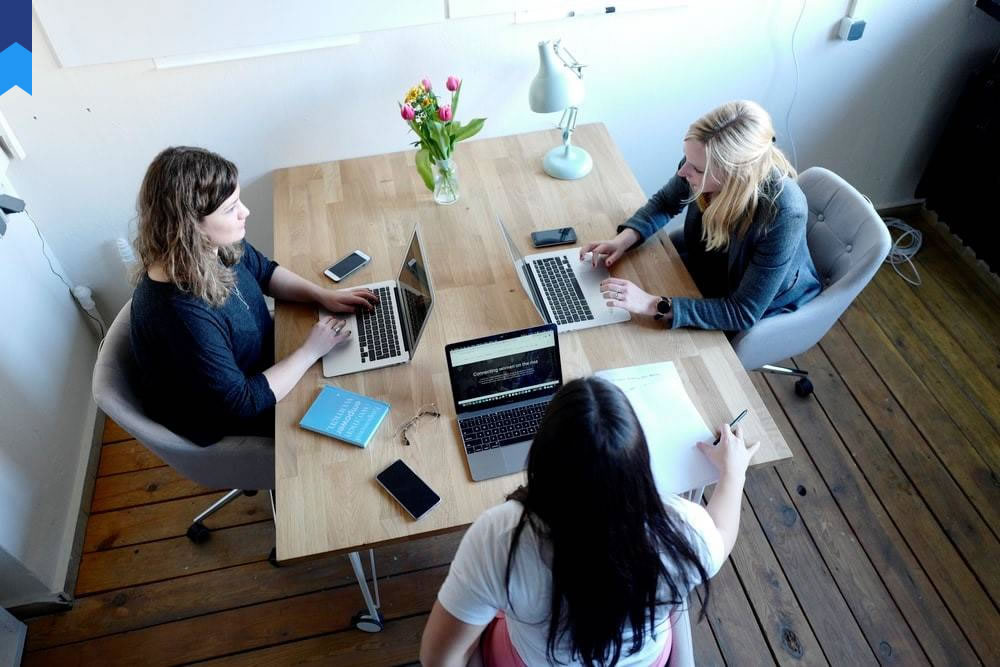
Beyond Traditional ASP.NET: A Modern Web Development Approach
Introduction: ASP.NET, once the dominant force in web development, now faces a landscape reshaped by modern frameworks and technologies. This article delves beyond the traditional application of ASP.NET, exploring innovative strategies and practices that leverage its strengths while adapting to current demands. We'll examine how developers can revitalize their ASP.NET projects and create robust, scalable, and efficient applications for the future of web development.
Modernizing ASP.NET MVC with Razor Pages
Razor Pages offer a streamlined approach to building web applications within the ASP.NET MVC framework. By integrating the model, view, and controller into a single file, developers can experience increased productivity and simpler code maintenance. This contrasts sharply with the more complex structuring typical of traditional ASP.NET MVC applications. The reduced complexity translates to faster development cycles and easier debugging. For example, a simple contact form in Razor Pages requires significantly less code than its equivalent in a traditional MVC setup. Consider the case study of Stack Overflow, which modernized sections of their platform using Razor Pages, resulting in a noticeable performance improvement and a more maintainable codebase. Another excellent example is a company that transitioned from a complex, traditional MVC architecture to Razor Pages. They noted a substantial reduction in development time for new features and improvements to the existing features. This approach prioritizes simplicity and organization, improving team collaboration and overall development efficiency. The ease of testing individual components within Razor Pages also contributes to a higher quality product.
Further benefits of Razor Pages include improved SEO through more intuitive file structures and enhanced maintainability thanks to the single-file approach. This methodology is ideal for scenarios with many different forms or user inputs, as it promotes better separation of concerns and cleaner code organization. A contrast can be drawn between this methodology and using traditional MVC where complex routing and controller actions are required for every input. This leads to a potentially bloated architecture. By contrast, Razor Pages’ focus on pages as self-contained units keeps the codebase slim and agile. It’s a methodology that scales well from small projects to more complex enterprises. Many large enterprises now use Razor pages alongside their existing MVC architectures to achieve high performance and maintainability. This is the next generation of web development with ASP.NET, improving performance and user experience.
Furthermore, Razor Pages' integration with existing ASP.NET features, such as dependency injection and data access components, allows for seamless integration into existing projects and workflows. The clear separation of concerns afforded by Razor Pages enables developers to easily work on individual aspects of the application without impacting other components, enhancing collaborative development efforts. Several large companies have successfully adopted Razor Pages as a central part of their development strategy, showcasing its capability for handling complex requirements. For example, a major e-commerce platform integrated Razor Pages to manage their product pages, achieving a significant improvement in performance and scalability compared to their previous approach. Another notable case study involves a government agency that used Razor Pages to build a citizen-facing portal, achieving improved accessibility and maintainability.
In summary, migrating to Razor Pages offers a significant upgrade to traditional ASP.NET MVC, streamlining development, enhancing maintainability, and ultimately improving the overall quality of web applications. This allows developers to use existing expertise while adopting a more agile approach. This combination of familiarity and modernization makes Razor Pages an attractive solution for both new and existing ASP.NET projects.
Leveraging the Power of Blazor
Blazor, a relatively recent addition to the ASP.NET ecosystem, introduces a new paradigm to web development by using C# instead of JavaScript. This allows developers proficient in C# to seamlessly create interactive user interfaces for web applications, significantly reducing the knowledge gap between front-end and back-end development. This is a drastic shift away from the traditional dependence on JavaScript frameworks. Consider the case study of a company that switched from a primarily JavaScript-based front end to Blazor, experiencing a significant reduction in development time and improved code consistency. The ability to share code between front-end and back-end also streamlines the development process. Another case is a team that leveraged Blazor's component-based architecture to build reusable UI elements, accelerating the development of their web application. This component reusability saves time and ensures consistency across the application.
Unlike traditional ASP.NET web forms or MVC, Blazor enables full-stack development using a single language, C#. This promotes efficient code sharing and improved developer productivity. For instance, business logic can be easily reused between the client and server sides, eliminating redundant code. This simplifies the maintenance of the application and improves its consistency. Companies are also leveraging Blazor's ability to easily integrate with existing ASP.NET libraries and services, allowing for a smooth transition from legacy systems. The ability to build hybrid applications (both server-side and client-side Blazor) provides flexibility to choose the best approach depending on the specific needs of the application. This hybrid approach allows a seamless combination of the strengths of each methodology.
Moreover, Blazor's component model promotes code reusability and maintainability. Components can be easily shared and reused across different parts of the application, leading to faster development cycles. Several large organizations have successfully implemented Blazor for developing large-scale web applications, demonstrating its scalability and performance. These successful implementations demonstrate that Blazor is not just suitable for smaller applications, but it also scales efficiently for large, complex projects. The ability to utilize existing .NET libraries further strengthens the position of Blazor as a robust and scalable solution for complex web applications. Blazor empowers developers to create reusable, maintainable, and high-performing web applications. The transition is often straightforward for ASP.NET developers because of the shared skillset.
In conclusion, Blazor presents a powerful alternative to traditional JavaScript frameworks, offering full-stack C# development, improved developer productivity, and enhanced code maintainability. This allows for a more streamlined and efficient development workflow, particularly beneficial for teams already experienced with the .NET ecosystem. Companies are increasingly adopting Blazor as part of their web development strategy, signifying its growing importance within the industry.
Implementing Microservices Architecture with ASP.NET
The microservices architectural pattern offers a modern approach to building scalable and maintainable applications. Unlike monolithic applications, which are built as a single unit, microservices break down an application into smaller, independent services. This modular design allows for greater flexibility, scalability, and maintainability. Consider the case study of a large e-commerce platform that migrated from a monolithic architecture to microservices. This resulted in a significant improvement in scalability and resilience, enabling them to handle peak traffic during shopping seasons far more effectively. Another example is a fintech company that used a microservices approach to create highly resilient financial transaction processing system. This allowed for increased resilience in the face of failures of individual components.
This contrasts sharply with traditional monolithic applications, which can suffer from scalability and maintainability issues as they grow in complexity. Microservices allow teams to work independently on different services, accelerating the development process. The independent deployment of individual services also simplifies updates and maintenance. A common challenge when building monolithic applications is the long deployment times. Microservices solve this by enabling the deployment of individual services independently. This leads to faster release cycles and reduced downtime.
Moreover, using ASP.NET Core, developers can build individual microservices with its lightweight and highly performant nature. The use of containers (like Docker) further improves deployment efficiency and portability. The benefits of using containers become clear in large-scale deployments, where managing many individual services without containers becomes extremely difficult. Many companies now leverage containerization as an integral part of their deployment strategy, further enhancing the scalability and resilience of their applications. A further aspect is that the decoupled nature of microservices allows for the use of different technologies for different services. This gives greater flexibility in technology selection.
In summary, adopting a microservices architecture with ASP.NET Core allows for building highly scalable, maintainable, and resilient applications. This contrasts sharply with the limitations of traditional monolithic applications. This approach is now considered a best practice for building modern, enterprise-level web applications. The benefits extend beyond just scalability, encompassing faster development cycles, easier maintenance, and enhanced resilience, making it a powerful tool for modern software development.
Enhancing Security with Modern Practices
Security is paramount in any web application. Traditional approaches to security often fell short of today's sophisticated threats. Modern ASP.NET development incorporates advanced security measures to protect against various vulnerabilities. Consider the case study of a banking application that implemented multi-factor authentication and robust input validation, significantly reducing its vulnerability to common attacks. Another case study involves a social media platform which successfully mitigated several major security threats through the implementation of advanced security practices and regular security audits. They regularly monitor for threats and perform continuous security assessment to protect against cyber attacks.
This contrasts with older systems that relied on basic authentication methods, leaving them vulnerable to various attacks such as SQL injection and cross-site scripting (XSS). Modern ASP.NET emphasizes the use of secure coding practices, input validation, and output encoding. This mitigates the risk of vulnerabilities. A crucial aspect of modern security is regular security auditing and penetration testing. These procedures help identify vulnerabilities before they can be exploited. Many large organizations now employ dedicated security teams to perform regular audits and vulnerability assessments. They also continuously improve their security protocols based on the identified vulnerabilities.
Further, implementing robust authentication mechanisms, such as OAuth 2.0 and OpenID Connect, enhances user security and data protection. This approach contrasts sharply with the reliance on simpler forms of authentication in older systems. The use of these protocols provides stronger protection against unauthorized access to user accounts and data. This enhanced security is critical in today's connected world, where the threats are increasingly sophisticated and persistent. These protocols ensure that the security is maintained throughout the entire authentication flow.
In conclusion, modern ASP.NET security practices go beyond basic measures, incorporating a multifaceted approach that includes secure coding, robust authentication, regular security audits, and proactive threat mitigation. The focus is on building secure applications from the ground up, using modern security protocols and best practices, a far cry from the more basic security mechanisms of traditional ASP.NET development.
API-First Approach with ASP.NET
An API-first approach emphasizes designing and building APIs before the user interface. This approach allows for better decoupling of the front-end and back-end, facilitating independent development and scaling. Consider the case of a large social media company which developed a robust API first, allowing multiple frontends (mobile, web, etc.) to interact with it without impacting each other's development process. Another successful example is a major payment processing company which used an API-first approach to offer its services to various third-party applications, significantly increasing its reach and market penetration.
This contrasts sharply with traditional approaches that prioritize the user interface, resulting in tightly coupled systems that are difficult to scale and maintain. API-first architectures improve team collaboration as frontend and backend teams can work concurrently, resulting in faster development cycles. This enables faster prototyping and testing since the API can be tested independently of the frontend. This approach is valuable during iterative development where quick changes need to be made.
Furthermore, an API-first approach allows for greater flexibility in choosing front-end technologies. Different front-end technologies (e.g., web, mobile, desktop) can all interact with the same back-end API. This removes the need for separate back-end implementations for each platform. This ensures consistency and simplifies the management of the application. The consistent API across different frontends improves maintainability, especially as the application grows in size and complexity.
In summary, the API-first approach with ASP.NET promotes decoupling, independent development, scalability, and technology flexibility. This contrasts significantly with traditional approaches which result in tightly coupled, difficult-to-maintain systems. This modern methodology is a key element for successful web application development in the current landscape.
Conclusion: Moving beyond traditional ASP.NET requires embracing modern techniques and practices. By adopting approaches like Razor Pages, leveraging Blazor's capabilities, implementing microservices architecture, prioritizing security with modern methods, and using an API-first design, developers can create robust, scalable, and maintainable web applications. These techniques not only enhance the development process but also contribute to the creation of high-performing, secure, and future-proof applications. This evolution of ASP.NET development allows for greater efficiency and productivity, delivering applications that are better suited to the dynamic demands of the modern web.