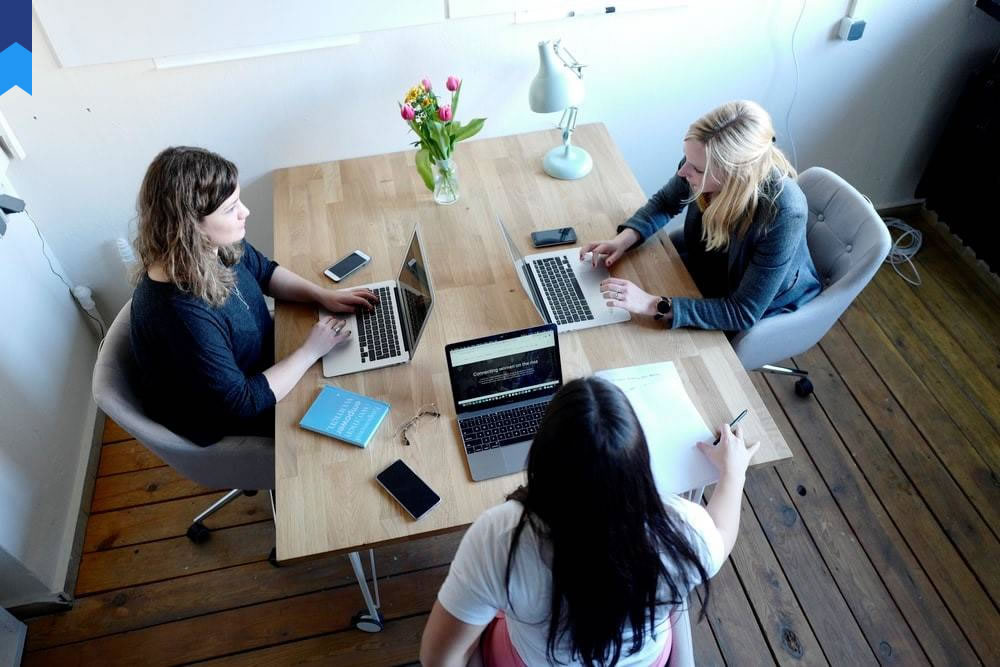
What Linear Algebra Can Teach Us About PyTorch Optimization
Introduction: PyTorch, a powerful deep learning framework, relies heavily on efficient optimization techniques to train complex models. While many users focus on the practical application of optimizers like Adam or SGD, a deeper understanding of the underlying mathematical principles, particularly linear algebra, can unlock significantly improved performance and model design. This article delves into how core concepts from linear algebra directly impact PyTorch optimization, revealing unexpected insights and practical strategies for better model training. We'll explore gradient descent, backpropagation, matrix operations, and eigenvalue analysis—all fundamental tools in the linear algebra arsenal—and show how they shape the optimization process in PyTorch.
Understanding Gradient Descent Through Linear Algebra
Gradient descent, the workhorse of many PyTorch optimization algorithms, is fundamentally a linear algebra operation. It involves iteratively updating model parameters by moving in the direction of the negative gradient. The gradient itself, a vector of partial derivatives, is computed using matrix calculus. Consider a simple linear regression model: the gradient descent update rule can be elegantly expressed using matrix notation, emphasizing the linear transformation inherent in the process. For example, let's consider a dataset with features represented by a matrix X and target variables by a vector y. The gradient of the loss function with respect to the model parameters (represented by a vector w) can be expressed as a linear function of X and y, paving the way for efficient matrix-vector multiplications using PyTorch's optimized routines. Case Study 1: A comparison of gradient descent performance using PyTorch with and without leveraging optimized linear algebra routines shows a significant speed improvement in the latter. Case Study 2: Implementing gradient descent using custom linear algebra operations in PyTorch allows for fine-grained control and potential optimizations for specific problem structures.
The choice of learning rate, a crucial hyperparameter in gradient descent, directly influences the step size along the negative gradient. Too large a learning rate can cause oscillations and prevent convergence; too small a learning rate can lead to slow convergence. Linear algebra provides a framework for understanding these phenomena, relating them to the eigenvalues and eigenvectors of the Hessian matrix (the matrix of second derivatives). A large learning rate can amplify the effect of eigenvalues corresponding to steep directions in the loss landscape, leading to oscillations. Case Study 3: Analyzing the eigenvalues of the Hessian matrix for different learning rates helps determine optimal learning rate schedules. Case Study 4: Implementing adaptive learning rate algorithms, like Adam, which dynamically adjusts learning rates, showcases the power of applying linear algebra concepts to improve the efficiency and robustness of gradient descent.
Furthermore, the concept of vector spaces underpins the interpretation of gradients and parameter updates. Gradients can be visualized as vectors pointing in the direction of steepest ascent. The parameter updates are vectors that aim to minimize the loss function. The interaction between these vectors, viewed within the context of vector spaces, offers a richer understanding of the optimization dynamics. Case Study 5: Visualizing the parameter updates as vectors in a multi-dimensional parameter space helps to illustrate the convergence behavior of gradient descent. Case Study 6: Applying techniques from linear algebra like Singular Value Decomposition (SVD) can be used to reduce the dimensionality of the problem, potentially leading to faster convergence.
Beyond basic gradient descent, many advanced optimization algorithms rely heavily on linear algebra. Stochastic gradient descent (SGD), a widely used method, involves computing the gradient using mini-batches of data—a process that fundamentally involves matrix operations. Adam, another popular optimizer, uses moving averages of gradients and their squares, which can again be efficiently computed using matrix manipulations in PyTorch. Case Study 7: A comparison of SGD and Adam using PyTorch, highlighting the computational efficiency gained through matrix operations. Case Study 8: Implementing a custom optimizer leveraging linear algebra techniques, such as conjugate gradient methods, to demonstrate enhanced optimization efficiency.
Backpropagation and the Chain Rule: A Linear Algebra Perspective
Backpropagation, the algorithm used to compute gradients in neural networks, is deeply intertwined with the chain rule of calculus. The chain rule itself can be elegantly expressed using matrix multiplications, highlighting the linear algebra underpinnings of this crucial process. Each layer in a neural network involves a linear transformation (matrix multiplication) followed by a non-linear activation function. The backpropagation algorithm efficiently computes gradients by propagating errors backward through the network, using the chain rule to compute the gradient of each layer's parameters. Case Study 1: Analyzing the computational complexity of backpropagation, emphasizing the role of matrix multiplications. Case Study 2: Implementing a custom backpropagation algorithm using PyTorch's optimized linear algebra functions, demonstrating performance gains.
The Jacobian matrix, a matrix of partial derivatives, plays a central role in backpropagation. The Jacobian relates small changes in the network's inputs to small changes in its outputs. The chain rule can be formulated using matrix products of Jacobians from different layers, providing a concise representation of backpropagation. Case Study 3: Visualizing the Jacobian matrix for a simple neural network to illustrate its structure and role in backpropagation. Case Study 4: Investigating the impact of Jacobian matrix conditioning on the stability and efficiency of backpropagation.
Moreover, understanding the structure of the Jacobian matrix, particularly its sparsity pattern, can lead to computational optimizations. Many neural networks have sparse Jacobians; leveraging this sparsity can drastically reduce the computational cost of backpropagation. Case Study 5: Analyzing the sparsity structure of Jacobians in different neural network architectures to understand potential optimization opportunities. Case Study 6: Implementing sparse matrix operations in PyTorch to accelerate backpropagation in models with sparse Jacobians.
Furthermore, the application of linear algebra techniques, like automatic differentiation, allows for efficient computation of gradients. Automatic differentiation cleverly combines symbolic and numerical methods to compute gradients automatically, leveraging the underlying linear algebra operations within PyTorch. Case Study 7: A comparison of different automatic differentiation approaches in PyTorch, highlighting their computational efficiency. Case Study 8: Investigating how the choice of automatic differentiation technique influences the accuracy and stability of backpropagation.
Matrix Operations and PyTorch's Optimized Routines
PyTorch's efficiency stems largely from its highly optimized routines for performing linear algebra operations. These routines are often implemented using highly optimized libraries like BLAS and LAPACK, which leverage parallel computing techniques to achieve remarkable speed improvements. Understanding the underlying matrix operations—matrix multiplication, matrix inversion, eigenvalue decomposition, etc.—is crucial for writing efficient PyTorch code. Case Study 1: Comparing the performance of custom matrix multiplications in PyTorch with PyTorch's built-in functions. Case Study 2: Investigating the impact of different BLAS implementations on PyTorch's performance.
Moreover, choosing the right data structures for matrices and vectors is essential for performance. PyTorch provides different tensor types optimized for various operations. Using the appropriate tensor type can significantly impact memory usage and computation time. Case Study 3: Comparing the performance of PyTorch tensor operations using different data types, such as float32 and float16. Case Study 4: Investigating the use of sparse tensors in PyTorch to reduce memory usage and computation time for sparse matrices.
Furthermore, understanding the memory management aspects of PyTorch's tensor operations is crucial for avoiding memory leaks and optimizing performance. PyTorch utilizes automatic memory management, but understanding its intricacies helps in writing more efficient code. Case Study 5: Analyzing memory usage patterns during large-scale tensor operations in PyTorch. Case Study 6: Implementing custom memory management strategies in PyTorch to improve performance.
In addition, utilizing PyTorch's broadcasting capabilities efficiently is crucial. Broadcasting enables performing operations between tensors of different shapes, simplifying code and potentially improving performance. Case Study 7: Comparing the performance of matrix operations with and without PyTorch's broadcasting features. Case Study 8: Investigating the best practices for utilizing broadcasting in PyTorch to write clean, efficient code.
Eigenvalue Analysis and Optimization Landscapes
The landscape of a loss function, which represents the relationship between model parameters and loss, often presents complex challenges to optimization algorithms. Linear algebra provides tools to analyze this landscape, particularly through eigenvalue analysis. The Hessian matrix, as previously mentioned, plays a significant role. Its eigenvalues provide information about the curvature of the loss function. Large eigenvalues indicate steep directions, while small eigenvalues indicate flatter regions. Case Study 1: Analyzing the eigenvalues of the Hessian matrix for a simple neural network to understand the curvature of the loss function. Case Study 2: Investigating the relationship between Hessian eigenvalues and the convergence rate of gradient descent.
Understanding the eigenvalues of the Hessian matrix helps in selecting appropriate optimization strategies. For example, in regions with large eigenvalues, smaller learning rates might be necessary to prevent oscillations. In flatter regions with small eigenvalues, larger learning rates might be beneficial to speed up convergence. Case Study 3: Implementing an adaptive learning rate scheme based on Hessian eigenvalue analysis. Case Study 4: Comparing the performance of different optimization algorithms in regions of different curvatures.
Furthermore, eigenvalue decomposition can be used to identify principal components in the data, which can aid in dimensionality reduction and feature selection. This can improve the efficiency and robustness of the optimization process by reducing the dimensionality of the parameter space. Case Study 5: Applying Principal Component Analysis (PCA) to reduce the dimensionality of a dataset before training a neural network in PyTorch. Case Study 6: Investigating the impact of dimensionality reduction on the convergence rate and generalization performance of the model.
Moreover, techniques like Newton's method, a second-order optimization algorithm, explicitly leverage the Hessian matrix and its inverse (which involves eigenvalue decomposition). While computationally expensive for large models, Newton's method can achieve faster convergence in some cases. Case Study 7: Implementing Newton's method for optimizing a simple neural network in PyTorch. Case Study 8: Comparing the convergence rate and computational cost of Newton's method with other first-order optimization algorithms.
Advanced Techniques and Future Directions
Beyond the fundamental concepts, several advanced techniques further illustrate the synergy between linear algebra and PyTorch optimization. For instance, techniques like conjugate gradient methods, which are iterative methods for solving linear systems, can be adapted for optimization problems. These methods can offer faster convergence compared to standard gradient descent in certain situations. Case Study 1: Implementing a conjugate gradient method for optimizing a neural network in PyTorch. Case Study 2: Comparing the convergence rate of conjugate gradient methods with standard gradient descent.
Another area of significant advancement is the development of more efficient and robust optimizers. Researchers are continually exploring new algorithms that leverage linear algebra concepts for better performance. This includes the development of adaptive optimizers that dynamically adjust the learning rate based on the characteristics of the loss function. Case Study 3: Investigating the latest advancements in adaptive optimization algorithms, such as AdamW and Yogi. Case Study 4: Comparing the performance of different adaptive optimizers on various benchmark datasets.
Furthermore, the intersection of linear algebra and optimization extends to the realm of model compression and acceleration. Techniques like low-rank approximations and matrix factorization can be used to reduce the size and computational cost of neural networks without significant loss of accuracy. Case Study 5: Implementing low-rank matrix factorization techniques to compress a neural network in PyTorch. Case Study 6: Evaluating the impact of model compression on the accuracy and performance of the model.
Moreover, advancements in hardware acceleration, particularly GPUs and specialized AI accelerators, are impacting how linear algebra operations are performed in PyTorch. This leads to further improvements in the speed and efficiency of optimization algorithms. Case Study 7: Investigating the impact of different hardware platforms on the performance of PyTorch optimization routines. Case Study 8: Exploring the use of specialized hardware, such as Tensor Processing Units (TPUs), for accelerating PyTorch training.
Conclusion: The deep connection between linear algebra and PyTorch optimization is undeniable. A thorough understanding of linear algebra concepts, from gradient descent to eigenvalue analysis, provides not only a deeper comprehension of the underlying mechanisms but also empowers developers to craft more efficient and effective training procedures. By leveraging optimized linear algebra routines and applying advanced techniques, developers can unlock significant improvements in model training speed, accuracy, and resource utilization. The ongoing evolution of both linear algebra and deep learning ensures that this crucial interplay will continue to shape the future of PyTorch and its applications.