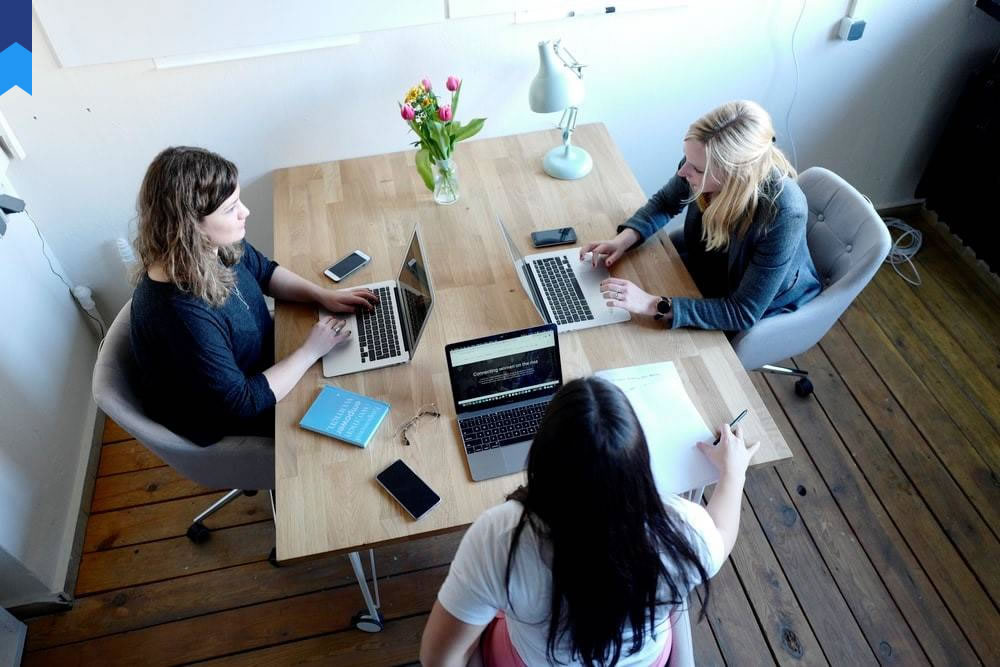
Hello World!
Introduction
ASP.NET Core MVC is a powerful and versatile framework for building modern web applications. It provides a robust foundation for creating scalable, maintainable, and secure applications. In this comprehensive guide, we will delve into the core concepts of ASP.NET Core MVC, exploring its key features, benefits, and best practices. From understanding the MVC pattern to mastering advanced techniques, this article will equip you with the knowledge needed to build exceptional web applications.
Understanding the Model-View-Controller (MVC) Pattern
ASP.NET Core MVC follows the Model-View-Controller (MVC) architectural pattern, a widely accepted approach to structuring web applications. The MVC pattern separates application logic into three distinct components:
- Model: Represents the data and business logic of the application. It handles data access, validation, and other business-related operations. For instance, a model could represent a product in an e-commerce application, managing its attributes, pricing, and inventory.
- View: Responsible for displaying the user interface (UI) of the application. It receives data from the controller and renders it into an HTML representation, which the browser interprets and displays. Views can be implemented using different templating engines, such as Razor, to create dynamic and interactive UIs.
- Controller: Acts as an intermediary between the model and the view. It receives user requests, interacts with the model to retrieve or manipulate data, and determines which view to render. Controllers handle user input, process data, and orchestrate the interaction between the model and the view.
By separating concerns, the MVC pattern promotes code reusability, testability, and maintainability. It allows developers to focus on specific areas of the application without affecting other parts, leading to a cleaner and more organized codebase.
Case Study: A popular e-commerce platform like Amazon uses the MVC pattern to handle its complex operations. The model manages product data, orders, and customer information. The view displays product listings, checkout pages, and user accounts. The controller manages user interactions, handles order processing, and updates data in the model.
Case Study: A social media platform like Twitter also leverages the MVC pattern. The model stores user profiles, tweets, and other data. The view displays timelines, user profiles, and tweet details. The controller handles user actions such as tweeting, following users, and interacting with content.
Setting Up an ASP.NET Core MVC Project
Creating an ASP.NET Core MVC project is straightforward. You can use Visual Studio, a popular Integrated Development Environment (IDE) for .NET development, or the .NET CLI (Command Line Interface). Here's a step-by-step guide:
- Visual Studio: Open Visual Studio and create a new project. Select "ASP.NET Core Web Application" and choose the "MVC" template. Configure the project settings, such as the name and location, and click "Create." Visual Studio will automatically set up the necessary files and dependencies for an ASP.NET Core MVC application.
- .NET CLI: Open a terminal or command prompt and navigate to the desired project directory. Run the following command: `dotnet new mvc -o MyMvcApp` This command creates a new ASP.NET Core MVC project named "MyMvcApp" in the current directory.
Once the project is created, you can run the application to see a default MVC application running in the browser. The default project includes basic routing, controllers, and views to demonstrate the fundamentals of ASP.NET Core MVC. You can then modify the project to add your custom features and functionalities.
Key Components of ASP.NET Core MVC
ASP.NET Core MVC offers a comprehensive set of features that facilitate the development of modern web applications. Some of the key components include:
- Routing: ASP.NET Core MVC uses a flexible routing system to map incoming requests to specific controllers and actions. You can define routes using attributes or conventions, allowing you to create clean and maintainable URLs.
- Controllers: Controllers handle user requests and interact with the model. They determine the appropriate actions to execute and select the view to render. Controllers use action methods to process requests and return results.
- Views: Views are responsible for rendering the UI of the application. They use the Razor syntax to create dynamic HTML content, including data from the model. Views can be organized into folders and namespaces for better structure.
- Models: Models represent the data and business logic of the application. They contain properties and methods that define the structure and behavior of the data. Models can interact with databases, perform validation, and implement business rules.
- Middleware: Middleware is a powerful concept in ASP.NET Core that allows you to intercept and modify HTTP requests and responses. It provides a mechanism to add functionality to your application, such as authentication, authorization, logging, and error handling.
- Dependency Injection: Dependency injection (DI) is a design pattern that promotes loose coupling between components. ASP.NET Core provides built-in support for DI, allowing you to inject dependencies into controllers and other services, making your code more modular and testable.
Case Study: A popular online banking platform might use ASP.NET Core MVC to handle user authentication, account management, and transaction processing. The model would represent customer accounts, transactions, and other data. Controllers would handle user logins, account transfers, and payment processing. Views would display account balances, transaction history, and other account-related information.
Case Study: A news website could leverage ASP.NET Core MVC to manage articles, categories, and user comments. The model would store article content, category details, and user information. Controllers would handle article creation, editing, and publishing. Views would display article listings, detailed articles, and comment sections.
Building a Simple ASP.NET Core MVC Application
Let's create a simple ASP.NET Core MVC application to illustrate the basic concepts. We'll build a basic "Hello World" application that displays a greeting message. Follow these steps:
- Create a new project: Start a new ASP.NET Core MVC project as described in the previous section. You can use Visual Studio or the .NET CLI to create the project.
- Create a controller: In the "Controllers" folder, add a new controller class named "HomeController." This controller will handle requests related to the home page.
- Add an action method: Inside the "HomeController," add an action method named "Index." This method will be executed when the user visits the home page.
- Return a view: In the "Index" action method, return a view named "Index." This view will display the "Hello World" message.
- Create a view: In the "Views/Home" folder, add a view file named "Index.cshtml." This view will contain the HTML code to display the greeting message. In the "Index.cshtml" file, write the following code:
```
Run the application: Press F5 to run the application. You should see the "Hello World!" message displayed in the browser. This demonstrates how ASP.NET Core MVC handles requests, routes them to the appropriate controller and action, and renders the corresponding view.
Conclusion
ASP.NET Core MVC provides a powerful and flexible framework for building modern web applications. It offers a robust foundation for creating scalable, maintainable, and secure applications. By understanding the MVC pattern, key components, and best practices, developers can leverage the capabilities of ASP.NET Core MVC to create exceptional web experiences.