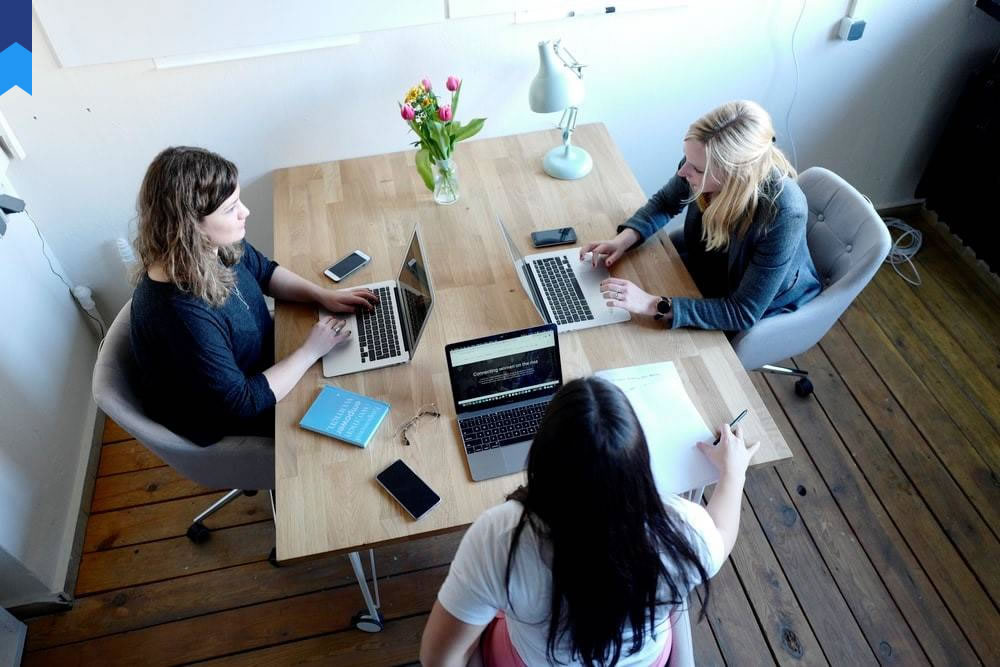
Arduino Mastery: A Deep Dive Into Advanced Techniques
Arduino programming: it's more than blinking LEDs and reading sensor data. This exploration delves into advanced techniques, pushing beyond the basics to unlock the true potential of this versatile platform. We'll tackle complex projects, unravel intricate code, and discover innovative applications that will transform your understanding of Arduino's capabilities.
Mastering Interrupts: Beyond the Basics
Interrupts are a cornerstone of efficient Arduino programming, allowing your microcontroller to respond to external events without halting the main program flow. Beyond simple button presses, interrupts enable sophisticated real-time control. Imagine a weather station autonomously logging data while simultaneously updating an LCD display and transmitting information via WiFi – interrupts make this possible. A crucial element is understanding interrupt priorities; properly managing them prevents conflicts and guarantees reliable operation. Consider a project integrating multiple sensors: a temperature sensor triggering an interrupt to activate a fan when a critical threshold is reached, while a light sensor simultaneously updates ambient light levels. Implementing interrupt routines requires careful consideration of timing and resource allocation, often involving preemptive multitasking strategies for optimal performance. For example, designing a robotic arm with multiple sensors requires precise control using interrupts. Each sensor (position, force, etc.) triggers an interrupt, resulting in a sophisticated, responsive system. This contrasts with a polled system that continuously checks each sensor, wasting processing power. Case study 1: An industrial automation system uses interrupts to handle various sensor inputs and control robotic actuators. Case study 2: An automotive system employs interrupts to monitor sensor data such as speed, engine temperature, and braking pressure, triggering crucial safety mechanisms instantly.
Effective use of interrupts dramatically improves responsiveness. A system using polling, constantly checking sensors, might miss critical events, whereas an interrupt-driven system guarantees immediate reaction. This is crucial in time-critical applications like robotics or industrial control. Proper implementation involves selecting the correct interrupt pin, configuring the interrupt mode (rising, falling, change), and writing efficient interrupt service routines (ISRs) that execute quickly without disrupting other processes. Mismanaged interrupts can lead to unexpected behavior or even system crashes. Techniques such as disabling interrupts temporarily for critical sections of code can be employed to ensure data integrity. Effective ISR design prioritizes speed and efficiency, minimizing the time spent within the ISR to avoid delaying other program execution. Optimizations such as using register variables instead of RAM variables can significantly boost performance. Experienced programmers use a combination of techniques to optimize their ISR's code, which improves the accuracy and responsiveness of the system, especially in time-critical applications.
Advanced Data Structures and Algorithms
While basic Arduino programming may use simple variables, tackling complex projects necessitates advanced data structures. Linked lists allow dynamic data management, crucial for projects with varying data sizes. Consider a logging system; a linked list efficiently handles a growing amount of sensor readings. Queues offer efficient data management, particularly for tasks like managing buffered sensor data or handling communication protocols. A robotic arm might use a queue to manage commands received from a computer, processing them sequentially. Trees, particularly binary search trees, are exceptionally valuable when dealing with large sorted datasets. Searching for a specific value becomes significantly faster than linear searches. For instance, a project categorizing sensor data could use a tree for fast data retrieval. The choice of data structure depends heavily on the specific application and its requirements in terms of speed, memory usage, and ease of implementation. Case study 1: A smart home system uses a tree structure to quickly locate and control various devices. Case study 2: A traffic monitoring system employs a queue to handle incoming data from multiple sensors, providing efficient management of traffic flow.
Algorithms are essential for efficient data processing. Sorting algorithms, like bubble sort or merge sort, arrange data for easier analysis. A project involving temperature readings over time benefits from sorted data for analysis. Search algorithms, such as binary search, rapidly locate specific values within sorted data. This is crucial for systems with large amounts of data. Advanced algorithms go beyond simple search and sort; pathfinding algorithms, such as A*, are essential for robotics, enabling robots to navigate efficiently. Understanding the complexities of these algorithms is crucial for optimizing performance and memory usage. Using an inappropriate algorithm can severely impact system performance. Optimizing data structures and algorithm choices requires considering factors such as memory constraints and processing power limitations of the microcontroller. This often involves a trade-off between speed and memory usage. The design process includes selecting appropriate algorithms and data structures considering the specific requirements of the project, including its real-time constraints and available memory. This involves assessing the scalability of the solution, ensuring its effectiveness even when the system size or data volume increases.
Wireless Communication Protocols
Arduino's versatility is amplified by its ability to communicate wirelessly. Beyond basic serial communication, exploring protocols like WiFi and Bluetooth expands possibilities. WiFi enables remote control and data transfer over longer distances. Imagine a smart irrigation system controlled via a smartphone application; WiFi is the key. Bluetooth offers lower power consumption and shorter-range communication, perfect for wearable devices or proximity sensors. A heart-rate monitor transmitting data to a smartphone would benefit from Bluetooth's low-power features. Choosing the right protocol depends on range, power requirements, and data transmission speed. Case study 1: An environmental monitoring system uses WiFi to transmit data from remote sensors to a central server. Case study 2: A smart lock uses Bluetooth to communicate with a smartphone for secure access.
Mastering wireless communication involves understanding the intricacies of each protocol. WiFi requires configuration of network parameters, including SSID and password. Bluetooth needs pairing and data formatting. Security considerations are crucial; protecting data from unauthorized access is paramount. Implementing encryption and authentication mechanisms are crucial to secure wireless communications. Efficient data handling is equally vital; minimizing data transmission reduces power consumption and bandwidth usage. Optimizing data structures and algorithms plays a role, ensuring efficient encoding and decoding processes. Using suitable libraries significantly simplifies the implementation of wireless communication. These libraries abstract away many low-level details, enabling developers to focus on higher-level functionalities. The choice of library depends on the specific protocol and the desired features. Thorough testing is crucial to ensure reliable communication. This involves checking data integrity, robustness against interference, and handling potential communication failures.
Real-Time Operating Systems (RTOS)
For complex applications exceeding the capabilities of bare-metal Arduino programming, Real-Time Operating Systems (RTOS) are invaluable. RTOSes manage multiple tasks concurrently, prioritizing tasks based on urgency. Imagine a robot that needs to simultaneously control motors, process sensor data, and communicate wirelessly; an RTOS makes this possible. Scheduling algorithms within the RTOS determine task execution order, optimizing system performance. Different RTOSes offer various features and capabilities, choosing the right one depends on the project's specific demands. Case study 1: A drone uses an RTOS to manage flight control, sensor data processing, and communication simultaneously. Case study 2: An industrial control system employs an RTOS to handle multiple tasks concurrently, ensuring real-time responsiveness.
Implementing an RTOS requires careful consideration of task prioritization, inter-task communication, and resource management. Tasks must be carefully defined, with appropriate priorities assigned to ensure critical functions are always executed promptly. Inter-task communication mechanisms, like semaphores or mutexes, prevent race conditions and data corruption. Memory management is also critical; efficient allocation and deallocation of resources prevent memory leaks and fragmentation. Choosing an appropriate RTOS involves evaluating the system's real-time requirements, available memory resources, and processing power. The RTOS should be selected to meet the project’s specific needs in terms of timing constraints, response times, and memory footprint. Implementing an RTOS adds complexity to the project, requiring familiarity with the RTOS’s APIs and concepts. Understanding concepts like task scheduling, context switching, and interrupt handling is crucial for successful implementation. A well-designed RTOS-based system can significantly improve the performance, reliability, and maintainability of complex Arduino projects.
Advanced Debugging and Testing Techniques
Debugging and testing are critical for successful Arduino programming. Beyond basic serial monitoring, advanced techniques are necessary for complex projects. Logic analyzers provide detailed insights into digital signals, identifying timing issues and glitches. Oscilloscope visualizes analog signals, revealing problems in sensor readings or signal processing. Systematic testing involves creating test cases that cover various scenarios and inputs, verifying the system's behavior under different conditions. Using appropriate test frameworks can streamline this process, allowing automated testing. Case study 1: A team uses a logic analyzer to debug timing issues in a high-speed data acquisition system. Case study 2: A company utilizes a rigorous testing procedure to ensure the reliability of their Arduino-based product.
Effective debugging practices often involve a combination of techniques. Using print statements for simple debugging, utilizing a debugger for deeper analysis, and employing a combination of hardware and software tools provide a complete debugging solution. This includes using logic analyzers to capture the state of multiple signals simultaneously, which is crucial in multi-threaded and real-time applications. The selection of testing and debugging tools depends on factors such as the complexity of the project, available resources, and the nature of the bugs being investigated. Systematic testing strategies, involving creating detailed test plans, test cases, and documenting test results are critical for ensuring high-quality Arduino projects. This approach significantly reduces the probability of unexpected failures, increasing system reliability and reducing maintenance costs. Understanding and applying efficient debugging and testing methodologies helps developers create reliable, maintainable, and high-quality Arduino-based systems. This process improves the overall effectiveness of development and reduces the likelihood of bugs.
Conclusion
Mastering Arduino programming goes far beyond introductory tutorials. This deep dive into advanced techniques empowers developers to tackle complex and innovative projects. From mastering interrupts and leveraging advanced data structures to harnessing wireless communication protocols and utilizing real-time operating systems, these techniques unlock the true potential of Arduino. By integrating effective debugging strategies, developers can ensure high-quality, reliable systems. The journey toward Arduino mastery is a continuous process of learning and experimentation. Embrace the challenges, explore the possibilities, and unlock the incredible potential within this versatile platform.