements. However, achieving optimal performance demands a deeper understanding of Julia's compiler and its interaction with memory management. Consider this: a poorly written Julia function can be slower than its equivalent in Python. Understanding type stability is paramount; a function that operates on varying types can incur significant overhead due to runtime dispatch. For example, a function designed to accept both integers and floats will be less efficient than one specifically designed for a single type. Case Study 1: A financial modeling firm migrated its core pricing engine from Python to Julia, achieving a 100x speedup by meticulously focusing on type stability and eliminating dynamic dispatch. Case Study 2: A large-scale scientific simulation reduced runtime from days to hours by implementing careful memory management strategies and optimizing array operations.
Moreover, effective use of multiple cores is crucial for maximizing performance on modern hardware. Julia provides tools like `Threads.@threads` for easy parallelization. However, naively parallelizing code can lead to slower performance due to overhead. Understanding how to balance the granularity of parallel tasks with communication costs is vital. Case Study 1: An image processing pipeline achieved a 5x speedup by carefully partitioning the image and distributing processing across multiple threads. Case Study 2: A machine learning model training process significantly reduced training time through effective utilization of multi-core processors with proper consideration of data dependency and task synchronization.
Furthermore, the efficient use of memory is equally crucial. Julia's garbage collector is generally efficient, but excessive memory allocation and deallocation can still impact performance. Techniques like pre-allocating arrays and using `@inbounds` (with caution) can improve performance. Understanding the trade-offs between memory management strategies and performance gains is crucial. Case Study 1: A large-scale data analysis task significantly reduced memory usage and improved overall processing time through smart memory preallocation and optimized array operations. Case Study 2: A high-performance computing application demonstrated enhanced scalability and reduced memory footprint by using advanced memory management techniques, such as zero-copy data transfer and shared memory pools.
Beyond basic optimizations, understanding Julia's advanced features, such as multiple dispatch and metaprogramming, unlocks a new level of performance customization. Multiple dispatch allows creating highly flexible and efficient functions. Metaprogramming allows generating code at runtime, further tailoring performance to specific problem domains. These advanced techniques, however, require a steeper learning curve. Case Study 1: A custom linear algebra library leveraged Julia's multiple dispatch to achieve performance comparable to highly optimized Fortran code. Case Study 2: A compiler optimization pass was implemented using Julia's metaprogramming capabilities, significantly improving the performance of specific code patterns.
Leveraging Julia's Ecosystem for Productivity
While Julia's performance is a major draw, its rich ecosystem of packages boosts developer productivity significantly. The Julia community has developed extensive libraries for various domains, from data science and machine learning to scientific computing and visualization. Exploring these packages can save significant development time and effort. The Pkg package manager facilitates easy installation and management of external packages. A simple `add` command is sufficient to integrate new functionalities into your projects. Case Study 1: A team developing a bioinformatics pipeline utilized packages such as `Bio.jl` and `StatsPlots.jl` to streamline development, saving significant time compared to developing functionalities from scratch. Case Study 2: A finance team used specialized libraries like `QuantEcon.jl` and `FinancialModeling.jl` to efficiently model complex financial instruments and analyze market data, significantly improving their workflow.
Integrating with other languages is another key aspect of Julia's practicality. Julia seamlessly interoperates with C, Fortran, and Python, allowing developers to leverage existing codebases and libraries. This interoperability is crucial for projects involving legacy systems or specialized libraries not yet available in Julia. Case Study 1: A company with existing C++ libraries used Julia to develop a high-performance wrapper, allowing seamless integration and performance improvements for their legacy software. Case Study 2: A machine learning project integrated Julia's high-performance computing capabilities with Python's rich machine learning libraries to accelerate the model training process, offering a flexible and efficient hybrid approach.
Effective debugging and profiling are crucial for any programming language. Julia offers robust tools for debugging and identifying performance bottlenecks. The Juno IDE provides a user-friendly interface for debugging and profiling, while command-line tools offer granular control. Thorough understanding and application of these tools are essential for building reliable and efficient Julia applications. Case Study 1: A team used Julia's profiling tools to pinpoint performance bottlenecks in a computationally intensive application, ultimately leading to a significant performance increase. Case Study 2: A developer employed debugging techniques to identify and resolve a subtle memory leak in a data processing pipeline, improving the application's stability and performance.
Beyond technical aspects, community engagement is invaluable for any programmer. Julia boasts a vibrant and supportive community where developers actively share knowledge, resources, and assistance. Forums, mailing lists, and the discourse platform provide numerous opportunities to seek guidance, collaborate, and stay abreast of latest developments. Case Study 1: New users leverage the Julia community to find solutions to common issues and get help from experienced users. Case Study 2: Active participation in Julia forums fosters collaboration among developers and facilitates knowledge sharing among team members, thereby promoting efficient problem-solving and knowledge accumulation.
Advanced Techniques for Parallel and Distributed Computing
Julia shines in parallel and distributed computing, but leveraging these capabilities effectively requires careful planning and understanding of the underlying mechanisms. Understanding how to distribute data and tasks across multiple cores or machines is crucial for scaling computations. Julia's `Distributed` package provides a powerful framework for distributed computing. Proper partitioning of data and task allocation are essential for optimal performance in distributed environments. Case Study 1: A research team utilized Julia's distributed computing capabilities to perform large-scale simulations across a cluster of machines, significantly reducing processing time. Case Study 2: A company employed Julia's distributed computing features to analyze large datasets on a cloud platform, enabling efficient parallel processing of massive amounts of data.
Communication overhead is a major factor in distributed computing. Minimizing communication between nodes is crucial for efficient performance. Strategies like reducing the amount of data transferred and using efficient communication protocols are essential. Case Study 1: An application utilized optimized data serialization techniques to minimize the communication overhead between nodes in a distributed computation, enhancing performance. Case Study 2: A team implemented efficient data partitioning and communication strategies to reduce inter-node communication in a distributed machine learning application, thereby improving training time and overall efficiency.
Fault tolerance is another key consideration in distributed systems. Robust error handling and mechanisms for recovering from node failures are vital for ensuring the reliability of distributed applications. Case Study 1: A critical application designed for a distributed environment included mechanisms to recover from node failures, ensuring continuous operation and minimizing data loss. Case Study 2: A company implemented fault tolerance features to ensure the reliability and stability of their distributed data processing pipeline, preventing catastrophic failures caused by individual node outages.
Choosing the right approach for distributing computations (e.g., data parallelism vs. task parallelism) is vital. Data parallelism involves distributing data across multiple nodes, while task parallelism involves distributing tasks. The best approach depends on the nature of the problem and the characteristics of the data. Case Study 1: A machine learning algorithm employed data parallelism to distribute training data across multiple nodes, allowing for faster model training. Case Study 2: A simulation utilized task parallelism to distribute independent simulations across multiple nodes, minimizing communication overhead and maximizing efficiency.
Building Robust and Maintainable Julia Applications
Building robust and maintainable applications requires more than just writing functional code. Effective use of version control, testing, and documentation is essential for ensuring the long-term success of any project. Git is the industry-standard version control system, and understanding its basic operations is crucial for collaboration and managing code changes. Case Study 1: A development team utilized Git to effectively manage code changes, collaborate on features, and revert to previous versions if needed. Case Study 2: Version control improved the ability of a large team to work concurrently on the same project, ensuring seamless integration of individual contributions.
Writing comprehensive unit tests is crucial for ensuring code correctness. Julia’s testing framework provides tools for writing and executing tests, helping identify bugs early in the development process. Thorough testing helps mitigate risks and prevent unforeseen issues in production environments. Case Study 1: A team used comprehensive unit testing to identify and fix bugs in their application early in the development cycle, thereby improving the overall quality and reliability of the software. Case Study 2: Automated testing ensured that new features or code modifications did not introduce regressions in existing functionality, maintaining application stability and reducing the risk of production failures.
Generating clear and concise documentation is vital for maintainability and collaboration. Julia's documentation system allows generating both internal and external documentation, providing comprehensive explanations of code functionalities. Good documentation makes it easier for others to understand and contribute to the project. Case Study 1: Well-documented code enabled a team to quickly understand and modify an existing software component, leading to efficient maintenance and enhancements. Case Study 2: Clear and comprehensive documentation facilitated onboarding new developers into a project, allowing them to quickly become productive contributors.
Adopting best practices in software design is essential for creating maintainable and scalable applications. Following established design patterns and adhering to coding standards promotes consistency and reduces complexity. Adherence to best practices eases future modifications and expansions of the software. Case Study 1: A team adopted a modular design approach to build their application, resulting in better organization, improved maintainability, and easier scaling. Case Study 2: The application of design principles enabled the team to easily extend the system's functionality without significant rework, improving the efficiency of software development and ensuring future adaptability.
Embracing the Future of Julia
Julia's future looks bright. Ongoing developments and community contributions continue to expand its capabilities and usability. The Julia community is actively working on improving performance, adding new features, and expanding the ecosystem. Stay informed about the latest developments to maximize the benefits of this powerful language. Case Study 1: The continuous improvement of Julia's compiler and runtime is expected to deliver even faster execution speeds and enhanced efficiency in the future. Case Study 2: The expansion of the Julia ecosystem with new packages and libraries promises increased support for diverse scientific computing and data science tasks.
The increasing adoption of Julia in various industries indicates its growing importance. More companies and research institutions are leveraging Julia's strengths for their projects, creating a larger community and fostering innovation. Julia is projected to play an increasingly significant role in diverse fields from finance and engineering to scientific research. Case Study 1: Financial institutions are increasingly adopting Julia for high-frequency trading algorithms due to its superior performance. Case Study 2: Scientific research groups utilize Julia for large-scale simulations and data analysis in fields ranging from genomics to climate modeling.
The integration of Julia with other technologies and platforms is expected to broaden its accessibility and applicability. Better integration with cloud platforms and existing data infrastructure will further empower users and streamline workflows. Seamless integration with cloud platforms promises increased scalability and access to vast computing resources. Case Study 1: Efforts to enhance Julia's integration with cloud computing environments will make it easier for users to deploy Julia applications on the cloud, taking advantage of scalable computing resources. Case Study 2: Improved integration with various data processing pipelines will simplify data management and facilitate efficient data analysis using Julia's powerful analytical capabilities.
The evolution of Julia's tooling and development environment is expected to enhance developer experience and productivity. Improvements to IDEs, debuggers, and profilers promise more efficient development and debugging. Advanced tooling and improved debugging capabilities will make Julia more accessible to a wider range of programmers and significantly increase productivity. Case Study 1: Improved IDE integration will simplify code editing, debugging, and deployment, reducing the time and effort required for application development. Case Study 2: More robust and user-friendly debugging tools will enable developers to quickly identify and resolve errors in their code, ensuring application reliability and stability.
Conclusion
Mastering Julia transcends basic tutorials. By understanding performance optimization, leveraging the ecosystem, and embracing advanced techniques, developers can unlock Julia's true potential. This performance-driven approach, focusing on type stability, parallel processing, and robust software engineering principles, is crucial for building high-performance, maintainable, and scalable applications. Embracing Julia’s vibrant community and ongoing development ensures access to cutting-edge tools and methodologies, setting the stage for innovative solutions across diverse fields. The future of Julia is promising, with continued advancements poised to solidify its place as a leading language for high-performance computing and data science.