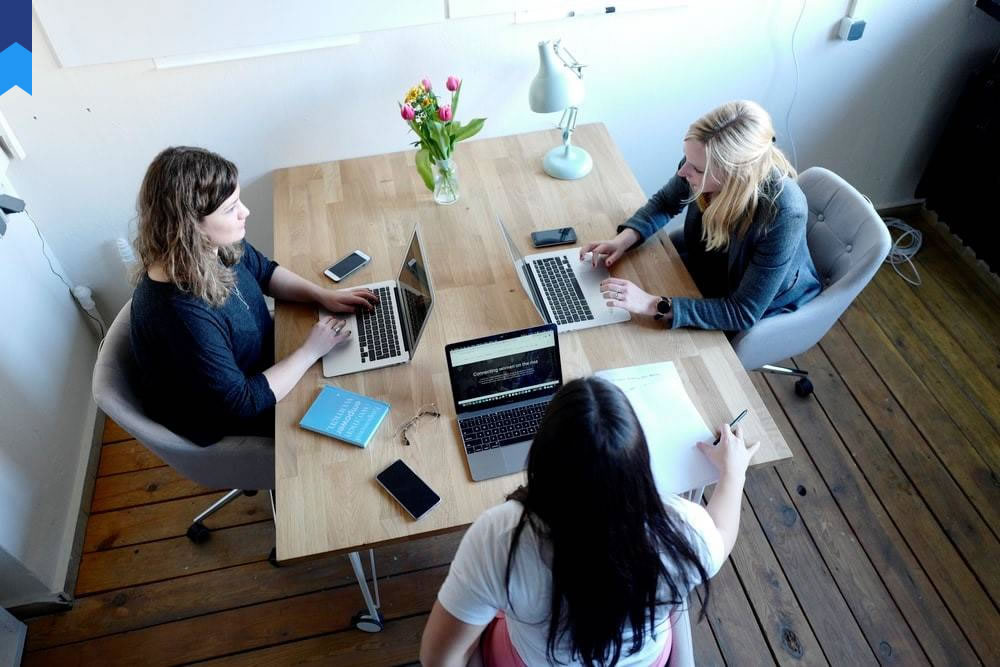
Beyond Traditional Compilers: A New Approach To Code Generation
Compiler design, traditionally a complex and often opaque process, is ripe for reinvention. This article delves into innovative approaches to code generation, moving beyond the established paradigms and exploring exciting new possibilities for optimization, efficiency, and adaptability.
Advanced Intermediate Representations (IR)
Modern compilers rely heavily on intermediate representations (IRs) to bridge the gap between source code and machine code. However, traditional IRs often struggle with the complexities of modern programming languages and hardware architectures. A new approach involves developing more sophisticated IRs that capture higher-level semantic information, enabling more aggressive optimizations. For instance, consider a compiler targeting a heterogeneous computing system comprising CPUs, GPUs, and specialized accelerators. A traditional IR might struggle to effectively map code to the optimal hardware resources. A more advanced IR, however, could incorporate annotations representing data dependencies, memory access patterns, and computation intensity, allowing the compiler to intelligently distribute the workload across the various hardware components.
Case Study 1: The LLVM project has pioneered advancements in IR design, enabling its use in a wide range of compilers for different programming languages and architectures. LLVM's sophisticated IR allows for complex optimizations that would be difficult to achieve with simpler IRs. Case Study 2: The Halide compiler leverages a domain-specific IR specifically designed for image processing, optimizing memory access and computation patterns to achieve exceptional performance.
Furthermore, incorporating machine learning techniques into the IR design process can allow the compiler to dynamically adapt its IR based on the specific characteristics of the input code and the target hardware. This adaptive IR could potentially unlock even greater levels of optimization. The incorporation of AI-driven techniques opens up the possibility of self-optimizing compilers capable of dynamically adapting their code generation strategies based on runtime performance feedback. This automated optimization process could significantly reduce development time and improve overall efficiency. This adaptive approach also has the potential to address challenges posed by the ever-evolving landscape of hardware architectures, ensuring compilers remain relevant and efficient in the face of future advancements.
Moreover, research into incorporating higher-order functions and more complex data structures into the IR allows for a more precise representation of program semantics, facilitating more advanced and fine-grained optimization techniques. The development of more expressive and nuanced IRs is essential for the effective compilation of modern programming languages featuring advanced features, such as generics, closures, and concurrency constructs. These advances pave the way for creating compilers that not only generate efficient machine code but also maintain the expressiveness and elegance of the source code.
Polyhedral Compilation Techniques
Polyhedral compilation offers a powerful framework for optimizing loops in programs. Traditional loop optimizations are often limited in their scope and effectiveness. Polyhedral compilation, however, leverages mathematical models to represent loops and their dependencies, enabling more aggressive and far-reaching transformations. This approach uses polyhedra to precisely represent the iteration space of loops. By analyzing these polyhedra, the compiler can identify opportunities for loop fusion, loop tiling, loop unrolling, and parallelization. These transformations can significantly improve performance, particularly on parallel architectures. Furthermore, polyhedral techniques can be employed to optimize memory accesses, reducing cache misses and improving data locality.
Case Study 1: The Pluto compiler uses polyhedral techniques to optimize scientific computing applications, achieving significant speedups on modern multi-core processors. Case Study 2: The PPCG compiler leverages polyhedral optimization for embedded systems, improving both code size and performance. These are just two examples, and further research continues to uncover novel application of these powerful techniques. These methods are crucial in achieving peak performance in various applications, including machine learning algorithms.
The use of sophisticated mathematical models in polyhedral compilation allows for more precise analysis and optimization compared to traditional heuristic-based methods. This precision is particularly crucial when dealing with complex loop nests and intricate data dependencies. The ability to reason formally about the transformation of loops ensures the correctness of the optimized code, a critical aspect that traditional heuristic methods often struggle to guarantee. The application of polyhedral techniques is not limited to traditional procedural programming languages; they are proving valuable in optimizing programs written in languages like Python and Java, enabling more efficient execution.
Moreover, polyhedral compilation offers potential benefits in the realm of automatic parallelization. The precise representation of data dependencies in the polyhedral model allows for the identification of opportunities for parallel execution that may be missed by simpler techniques. This opens the door to more efficient utilization of parallel architectures. The ability to transform and optimize loops in a systematic and rigorous manner enables the generation of optimized code that effectively leverages the power of modern multi-core processors and massively parallel systems. By extending the reach of parallelization techniques and providing rigorous guarantees, polyhedral compilation continues to advance the state of the art in compiler optimization.
Just-in-Time (JIT) Compilation and Dynamic Optimization
Traditional compilers perform compilation at compile time, generating machine code that is fixed. JIT compilation, in contrast, performs compilation at runtime. This allows for dynamic optimization based on the runtime behavior of the program. This is particularly beneficial for applications with dynamic behavior, such as interpreters and virtual machines, where the runtime environment is not fully known at compile time. JIT compilers can profile the running program and optimize the code based on observed execution patterns. They also allow for adaptations to the specific hardware on which the program is running, achieving optimal performance across varying architectures.
Case Study 1: The Java Virtual Machine (JVM) employs JIT compilation to optimize Java bytecode at runtime. Case Study 2: Many modern JavaScript engines utilize JIT compilation to improve the performance of web applications. The adaptability and efficiency of JIT compilation have led to its widespread adoption in various programming environments. This approach holds immense potential for future applications requiring dynamic adjustments and real-time optimization.
Furthermore, advances in machine learning are driving the development of intelligent JIT compilers capable of learning optimal compilation strategies based on past experience. These intelligent compilers can adapt their optimization techniques to specific application domains and hardware platforms, achieving even greater performance gains. Machine learning can enhance the effectiveness of profile-guided optimization, predicting future program behavior with greater accuracy, resulting in more efficient code generation. This approach, combining the flexibility of JIT compilation with the power of machine learning, represents a significant leap forward in compiler technology.
Moreover, the combination of JIT compilation and dynamic code generation techniques unlocks new possibilities for optimization beyond what is possible with static compilation. This includes the ability to dynamically specialize code for specific input data, generating optimized versions tailored to individual inputs. This targeted specialization can significantly improve performance in applications where data patterns exhibit predictable characteristics. The dynamic nature of JIT compilation also allows for adaptive optimization strategies, adjusting the generated code to accommodate changes in the runtime environment or unexpected behavior patterns, leading to improved robustness and efficiency in dynamic applications.
Domain-Specific Compilation
General-purpose compilers often struggle to achieve optimal performance for specific application domains. Domain-specific compilers, however, are designed to exploit the unique characteristics of a particular application domain. By incorporating domain-specific knowledge into the compiler, significant performance improvements can be achieved. For example, a compiler for image processing applications might incorporate knowledge of common image processing operations and data structures, enabling more effective optimization.
Case Study 1: The Halide compiler, previously mentioned, exemplifies domain-specific compilation for image processing. Case Study 2: Compilers for high-performance computing applications often incorporate specialized optimizations for linear algebra operations and other common computations in scientific computing. These examples illustrate the power of tailoring compilation techniques to the demands of specific applications.
The specialization inherent in domain-specific compilers allows for the incorporation of advanced optimization techniques that might not be feasible in general-purpose compilers. This includes the ability to exploit specific hardware capabilities or to make assumptions about the input data that would be inappropriate in a more general context. This targeted optimization leads to more efficient and effective code for the specific domain. The design of effective domain-specific compilers requires an in-depth understanding of both the target application domain and the underlying hardware architecture. This expertise enables developers to craft optimization strategies that leverage both the application's characteristics and the strengths of the hardware.
Furthermore, the development of domain-specific compilers fosters a symbiotic relationship between compiler technology and application development. By closely aligning the compiler with the needs of the application, developers gain more control over the generated code, potentially enabling new functionalities and performance levels that would not be attainable with general-purpose compilers. This integration allows for closer collaboration between compiler engineers and application developers, enhancing the overall efficiency and effectiveness of software development workflows.
Hardware-Aware Compilation
Modern hardware architectures are becoming increasingly complex, with features such as multi-core processors, SIMD instructions, and specialized hardware accelerators. Traditional compilers often fail to fully exploit these features, leading to suboptimal performance. Hardware-aware compilation focuses on generating code that effectively utilizes the specific capabilities of the target hardware. This includes considering factors such as cache sizes, memory bandwidth, and the availability of specialized instructions.
Case Study 1: Compilers targeting GPUs often incorporate specialized optimizations for parallel execution and memory access patterns. Case Study 2: Compilers for embedded systems often prioritize code size and energy efficiency, taking into account the constraints of the target hardware. The ability to tailor compilation processes to specific hardware characteristics ensures optimized performance and efficient resource utilization.
The development of hardware-aware compilation techniques requires close collaboration between compiler designers and hardware architects. This collaboration ensures that the compiler can effectively exploit the full potential of the target hardware. This collaboration often involves the development of new compiler optimizations, new intermediate representations, and new code generation strategies, leading to significant advancements in both compiler and hardware design. The resulting synergy between hardware and software optimization techniques can lead to remarkable performance enhancements in various application domains.
Moreover, the increasing prevalence of heterogeneous computing systems, composed of CPUs, GPUs, and specialized hardware accelerators, presents significant challenges for compiler technology. Hardware-aware compilation plays a critical role in achieving efficient utilization of such systems. Sophisticated compilation techniques are required to effectively distribute workloads across the heterogeneous components, optimize data transfer between components, and tailor the generated code to the unique capabilities of each component. By addressing these challenges, hardware-aware compilation contributes to a more efficient and effective use of advanced computing resources.
Conclusion
The future of compiler design lies in moving beyond traditional approaches. By embracing advanced IRs, polyhedral compilation, JIT compilation, domain-specific compilation, and hardware-aware compilation, we can unlock new levels of performance, efficiency, and adaptability. The integration of machine learning and artificial intelligence will further revolutionize the field, leading to self-optimizing compilers capable of adapting to ever-changing hardware and software landscapes. The continuous innovation in these areas promises a future where software development is more efficient, more powerful, and more accessible than ever before. The challenge lies in effectively integrating these disparate approaches and creating a unified framework for next-generation compilation technology.