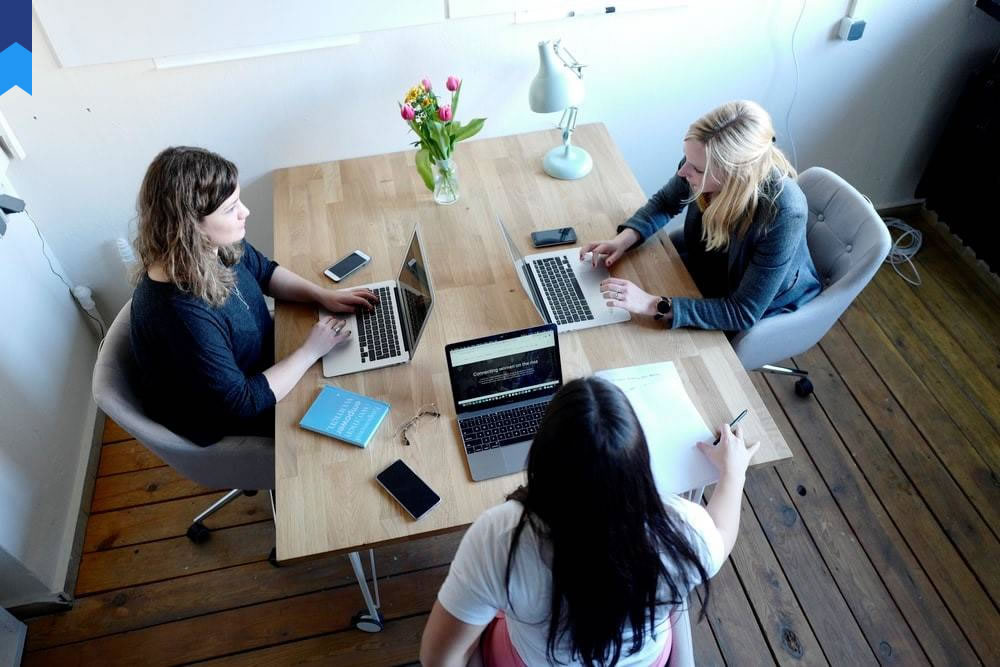
Beyond Traditional Android Development: A Modular Approach
Android development has traditionally relied on monolithic application structures, leading to challenges in maintainability, scalability, and testability. This article explores a new approach: modular Android development, offering a path towards more robust and efficient applications.
Understanding Modular Android Development
Modular Android development involves breaking down a large application into smaller, independent modules. Each module focuses on a specific feature or functionality, promoting better organization, reusability, and independent development cycles. This approach contrasts sharply with the traditional, monolithic architecture where all components reside within a single application module. This fragmentation not only improves code organization but also allows for parallel development across different teams, dramatically shortening development timelines. Consider the case of a large e-commerce application. A modular design could separate features like user authentication, product catalog, shopping cart, and payment processing into distinct modules. This segregation allows for focused development and easier debugging, as issues in one module won't necessarily affect others. Another example is a news application with modules for news feeds, user profiles, and notifications. These modules can be developed and tested independently, reducing the risk of cascading failures and simplifying maintenance.
Adopting a modular approach offers several key advantages. First, it enhances maintainability. Isolating functionalities into separate modules simplifies codebase management, making it easier to identify and fix bugs. Updates and modifications become less disruptive as changes within a module are less likely to impact other parts of the application. Second, modularity significantly improves testability. Smaller, isolated modules are much easier to test thoroughly than a large, complex codebase. Unit testing, integration testing, and other testing strategies become more efficient and comprehensive. For instance, the authentication module can be tested rigorously in isolation before integrating it with other modules. Third, it fosters code reusability. Modules developed for one application can potentially be reused in other projects, reducing development time and effort. This is especially beneficial for companies with multiple Android apps sharing similar features.
The transition to a modular architecture involves careful planning and design. A well-defined modular structure requires meticulous analysis of the application's functionality and dependencies. Identifying clear boundaries between modules is essential to avoid tight coupling and ensure independence. Effective communication and collaboration within the development team are crucial for successful implementation. Case studies show that modular design helps reduce overall development time by enabling parallel development streams. For instance, a study by Google demonstrated a significant improvement in developer productivity when teams used a modular architecture. Furthermore, the ability to release updates to individual modules independently means that users don't have to wait for an entire application update to experience new features or bug fixes, leading to enhanced user satisfaction and engagement.
Migrating an existing monolithic application to a modular structure can be a complex undertaking. A phased approach, starting with the most independent functionalities, is generally recommended. Refactoring existing code to conform to the new modular structure requires careful planning and execution. It's important to balance the benefits of modularity with the cost of migration. This careful transition needs a well-defined strategy to minimize disruption to ongoing development and to ensure that the migration process itself doesn't introduce new problems or instability. Successful migration hinges on a thorough understanding of the existing codebase, and diligent testing at each phase of the transition.
Implementing Modular Design in Android
Android provides built-in support for modularity through its module system, allowing developers to structure their applications using multiple modules. Each module can contain its own source code, resources, and manifest file. This decoupling allows for greater flexibility and organization. The use of dependency injection frameworks further enhances modularity by managing dependencies between modules effectively. Dagger or Hilt are popular choices, allowing for cleaner code and better testability. The benefits are substantial, improving code maintainability, testability and overall application structure. A well-structured modular application becomes significantly easier to manage and maintain over time. Imagine a complex app with features like maps, social media integration, and in-app purchases; each of these could exist as its own module, independently versioned and deployed.
Proper module dependencies are crucial in modular design. Circular dependencies should be strictly avoided to prevent compilation and runtime issues. Clearly defining interfaces between modules ensures loose coupling and promotes independent development. Choosing appropriate module boundaries is a key decision impacting development efficiency and maintainability. An improper modular design can lead to unexpected complexities and challenges in later development phases. For instance, if modules are too tightly coupled, changes in one module can unintentionally affect other modules, slowing down development and increasing the likelihood of errors. Well-defined interfaces and careful consideration of dependencies ensure efficient, independent development.
Case studies show the effectiveness of this approach. Many successful apps have adopted a modular design, resulting in improved team productivity and reduced development time. For example, several large-scale applications from major tech companies have adopted this design pattern, demonstrating its effectiveness in managing complexity and maintaining high code quality. Moreover, the use of modularity enables more efficient testing, as individual modules can be tested independently, leading to better quality assurance and fewer bugs. The ability to update individual modules independently, without requiring an update to the entire application, allows for quicker response to bug fixes and feature additions, directly contributing to user satisfaction.
This modern approach to Android development prioritizes organization and scalability. Effective modularization is not just about splitting code into smaller chunks; it's about architecting the application in a way that allows for independent development, testing, and deployment of features. This includes careful consideration of module dependencies, versioning, and communication between modules. Clear documentation of interfaces and responsibilities ensures that different teams can work on different parts of the application concurrently without interfering with each other's work. The adoption of well-established design patterns, such as the Model-View-ViewModel (MVVM) architecture, complements the modular design and further enhances the application's maintainability and testability. The result is a more robust and easier-to-maintain application architecture.
Testing Strategies in Modular Android Development
Testing in a modular Android application differs significantly from testing a monolithic application. The modular nature allows for more focused and efficient testing strategies. Unit testing individual modules becomes easier and more effective. With isolated functionalities, developers can test individual units of code without the interference of other components. This isolation enhances the accuracy of testing and reduces the risk of false positives or negatives. This targeted approach enhances the efficiency of the testing phase, which is particularly crucial in larger applications. A comprehensive suite of unit tests can quickly identify and pinpoint problems in individual modules before integration, significantly reducing debugging time and cost.
Integration testing plays a critical role in ensuring the seamless interaction between different modules. Testing the interfaces between modules verifies their proper communication and data exchange. This level of testing focuses on the interaction between various components rather than the internal workings of individual modules. Comprehensive integration tests verify the overall application functionality and uncover potential integration issues early on. Robust integration tests provide confidence in the smooth functioning of the application as a whole.
Case studies reveal the substantial benefits of these testing approaches. Companies employing modular architecture and rigorous testing have reported significant reductions in bug detection and resolution times, leading to improved product quality and reduced costs. The ability to run automated tests for individual modules speeds up the testing process and allows for more comprehensive testing, enhancing the overall quality and reliability of the software. Moreover, automated tests can be integrated into the continuous integration/continuous delivery (CI/CD) pipeline to automate the testing process and ensure consistent quality across different builds.
Employing advanced testing tools and techniques further enhances the testing process. Code coverage tools help developers identify untested code, ensuring comprehensive testing. Mocking and stubbing techniques help isolate units during testing, enabling more precise and accurate testing. Adopting these tools and techniques helps create a more reliable and robust software development process. The use of proper testing frameworks, such as JUnit and Mockito, further enhances the quality of the testing procedure. Integration of testing practices into the development lifecycle ensures that testing is an integral part of the development workflow and not an afterthought. This fosters a culture of quality and leads to improved application robustness and reliability.
Advanced Modularization Techniques
Beyond basic modularization, advanced techniques can significantly enhance scalability and maintainability. Feature flags allow developers to enable or disable features at runtime without deploying a new version of the application. This provides exceptional flexibility for A/B testing and managing feature rollouts. This capability significantly enhances the ability to respond to changing market demands or user feedback quickly. This approach allows for a more dynamic application environment, making it adaptable to varying circumstances.
Component-based architecture takes modularity to the next level, treating each module as a self-contained component that can be developed, tested, and deployed independently. This level of decoupling allows for easier reuse and integration with other applications or systems. Component-based architecture facilitates greater flexibility and scalability, especially in larger projects. This high level of modularity fosters collaboration and independent development while maintaining a strong foundation of interoperability.
Case studies showcasing successful implementations of these advanced techniques demonstrate the significant improvements in development speed, maintainability, and scalability. Companies adopting these practices often report enhanced developer productivity and a decrease in time-to-market. The ability to iterate on features quickly and release updates frequently gives a significant competitive advantage. Furthermore, the high degree of modularity contributes to long-term maintainability, even as the application grows and evolves over time.
The careful consideration of module interfaces and dependencies is crucial in advanced modularization. Overly complex interdependencies between modules can negate the benefits of modularity. The use of design patterns and architectural best practices, such as dependency injection and inversion of control, ensures loose coupling and enhances maintainability. Regular code reviews and testing help ensure that these principles are adhered to and that the modular architecture remains effective. This helps to maintain a clean and well-structured codebase, even as the application expands.
Future Trends in Android Modular Development
The future of Android modular development lies in continuous improvement and refinement of existing techniques. Improved tooling and frameworks will simplify the development and maintenance of modular applications. The emergence of new architectural patterns and design principles will further enhance the effectiveness of modularity. This ongoing evolution ensures that Android development keeps pace with the ever-changing technological landscape.
Increased emphasis on microservices architecture in Android development will lead to even greater decoupling and scalability. This approach treats each module as a separate microservice, enabling independent scaling and deployment. Microservices further enhance the flexibility and scalability of the application, allowing for efficient scaling of individual components based on their specific needs. This results in a highly responsive and adaptable application, capable of handling varying loads and user demands.
Case studies on cutting-edge modular applications highlight the advantages of these approaches. These examples demonstrate how adopting advanced modularization techniques can significantly improve application performance, scalability, and maintainability. Furthermore, continuous integration and continuous deployment (CI/CD) practices will become increasingly crucial in managing complex modular applications, streamlining the development and deployment process and ensuring rapid delivery of features and bug fixes. This integration streamlines the release cycle and enables faster responses to user feedback and market changes.
The adoption of AI and machine learning in Android development will provide new opportunities for enhancing modularity. AI-powered tools can assist developers in identifying optimal module boundaries, automating testing processes, and optimizing application performance. This integration of AI technologies into the development pipeline enhances efficiency and improves application quality. Moreover, the use of AI-driven code analysis can help identify potential problems and vulnerabilities within the modular architecture, leading to more robust and reliable applications.
Conclusion
Modular Android development presents a compelling alternative to traditional monolithic approaches. By embracing modularity, developers can create more maintainable, scalable, and testable applications. The benefits extend to improved team collaboration, faster development cycles, and enhanced user experience. While transitioning to a modular architecture may require careful planning and execution, the long-term advantages far outweigh the initial investment. The future of Android development is undeniably heading towards greater modularity, with advanced techniques and AI-powered tools further enhancing its potential. The benefits of modularity—enhanced maintainability, improved testability, and increased scalability—will continue to drive its adoption, resulting in more robust, efficient, and user-friendly applications.