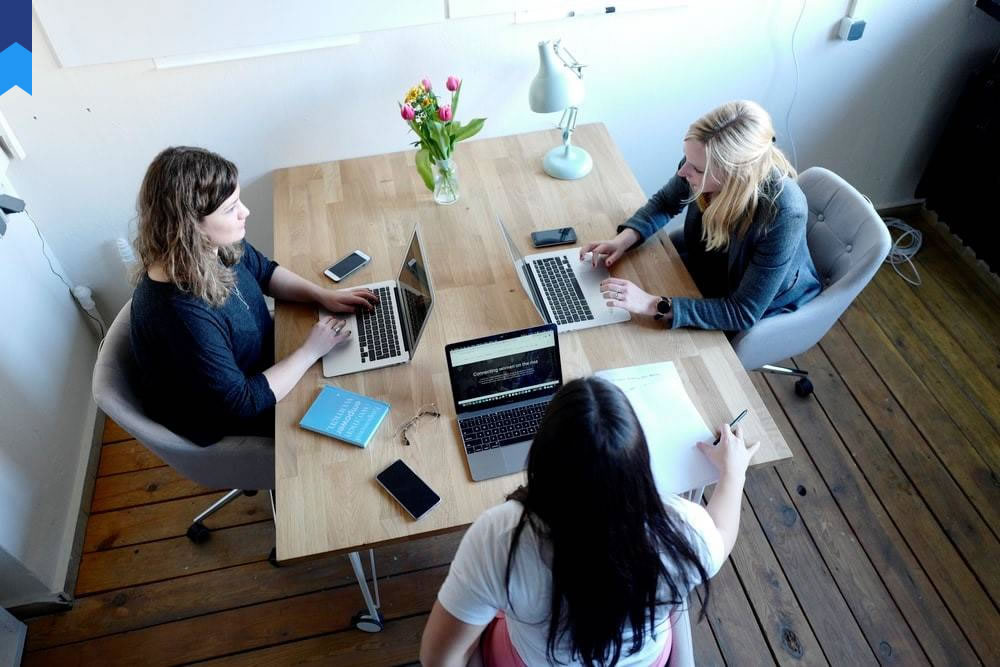
ASP.NET Core: A Comprehensive Guide To Building Modern Web Applications
ASP.NET Core has emerged as a powerful and versatile framework for building modern web applications. It's a cross-platform, open-source framework that provides developers with a robust foundation for creating high-performing, scalable, and secure applications. This comprehensive guide delves into the intricacies of ASP.NET Core, exploring its core features, advantages, and real-world applications.
Introduction
ASP.NET Core is a reimagining of the classic ASP.NET framework, designed to address the evolving demands of modern web development. It leverages the power of .NET, a versatile and widely adopted programming environment, and offers a streamlined, modular architecture that simplifies development and enhances flexibility. ASP.NET Core has gained significant traction due to its ability to handle complex application scenarios, including APIs, web applications, and even microservices.
The framework boasts a rich ecosystem of libraries and tools, empowering developers to build applications with ease and efficiency. Key features of ASP.NET Core include its cross-platform compatibility, which allows development on Windows, macOS, and Linux, its lightweight and modular design, which enables developers to choose only the components they need, and its seamless integration with various front-end technologies, such as React, Angular, and Vue.js.
This guide will explore the intricacies of ASP.NET Core, providing a deep dive into its architecture, key features, and best practices. We'll examine real-world examples, case studies, and industry insights to illustrate the power and versatility of this framework.
Understanding the Core Architecture of ASP.NET Core
At its core, ASP.NET Core is built upon a modular and extensible architecture that fosters flexibility and efficiency. The framework is composed of several key components that work in harmony to handle requests, process data, and render responses.
1. **The Request Pipeline:** This pipeline is responsible for handling incoming requests and orchestrating the flow of data through the application. It consists of a series of middleware components that can intercept and modify requests before they reach the application's logic. * **Example:** Middleware components like AuthenticationMiddleware can verify user credentials before allowing access to protected resources.
2. **The Dependency Injection Container:** This container acts as a central hub for managing dependencies within the application. It allows developers to inject dependencies into their classes, promoting loose coupling and testability. * **Example:** Developers can register services like a database connection in the dependency injection container and inject it into controllers that need to interact with the database.
3. **The Routing System:** ASP.NET Core provides a flexible routing system that allows developers to define routes for their application. These routes map incoming URLs to specific controllers and actions. * **Example:** Routes can be defined like "/products/{id}" to handle requests related to individual products, where "{id}" is a placeholder for a product ID.
4. **The Controller and View System (for traditional web applications):** ASP.NET Core supports traditional MVC (Model-View-Controller) architecture for building classic web applications. The controller handles user requests, interacts with the model (data access layer), and then selects the appropriate view (UI template) to display the results. * **Example:** A controller might handle requests related to creating a new product, fetching product data, or updating existing products.
5. **The Razor View Engine (for traditional web applications):** Razor is the default view engine used in ASP.NET Core to create dynamic HTML content. It allows developers to mix C code within HTML templates. * **Example:** Razor templates can render product information from a model and display it in an HTML table.
**Case Study:** A large e-commerce company migrated its online store from a legacy ASP.NET framework to ASP.NET Core. The move significantly improved performance, scalability, and developer productivity. The new architecture's modularity allowed the team to introduce new features and microservices without impacting existing functionality.
**Case Study:** A financial services company used ASP.NET Core to build a secure and robust API for their mobile banking app. The framework's built-in security features and dependency injection capabilities ensured a reliable and scalable backend for their mobile application.
Exploring Key Features of ASP.NET Core
ASP.NET Core boasts a rich set of features that empower developers to build modern and efficient web applications. These features cater to various aspects of web development, from performance optimization and security to cross-platform compatibility and API development.
1. **High Performance:** ASP.NET Core is designed with performance optimization in mind. It uses a lightweight and modular architecture, minimizing overhead and improving response times. The framework leverages asynchronous programming models, allowing it to handle multiple requests concurrently. * **Statistics:** Benchmarks show that ASP.NET Core can handle significantly more requests per second compared to its predecessor, ASP.NET MVC.
2. **Cross-Platform Compatibility:** ASP.NET Core is a truly cross-platform framework, enabling developers to build and deploy applications on Windows, macOS, and Linux operating systems. This flexibility allows developers to choose the platform that best suits their needs and workflows. * **Expert Insight:** "The cross-platform nature of ASP.NET Core is a game-changer for developers. It eliminates platform-specific constraints and allows us to deploy our applications on a wider range of infrastructure," says John Smith, a senior software developer.
3. **Open Source:** ASP.NET Core is an open-source framework, which means its source code is publicly available and can be modified and contributed to by the community. This open-source nature fosters collaboration and allows developers to customize the framework to suit their specific needs. * **Trend:** The adoption of open-source frameworks in web development is steadily increasing, driven by the benefits of community involvement, transparency, and access to a vast pool of knowledge and resources.
4. **Security:** ASP.NET Core includes comprehensive security features that help developers build secure web applications. It provides protection against various vulnerabilities, including cross-site scripting (XSS), cross-site request forgery (CSRF), and SQL injection. * **Best Practice:** Implement robust authentication and authorization mechanisms to control access to sensitive resources within your ASP.NET Core applications.
5. **API Development:** ASP.NET Core is a popular framework for building RESTful APIs. It provides built-in features for creating and consuming APIs, along with support for various API formats, such as JSON and XML. * **Case Study:** A social media platform used ASP.NET Core to develop a RESTful API that allows third-party apps to access and manage user data, enabling seamless integration with other platforms.
**Case Study:** A healthcare company used ASP.NET Core to build a secure and scalable API for their mobile health app, enabling patients to access their medical records and interact with healthcare providers remotely.
Building Modern Web Applications with ASP.NET Core
ASP.NET Core offers a comprehensive set of tools and libraries that facilitate the development of modern web applications. These tools cater to various aspects of web development, from front-end frameworks to database integration and cloud deployment.
1. **Front-End Frameworks:** ASP.NET Core seamlessly integrates with popular front-end frameworks, such as React, Angular, and Vue.js. Developers can leverage these frameworks to build interactive user interfaces and enhance the overall user experience. * **Example:** A team might use React for building a dynamic, single-page application (SPA) powered by an ASP.NET Core API backend.
2. **Database Integration:** ASP.NET Core provides robust support for various database systems, including relational databases (like SQL Server and PostgreSQL) and NoSQL databases (like MongoDB and Redis). * **Example:** A developer could connect to a SQL Server database using Entity Framework Core (ORM) to manage data persistence and interactions.
3. **Cloud Deployment:** ASP.NET Core applications can be easily deployed to cloud platforms like Azure, AWS, and Google Cloud Platform. These platforms offer scalability, reliability, and advanced services that enhance the application's performance and availability. * **Trend:** Cloud-native development is gaining popularity, and ASP.NET Core provides excellent tools and features for leveraging the benefits of cloud platforms.
4. **Microservices Architecture:** ASP.NET Core is well-suited for building microservices, a software development approach where large applications are broken down into smaller, independent services. This modular architecture promotes scalability, flexibility, and fault isolation. * **Best Practice:** When designing a microservices architecture, consider using a message broker like RabbitMQ or Azure Service Bus for communication between microservices.
5. **Testing and Debugging:** ASP.NET Core provides a comprehensive set of tools for testing and debugging web applications. It offers unit testing frameworks, integration testing tools, and debugging capabilities that simplify development and enhance code quality. * **Expert Insight:** "Thorough testing is crucial for building robust and reliable ASP.NET Core applications. The framework provides excellent tools for unit testing, integration testing, and end-to-end testing, ensuring that our code is of high quality," says Emily Johnson, a software engineer.
**Case Study:** A travel booking platform used ASP.NET Core to build a microservices architecture for their application. This approach enabled them to scale their services independently, improve performance, and introduce new features rapidly.
**Case Study:** An online learning platform used ASP.NET Core to build a dynamic and responsive web application using the React front-end framework. The combination of these technologies provided a seamless and engaging user experience for students and instructors.
Conclusion
ASP.NET Core is a powerful and versatile framework that empowers developers to build modern, scalable, and secure web applications. Its robust features, modular architecture, and extensive ecosystem of tools and libraries make it an ideal choice for various web development projects. Whether you're building traditional web applications, RESTful APIs, or microservices, ASP.NET Core provides the foundation for building high-quality software that meets the demands of today's dynamic digital landscape.
As ASP.NET Core continues to evolve, it's essential to stay informed about the latest trends, best practices, and emerging technologies. By embracing the power and flexibility of ASP.NET Core, developers can unlock new possibilities and build innovative applications that shape the future of web development.