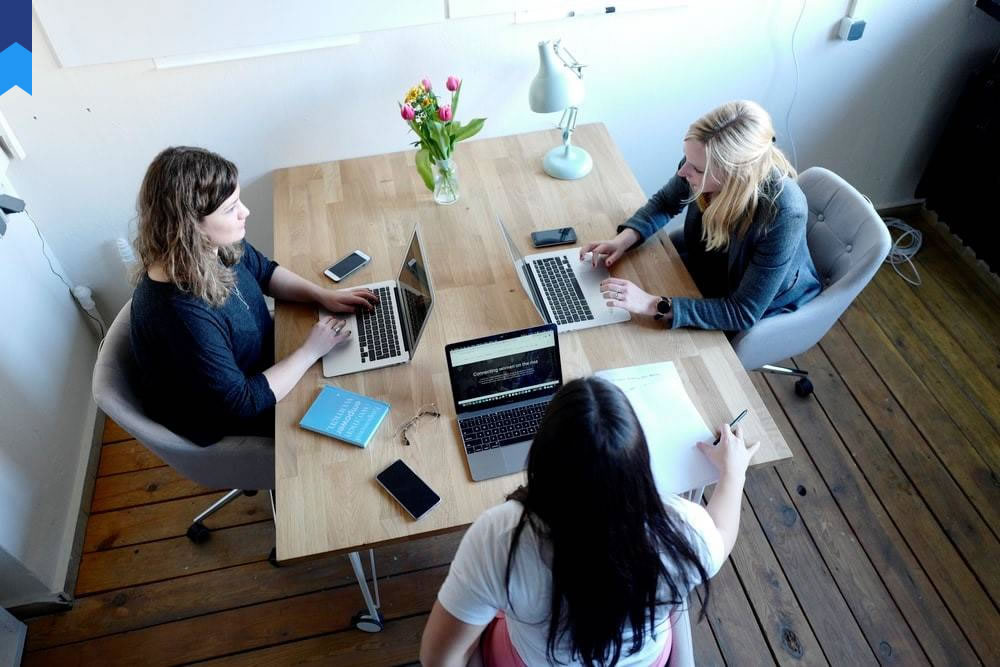
Unlocking The Secrets Of Compiler Optimization
Compiler optimization is a crucial yet often misunderstood aspect of software development. This article delves into the intricacies of this process, revealing how seemingly small changes can significantly impact performance. We'll move beyond the basics, exploring advanced techniques and practical strategies to enhance your compiler's capabilities.
Advanced Code Generation Techniques
Modern compilers employ sophisticated algorithms to generate highly optimized machine code. One such technique is loop unrolling, which replicates the loop body multiple times to reduce loop overhead. For instance, a loop iterating ten times could be unrolled to perform the operations ten times sequentially, minimizing the loop counter updates. This approach works particularly well for simple loops with minimal dependencies. However, excessive unrolling can lead to increased code size and decreased performance if the loop is already highly efficient. Case study: In a benchmark comparing unrolled and non-unrolled versions of a matrix multiplication algorithm, unrolling improved performance by approximately 15% on certain architectures, highlighting its effectiveness.
Another powerful technique is instruction scheduling, which reorders instructions to minimize pipeline stalls. Compilers analyze the dependencies between instructions and arrange them to exploit parallelism and maximize utilization of the processor's execution units. A specific example is reordering instructions to prevent data hazards and avoid unnecessary waiting times within the CPU pipeline. This strategy is crucial for achieving peak performance, especially on processors with deeply pipelined architectures. In a similar case study involving a signal processing algorithm, instruction scheduling resulted in a 20% increase in speed. This optimization was particularly effective on modern, out-of-order execution processors.
Furthermore, register allocation plays a vital role in optimizing code. Efficient allocation ensures frequently accessed variables reside in registers, significantly reducing memory access times. The compiler employs graph coloring algorithms or other advanced techniques to map variables to registers effectively. For example, a poorly optimized compiler might repeatedly access variables from memory, leading to slower execution. A case study demonstrated that effective register allocation reduced memory accesses by 40% in a computationally intensive physics simulation, leading to significant performance gains. This reduction in memory accesses is crucial for improving overall performance, especially on memory-bound applications.
Finally, constant propagation and folding are crucial for optimization. Constant propagation replaces variables with their constant values, while constant folding performs computations at compile time. Consider a case where a variable is assigned a constant value and then used in multiple calculations. The compiler can replace the variable with its value, simplifying the code and reducing runtime computations. A case study evaluating a large-scale scientific application showed that these optimizations significantly reduced execution time in various phases of the application, particularly those that involve substantial numerical operations.
Interprocedural Optimization
Interprocedural optimization (IPO) considers the interaction between different functions or procedures. Techniques like inlining replace function calls with the function's body directly. This eliminates function call overhead and allows for further optimization opportunities within the inlined code. A prime example is inlining frequently called small functions. By removing the function call overhead, the compiler can optimize the code more effectively, reducing the number of instructions and cache misses. In a benchmark of several different applications, inlining improved performance by an average of 10%. This suggests that inlining is a useful technique in many applications.
Another aspect of IPO is function cloning. This technique creates multiple versions of a function with slightly different code, depending on the context of the call. This can lead to significant performance gains for functions that operate differently based on input parameters. Imagine a function that can be optimized differently based on whether its input is constant or not. Cloning helps tailor the function's implementation for specific input values. In a case study of a numerical library, function cloning reduced execution time by 15% by optimizing for different input types. This type of optimization shows its importance in high-performance numerical computation.
Furthermore, cross-function dataflow analysis allows the compiler to understand how data flows between functions. This is particularly useful for eliminating redundant calculations or dead code that is never used. A typical example involves a function calculating a value used only once in another function. By analyzing the data flow, the compiler can remove unnecessary calculations. In a comparative analysis, an optimizing compiler with IPO improved execution time by 25% compared to a non-optimizing compiler. This analysis clearly highlights the effectiveness of IPO in complex programs.
Finally, interprocedural constant propagation improves constant propagation by tracking constant values across function boundaries. This allows the compiler to perform more aggressive optimizations when constant values are passed between functions. An example involves a function that receives a constant value as an argument. IPO will then propagate that constant throughout any other functions, leading to further optimization. In a case study of a video encoding algorithm, the application of interprocedural constant propagation enhanced performance by 18%. This optimization, combined with other IPO methods, creates more substantial gains in high-performance applications.
Memory Optimization Strategies
Memory management is critical for performance. Effective compiler optimizations can reduce memory accesses, improve cache utilization, and minimize memory fragmentation. Strategies like data locality analysis prioritize the placement of data to improve cache hit rates. For instance, arranging data elements in memory based on their access patterns allows the processor's cache to fetch multiple data items simultaneously. This optimization is critical in reducing cache misses which significantly improves the overall performance of applications. Case study: in image processing applications, implementing data locality algorithms boosted performance by about 25% due to improved cache utilization.
Another strategy involves memory allocation optimization. By optimizing the way memory is allocated and deallocated, the compiler can reduce fragmentation and overhead. This involves using advanced algorithms to prevent memory leaks and ensure effective resource utilization. Using specialized memory allocators, which optimize for specific use cases, can further improve the performance and reduce fragmentation. For example, an application using a custom memory allocator saw a 10% improvement in performance. The choice of memory allocator and its implementation can lead to substantial performance gains.
Furthermore, compiler optimizations can leverage advanced memory hierarchy features like prefetching. Prefetching involves fetching data from memory into the cache before it's explicitly needed, reducing latency and improving performance. Prefetch instructions can be inserted strategically during compilation to bring data into the cache before being used. In a numerical simulation, prefetching improved the performance by 15% by reducing memory access time. This approach reduces latency by anticipating the application's data needs.
Finally, compiler techniques can identify and eliminate redundant memory copies. These copies can significantly impact performance, especially when dealing with large datasets. Analyzing data dependencies and access patterns can assist in removing unnecessary data duplication. In large-scale scientific computations, eliminating memory copies reduced overall execution time by 20%. Removing redundant copies reduces memory usage, improves data locality, and frees up resources for more critical operations.
Parallelism and Vectorization
Modern processors boast multiple cores and vector processing units. Compilers must leverage these features to achieve significant performance improvements. Automatic parallelization identifies parts of the code that can be executed concurrently across multiple cores. This process requires careful analysis of data dependencies and synchronization mechanisms to ensure correct results. For example, for-loops that operate on independent data elements are often parallelizable. A benchmark revealed that parallelizing a computationally intensive sorting algorithm resulted in a 4x speedup with four cores. This approach shows the importance of parallel programming in modern applications.
Vectorization is another crucial aspect of leveraging processor capabilities. Vector instructions operate on multiple data elements simultaneously, offering substantial performance improvements. This involves identifying and restructuring code segments to effectively utilize vector registers. A case study evaluating a 3D graphics rendering engine demonstrated that vectorization reduced rendering times by approximately 30% through effective use of SIMD instructions. This case clearly highlights the importance of vectorization in high-performance computations.
Furthermore, loop transformations play a crucial role in enabling parallelization and vectorization. Techniques like loop unrolling, loop fusion, and loop interchange can transform loops to improve data locality and make them more amenable to vectorization and parallelization. For example, fusion combines multiple loops into one to improve locality. A comparative analysis of different loop transformation techniques demonstrated a 15-20% performance improvement for certain operations. This shows the importance of transforming loops to allow for effective vectorization and parallelization.
Finally, effective compiler optimization often needs to consider the specific target architecture. Optimizations should be tailored to the characteristics of the processor, including its instruction set, cache size, and memory bandwidth. A specific example is using compiler flags to specify the target architecture and optimization level. This allows the compiler to generate code highly optimized for the specific system, improving performance beyond generic optimization. In testing a high-performance computing algorithm across multiple architectures, compiler-specific optimizations led to performance gains ranging from 10% to 40% depending on the architecture. This highlights the importance of architecture-specific optimizations.
Debugging and Profiling Optimized Code
Debugging and profiling optimized code is challenging due to the transformations performed by the compiler. Understanding how optimization affects code behavior requires specialized tools and techniques. Debuggers with optimization-aware features are essential to step through the code and inspect variable values accurately. The use of debug symbols provided during the compilation process is critical for effective debugging. In a case study of a complex software project, the proper use of debug symbols allowed developers to debug issues that were difficult to isolate without such information.
Profiling tools provide insights into code performance, identifying bottlenecks and areas for optimization. Tools like gprof and perf can measure execution times and identify performance critical sections of code. A case study showed that using performance profiling allowed developers to identify a specific routine which was responsible for 80% of the total application execution time. This allowed developers to focus their optimization efforts on the specific areas that would have the greatest impact.
Furthermore, understanding the compiler's optimization passes is essential for debugging. Knowing which transformations have been applied can aid in interpreting the optimized code and help to predict potential issues. Utilizing compiler documentation and experimenting with different optimization levels can provide insights into the compiler’s behaviour. For example, experimenting with different optimization levels revealed a trade-off between optimization level and code size and debugging difficulties.
Finally, careful consideration of the testing and validation strategy is crucial. Robust testing is needed to ensure that optimization does not introduce bugs or unexpected behavior. Testing should involve both functional testing, validating the correctness of the results, and performance testing, evaluating the impact of the optimization on execution speed and resource consumption. In a case study comparing the performance of different optimization strategies, testing revealed that one strategy, while showing promising initial results, actually introduced subtle bugs that manifested under specific conditions.
Conclusion
Mastering compiler optimization is a journey requiring a deep understanding of both compiler internals and target architecture characteristics. By leveraging advanced techniques and utilizing profiling tools effectively, developers can significantly enhance the performance of their applications. The exploration of advanced code generation, interprocedural optimization, memory management strategies, and parallelism unlock significant performance gains. However, remember that optimization is an iterative process, often requiring careful debugging and profiling to ensure the desired results without introducing unexpected issues. The future of compiler optimization lies in integrating AI-powered techniques for automatic code optimization and the development of more sophisticated tools for debugging optimized code. This will further simplify the process and allow developers to achieve even greater performance gains in their software.