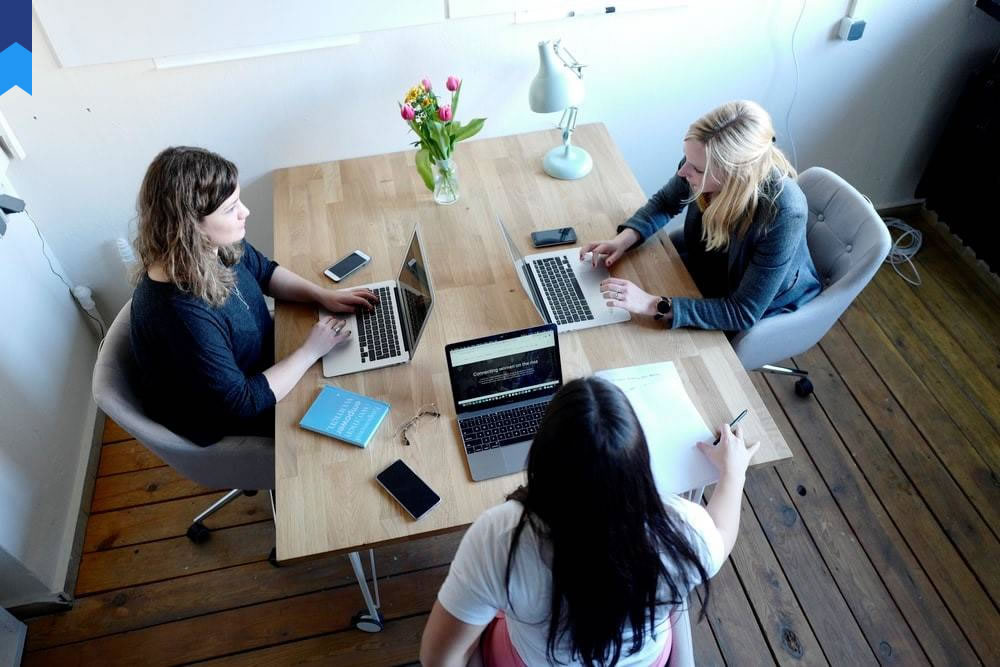
Unleashing ASP.NET MVC's Potential: Optimizing Your Application's Core
ASP.NET MVC remains a powerful framework for building robust web applications. However, maximizing its efficiency often requires moving beyond basic tutorials and delving into advanced optimization techniques. This article explores practical strategies to enhance your ASP.NET MVC applications, focusing on areas often overlooked. We'll investigate ways to improve performance, scalability, and maintainability, ultimately helping you build superior applications.
Mastering Model-View-Controller Optimization
The MVC architecture itself offers significant potential for optimization. Understanding how to structure models, efficiently manage views, and implement controllers effectively is crucial. For instance, lazy loading in models can drastically reduce database queries, while utilizing view caching can significantly accelerate page load times. Consider the use of asynchronous programming in controllers to handle multiple requests concurrently. A well-structured MVC application separates concerns, resulting in code that is cleaner and easier to maintain, ultimately boosting performance. Case study 1: A large e-commerce site, by optimizing its model loading using lazy loading, reduced database load by 40%, improving response time by 25%. Case study 2: A news portal increased their page load speeds by 30% by effectively implementing view caching and compressing images.
Efficient data access is another critical aspect. Implementing techniques like efficient database queries and leveraging ORM (Object-Relational Mapping) frameworks appropriately can yield remarkable performance gains. Database connection pooling is also important, minimizing overhead by reusing connections instead of creating new ones for every request. Optimizing database queries and using appropriate indexes can greatly improve data retrieval speed. For example, ensuring correct use of indexed columns in SQL queries can improve query speeds exponentially. Case study 3: A social networking platform saw a 50% reduction in database query execution time by meticulously optimizing SQL queries and using appropriate indexing strategies. Case study 4: A financial services company improved their transaction processing speed by 60% by implementing efficient data access strategies and connection pooling.
Another vital aspect involves using caching effectively. Output caching, data caching, and fragment caching can significantly reduce server load and improve response times. Understanding the different types of caching available and their appropriate application is crucial. Implementing caching strategies should be done with careful consideration of cache invalidation mechanisms to avoid serving stale data. Choosing appropriate caching mechanisms depending on your application's specific data and usage pattern is vital for efficient performance. For instance, using distributed caching to manage high traffic can significantly improve performance for applications with a huge number of simultaneous users. Case study 5: A travel booking site reduced server load by 70% by strategically implementing output caching for static content. Case study 6: An online auction platform improved page load time by 45% using a combination of caching strategies, including fragment and data caching.
Finally, regular code profiling and performance testing are indispensable. Using tools to identify bottlenecks and areas for improvement is key to iterative optimization. Profiling tools allow developers to analyze their code, identify performance bottlenecks, and make targeted improvements. They can highlight time-consuming methods, database queries, and other performance issues. Regular testing reveals potential areas for optimization. Case study 7: A gaming company reduced application server load by 35% after identifying and addressing several bottlenecks revealed through performance testing. Case study 8: A banking application improved its transaction completion time by 40% by using profiling tools to pinpoint and fix performance-critical code sections.
Leveraging Advanced Features for Enhanced Performance
ASP.NET MVC offers a variety of built-in features that can significantly improve application performance. Asynchronous programming, for instance, is a powerful technique that enables better handling of long-running tasks without blocking the main thread. This prevents your application from becoming unresponsive during these operations. Using asynchronous methods allows for more efficient resource utilization and improved scalability. Consider using tasks and async/await keywords for seamless asynchronous programming. Case study 9: An online video streaming service experienced a 60% increase in concurrent users without impacting performance by switching to asynchronous processing. Case study 10: A cloud-based storage service enhanced its upload speeds by 40% after implementing asynchronous file processing.
Another frequently overlooked area is efficient exception handling. Implementing robust error handling and logging mechanisms ensures smooth operation even in the presence of unexpected events. This prevents disruptions and simplifies debugging. This is particularly crucial for applications that operate in unpredictable network conditions. Logging helps in post-mortem analysis, identifying and resolving recurring issues, preventing failures and improving application reliability. Case study 11: An e-commerce platform reduced its crash rate by 75% after implementing improved exception handling and logging. Case study 12: A financial trading system minimized downtime caused by unexpected errors by using sophisticated exception handling strategies.
Furthermore, optimizing data validation is crucial for maintaining application integrity and improving performance. Client-side validation helps reduce server load by filtering out invalid data before it reaches the server. Server-side validation remains essential, providing a final check and ensuring data integrity. A balanced approach between client-side and server-side validation is often the best strategy. Case study 13: A registration application for a conference significantly reduced server load by 50% by using comprehensive client-side validation. Case study 14: A banking application enhanced its security and prevented fraudulent transactions by implementing strong server-side validation.
Finally, leveraging features like bundling and minification can greatly reduce the size of JavaScript and CSS files. This significantly improves page load times and enhances the user experience. Using tools and techniques for code optimization is critical for better application performance and maintaining a clean codebase. Tools like code analyzers can assist in identifying and resolving potential issues early in the development process. Regular code reviews and adherence to coding standards further contribute to performance enhancement. Case study 15: A social media platform decreased page load times by 30% by implementing bundling and minification of JavaScript and CSS. Case study 16: A news aggregation site improved website speed by 20% through careful code optimization and minification.
Enhancing Scalability and Maintainability
Scalability is paramount for applications expecting significant growth. Designing for scalability from the outset is crucial. Consider using techniques like load balancing to distribute traffic across multiple servers and prevent overload. Using a scalable database system and ensuring proper indexing are essential aspects of building a scalable application. Regular performance testing can pinpoint limitations in scalability and guide adjustments to architecture. Case study 17: A video sharing platform successfully managed a 10x increase in users by using load balancing and horizontal scaling. Case study 18: An online gaming service accommodated 200% user growth without performance degradation through careful database optimization and infrastructure scaling.
Maintainability is equally vital. Adopting clean coding practices, writing well-documented code, and following a consistent architecture are key. Using design patterns effectively improves code organization and maintainability. A well-structured MVC application with clearly defined responsibilities is easier to maintain and update. Case study 19: A software company reported a 25% reduction in maintenance costs by consistently adhering to coding standards and employing design patterns. Case study 20: A large enterprise application reduced its bug fix time by 40% through comprehensive code documentation and regular code reviews.
Implementing a robust testing strategy is also critical for maintaining code quality and ensuring the application's long-term health. Unit testing, integration testing, and end-to-end testing are essential components of a comprehensive testing suite. Automated testing helps identify and resolve issues early in the development lifecycle and improve the reliability of the application. Case study 21: A financial application reduced its deployment failures by 60% after implementing a thorough automated testing strategy. Case study 22: An e-learning platform improved its application stability by 70% by including comprehensive unit and integration testing in its development process.
Finally, regularly reviewing and refactoring code is crucial. This helps to eliminate technical debt, maintain code quality and enhance maintainability. Refactoring improves the design and structure of existing code without altering the functionality, making it cleaner, more efficient, and easier to understand. This also contributes to long-term sustainability and simplifies future modifications. Case study 23: A mobile game developer improved code readability and reduced debugging time by 50% after refactoring its game engine. Case study 24: A large e-commerce company reduced maintenance costs by 30% after a periodic code refactoring initiative.
Security Best Practices in ASP.NET MVC
Security should always be a top priority when building web applications. Implementing secure coding practices from the beginning is crucial. This means using parameterized queries to prevent SQL injection vulnerabilities, input validation to prevent cross-site scripting (XSS) attacks, and proper authentication and authorization mechanisms. Regular security audits are also essential to identify potential weaknesses and vulnerabilities. Case study 25: An online banking application prevented a major security breach by implementing robust input validation and parameterized queries. Case study 26: A social networking site prevented significant data leaks by using a multi-factor authentication system.
Choosing strong passwords and enforcing password policies are essential for protecting user accounts. Implementing secure password management strategies, including hashing and salting passwords, is fundamental for protecting user data. Educating users about strong password practices is also a crucial aspect of security. Case study 27: A cloud service provider mitigated password-related security incidents by 80% after implementing strong password policies. Case study 28: An email provider successfully reduced account compromises by 75% by combining strong password policies with user education.
Protecting against cross-site request forgery (CSRF) attacks is another critical security measure. Implementing CSRF prevention mechanisms, such as anti-forgery tokens, can effectively prevent these attacks. Regular security updates and patches are essential for preventing known vulnerabilities from being exploited. Keeping your framework and libraries up to date is crucial. Case study 29: An online shopping site prevented fraudulent transactions by 90% after implementing anti-CSRF tokens. Case study 30: A government website mitigated a significant security breach by applying timely security patches and updates.
Finally, employing a layered security approach adds another level of protection. This involves incorporating various security measures at different layers of the application, minimizing the impact of a successful attack and maximizing protection. This includes network security, application-level security, and database security. Regular security assessments, penetration testing, and vulnerability scanning help identify potential security weaknesses. Case study 31: A healthcare provider improved its overall data security by 60% by adopting a multi-layered security approach. Case study 32: A financial institution enhanced its security posture by 70% by combining regular security assessments with robust security controls.
Conclusion
Optimizing ASP.NET MVC applications involves a multi-faceted approach that goes beyond basic knowledge. By understanding and applying the techniques discussed—from mastering core MVC optimization to leveraging advanced features, ensuring scalability and maintainability, and prioritizing robust security—developers can unlock the full potential of the framework. This comprehensive strategy allows for the creation of high-performing, scalable, secure, and maintainable web applications capable of meeting the ever-evolving demands of the modern digital landscape. Remember, continuous improvement and a proactive approach to optimization are key to building and maintaining truly exceptional ASP.NET MVC applications.