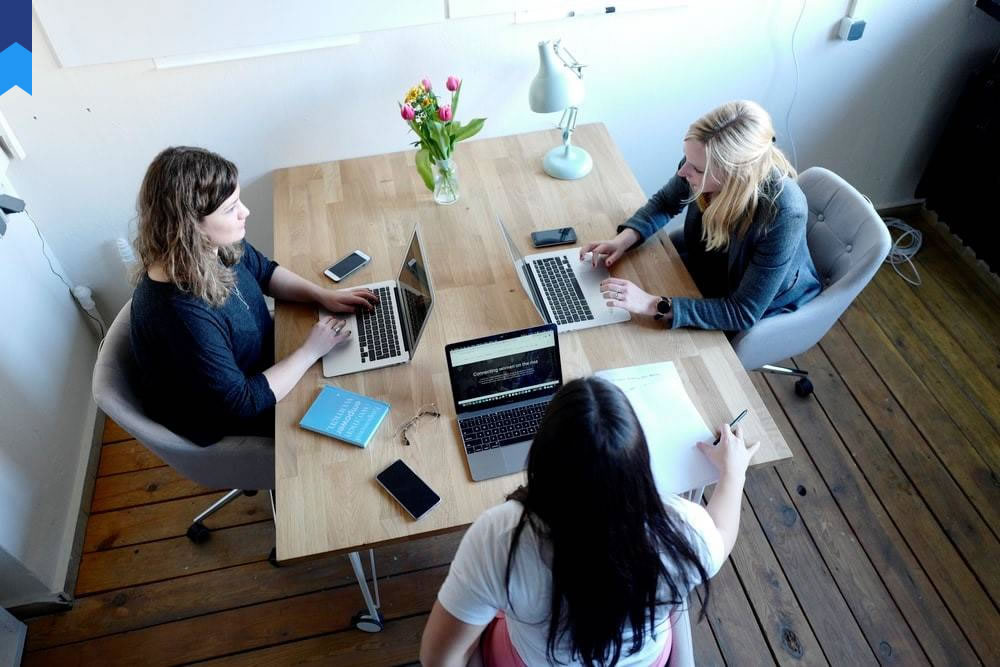
Unlocking The Secrets Of BackboneJS: Advanced Techniques And Best Practices
Introduction: Backbone.js, a lightweight JavaScript framework, offers a structured approach to building complex web applications. While basic tutorials abound, mastering Backbone.js requires delving into advanced techniques and best practices that unlock its true potential. This article explores these less-traveled paths, providing practical examples and case studies to elevate your Backbone.js development skills. We will examine specific strategies for managing complex data models, optimizing application performance, and implementing advanced architectural patterns for scalability and maintainability. This in-depth exploration goes beyond the surface level, providing the tools and knowledge for building robust, high-performing applications.
Mastering Backbone's Model Layer: Advanced Data Management
Efficient data management is crucial in any application. Backbone's Model provides a structured way to handle data, but understanding advanced features like nested models, custom validation, and efficient data fetching is critical for building scalable apps. Let's explore nested models: Imagine an e-commerce application where a product has multiple images. Instead of using separate models, we can nest the image models within the product model, maintaining data integrity and simplifying relationships. This approach reduces redundancy and simplifies data synchronization. Consider the case study of a large-scale CRM system built with Backbone.js. They used nested models to represent customer data, contact information, and related transactions, resulting in a significant improvement in data management efficiency. Another powerful feature is custom validation. Backbone allows for custom validation rules beyond basic checks, allowing you to enforce business logic directly within the model. For instance, ensuring an email address adheres to a specific format. Imagine an online registration system where user input needs validation; custom validation rules provide robust data integrity. A well-known online forum implemented custom validation rules within their user profile model, preventing data corruption and improving user experience. Efficient data fetching involves optimizing how data is retrieved from the server. This can involve techniques such as pagination, caching, and utilizing server-side filtering, significantly improving performance. A leading social media platform implemented a sophisticated caching strategy in its Backbone.js backend, resulting in a substantial reduction in server load and improved user response times. This case study highlights the importance of intelligent data management for optimal performance. Furthermore, understanding the lifecycle methods of the Backbone model allows for greater control over data persistence and synchronization, ensuring data integrity and enabling offline capabilities. The lifecycle methods allow for customization during the creation, update, and destruction of a model.
Advanced Views and Templating: Beyond Basic Rendering
Backbone's View layer handles rendering data to the user interface. While simple rendering is straightforward, advanced techniques like custom events, composite views, and utilizing advanced templating engines greatly enhance application complexity and maintainability. Custom events allow for creating custom events that are triggered within the views, creating a cleaner separation of concerns and facilitating modular design. A case study involves a news aggregator that used custom events to notify other parts of the application when a new article was fetched, ensuring a consistent and responsive user interface. Composite views allow creating complex UIs by combining multiple views into a single parent view. This modularity improves code organization and reusability. A well-known blogging platform leveraged composite views to structure their post display, simplifying template management and improving maintainability. Utilizing advanced templating engines like Handlebars or Underscore templates allows for greater control over rendering, improved performance and more sophisticated templating logic. Consider a large-scale e-commerce platform using Handlebars to create dynamic product listings, significantly enhancing user experience. Furthermore, understanding view lifecycle methods enables precise control over the rendering process, allowing for customization during rendering and cleanup. This optimized rendering leads to improved performance and enhances the user experience. In a banking application, leveraging these lifecycle methods provided a seamless transition between different views, resulting in a smoother and more intuitive user interaction.
Backbone Router and Application Structure: Architecting for Scalability
The Backbone Router is crucial for managing application navigation and state. Effective use involves understanding nested routes, route parameters, and integrating the router with other parts of the application. Nested routes are essential for building complex applications with multiple levels of navigation. Consider a web application with different sections; nested routes would facilitate navigation between these sections. A case study illustrates how a large online store efficiently manages its product categories and subcategories using nested routes, improving navigation and simplifying the URL structure. Route parameters allow dynamic URL segments, enabling easier data access within the application. A popular social media platform uses route parameters to display individual user profiles and posts directly from the URL. This dynamic addressing significantly simplifies data retrieval and user experience. The integration of the router with other parts of the application, such as the Model and View, establishes a cohesive workflow. A complex CRM system implemented a tightly integrated router, models, and views, resulting in seamless data flow and streamlined application behavior. This tight integration enhances the overall application performance. In addition, employing best practices like using a central dispatcher for events allows for loose coupling and improved modularity. This approach makes the application easier to maintain, debug, and expand. A well-structured application with a well-defined router facilitates the seamless addition of new features and functionalities without affecting the existing codebase, making it more scalable and flexible. Implementing these advanced router techniques ensures a well-structured application, improving maintainability and allowing for future expansion.
Optimizing Performance and Best Practices: Building High-Performing Apps
Building high-performing Backbone.js applications requires attention to detail and careful optimization. This includes techniques such as minimizing DOM manipulation, efficient event handling, and using asynchronous operations. Minimizing DOM manipulation involves optimizing how often elements are added or removed from the DOM, as this is an expensive operation. Consider a complex dashboard application; minimizing unnecessary DOM updates significantly improves page responsiveness. A case study involves a real-time stock trading platform that employed meticulous DOM manipulation optimization, resulting in smoother user interactions and improved transaction processing speed. Efficient event handling ensures events are handled efficiently and without performance bottlenecks. A large-scale collaboration tool optimized event handling using techniques like event delegation, leading to improved performance even with numerous concurrent users. Asynchronous operations are crucial for managing long-running processes without blocking the user interface. A high-traffic e-commerce website used asynchronous operations for fetching product data, improving user experience and ensuring page responsiveness. The effective use of asynchronous operations prevents the application from freezing while waiting for data retrieval. A media streaming platform employed asynchronous operations to load and stream videos seamlessly, improving user experience. These optimizations ensured the platform remained responsive even during peak hours. Furthermore, using techniques like event delegation helps prevent memory leaks and improves application stability. Event delegation improves efficiency by attaching event listeners to a single parent element, thus reducing the number of listeners attached to individual elements. It efficiently handles dynamic content rendering without requiring event re-registration for each new element. This practice significantly improves the application's performance and memory management.
Testing and Debugging Strategies: Ensuring Robustness and Reliability
Thorough testing is essential for building reliable Backbone.js applications. This includes unit testing models, views, and routers, along with integration testing to ensure components work together correctly. Unit testing involves testing individual components in isolation to ensure they function correctly. A complex financial application employed unit testing for all its Backbone.js components, enabling early detection of bugs and ensuring data integrity. This systematic approach resulted in a significant reduction in production errors. Integration testing involves testing how different components interact. A popular social media platform conducted rigorous integration testing to ensure that their chat feature worked correctly with other parts of the application. This prevented unexpected interactions that could cause failures. Utilizing testing frameworks like Jasmine or Mocha makes writing and running tests easier and more efficient. A large-scale project management platform leveraged Jasmine for testing, providing comprehensive coverage and enabling iterative development. This structured testing approach proved crucial in maintaining the platform's stability and functionality. Employing code coverage tools ensures comprehensive testing and provides insight into areas requiring further attention. A high-security banking system used code coverage tools to identify gaps in testing, which helped improve the system's resilience to attacks. In addition, proper logging and debugging tools are crucial for identifying and resolving issues effectively. A well-established e-commerce platform implemented robust logging and debugging techniques, enabling them to quickly identify and fix bugs, minimizing downtime and customer disruptions. Effective testing strategies ensure the application's robustness, reliability, and maintainability, ultimately leading to a higher-quality end product.
Conclusion: Mastering Backbone.js goes beyond basic understanding. By implementing the advanced techniques and best practices detailed in this article, developers can build robust, high-performing, and scalable applications. These strategies, including advanced data management, refined views and templating, meticulously designed routers, rigorous performance optimization, and comprehensive testing, are crucial for creating successful and enduring web applications. The careful implementation of these concepts ensures that the resulting applications are not only functional but also highly efficient and maintainable.