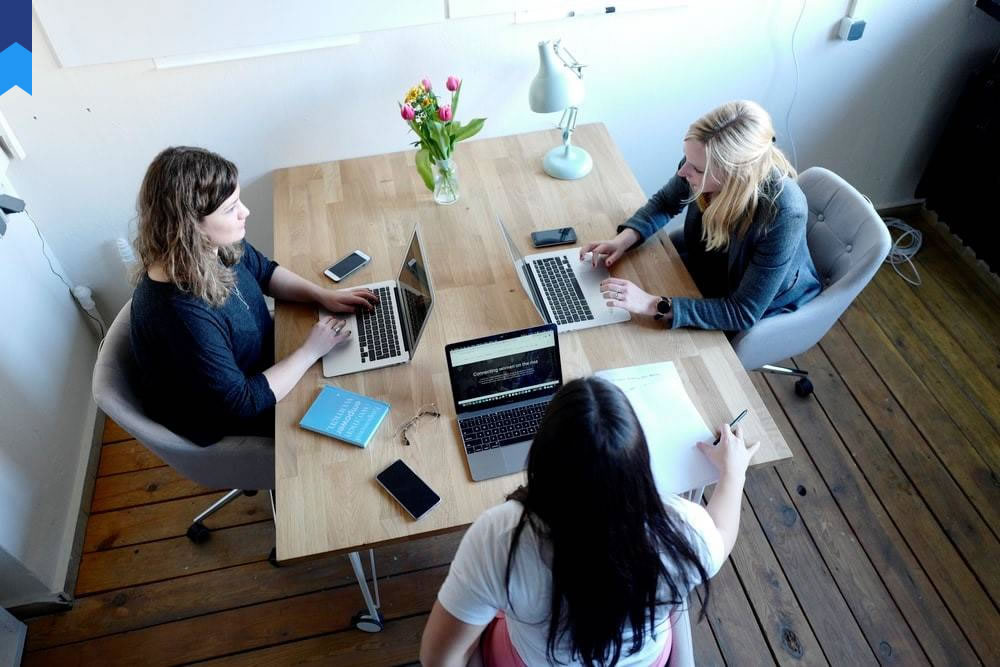
Algorithm Design: A Deep Dive Into Practical Optimization
Algorithm Design: A Deep Dive into Practical Optimization
Introduction
Designing and analyzing algorithms is fundamental to computer science, impacting everything from the speed of internet searches to the efficiency of medical imaging. This article delves beyond introductory concepts, exploring advanced techniques and practical considerations for optimizing algorithms. We'll examine how to choose the right algorithm for a given task, how to analyze its efficiency, and how to improve performance in real-world scenarios. We’ll uncover some counterintuitive truths about algorithm optimization, challenging conventional approaches and highlighting less-explored methods that yield significant improvements. This deep dive will equip you with the knowledge and strategies to create algorithms that are not just functional, but truly efficient and optimized.
Understanding Algorithm Complexity
Understanding algorithm complexity is crucial for effective algorithm design. Big O notation provides a standardized way to describe how the runtime or space requirements of an algorithm scale with the input size. For instance, a linear algorithm (O(n)) scales directly with input size, while a quadratic algorithm (O(n²)) scales proportionally to the square of the input size. Recognizing these complexities helps us predict performance and select algorithms suited to the scale of the problem. Consider searching: a linear search has O(n) complexity, while a binary search on a sorted array achieves O(log n), making it significantly faster for large datasets. Case study: Comparing linear search in a phone contact list versus binary search after sorting the list dramatically illustrates this difference in performance. Another case study: The impact of using a naive algorithm for sorting a million records versus using merge sort (O(n log n)). Furthermore, optimizing nested loops is critical in reducing quadratic complexities. Instead of looping through all elements twice, explore alternatives like hash tables or optimized data structures for faster lookups and significantly improved efficiency. Understanding the trade-offs between different complexity classes is key to designing performant algorithms. For instance, a space-optimized algorithm might prioritize lower memory usage at the cost of slightly higher runtime. Selecting the appropriate algorithm depends on the specific constraints and the relative importance of time versus space. This careful consideration of complexity is the foundation of efficient algorithm design.
Advanced Optimization Techniques
Beyond basic algorithm selection, advanced optimization techniques can significantly enhance performance. Dynamic programming breaks down complex problems into smaller, overlapping subproblems, storing solutions to avoid redundant computations. For example, the Fibonacci sequence calculation can be drastically optimized using dynamic programming. Another example is the shortest path algorithm, Dijkstra's algorithm, which utilizes dynamic programming to efficiently find the shortest paths in a graph. Greedy algorithms make locally optimal choices at each step, aiming for a global optimum. A classic example is Huffman coding, which builds an optimal prefix-free binary code for data compression. Another example is Kruskal's algorithm for finding the minimum spanning tree in a graph. Divide and conquer strategies break problems into smaller subproblems, recursively solving them, and combining the results. Merge sort, a classic example of divide and conquer, showcases how this method achieves O(n log n) complexity, superior to many quadratic algorithms. Quick sort, while also utilizing this strategy, can demonstrate the importance of choosing efficient pivot selection strategies for optimal performance. Selecting the right optimization technique depends on the problem's structure and the algorithm's inherent properties. The challenge lies in correctly identifying where these techniques can be applied to yield the most impactful improvements.
Parallel and Distributed Algorithms
With the rise of multi-core processors and cloud computing, parallel and distributed algorithms have become increasingly important. These algorithms leverage multiple processors or machines to perform computations concurrently, significantly speeding up execution time. MapReduce, a widely used framework for distributed data processing, exemplifies how large datasets can be processed efficiently across multiple machines. Another example is parallel sorting algorithms, which divide the task of sorting among multiple cores, achieving significant speed improvements compared to sequential sorting methods. Parallel algorithms require careful consideration of synchronization, data sharing, and communication overhead to avoid performance bottlenecks. Case study: Comparing the performance of a parallel matrix multiplication algorithm against a sequential one highlights the benefits of parallelism, particularly for large matrices. Another case study: Analyzing the throughput of a distributed web search engine using map reduce shows the scalability and resilience provided by distributed computing. The design and implementation of parallel and distributed algorithms require specialized knowledge and expertise in distributed systems and concurrency control. Efficient communication and data partitioning are key to minimizing overhead and maximizing performance gains. Mastering these concepts unlocks the potential for significant performance improvements in tackling massive datasets and complex computational tasks.
Profiling and Performance Tuning
Profiling tools are indispensable for identifying performance bottlenecks in algorithms. These tools provide detailed information about the execution time of different parts of the code, allowing developers to focus their optimization efforts on the most critical areas. Visualizing performance with profiling data can help pinpoint specific lines of code or functions that are consuming excessive time. Case study: Using a profiler to identify a slow database query within a larger algorithm demonstrates the effectiveness of targeted optimization. Another case study: Analyzing the performance of a web application using profiling tools can reveal bottlenecks in network requests or database interactions, allowing for targeted optimizations. Performance tuning involves applying various techniques to improve algorithm performance based on profiling results. This may involve optimizing data structures, using more efficient algorithms, or improving the code’s efficiency. For instance, using optimized libraries or employing vectorization techniques can significantly enhance performance. Furthermore, algorithmic choices made early on have a significant impact on future optimization efforts. Choosing a well-suited algorithm from the outset minimizes the need for later, more extensive performance tuning. By carefully considering the design and implementation, proactive optimization reduces the need for time-consuming post-development improvements.
Conclusion
Designing and analyzing efficient algorithms is a multifaceted challenge demanding a deep understanding of theoretical concepts and practical optimization techniques. This article has explored advanced strategies beyond the basics, highlighting the importance of algorithm complexity analysis, advanced optimization methods like dynamic programming and greedy approaches, the power of parallel and distributed computing, and the crucial role of profiling and performance tuning. Mastering these techniques enables developers to build high-performance systems, capable of handling ever-growing datasets and complex computational problems. The ongoing evolution of hardware and software continues to shape the landscape of algorithm design, demanding constant adaptation and innovation in pursuit of ever-greater efficiency and scalability.