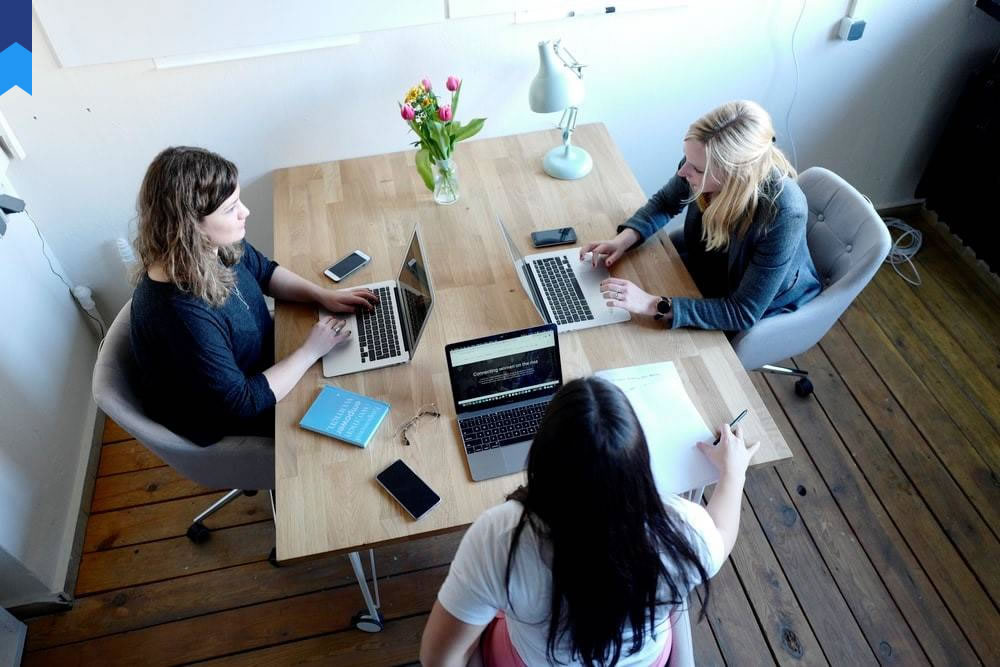
How to Auto-Generate your Providers with Flutter Riverpod Generator?
How to Auto-Generate your Providers with Flutter Riverpod Generator?
Learning the fundamental concept of the Flutter Riverpod repository is crucial. It will help you know the primary role of the Flutter Riverpod and how you can auto-generate your provider. Flutter Riverpod offers six different types of providers that the developers can use. But, writing several providers manually is an error-prone task. Therefore, selecting one provider is quite a challenging task.
But now, you can easily annotate the code with Riverpod and allow the build_runner to generate each provider on the fly. However, you can consult with Flutter developers at www.flutteragency.com if you do not have expertise in this. Here, we will discuss how you can auto-generate the providers easily with the Flutter Riverpod generator.
An Introduction to the Flutter Riverpod
Riverpod is one of the agile data binding and reactive caching frameworks for Dart or Flutter. It takes care of the state management on its own. It is a unique framework that can fetch, combine, cache, and recompute network requests. So, it eliminates the chances of manual errors. It is mainly a reconstruction of the Provider package, further indicating that the Flutter Riverpod reduces the dependencies.
The major advantages of using Riverpod over the providers are:
- There is no need to get the 'provider not found' exception.
- Users can facilitate the combinations of the providers significantly instead of the error-prone and tedious ProxyProvider.
- You can also define the global providers.
Use Cases
Now, you must be thinking about its use cases to understand the Flutter Riverpod in a better way. You can consult with Flutter developers for a better understanding. However, here is the list of the use cases of the Flutter Riverpod.
- It catches the programming errors at the compile-time instead of the runtime.
- The Riverpod can fetch data and cache and then update it from remote sources.
- This specific framework can update your UI quickly and seamlessly.
- It depends on the computed or asynchronous state.
- Users can write testable codes and keep the logic away from the widget tree.
- It allows the users to create, combine, and use the providers using the minimal boilerplate code.
- This framework will help you to dispose of a provider's status when it is no longer in use.
How Can You Auto Generate the Providers Using Flutter Riverpod Generator?
Writing Flutter apps with the help of Riverpod has become pretty straightforward and time-saving. When talking about auto-generating the providers using the Flutter Riverpod generator, many things need to be covered. However, you can consult with Flutter developers to learn more about the entire process.
In this article, we will discuss the fundamental things, such as how you can generate the providers from the functions with the new @riverpod syntax. Hence, this section will cover how you can declare the providers using @riverpod syntax, pass the arguments to a provider that overrides the old family modifier's constraints, and convert the FutureProvider to the new syntax. So, let's begin without delay.
Step 1: New Project and the Mock Data
First, you must create the new project and include a data folder in your root directory. You have to update the pubspec.yaml file to access and use the data folder.
dependencies:
# orflutter_riverpod/hooks_riverpod as per https://riverpod.dev/docs/getting_started
riverpod:
# the annotation package containing @riverpod
riverpod_annotation:
dev_dependencies:
# a tool for running code generators
build_runner:
# the code generator
riverpod_generator:
You can begin the code generator in the 'watch' mode. So, for that to happen, you must run the 'dart run build_runner watch' command on the terminal. However, if you encounter version conflict, you may try another one,' flutter pub run build_runnerwatch .' It will look after every dart file in your new project. Additionally, it will update your generated code automatically when you make any changes.
Step 2: Produce the First Annotated Provider
Now it is time to proceed by producing some providers. In this step, you must begin with creating some simple providers.
// dio_provider.dart
import 'package:dio/dio.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
// a provider for the Dio client to be used by the rest of the app
finaldioProvider = Provider<Dio>((ref) {
returnDio();
});
After creating the providers, you should modify the files to use the new syntax.
import 'package:dio/dio.dart';
// 1. import the riverpod_annotation package
import 'package:riverpod_annotation/riverpod_annotation.dart';
// 2. add a part file
part 'dio_provider.g.dart';
// 3. use the @riverpod annotation
@riverpod
// 4. update the declaration
Diodio(DioRef ref) {
returnDio();
}
After you save this file, the build_runner will start working and create dio_provider. g. dart in that same data folder. Your new .g. dart files will automatically generate with the existing ones. Hence, there is no need to make any changes to your folder structure. However, you will see the following structure when you open your generated file.
// GENERATED CODE - DO NOT MODIFY BY HAND
part of 'dio_provider.dart';
// **************************************************************************
// RiverpodGenerator
// **************************************************************************
// ignore_for_file: avoid_private_typedef_functions, non_constant_identifier_names, subtype_of_sealed_class, invalid_use_of_internal_member, unused_element, constant_identifier_names, unnecessary_raw_strings, library_private_types_in_public_api
/// Copied from Dart SDK
class _SystemHash {
_SystemHash._();
staticint combine(int hash, int value) {
// ignore: parameter_assignments
hash = 0x1fffffff & (hash + value);
// ignore: parameter_assignments
hash = 0x1fffffff & (hash + ((0x0007ffff & hash) << 10));
return hash ^ (hash >> 6);
}
staticint finish(int hash) {
// ignore: parameter_assignments
hash = 0x1fffffff & (hash + ((0x03ffffff & hash) << 3));
// ignore: parameter_assignments
hash = hash ^ (hash >> 11);
return 0x1fffffff & (hash + ((0x00003fff & hash) << 15));
}
}
String $dioHash() => r'58eeefbd0832498ca2574c1fe69ed783c58d1d8f';
/// See also [dio].
finaldioProvider = AutoDisposeProvider<Dio>(
dio,
name: r'dioProvider',
debugGetCreateSourceHash:
constbool.fromEnvironment('dart.vm.product') ? null : $dioHash,
);
typedefDioRef = AutoDisposeProviderRef<Dio>;
You will notice some relevant things in this specific file. The file will contain the required dioProvider along with extra properties you need for debugging. Also, it defines the DioRef type like AutoDisposeProviderRef<Dio>. So, it implies that you have to write the following code.
part 'dio_provider.g.dart';
@riverpod
Diodio(DioRef ref) {
returnDio();
}
After this, the Riverpod generator will produce the corresponding DioRef and the DioProvider type, which you must pass as arguments to your function. Each provider produced with the Riverpod generator will, by default, use the autoDispose modifier.
Step 3: Produce a Provider For the Repository Class
After getting the dioProvider, you must try using it somewhere. For instance, let's think you have a MoviesRepository class which will further define a few techniques to fetch the movie's data.
You may write the following code to produce a provider for this specific repository.
part 'movies_repository.g.dart';
@riverpod
MoviesRepositorymoviesRepository(MoviesRepositoryRef ref) =>MoviesRepository(
client: ref.watch(dioProvider), // the provider we defined above
apiKey: Env.tmdbApiKey, // a constant defined elsewhere
);
As a result, the Flutter Riverpod generator will produce the MoviesRepositoryProvider along with the MoviesRepositoryRef type for you. While producing the providers for any repository, do not include the @riverpod annotation. Instead, produce an individual global function that will return an instance of the specific repository to annotate it.
Step 4: Produce and Read An Annotated FutureProvider
In this part, you should convert the FutureProvider to use @riverpod annotation.
@riverpod
Future<TMDBMovie>movie(
MovieRef ref, {
requiredintmovieId,
}) {
return ref
.watch(moviesRepositoryProvider)
.movie(movieId: movieId);
}
Then, you can watch the annotation inside the widget.
classMovieDetailsScreen extends ConsumerWidget {
constMovieDetailsScreen({super.key, required this.movieId});
finalintmovieId;
@override
Widget build(BuildContext context, WidgetRef ref) {
// movieId is a *named* argument
finalmovieAsync = ref.watch(movieProvider(movieId: movieId));
returnmovieAsync.when(
error: (e, st) => Text(e.toString()),
loading: () =>CircularProgressIndicator(),
data: (movie) =>SomeMovieWidget(movie),
);
}
}
As you may notice, movieId is mainly a named argument, as we have referred to it in the movie section.
@riverpod
Future<TMDBMovie>movie(
MovieRef ref, {
requiredintmovieId,
}) {
return ref
.watch(moviesRepositoryProvider)
.movie(movieId: movieId);
}
It simply implies that there is no need to limit to defining a specific provider family along with the single positional argument. You must define a specific function using a 'ref' object and positional arguments as many as you want. The Riverpod generator will look after the rest of them.
Conclusion
All in all, the Riverpod generator will bring several benefits to your table and give logical reasons to use it. It will generate the correct type of provider automatically, eliminating the chance of any error. Additionally, this framework offers a simplified process to create the providers with arguments.
Also, the biggest advantage of using the Riverpod is it offers less type errors during runtime and improves type safety. However, you can use it on your own or consult with Flutter developers to try this framework and to learn about features in flutter 3.7.
SIIT Courses and Certification
Also Online IT Certification Courses & Online Technical Certificate Programs