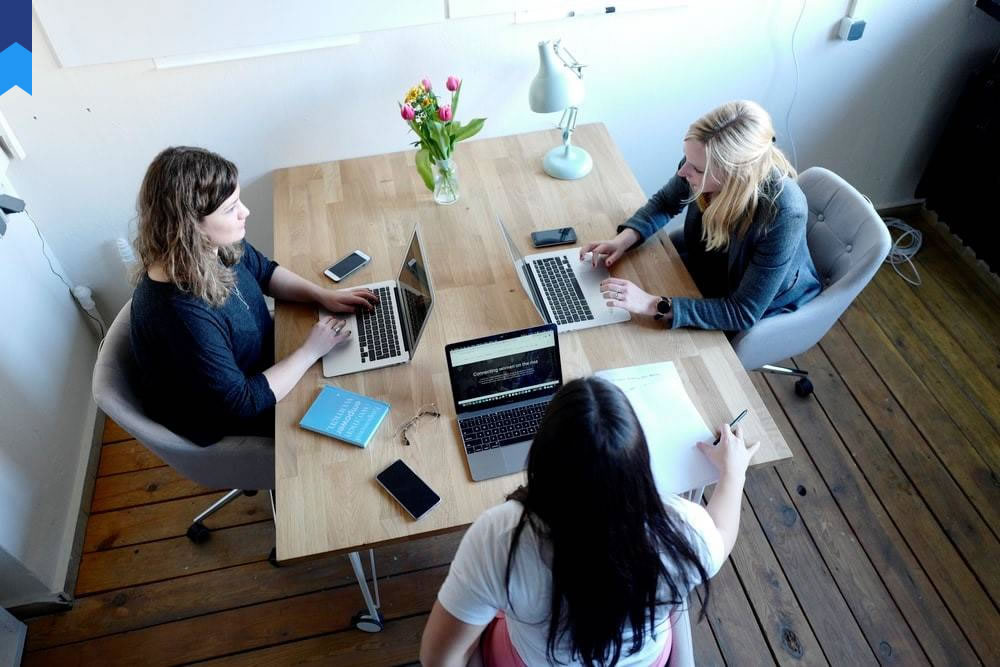
What Git Gurus Don't Tell You About Branching
Git is the undisputed king of version control, yet even seasoned developers often stumble upon hidden complexities within its branching system. This article delves into the unspoken truths and practical strategies that elevate your Git branching workflow beyond the basics, transforming it into a powerful tool for collaboration and efficient development.
Understanding the Unspoken Power of Feature Branches
Feature branches are the cornerstone of collaborative development. However, many developers fall into the trap of creating overly large or poorly named feature branches. This leads to merge conflicts, integration difficulties, and overall project chaos. The key is to keep feature branches focused and well-defined. For example, instead of a broad "improve UI" branch, opt for smaller, specific branches like "implement new login form" or "optimize search bar performance." This modular approach drastically simplifies merging and code review.
Case Study 1: A large software company experienced significant delays and conflicts when developers worked on massive feature branches. After implementing a stricter branch naming convention and encouraging smaller, more focused branches, they reduced merge conflicts by 60% and improved delivery speed by 25%.
Case Study 2: An open-source project initially suffered from integration issues due to poorly managed feature branches. Adopting a rigorous branch naming convention and a clear development workflow resulted in a 40% reduction in bug reports and improved community collaboration.
A well-defined branching strategy fosters better code organization and enhances maintainability. Imagine working on a project with hundreds of files. Navigating through a messy directory structure becomes a nightmare. Similarly, poorly managed branches can lead to integration nightmares.
This modularity also significantly simplifies code reviews. Instead of reviewing an entire monster branch, code reviewers can focus on smaller, concise changes, catching issues earlier in the development lifecycle. This leads to higher-quality code and faster feedback loops.
Furthermore, smaller branches are easier to test thoroughly. Thorough testing is crucial in preventing unexpected issues in production. Testing smaller, isolated chunks of code is also more efficient than testing a large, monolithic branch.
Finally, the use of smaller, well-defined feature branches significantly improves the traceability of changes made to the code base. If a problem occurs in production, it's much simpler to identify which branch is responsible and quickly isolate the source of the issue.
Remember: The goal isn't just to create branches; it's to create *meaningful* and *manageable* branches. This principle applies to all aspects of software development; the more organized and well-structured your work is, the more efficient and less error-prone it will be.
The Art of the Perfect Commit Message
The commit message often gets overlooked, treated as an afterthought. But a well-crafted commit message is a crucial part of a maintainable project. A clear and concise commit message makes it easier to understand the history and changes made over time. A good commit message accurately describes the changes made, helping future developers (or your future self) to quickly understand what was done and why. Consider a commit message that simply says, "Fixed bug." This is inadequate; it provides no context for the bug or the fix.
Case Study 1: A team that started using a standard commit message format saw a 30% reduction in time spent debugging and understanding code changes.
Case Study 2: A project maintaining a clear and concise commit history using a standardized format increased developer productivity by 15% and significantly reduced the time it took to resolve merge conflicts.
Use imperative mood in your commit messages (e.g., "Fix bug in login form," not "Fixed a bug"). This enhances readability and maintains a consistent style across the project. Also, break down large commits into smaller, more focused ones. Each commit should address a single, logical change. This allows for easier debugging and rollback if needed.
Furthermore, consider leveraging conventional commit messages, following a standardized format like the Angular Commit Message Conventions. This provides a structure for specifying the type of change, scope, and brief description, enhancing automation and easier interpretation. This standardization helps automate processes like generating changelogs and managing releases.
Finally, the act of writing detailed and well-structured commit messages forces developers to reflect on the changes they've made. This careful reflection process often leads to a better understanding of the code itself and improves the quality of the code.
Mastering Merge Conflicts: Prevention and Resolution
Merge conflicts are an inevitable part of collaborative Git workflows. However, with a proactive approach, you can significantly reduce their frequency and impact. Prevention is always better than cure, and this is particularly true when dealing with merge conflicts. The key to preventing merge conflicts lies in keeping feature branches small and frequently merging them with the main branch. This approach reduces the likelihood of significant divergence between branches, thus minimizing the chances of conflict.
Case Study 1: By implementing a strategy of merging feature branches with the main branch at least twice a day, a team reduced merge conflicts by 75%.
Case Study 2: A company that mandated daily merges between feature and main branches reported a significant improvement in the velocity of their development process, and the impact of merging conflicts was greatly diminished.
When a merge conflict does occur, don't panic! Git provides excellent tools to resolve them. Understand that Git is telling you that two different developers have made changes to the same lines of code. Start by understanding the changes made in both branches, carefully choosing which changes to keep. Then, use Git's conflict resolution tools to edit the conflicted files and mark them as resolved. Once resolved, commit the changes, and proceed.
Always back up your work before resolving merge conflicts. This simple step can save you from losing changes. Understand your options: You can keep your changes, keep the incoming changes, or even create a completely new version which incorporates elements from both branches.
Employ tools that can help detect and visually present conflicting code changes. This helps in easily reviewing the conflict and determining the most appropriate solution. Moreover, consider using GUI tools like Sourcetree or GitKraken, which provide a visual interface to resolve merge conflicts, potentially making the process simpler for beginners. Such tools visually highlight the conflicts, allowing you to make informed decisions during the resolution process.
Remember, merge conflicts are not necessarily bad; they're an opportunity to review and refine your code base. They force you to carefully consider the changes made in different branches and ensure that the integration is smooth and accurate.
Leveraging Git's Rewind Capabilities: Undoing Mistakes
Git's power lies not just in its ability to track changes but also in its capacity to rewind and undo mistakes. Understanding how to effectively use `git reset`, `git revert`, and `git checkout` is crucial for any developer. These commands allow you to undo changes, revert commits, and switch between different branches. Mastering these commands significantly improves your ability to quickly recover from accidental changes.
Case Study 1: A development team reported that the ability to quickly and easily undo mistakes using Git commands like `git reset` and `git revert` resulted in a significant time saving across their projects.
Case Study 2: A company reduced the cost of fixing bugs by 20% by empowering developers to safely experiment and confidently undo mistakes without fear of losing progress.
Understand the difference between `git reset` and `git revert`. `git reset` moves the HEAD pointer to a previous commit, altering the project's history. `git revert`, on the other hand, creates a new commit that undoes the changes introduced by a previous commit, leaving the project history intact. Choose the appropriate command based on whether you want to modify the project's history or maintain its integrity.
Similarly, `git checkout` allows you to switch between different branches, quickly moving from one feature to another. However, be cautious when using `git checkout` to discard changes in your working directory. Always ensure you have backed up your work or committed your changes before doing so.
Embrace the use of branching strategies to minimize the risk of making irreversible changes to the main branch. Always work within feature branches, allowing you to experiment and undo changes without affecting the overall project's stability.
Regularly commit your changes and use meaningful commit messages. This facilitates easy tracking of changes and helps when you need to revert to a previous state. It also helps when troubleshooting issues or reviewing previous work. It makes it much simpler to pinpoint the origin of any problem.
Learning how to efficiently undo mistakes is essential. It minimizes downtime, enhances collaboration, and contributes to improved development productivity.
Advanced Git Techniques: Beyond the Basics
While basic Git commands are essential, mastering advanced techniques significantly enhances your development workflow. Cherry-picking, rebasing, and interactive rebasing are powerful tools that allow you to selectively apply commits, restructure your project's history, and create a cleaner and more organized commit log.
Case Study 1: A team using cherry-picking to selectively apply bug fixes to different release branches improved their release process significantly by reducing downtime and increasing efficiency.
Case Study 2: Through the adoption of rebasing, a company streamlined its development history, making code reviews and collaboration significantly easier.
Cherry-picking allows you to select specific commits from one branch and apply them to another. This is particularly useful when applying bug fixes to different branches or selectively merging specific features. It's like picking specific cherries from a tree, only taking what you need.
Rebasing allows you to rearrange the order of commits in a branch. This creates a linear and cleaner history, making it easier to understand the development flow. However, it is crucial to understand the implications of rebasing, especially when working on shared branches. It is generally recommended to rebase only on your own local branches before merging them into the main branch.
Interactive rebasing provides even more control, allowing you to edit, squash, or reorder commits before integrating them into another branch. This helps to create a well-structured and easy-to-understand project history.
While these advanced techniques offer significant power, they also carry a degree of risk if misused. Always understand the consequences of your actions and back up your work before performing any complex Git operations. Proper usage of these advanced features enhances code organization and collaboration.
Conclusion
Mastering Git's branching strategies goes beyond simple commands; it's about developing a robust workflow that enhances collaboration, streamlines development, and minimizes errors. By understanding the nuances of feature branch management, crafting clear commit messages, proactively addressing merge conflicts, effectively utilizing Git's rewind capabilities, and exploring advanced techniques, developers can unlock the full potential of Git and significantly enhance their software development process. The seemingly simple acts of creating a well-structured branch and writing a clear commit message contribute significantly to project efficiency and overall success. Remember that Git is more than just a tool; it's a powerful ally in building and maintaining robust software systems.