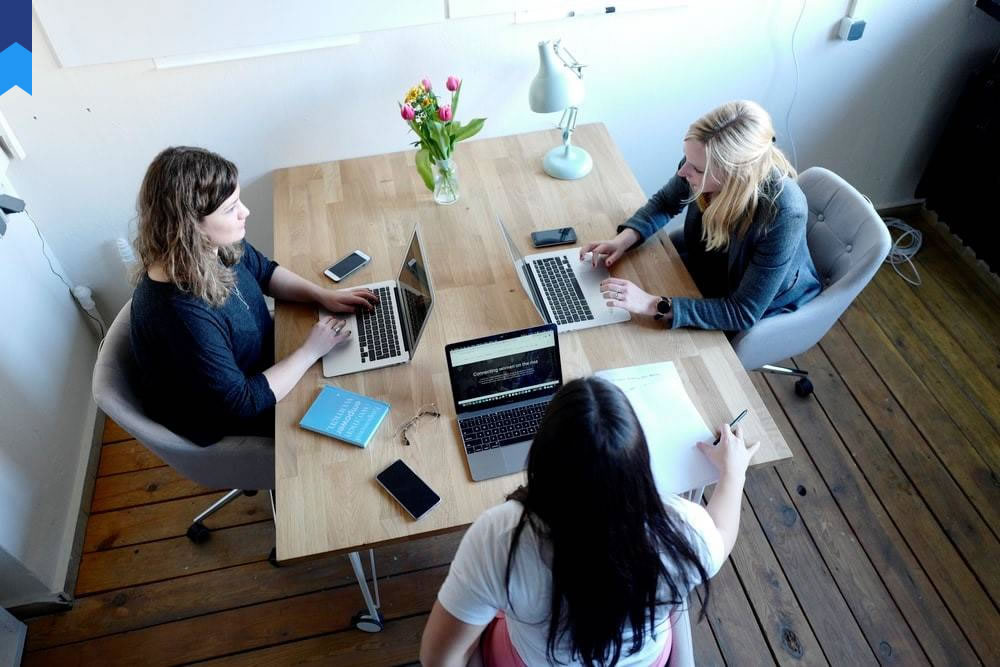
Unlocking the Secrets of Go Concurrency: Mastering Goroutines and Channels
Go's concurrency model, built upon goroutines and channels, is a powerful tool that distinguishes it from other programming languages. This article delves into advanced techniques, moving beyond basic introductions to uncover the true potential of Go's concurrency features. We'll explore efficient resource management, sophisticated error handling, and innovative approaches to problem-solving. Understanding these nuances will dramatically improve the performance and robustness of your Go applications.
Efficient Resource Management with Goroutines
Goroutines provide lightweight concurrency, allowing thousands of concurrent operations without the overhead of traditional threads. Effective management of these goroutines is crucial for avoiding resource exhaustion. One effective approach is using context packages to manage the lifecycle of goroutines, enabling graceful shutdown and preventing resource leaks. For instance, a context can be canceled when a long-running operation needs to be stopped. This prevents the goroutine from consuming resources indefinitely.
Consider a scenario involving image processing. Each image is processed concurrently in a separate goroutine. With the context package, we can easily cancel these goroutines if the user decides to interrupt the process. This also prevents orphaned goroutines from accumulating and consuming system resources. This efficient resource management translates directly to improved application responsiveness and stability.
Another key aspect is properly handling goroutine leaks. A leaked goroutine is a goroutine that continues to run even after its work is complete, often because it doesn't have a way to signal its completion. This can lead to resource exhaustion, particularly in long-running applications. Proper use of wait groups or channels ensures all goroutines complete before the program exits, avoiding this common pitfall.
Case Study 1: A large-scale image processing application using goroutines for parallel processing experienced significant performance gains after implementing context-based cancellation for gracefully handling user interruptions and avoiding resource starvation. Case Study 2: A distributed system using goroutines for communication between nodes overcame issues with goroutine leaks by integrating wait groups into its design for improved reliability.
Effective error handling within concurrent code is paramount. Properly handling panics within goroutines to prevent cascading failures is crucial. Employing mechanisms like recover functions within goroutines can prevent a single failure from bringing down the entire application. Channels offer a structured approach to handling errors. Errors can be signaled along channels and collected by a dedicated goroutine, facilitating centralized error handling and logging.
Techniques like using channels to signal errors provides a robust mechanism. Instead of relying on panic, sending an error message across a channel gives more controlled error handling, improving application reliability. Consider a database interaction; sending an error message to a specific channel allows for graceful handling of database errors without halting other parts of the application.
Case Study 3: A microservice architecture utilizing goroutines for internal communication successfully improved resilience by using channels for error reporting. This method helped pinpoint the source of errors without halting the entire system. Case Study 4: A high-frequency trading application used channels for error signaling and recovery, ensuring continued operation even during transient failures and minimizing downtime.
Mastering Channels for Inter-Goroutine Communication
Channels are the primary mechanism for communication and synchronization between goroutines in Go. They provide a safe and efficient way to pass data between concurrently running functions. Beyond basic send and receive operations, understanding buffered channels, select statements, and channel directions unlocks more sophisticated concurrency patterns.
Buffered channels provide a mechanism for asynchronous communication. They allow a certain number of messages to be sent without blocking the sending goroutine, thus increasing concurrency. Understanding buffer size optimization is vital for performance tuning. An adequately sized buffer can significantly improve throughput, while an overly large buffer might consume excessive memory.
The select statement provides a powerful way to handle multiple channels concurrently. It allows a goroutine to wait on multiple channels and react to the first one that becomes ready. This is crucial in scenarios where a goroutine needs to respond to events from multiple sources.
Case Study 5: A real-time chat application used buffered channels to handle incoming messages asynchronously, preventing blocking and maintaining responsiveness. Case Study 6: A network server using the select statement handled connections from multiple clients concurrently, significantly increasing throughput and scalability.
Channel directions (unidirectional channels) enforce data flow, enhancing type safety and preventing accidental misuse. Sending-only channels prevent accidental reads, and receiving-only channels prevent accidental writes. This improves code clarity and reduces potential errors, which is particularly useful in complex concurrent systems.
Unidirectional channels contribute to cleaner code and improved maintainability by explicitly defining the direction of data flow. Using these helps prevent subtle errors and enhances predictability in concurrent programming. This improves the readability and maintainability of your codebase, especially valuable in larger projects.
Case Study 7: A distributed data processing pipeline benefited from using unidirectional channels to enforce data flow, ensuring data integrity and preventing deadlocks. Case Study 8: A concurrent data transformation task improved its reliability through unidirectional channels, making it easier to understand and maintain.
Advanced Concurrency Patterns
Go's concurrency features extend beyond simple goroutines and channels. Advanced patterns, such as worker pools and fan-out/fan-in, significantly improve application efficiency and scalability. Understanding and implementing these patterns is essential for building robust and high-performance Go applications.
Worker pools provide a way to manage a fixed number of worker goroutines. Tasks are submitted to the pool, and worker goroutines pick up tasks and process them concurrently. This efficiently utilizes resources, especially in scenarios with many short-lived tasks. Properly sizing the worker pool is crucial; too few workers lead to underutilization, while too many can lead to context switching overhead.
The fan-out/fan-in pattern is another valuable technique. Fan-out involves splitting a task into multiple subtasks processed concurrently. Fan-in then collects the results from these subtasks. This pattern is ideal for parallel processing of large datasets or complex computations.
Case Study 9: A large-scale data processing application leveraged a worker pool to efficiently process incoming data streams, improving throughput and scalability. Case Study 10: A distributed search engine used the fan-out/fan-in pattern to search multiple indexes concurrently, significantly reducing search times.
Efficient synchronization primitives, such as mutexes and atomic operations, are vital for managing shared resources across multiple goroutines. While simple, improper use can lead to deadlocks or race conditions. Careful consideration of these aspects is critical for building reliable concurrent applications.
Mutexes (mutual exclusion) ensure that only one goroutine can access a shared resource at a time, preventing race conditions. Atomic operations provide atomic updates to shared variables without the need for explicit locking, improving performance in specific scenarios.
Case Study 11: A shared counter application avoided race conditions by using a mutex to protect the shared counter variable. Case Study 12: A high-performance caching system used atomic operations to update cache entries without locking, improving performance.
Testing and Debugging Concurrent Go Code
Testing and debugging concurrent Go code presents unique challenges due to the non-deterministic nature of concurrency. Standard testing techniques are insufficient, necessitating specialized approaches to ensure correctness and identify subtle issues.
Race detectors are essential tools for identifying race conditions. The Go toolchain provides a built-in race detector that can detect data races during program execution. Running tests with the race detector enabled is crucial for finding concurrency bugs early in the development process.
Systematic testing of concurrent code requires careful design and execution of test cases that cover various execution scenarios. Testing must include scenarios that stress the concurrency model, like high concurrency loads or network disruptions. These tests are vital to verifying the resilience and reliability of the system under stressful conditions.
Case Study 13: A payment processing system used race detection to identify and fix a race condition that could lead to incorrect transaction processing. Case Study 14: A distributed file system used stress tests with high concurrency loads to ensure its stability and data integrity under pressure.
Debugging concurrent code requires specialized techniques. Debugging tools like the Go debugger, coupled with logging and tracing, can provide valuable insights into the execution flow of concurrent programs. Understanding the order of events and the interactions between goroutines is essential for effective debugging.
Logging and tracing provide visibility into the state of concurrent operations. Logging key events or variables helps to trace the execution of individual goroutines, while tracing provides a broader view of the interaction of multiple goroutines. This detailed information is critical to understand and fix errors related to inter-goroutine communication and resource sharing.
Case Study 15: A real-time analytics dashboard used logging to pinpoint the source of a performance bottleneck related to excessive context switching. Case Study 16: A microservice application used distributed tracing to identify and fix a deadlock between two interacting services.
Conclusion
Go's concurrency model offers unmatched power and efficiency. Beyond the basics, mastering advanced techniques like efficient resource management, sophisticated channel usage, and advanced concurrency patterns is crucial for building robust and high-performance applications. A commitment to thorough testing and debugging using specialized tools is essential for maintaining the reliability and stability of concurrent Go programs. This detailed understanding of Go's concurrency model enables developers to create sophisticated, scalable, and resilient applications that excel in complex computational environments.
By embracing the intricacies of Go's concurrency model, developers can unlock a world of possibilities, developing applications that are not only efficient but also robust and capable of handling challenging concurrency scenarios with elegance and grace. This journey extends beyond simple introductions, demanding a deeper understanding of efficient resource management, intricate channel interactions, and advanced concurrency patterns. The rewards, however, are substantial: highly performant, reliable, and scalable applications that can address complex real-world problems effectively.