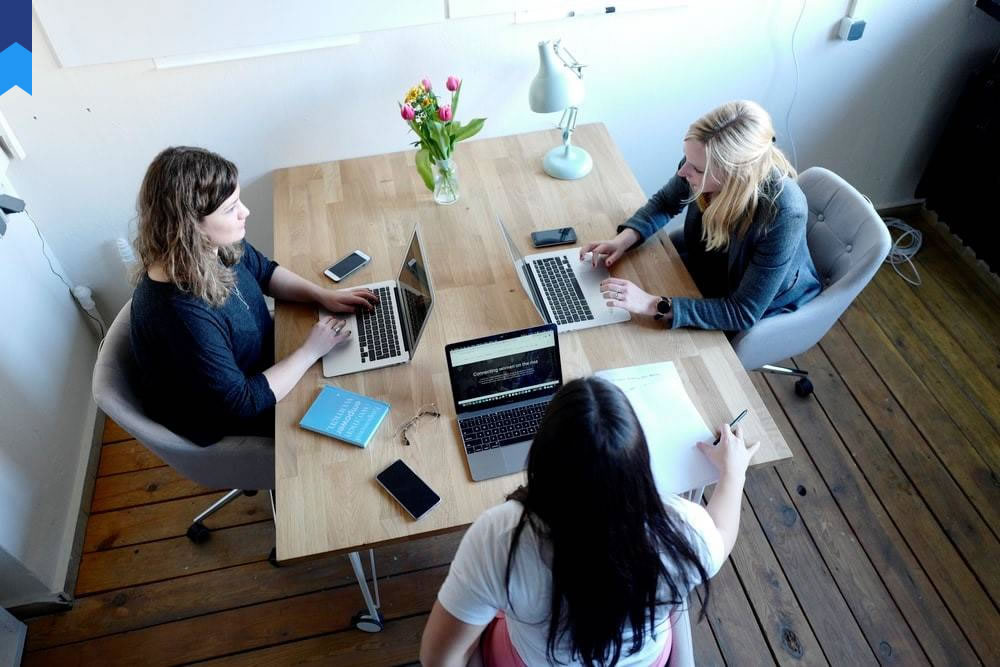
Beyond Traditional R: Mastering Data Wrangling And Visualization
R programming, a cornerstone of data science, often presents itself as a steep learning curve. This article transcends basic tutorials, delving into advanced techniques for data manipulation and visualization to unlock R's true potential.
Data Wrangling with dplyr and tidyr: Beyond the Basics
The tidyverse, particularly dplyr and tidyr, are game-changers in R. Moving beyond simple data filtering and selection, mastering these packages involves understanding complex joins, reshaping data with pivoting functions, and utilizing advanced subsetting techniques. Let's explore some key advanced functions.
Case Study 1: Imagine analyzing a large dataset of customer transactions spread across multiple tables – one for purchases, another for customer demographics, and a third for product information. Using traditional base R, joining these tables would be time-consuming and prone to errors. dplyr's `left_join`, `inner_join`, and `full_join` functions, combined with concise syntax, streamline this process dramatically. For instance, `left_join(purchases, demographics, by = "customer_ID")` efficiently merges the purchase data with customer demographics based on a common ID.
Case Study 2: Analyzing survey data often involves handling wide datasets where multiple response variables are spread across columns. Tidyr's `pivot_longer()` and `pivot_wider()` functions excel at transforming such data into tidy formats suitable for analysis and visualization. This simplifies tasks like summarizing responses based on different factors and facilitates more efficient analyses.
Beyond basic functions, understanding the nuances of data imputation using packages like `mice` for handling missing data is crucial. Implementing advanced data cleaning techniques and handling outliers intelligently is also an important aspect that many beginners overlook. We can compare techniques such as winsorizing and trimming to address outliers, selecting the most appropriate approach based on the data's characteristics and research objectives.
Experts consistently stress the importance of data quality in analysis. Data cleaning is not merely a preliminary step but an iterative process that needs to be meticulously carried out. The tidyr and dplyr packages simplify that process.
The efficiency gains provided by dplyr and tidyr are substantial. Studies show a significant reduction in code length and execution time compared to base R, contributing to better reproducibility and less opportunity for errors. Data scientists often report improved collaboration due to the improved readability of tidyverse code.
Mastering these packages involves experimenting with different functions, tackling real-world datasets, and consistently refining your techniques. The more familiar you become with the tidyverse ecosystem, the more efficient and robust your data-wrangling will be.
Furthermore, understanding advanced features such as custom functions within the dplyr framework allows for creating reusable code snippets that efficiently address recurring data manipulation needs. Creating user-defined functions helps in streamlining workflows, making the code easier to manage and more efficient. These functions can encapsulate complex cleaning, transformation and subsetting routines which can then be applied across various datasets.
Exploring the potential of these powerful tools enhances data analysis capabilities greatly, leading to insights that might otherwise be missed due to cumbersome coding or lack of streamlined data handling.
The capabilities of dplyr go beyond simple data manipulation. Utilizing dplyr in conjunction with other packages enhances efficiency. For instance, combining dplyr with data.table allows for substantial performance gains when dealing with extremely large datasets. This demonstrates the synergistic potential of various R packages.
Advanced Data Visualization with ggplot2: Beyond Static Charts
ggplot2, a grammar of graphics package, is essential for creating visually appealing and informative charts in R. Going beyond simple bar charts and scatter plots, we'll explore techniques for enhancing data storytelling and communicating insights more effectively.
Case Study 1: Instead of a simple bar chart showing sales figures across different product categories, use faceting to create multiple small charts, one for each region. This granular view reveals regional sales patterns which may be concealed in a single chart. Adding a trend line can emphasize growth or decline over time, enhancing the narrative.
Case Study 2: A scatter plot displaying the relationship between advertising spend and sales can be significantly improved by incorporating a smoother (LOESS curve) to show the overall trend. Adding color or size aesthetics based on another variable, like seasonality, can unveil additional information and show more complex relationships between the data points.
Beyond basic aesthetics, exploring advanced features like custom themes, annotations, and scales is crucial. A custom theme allows you to create a consistent visual style throughout your reports, enhancing their professionalism. Annotations allow you to highlight specific data points or patterns to improve understanding. Carefully chosen scales, such as logarithmic scales for skewed data, ensure that your visualizations are accurate and interpretable.
Interactive visualizations with packages like `plotly` and `shiny` offer a significant advancement, providing a more engaging and dynamic way to explore data. These packages can transform static ggplot2 charts into interactive dashboards allowing users to dynamically filter, zoom, and explore datasets. This approach can significantly deepen understanding and facilitate insights that static plots often miss.
Consider using interactive visualizations when working with large datasets or when presenting to stakeholders who may not be statistically inclined. Such an approach ensures the story told by the data is more accessible and impactful. The ability to explore different subsets of data with ease improves the data analysis process, aiding in making more accurate interpretations.
Experts argue that effective visualization is paramount to meaningful data analysis. A well-constructed chart can instantly clarify complex trends, making insights accessible to both technical and non-technical audiences. ggplot2's power lies not only in its visual capabilities but also in its clear and concise syntax.
Advanced ggplot2 techniques involve understanding and utilizing geoms, facets, scales, and coordinate systems effectively. Combining these elements allows creation of sophisticated visualizations conveying detailed data information.
Mastering these techniques allows users to craft compelling visualizations that go beyond simply presenting data, actively guiding viewers through the insights contained within. The ability to craft clear and concise visual narratives greatly enhances the effectiveness of data analysis.
Moreover, integrating animation into visualizations through packages such as `gganimate` adds another layer of storytelling. This dynamic approach allows viewers to trace changes in data over time, providing a richer understanding of trends and patterns.
Working with Big Data in R: Efficient Solutions
Analyzing large datasets requires efficient techniques to avoid memory issues and slow processing times. We will explore strategies for optimizing R code and leveraging tools specifically designed for big data.
Case Study 1: A company with millions of customer records needs to perform a complex analysis. Loading the entire dataset into R's memory would be impractical. Using tools such as `data.table` or `sparklyr` allows processing of data in chunks or distributing the computations across multiple cores or machines. This parallel processing speeds up computations significantly and avoids memory issues.
Case Study 2: A research team working with genomic data, involving billions of data points, faces similar challenges. Using `sparklyr`, an R interface to Apache Spark, allows them to distribute their analysis over a cluster of machines, handling the data volume effectively. This demonstrates the scalability offered by these big-data solutions.
Beyond using packages designed for large data handling, optimizing R code is crucial. Vectorized operations are significantly faster than using loops. Writing functions to avoid repetitive code and using appropriate data structures minimizes overhead. Profiling your code to identify bottlenecks guides you towards areas needing optimization.
Experts highlight the importance of understanding data structures and algorithms when dealing with big data. Choosing appropriate data structures for your specific data and understanding the computational complexities of different algorithms are crucial for efficiency. This includes understanding the tradeoffs between different approaches.
Memory management becomes paramount when handling large data. Techniques like garbage collection and careful data structure selection can significantly reduce memory footprint, preventing crashes and enabling efficient processing. These techniques require understanding of R's internal memory management, improving efficiency.
Efficient data handling in R for large datasets extends beyond using optimized packages. Combining multiple approaches strengthens effectiveness significantly. Using data.table in combination with dplyr, for example, leverages the strengths of each package for faster data manipulation and aggregation.
Moreover, effective data subsetting and sampling strategies are crucial. Analyzing a representative sample instead of the entire dataset can dramatically reduce processing time without compromising the validity of results. Careful sampling allows for fast data analysis that offers valuable insights while avoiding limitations imposed by huge dataset sizes.
Furthermore, leveraging cloud computing resources can significantly augment R's capacity to handle big data. Cloud-based platforms provide scalable computing resources, enabling efficient processing of extremely large datasets. This adaptability and flexibility make cloud solutions invaluable for big-data projects.
Careful consideration of these techniques is crucial for successful big data analysis using R. The combination of optimized packages and well-structured code forms a robust approach.
Reproducible Research with R Markdown: Beyond Static Reports
Reproducible research is crucial for ensuring the validity and reliability of findings. R Markdown facilitates this by combining code, results, and narrative in a single document.
Case Study 1: A research project involving complex statistical modeling benefits significantly from R Markdown. The code for data cleaning, model building, and visualization is embedded directly within the report. This makes it possible to regenerate the entire analysis from scratch, ensuring that all results are verifiable and reproducible.
Case Study 2: A business analyst creating a report on customer behavior can integrate data visualization directly into the report. Each chart is generated dynamically from the data, making it easier to update the report whenever new data becomes available, guaranteeing the report's accuracy and relevance.
R Markdown goes beyond simple reports. It can generate interactive web applications, HTML slides, and even books. This adaptability makes it a versatile tool for diverse data-driven communication needs. The dynamic nature of R Markdown offers substantial advantages in disseminating research findings.
Experts emphasize the importance of documentation in reproducible research. Clear and concise documentation within R Markdown ensures that others can understand and replicate your analysis. This improves transparency and enables collaboration.
Using R Markdown effectively involves mastering its syntax, learning how to incorporate various types of output (plots, tables, equations), and employing version control systems like Git to track changes over time. This structured workflow promotes clarity and simplifies collaborative efforts.
Mastering R Markdown’s advanced features enables creation of sophisticated, self-contained reports that include not only results but also the entire workflow that generated them, leading to highly trustworthy analysis.
The integration of various output formats adds to the versatility of R Markdown. The ability to seamlessly create different output formats from the same source file strengthens its role as a central tool for creating comprehensive reports.
Furthermore, R Markdown’s ability to incorporate LaTeX for mathematical equations enhances its power in communicating complex technical information clearly and effectively, aiding in precise scientific communication.
The combination of R’s statistical capabilities and R Markdown’s reporting functionality creates a robust framework for reproducible research. The ease of integrating code and narrative into a single, consistent document is a significant advantage in producing transparent and verifiable results.
Integrating R with Other Tools: Expanding Capabilities
R's power is further amplified when integrated with other tools and technologies.
Case Study 1: A data engineer working with a large database can use R in conjunction with SQL to pull data efficiently. R's analytical capabilities are then applied to the extracted data, resulting in powerful combined data analysis.
Case Study 2: A web developer can integrate R's visualizations into a web application using tools like Shiny. This enables interactive exploration of data directly within the application, enriching user experience significantly.
Integrating R with other tools enhances the capabilities of the entire system. This combined approach allows for a more streamlined workflow, making the complete data process more efficient.
Experts often encourage exploration of different tools and technologies to maximize effectiveness. The integration of R with other platforms strengthens data science workflows significantly.
Effective integration relies on understanding the strengths and weaknesses of each tool and how they can complement each other. Careful planning allows for robust and efficient workflows.
Modern data science projects often necessitate integrating multiple technologies and tools. R acts as a pivotal component in this technological ecosystem, expanding overall functionality significantly.
The versatility of R allows seamless integration with numerous technologies and platforms, fostering collaborative work environments and enriching the entire data science workflow.
Furthermore, integrating R with machine learning frameworks like TensorFlow and Keras expands R’s capabilities into the realm of predictive modeling and deep learning. This combination opens up avenues for more sophisticated data analysis and insight generation.
The combination of R with diverse technologies and frameworks showcases its adaptability and emphasizes its role as a critical tool within a broader data science environment.
Conclusion
Mastering R extends far beyond basic tutorials. This article has highlighted advanced techniques in data wrangling, visualization, big data handling, reproducible research, and integration with other tools. By embracing these advanced approaches, data scientists can unlock R's true potential, extracting deeper insights and creating more impactful visualizations. The future of R lies in its continued evolution and integration within a broader data science ecosystem, empowering users to tackle increasingly complex data challenges with sophistication and efficiency.
The journey to mastering R is continuous. Consistent practice, exploration of new packages, and a keen understanding of the underlying principles are crucial for success. As the field of data science evolves, mastering advanced R techniques remains essential for remaining at the forefront of this dynamic discipline. The key to success lies in continuous learning and adaptive utilization of the vast resources available within the R ecosystem.
By adopting these advanced strategies, data scientists can improve the quality, efficiency, and impact of their work, ultimately driving more informed decisions and contributing to a deeper understanding of the data-rich world around us.