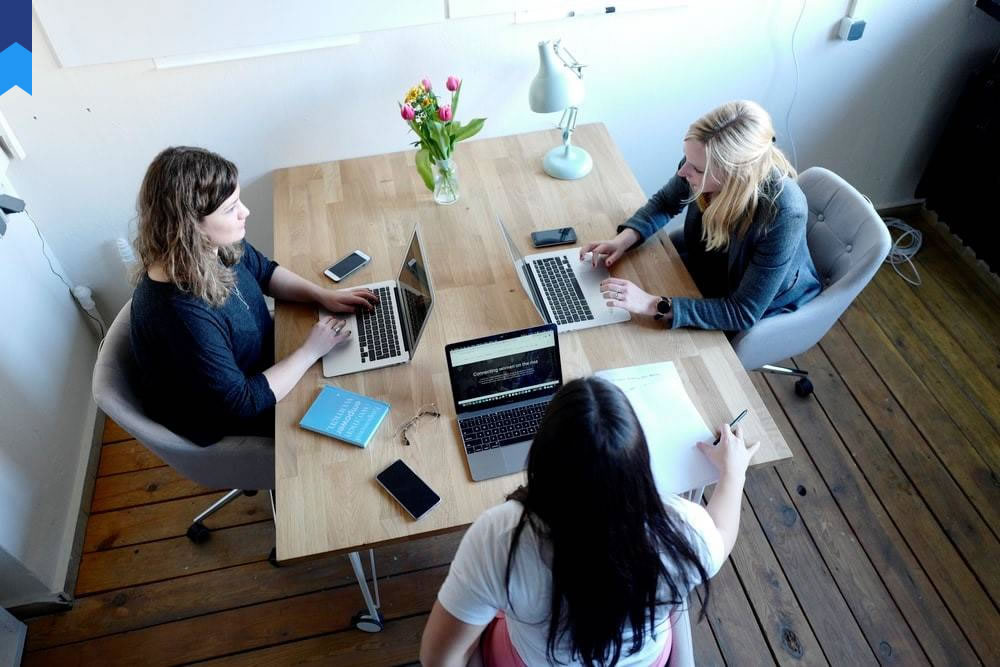
Beyond Traditional D: Mastering Advanced Techniques
D programming language, known for its performance and modern features, often gets overlooked. This article delves beyond the basics, exploring advanced techniques that unlock its true power.
Unleashing the Power of Templates
Templates are a cornerstone of D’s metaprogramming capabilities. They allow you to write generic code that works with various data types without sacrificing performance. Consider a simple example: a function to find the maximum of two numbers. In traditional languages, you might write separate functions for integers, floats, and so on. In D, you can use templates to create a single, highly efficient function:
T max(T)(T a, T b) { return a > b ? a : b; }
This function works with any type `T` that supports the `>` operator. This significantly reduces code duplication and enhances maintainability. This efficiency extends beyond simple comparisons. Consider complex data structures where you might need to compare elements based on specific criteria. Templates allow you to encapsulate these criteria within the template itself, leading to highly reusable code that can adapt to different data structures with ease.
Case Study 1: Imagine building a game engine. Templates could be used to create generic containers for game objects, regardless of their type (player characters, enemies, items). This would allow for a flexible and efficient architecture.
Case Study 2: A scientific computing library could leverage templates to efficiently handle various numerical types and vector operations, adapting to specific hardware architectures for optimal performance.
The use of templates goes beyond simple functions; they can also be applied to classes and even entire modules, enabling code generation and specialized behavior at compile time. This drastically improves runtime efficiency while maintaining the elegance and conciseness of the codebase. Mastering templates opens a world of possibilities for writing highly efficient and adaptable D code.
Furthermore, understanding template specialization allows for fine-grained control over code generation, enabling optimization based on specific types. This advanced technique provides developers with granular control over the compilation process, resulting in potentially significant performance gains in critical sections of code. Exploring advanced template metaprogramming techniques in D, such as template constraints and compile-time computations, reveals a powerful system for highly optimized and reusable code.
Conquering Concurrency with Ease
Modern applications demand concurrent processing. D's approach to concurrency, built around its powerful `std.concurrency` module, stands out for its safety and efficiency. The core concepts revolve around tasks and channels, allowing developers to express concurrency without getting lost in low-level threading details. A common task involves processing a large dataset in parallel. Using D's concurrency features, we can efficiently break down the task and distribute it across multiple cores.
Case Study 1: A large-scale data processing application could use D's concurrency features to efficiently process terabytes of data in parallel, dramatically reducing processing times. This would be essential for applications involving real-time analysis of large datasets.
Case Study 2: A web server could leverage D's concurrency model to handle multiple client requests simultaneously, maintaining high throughput and responsiveness, crucial for delivering a positive user experience under heavy load.
D offers a robust set of tools for managing shared resources and preventing race conditions. These include features like atomic operations and mutexes, integrated directly into the language and its standard library. This promotes safer concurrency paradigms, and reduces the risk of introducing hard-to-detect bugs. Mastering these mechanisms prevents data corruption and ensures application stability. Effective concurrency management is critical for maximizing the utilization of multi-core processors, and D provides the right tools to do it safely and efficiently. Its high-level concurrency abstractions allow for cleaner, more maintainable code compared to languages requiring direct interaction with operating system threads.
Beyond basic task management, D's concurrency capabilities extend to sophisticated concepts like parallel algorithms and distributed computing. Advanced techniques, such as work-stealing algorithms, further optimize the distribution of work across available cores. This careful design promotes scalability and efficiency, making D an attractive choice for complex concurrent applications. Understanding these intricacies empowers developers to build robust, scalable, and high-performance systems.
Mastering Memory Management
D provides robust memory management capabilities to address one of the most significant challenges in programming: managing memory effectively. D's approach is a hybrid, combining features of both garbage collection and manual memory management. This unique blend offers the advantages of both worlds: the ease of use of garbage collection and the fine-grained control offered by manual memory management. Using D's built-in garbage collection allows developers to focus on application logic, leaving the complexities of memory management to the runtime environment.
Case Study 1: A real-time embedded system might utilize manual memory management techniques for precise control over memory allocation and deallocation, optimizing performance in resource-constrained environments.
Case Study 2: A high-performance trading application could benefit from the fine-grained control over memory management offered by D, optimizing performance in scenarios where garbage collection pauses could be detrimental.
However, D also provides the tools for manual memory management when fine-grained control is necessary. The `new` and `delete` operators provide explicit control over memory allocation and deallocation, similar to C++. This combination caters to both rapid prototyping and situations where precise memory control is paramount, leading to highly optimized code and improved performance. This hybrid approach differentiates D from languages that rely solely on garbage collection, providing flexibility to address various application requirements.
Furthermore, D offers features like compile-time memory management checks, helping developers identify potential memory leaks and dangling pointers during compilation. These sophisticated tools not only catch errors early but also contribute to more reliable and secure code. The powerful combination of these features establishes D as a competitive choice for projects requiring both developer convenience and precise memory control.
Exploring the D Standard Library
The D standard library is a rich repository of pre-built modules, providing various functionalities for common tasks. Instead of reinventing the wheel, developers can leverage this extensive library to accelerate development. For instance, the `std.algorithm` module contains a wide range of algorithms, including sorting, searching, and transformation. These algorithms are highly optimized, making them ideal for various applications. A common use case is sorting a large dataset: using the standard library's `sort` function eliminates the need to write custom sorting algorithms, saving development time and reducing the risk of introducing errors.
Case Study 1: Imagine developing a web application. The standard library's networking modules can be used to create efficient HTTP servers and clients, significantly accelerating development. This allows developers to focus on the core application logic rather than low-level networking details.
Case Study 2: A scientific computing project could use the standard library's math modules for highly optimized numerical computations, providing readily available and tested functionalities. This streamlines the development process and ensures numerical accuracy.
The standard library also includes modules for various other tasks, including regular expressions, string manipulation, and date and time processing. The breadth and depth of these modules make the D standard library a valuable resource for any D developer. By effectively utilizing the standard library, developers can significantly reduce development time, improve code quality, and leverage pre-tested, optimized functionalities. It forms a critical component of efficient and productive D development.
Beyond the core modules, D boasts a vibrant ecosystem of third-party libraries, providing even more functionalities. These external libraries expand the capabilities of the language further, offering solutions for various specific tasks and problem domains. This wide range of options allows developers to select appropriate components for their projects, further accelerating the development process and making D adaptable to various needs. This vibrant ecosystem constantly evolves, with new libraries continually emerging, contributing to the ongoing enhancement of the D development experience.
Leveraging Metaprogramming for Advanced Capabilities
Metaprogramming in D takes advantage of compile-time code generation to create highly customized and optimized programs. This approach extends beyond templates; it involves generating code based on various factors. For instance, D's metaprogramming capabilities can be used to generate optimized code for specific hardware architectures or data types. This allows for the creation of highly efficient and portable code without manual optimization for each target platform. This approach significantly reduces development time while maintaining high performance.
Case Study 1: A high-performance computing application could use metaprogramming to generate specialized code for different hardware platforms, maximizing performance and portability across various systems. This approach ensures that the application runs optimally on each target architecture.
Case Study 2: A compiler itself could be written in D using metaprogramming, demonstrating the power and flexibility of D’s capabilities. This allows for highly customized compilation processes optimized for specific languages or hardware platforms.
Metaprogramming can be used to create domain-specific languages (DSLs) embedded within D. A DSL tailored to a specific problem domain simplifies the development process within that domain, improving code readability and maintainability. This technique makes D a powerful tool for creating customized development environments tailored to specific application needs.
Exploring D’s advanced metaprogramming techniques opens up new avenues for highly efficient, optimized, and customizable code. It extends the boundaries of what’s possible, allowing developers to write sophisticated programs with enhanced performance and adaptability across diverse platforms. Mastering these techniques is essential for unlocking the full potential of D in various applications.
Conclusion
D programming language, often underestimated, offers a compelling blend of performance, safety, and modern features. This exploration of advanced techniques reveals its true power, going beyond basic usage. By mastering templates, concurrency, memory management, the standard library, and metaprogramming, developers can unlock the potential of D for creating high-performance, maintainable, and efficient applications across various domains. The combination of these capabilities positions D as a compelling choice for diverse projects, offering a powerful and flexible alternative in the programming landscape. The ongoing growth of its community and its extensive ecosystem ensures that D remains a viable and attractive option for years to come.