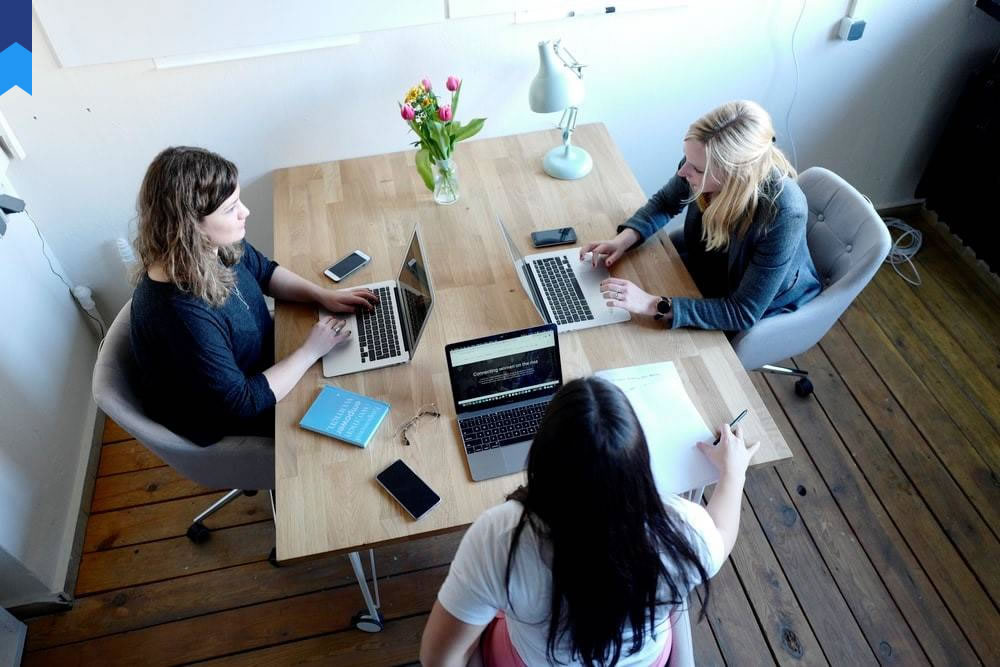
Algorithm Mastery: A Deep Dive
Introduction: Unlocking the power of algorithms is essential for anyone serious about computer science. This deep dive will move beyond superficial introductions, exploring practical applications and innovative techniques. We'll dissect complex concepts, providing concrete examples and real-world case studies to illuminate the often-abstract nature of algorithmic thinking. From basic sorting to advanced graph traversal, we'll equip you with the knowledge and tools to confidently tackle algorithmic challenges. Prepare to master the core principles and uncover the hidden elegance behind these fundamental building blocks of computation.
Sorting Algorithms: Beyond the Basics
Sorting algorithms form the bedrock of many computer science applications. Beyond the familiar bubble sort and insertion sort, we’ll delve into more efficient methods like merge sort and quicksort. Merge sort, known for its guaranteed O(n log n) time complexity, excels in handling large datasets. We'll examine its divide-and-conquer approach, illustrating how it recursively divides the problem into smaller subproblems until a solution is reached. A case study would be its application in large-scale data analysis, where the speed and consistency of merge sort are crucial for processing vast amounts of information. Conversely, quicksort, while offering similar average-case performance, can exhibit O(n^2) worst-case behavior. Understanding this trade-off is vital. We’ll analyze scenarios that lead to this worst-case scenario and explore strategies to mitigate it. A real-world example is its use in operating systems for scheduling processes based on priority. Understanding the nuances of both algorithms is key to selecting the right tool for the job. Different data structures can significantly impact performance. For example, linked lists might be suitable for merge sort due to their efficient merging capabilities. On the other hand, arrays might offer better performance for quicksort due to efficient random access. Furthermore, the implications of stable sorting versus unstable sorting will be discussed, illustrating the importance of understanding data integrity needs. The choice between recursive and iterative implementation will also be considered, emphasizing the potential trade-offs between code elegance and performance. Finally, we'll touch upon advanced sorting techniques such as radix sort and counting sort, suitable for specific data types and constraints, showcasing how to adapt algorithm choices to specific problem requirements.
Graph Algorithms: Navigating Complex Networks
Graph algorithms are fundamental to solving problems involving relationships and connections. We'll explore breadth-first search (BFS) and depth-first search (DFS), two cornerstones of graph traversal. BFS systematically explores nodes layer by layer, ideal for finding shortest paths in unweighted graphs. This is particularly useful in network routing and social network analysis. Consider a scenario where we need to determine the shortest path between two nodes in a social network—BFS provides an efficient solution. Conversely, DFS explores nodes by going as deep as possible along each branch before backtracking. DFS is crucial in topological sorting, detecting cycles in directed graphs, and solving puzzles such as mazes. A compelling example of DFS in action is in detecting deadlocks in operating systems, where it helps identify cyclical dependencies among processes. Beyond basic traversal, we'll also explore minimum spanning trees (MST) and their application in network design. Prim's and Kruskal's algorithms will be compared, showcasing different approaches to finding the MST. A real-world case study includes their use in designing efficient telecommunication networks or power grids where minimizing cost is paramount. Finally, the complexities of shortest path algorithms like Dijkstra's algorithm and the Bellman-Ford algorithm will be analyzed. Dijkstra's algorithm efficiently finds the shortest paths from a single source node in a graph with non-negative edge weights. The significance of this lies in its use in GPS navigation systems and network optimization. The Bellman-Ford algorithm, while more computationally expensive, can handle graphs with negative edge weights, making it suitable for specific network scenarios. We'll unpack their underlying mechanisms and compare their efficiency in various graph types.
Dynamic Programming: Optimizing Recursive Solutions
Dynamic programming is a powerful technique for solving optimization problems by breaking them down into overlapping subproblems. Unlike brute-force approaches, dynamic programming avoids redundant calculations by storing and reusing solutions to previously encountered subproblems. This significantly improves efficiency, especially in problems with exponentially growing search spaces. A quintessential example of this efficiency is in the computation of Fibonacci numbers. A naive recursive approach leads to exponential time complexity, while a dynamic programming solution reduces this to linear time. Case studies illustrate its applications in sequence alignment (used in bioinformatics for analyzing DNA sequences) and finding the longest common subsequence of two strings. We'll explore various methods, including top-down (memoization) and bottom-up (tabulation) approaches, highlighting their trade-offs. A classic problem that perfectly illustrates the power of dynamic programming is the knapsack problem, where we need to select items with maximum value within a given weight constraint. This is used in resource allocation and optimization in logistics and project management. Moreover, this section will cover intricate concepts such as optimal substructure and overlapping subproblems, providing a solid understanding of when dynamic programming can be applied effectively. The complexities of solving various forms of the knapsack problem (0/1, fractional, unbounded) will be discussed. Furthermore, we'll showcase real-world applications of dynamic programming in areas like resource management and financial modeling. For example, portfolio optimization in finance often relies on dynamic programming techniques. The impact of choosing the right implementation (memoization versus tabulation) in terms of time and space complexity will be highlighted. Choosing between iterative and recursive approaches is a critical aspect of achieving optimal performance. We'll consider real-world examples where one approach demonstrably outperforms the other, showing how to make these decisions based on practical considerations.
Data Structures: Beyond Arrays and Lists
Effective data structures are crucial for efficient algorithm implementation. We’ll move beyond simple arrays and linked lists to explore trees, graphs, and hash tables. Binary search trees (BSTs) provide efficient searching, insertion, and deletion of data, with an average time complexity of O(log n). However, their performance degrades to O(n) in the worst case (e.g., a skewed tree). We'll discuss self-balancing trees like AVL trees and red-black trees which guarantee logarithmic time complexity even in the worst case. Real-world applications include their use in databases and operating systems for managing large amounts of hierarchical data. Furthermore, heaps, particularly min-heaps and max-heaps, provide efficient ways to retrieve the smallest or largest element in a collection. Their applications include priority queues and heapsort. Hash tables offer average-case O(1) time complexity for search, insertion, and deletion operations, making them highly efficient for implementing dictionaries and caches. We'll explore various collision resolution techniques, such as separate chaining and open addressing, and discuss their implications for performance. A critical case study examines the performance differences between different hash table implementations, highlighting the importance of choosing the right approach for a given application. Furthermore, the use of hash tables in databases for indexing, enabling faster data retrieval is a key point. This section will also explore advanced data structures such as tries and bloom filters, showing how they are applied to specific problems in areas such as text processing and network security. For example, Tries are incredibly efficient for autocompletion and spell-checking algorithms. Bloom filters are used in networking and databases for approximate membership testing, striking a balance between space efficiency and false positives.
Algorithm Analysis and Optimization
Understanding the efficiency of an algorithm is crucial for selecting the right solution for a problem. We'll cover big O notation and its use in analyzing the time and space complexity of algorithms. We'll examine how different algorithms scale with increasing input size. This is vital for choosing an algorithm that can handle large datasets efficiently. For example, an algorithm with O(n^2) time complexity may become impractical for very large inputs. Understanding these complexities allows developers to anticipate performance bottlenecks and choose more efficient options. Case studies will compare algorithms with different time and space complexities, providing a practical understanding of the trade-offs involved. Optimization techniques such as memoization and dynamic programming, already introduced, will be revisited within the context of algorithm analysis. We'll also delve into algorithm design paradigms like greedy algorithms and divide-and-conquer strategies. Greedy algorithms make locally optimal choices at each step, often leading to near-optimal overall solutions. Divide-and-conquer algorithms break a problem into smaller subproblems, solve them recursively, and combine the solutions. Real-world applications include their use in optimization problems like scheduling and resource allocation. Finally, we’ll touch upon advanced algorithm optimization techniques, such as using parallel processing and specialized hardware to accelerate computation. We will compare the efficiency gains achieved by utilizing parallel computing for specific algorithms. This exploration will demonstrate how advancements in hardware and software can significantly impact algorithm performance. The importance of profiling and benchmarking algorithms to identify and address performance bottlenecks will be addressed. A thorough understanding of the time and space complexity will help prioritize and guide optimization efforts. Efficient coding practices and appropriate data structure selection also play a crucial role in algorithm optimization.
Conclusion: Mastering the fundamentals of computer science, particularly algorithms and data structures, is paramount for success in this ever-evolving field. This deep dive has provided a solid foundation, moving beyond basic introductions to explore practical applications and innovative techniques. Through numerous examples and case studies, we’ve illuminated the core concepts and empowered you to confidently tackle real-world challenges. The knowledge gained here will serve as a cornerstone for more advanced studies and professional endeavors, equipping you to design, analyze, and optimize algorithms with efficiency and elegance. Continuous learning and practical application are vital to solidifying your understanding and adapting to the constantly evolving landscape of computer science. By embracing a hands-on approach and expanding your knowledge, you’ll not only master the techniques but also unlock the inherent creativity and problem-solving power that resides within the realm of algorithms.