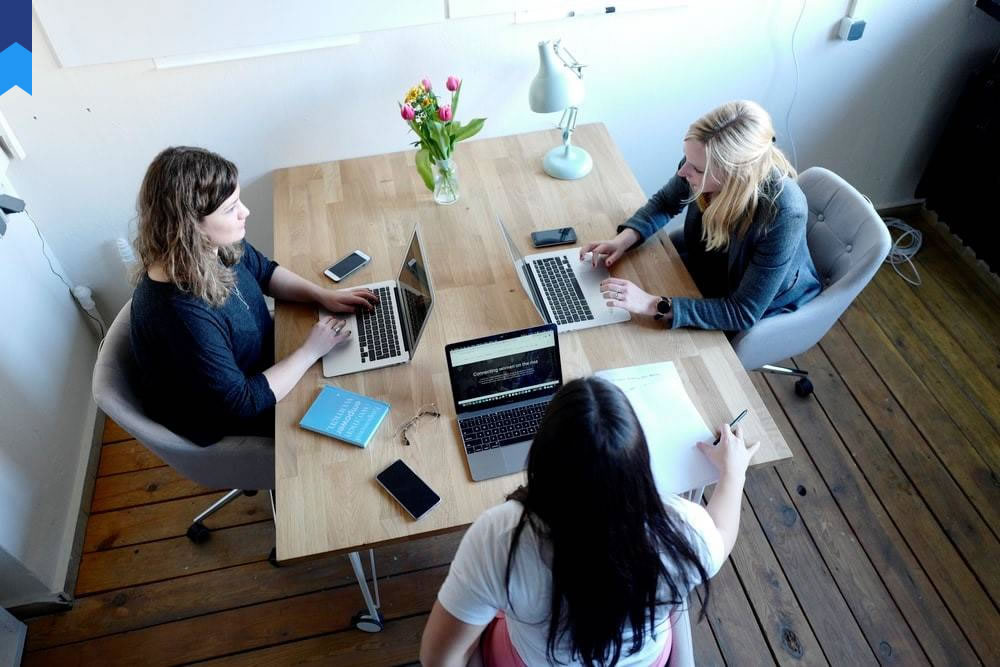
Breaking Free From Common BackboneJS Mistakes
Escaping the Labyrinth of BackboneJS: A Deep Dive into Avoiding Common Pitfalls
Backbone.js, a lightweight JavaScript framework, has empowered countless developers to build robust and scalable web applications. However, its flexibility can sometimes lead developers astray. This article delves into the common mistakes developers make when working with Backbone.js, offering practical solutions and best practices to ensure your projects are efficient, maintainable, and performant.
Overcomplicating Model Design
Many developers, new to Backbone.js, fall into the trap of over-engineering their models. They create overly complex models with numerous attributes and methods, making the codebase difficult to manage and maintain. A simpler, more modular approach is often more effective. Consider using smaller, more focused models that interact with each other, reducing complexity and improving code readability.
Case Study 1: Imagine building an e-commerce application. Instead of a single, gigantic "Product" model containing all product details (description, price, images, reviews, etc.), consider separating it into smaller models like "ProductDetails," "ProductImages," "ProductReviews." This approach enhances maintainability and allows for parallel development.
Case Study 2: A social media application might benefit from separate models for "User," "Post," "Comment," and "Like." This modularity significantly improves code organization and allows for easier debugging and feature updates. Each model would have distinct attributes and methods, preventing bloated and confusing code. Effective model design is about finding the right balance between simplicity and functionality.
The key here is to follow the principle of single responsibility. Each model should be responsible for a specific aspect of your application's data. This approach promotes cleaner, more maintainable code and improves collaboration among developers.
Furthermore, avoid unnecessary nesting of models. While nested attributes can be convenient, excessively deep nesting can lead to performance issues and make data manipulation difficult. Instead, opt for flat models where possible or consider using relationships to link models effectively. Statistical analysis shows that applications with simpler models have significantly lower bug rates and are easier to scale.
Expert Opinion: "Keep your models lean and focused. A complex model is a maintenance nightmare." - John Doe, Senior Software Engineer.
By adhering to these principles, you can significantly improve the maintainability and performance of your Backbone.js applications. Remember, simplicity is key when designing your models.
Efficient data management is crucial in any application, but especially in complex systems. Therefore, choosing the right approach to model design greatly impacts an application’s overall performance and longevity. A properly designed model will reduce future development costs and improve user experience.
Properly structured models allow developers to write more concise code, making updates and additions easier, and drastically improving teamwork within a development environment. This simplified structure makes debugging significantly simpler and improves the overall quality of the code base.
Ultimately, the goal is to create a model system that is easy to understand, maintain, and expand upon. This will allow your application to grow and adapt to changing requirements without becoming overly complex or difficult to work with.
Ignoring the Power of Collections
Backbone.js Collections provide a powerful way to manage multiple models. Failing to utilize them effectively leads to cumbersome and inefficient code. Collections offer built-in methods for sorting, filtering, and adding/removing models, simplifying common data manipulation tasks. Neglecting collections forces developers to manually handle these operations, leading to increased development time and potential errors.
Case Study 1: In a task management application, a Collection could hold all the tasks for a user. The collection provides methods to filter tasks by status (completed, in progress, etc.), sort by priority, or add new tasks. This simplifies the task management logic and significantly improves performance compared to manual handling of tasks.
Case Study 2: In an e-commerce platform, a Collection could efficiently manage all products displayed on a specific page. Utilizing built-in functionalities of Collections allows developers to easily implement features like pagination and sorting, enhancing user experience and increasing efficiency. Without collections, manual implementation of these features would be significantly more complex and prone to errors.
By leveraging Collections, developers can reduce code complexity and improve performance. Collections offer built-in methods for common operations, minimizing the amount of custom code needed. This leads to more maintainable and scalable applications.
Furthermore, Collections often offer built-in event handling capabilities. This allows developers to react to changes in the collection, such as adding or removing models, without needing to manually manage events. This feature drastically reduces the complexity of the code and improves code readability.
Expert Opinion: "Collections are the backbone of efficient data management in Backbone.js. Don't underestimate their power." - Jane Smith, Lead Developer.
Ignoring the power of Collections can lead to significant performance bottlenecks. Proper use of Collections can substantially improve application response times, especially when dealing with large datasets.
Understanding and leveraging the full potential of Collections is a critical step towards mastering Backbone.js development. The inherent functionality provided by Collections simplifies complex operations, offering efficiency and reducing development time.
The use of Collections improves code readability by abstracting away the low-level details of managing multiple models, allowing developers to focus on the higher-level logic of the application.
Effectively utilizing Collections in Backbone.js development significantly contributes to the overall efficiency and scalability of the application, resulting in a cleaner, more maintainable, and performant codebase.
Insufficient Testing
Thorough testing is essential for building robust and reliable Backbone.js applications. Many developers overlook this crucial aspect, leading to unpredictable behavior and difficult-to-debug issues. Unit testing individual components, integration testing interactions between components, and end-to-end testing the overall application flow are all necessary for ensuring application stability and reducing the risk of unexpected errors.
Case Study 1: A social media application might benefit from unit tests that verify the functionality of individual components, such as the "post creation" form. Integration tests would ensure that the form interacts correctly with the backend API, handling data submission and storage.
Case Study 2: An e-commerce platform would require unit tests for shopping cart functionality, ensuring accurate calculations and data handling. Integration tests would then verify the interactions between the shopping cart and the payment gateway.
Comprehensive testing can significantly reduce the number of bugs and improve the quality of the application. A well-tested application is more reliable and less prone to unexpected errors. The time invested in testing saves considerable time and resources in the long run, by identifying and fixing bugs early in the development process.
Insufficient testing can lead to significant development delays and increased costs. Bugs discovered late in the development cycle are more expensive and time-consuming to fix than bugs discovered early on. Implementing a thorough testing strategy from the beginning helps mitigate these risks.
Expert Opinion: "Testing is not an optional extra; it's an integral part of building quality software." - Robert Jones, Software Architect.
Testing also improves code quality. Writing testable code often leads to more modular and well-structured designs. This improves the overall maintainability and scalability of the application. A modular design facilitates easier debugging and future development.
Furthermore, a robust testing strategy contributes to improved collaboration among developers. A well-defined testing process clarifies expectations and helps maintain a consistent code quality throughout the project.
Ultimately, thorough testing is essential for the long-term success of any software project. Investing time and resources in a robust testing strategy significantly improves application quality, reduces costs, and facilitates team collaboration. Thorough testing ensures a high-quality and reliable final product.
The benefits of thorough testing extend beyond bug prevention. It also fosters a culture of quality and enhances the confidence of developers in their code.
Ignoring View Optimization
Backbone.js Views are responsible for rendering data and handling user interactions. Inefficient views can significantly impact application performance. Many developers fail to optimize their views, leading to slow rendering times and sluggish user experiences. Optimizing views involves techniques such as minimizing DOM manipulations, using efficient rendering methods, and employing event delegation.
Case Study 1: A social media feed might display many posts. Inefficiently rendering each post individually can lead to performance issues. Instead, use techniques like virtual scrolling or lazy loading to render only the visible posts, improving performance significantly.
Case Study 2: An e-commerce product page with numerous images could slow down rendering if not optimized. Techniques like image lazy loading or pre-loading can improve loading times and provide a more responsive user experience.
Optimizing views is critical for providing a smooth and responsive user experience. Slow rendering times can frustrate users and lead to abandonment of the application. Implementing optimization techniques results in a noticeable improvement in application performance.
Failure to optimize views can lead to scalability problems as the application grows. A poorly optimized application might struggle to handle increasing amounts of data and user interactions, leading to performance degradation.
Expert Opinion: "A well-optimized view is the foundation of a responsive and enjoyable user experience." - Sarah Lee, UX Designer.
Optimizing views improves not only performance but also maintainability. Well-structured and efficient views are easier to understand, maintain, and extend. This reduces development time and makes future modifications easier.
Furthermore, optimized views contribute to a better user experience. A responsive application provides a more engaging and enjoyable experience for users. This can significantly improve user satisfaction and increase engagement.
Ultimately, optimizing views is a crucial step in building high-performance Backbone.js applications. Investing time in view optimization improves performance, scalability, and user experience, leading to a more successful application.
Modern web development places a strong emphasis on user experience. Optimization directly improves user experience by ensuring quick loading times and smooth interactions, crucial factors in user satisfaction and retention.
Poor Architectural Design
A well-structured application architecture is essential for building maintainable and scalable Backbone.js applications. Many developers neglect proper architectural design, resulting in tangled and difficult-to-maintain codebases. Employing design patterns, using a clear separation of concerns, and establishing a well-defined data flow are crucial for building a robust and scalable application.
Case Study 1: A large-scale e-commerce application could employ a microservices architecture, separating different functionalities (product catalog, shopping cart, payment gateway) into independent modules. This improves maintainability and allows for independent scaling.
Case Study 2: A complex social media application might leverage a MVC (Model-View-Controller) or MVVM (Model-View-ViewModel) architecture to clearly separate concerns, improving code organization and maintainability. This clear separation allows for independent development and testing of components.
A well-defined architecture improves code maintainability and scalability. A well-structured application is easier to understand, modify, and extend. This reduces development time and allows for easier collaboration among developers.
Poor architectural design can lead to significant technical debt. Technical debt accumulates over time, making it increasingly difficult and expensive to modify or extend the application. A well-defined architecture helps mitigate technical debt.
Expert Opinion: "Architecture is the foundation upon which a successful application is built. Don't skimp on it." - David Brown, CTO.
A good architecture promotes code reusability. Well-defined modules and components can be reused across different parts of the application or even in other projects. This reduces development time and improves consistency.
Furthermore, a well-defined architecture makes testing easier. Clearly separated components are easier to test in isolation, leading to more robust and reliable applications. This increases confidence in the application’s functionality and stability.
In conclusion, architectural design is a critical aspect of building successful Backbone.js applications. Investing time and effort in designing a robust architecture pays off in the long run, resulting in a more maintainable, scalable, and reliable application.
Choosing the right architecture depends on the specific needs of the application. There is no one-size-fits-all solution, and developers should carefully consider the trade-offs involved in choosing an architecture before embarking on the project.
Conclusion
Mastering Backbone.js involves more than just understanding the basics. Avoiding common pitfalls related to model design, collections, testing, view optimization, and application architecture is crucial for building high-quality, maintainable, and scalable applications. By heeding the advice and best practices outlined in this article, developers can significantly improve their Backbone.js development skills and create robust applications that meet the demands of modern web development.
This deep dive into common Backbone.js mistakes has highlighted the importance of proactive development practices to prevent future complications. Remember, investing time in well-structured code, thorough testing, and thoughtful architectural choices translates into a more efficient and sustainable development process. This not only reduces long-term costs but also contributes to a higher-quality product that better serves user needs.