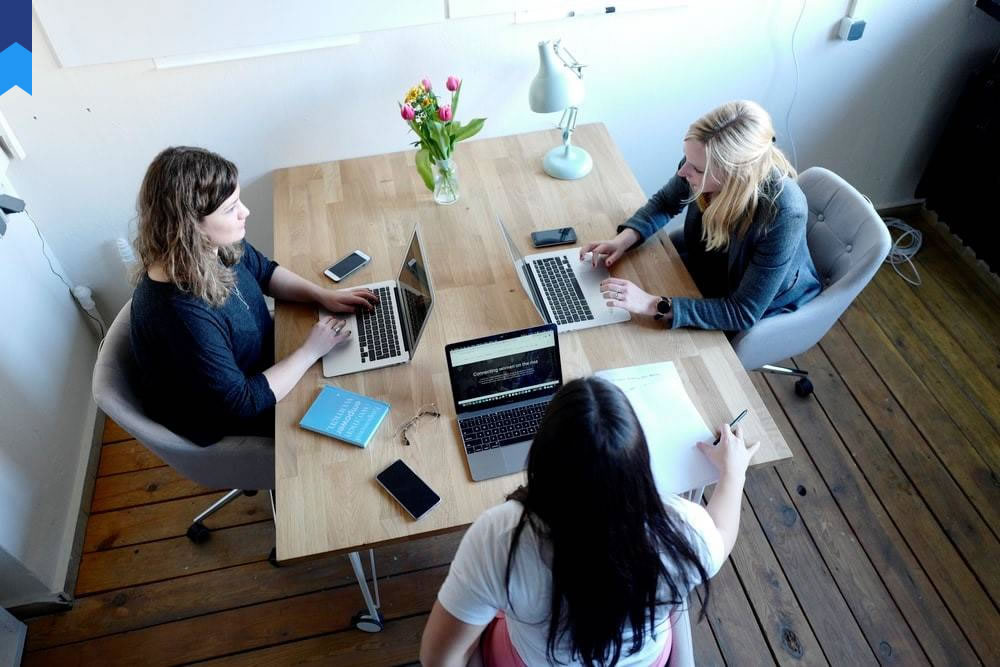
Breaking Free From Common Ethereum Development Mistakes
Ethereum, a decentralized platform renowned for its smart contract capabilities and burgeoning decentralized applications (dApps), presents a unique set of challenges for developers. While its potential is immense, numerous pitfalls can derail even the most experienced programmers. This article delves into these common mistakes, offering practical solutions and innovative approaches to ensure successful Ethereum development.
Gas Optimization Strategies: Mastering Efficiency on the Ethereum Network
Gas optimization is paramount in Ethereum development. High gas fees can significantly impact the cost-effectiveness and scalability of dApps. A common mistake is neglecting efficient coding practices. For example, unnecessary loops, complex computations, and inefficient data structures can drastically inflate gas consumption. Optimizing code through techniques like using the most efficient data structures, minimizing external calls, and employing pre-compiles can significantly reduce gas costs. Let’s examine two case studies. In the first, a dApp using Solidity failed to optimize for storage access, resulting in a 50% increase in gas fees compared to a more efficient implementation. In the second, a decentralized exchange (DEX) employed an inefficient algorithm for matching orders, leading to significantly higher transaction fees than its competitors. Solidity best practices, such as avoiding unnecessary state variables, and using efficient loops, are critical for reducing gas usage. This directly impacts the user experience and the economic viability of the application.
Furthermore, developers often overlook the importance of gas estimation. Accurate gas estimation is crucial for preventing transaction failures due to insufficient gas. Underestimating gas needs can lead to transaction reversals and wasted funds, while overestimating can increase costs needlessly. Utilizing tools such as gas analyzers and debuggers can improve gas estimation accuracy. Effective use of these tools helps identify inefficient code segments and rectify them. Analyzing contract bytecode and employing tools such as Remix or Hardhat can unveil areas for improvement. Advanced techniques like inlining functions and using assembly code can further refine gas efficiency, but require a higher level of expertise and should be implemented cautiously.
Advanced techniques like optimizing storage layouts and employing efficient data structures can significantly enhance gas efficiency. For example, using packed storage can reduce the amount of storage consumed, leading to lower gas fees. Understanding the complexities of Ethereum's internal mechanisms and the gas cost associated with different operations is essential for effective optimization. This requires a deep understanding of the Ethereum Virtual Machine (EVM) and its operation. Regular code reviews and the adoption of rigorous testing methodologies play a vital role in identifying and rectifying gas optimization issues.
Careful planning and design are critical for gas optimization. The design choices made at the beginning of a project can significantly affect the gas efficiency of the final product. Thoroughly testing and profiling the application with various inputs and scenarios is essential for discovering and fixing gas consumption bottlenecks. Utilizing tools like Remix and Hardhat can simplify and streamline this process. This iterative process, combining coding best practices with rigorous testing, can result in substantially more efficient applications.
Security Best Practices: Shielding Against Common Vulnerabilities
Security is paramount in Ethereum development, yet vulnerabilities remain a prevalent issue. Many developers overlook crucial security best practices, leaving their dApps susceptible to attacks. One common mistake is failing to perform thorough security audits before deployment. Without rigorous audits, vulnerabilities can easily go unnoticed, potentially leading to significant financial losses and reputational damage. Consider the case of The DAO hack, where a vulnerability in the smart contract allowed attackers to drain millions of dollars worth of ETH. This underscored the critical importance of thorough security audits. Similarly, a vulnerability discovered in a DeFi protocol led to a massive exploit, highlighting the need for comprehensive security assessments.
Another prevalent mistake is neglecting input validation. Failing to validate inputs can expose dApps to various attacks, such as integer overflow and underflow. These vulnerabilities can be easily exploited by malicious actors, compromising the security and integrity of the application. For instance, a project relying on user-provided inputs without proper validation was exploited, allowing attackers to manipulate data and disrupt the application’s functionality. A similar scenario involved a flawed access control mechanism leading to unauthorized access and fund theft. To prevent such vulnerabilities, meticulous input validation is critical. All user inputs must be carefully sanitized and validated to prevent malicious manipulation.
Furthermore, developers often overlook the significance of access control mechanisms. Inadequate access control can grant unauthorized users access to sensitive data or functionalities, resulting in breaches and potential financial losses. Implementing robust access control measures is essential for protecting sensitive information and securing the overall integrity of the dApp. A well-defined access control system should carefully regulate user permissions and restrict access only to authorized entities. Without this, malicious actors could potentially gain unwarranted access, leading to significant security vulnerabilities.
Regular security updates and patches are crucial for addressing newly discovered vulnerabilities. The rapidly evolving nature of the Ethereum ecosystem means that new vulnerabilities are constantly being discovered. Therefore, it’s vital to stay updated on security alerts and promptly address any identified vulnerabilities. Failing to apply patches leaves dApps open to exploitation. The swift adoption of patches and updates is a crucial aspect of maintaining the application's security posture. Active monitoring for security threats and the proactive implementation of security measures are essential for minimizing vulnerabilities and preventing exploits.
Scalability Solutions: Addressing Ethereum's Transaction Throughput Limitations
Ethereum's scalability has been a persistent challenge. High transaction volumes can lead to network congestion and slow transaction confirmation times, significantly impacting the user experience. Developers often fail to consider scalability during the design phase, leading to applications that struggle to handle large numbers of transactions. Layer-2 scaling solutions, such as state channels, rollups, and sidechains, offer promising avenues to address this issue. However, many developers overlook the complexities involved in implementing and integrating these solutions.
State channels, for instance, allow for off-chain transactions that are only settled on the main Ethereum blockchain periodically. This reduces the load on the main network and improves transaction throughput. However, the complexity of implementing and managing state channels can be a deterrent. Understanding the intricacies of state channel management, including the complexities of state transitions, settlement processes, and security considerations, is critical for successful implementation. A poorly designed state channel can easily lead to vulnerabilities and inefficiencies.
Rollups are another popular layer-2 scaling solution. They bundle multiple transactions off-chain, then submit a concise summary to the main chain for verification. This significantly reduces the load on the main chain while maintaining security. However, developing and deploying rollups requires specialized knowledge and infrastructure. The technical intricacies involved in data compression, cryptographic verification, and dispute resolution mechanisms demand a deep understanding of cryptographic principles and consensus mechanisms. Failure to address these issues correctly can significantly impact performance and security.
Sidechains provide an alternative approach to scaling by creating separate blockchains that are linked to the main Ethereum chain. They offer greater flexibility in terms of consensus mechanisms and transaction throughput, but also introduce challenges related to security and interoperability. The complexities of securing the sidechain, ensuring its compatibility with the main chain, and managing the flow of assets between the two chains can be significant. A poorly secured or designed sidechain could undermine the security of the entire system.
Smart Contract Design Patterns: Building Robust and Maintainable Applications
Smart contract design patterns are crucial for developing robust and maintainable dApps. Many developers overlook the importance of adhering to established design patterns, resulting in poorly structured and difficult-to-maintain code. Common mistakes include neglecting modularity and reusability, leading to complex and hard-to-understand codebases. Employing well-established design patterns, such as the Factory pattern, the Singleton pattern, and the Proxy pattern, can enhance code quality and maintainability.
The Factory pattern, for instance, can be used to create objects without specifying their concrete classes. This enhances flexibility and simplifies the creation of new objects. In the context of smart contracts, this pattern can be used to dynamically deploy contracts based on user input or other criteria. However, improper implementation can lead to vulnerabilities, particularly if not properly secured. The Singleton pattern ensures that only one instance of a class is created, often used for managing resources or global state. However, it can lead to unexpected behavior or difficulties in scaling if not implemented carefully.
The Proxy pattern allows for indirect access to an object through an intermediary. This can be beneficial for enhancing security and maintainability. In the case of smart contracts, the proxy pattern can be employed to control access to the underlying contract or to upgrade the contract without affecting the users. The proper implementation of proxy contracts is essential for enhancing security and flexibility. Incorrect implementation can introduce vulnerability and unexpected behaviors.
Developers frequently overlook the importance of error handling and exception management in their smart contracts. Ignoring these aspects can lead to application failures or unpredictable behavior. Properly handling errors and exceptions is paramount for maintaining the integrity and reliability of the dApp. Implementing robust error handling mechanisms can help to prevent unexpected disruptions and maintain application stability. The correct usage of `require`, `revert`, and `assert` statements in Solidity code is essential for appropriate error handling.
Testing and Debugging Strategies: Ensuring Reliability and Functionality
Thorough testing and debugging are essential for ensuring the reliability and functionality of Ethereum dApps. Many developers underestimate the importance of comprehensive testing, leading to deployments with hidden bugs and vulnerabilities. Automated testing frameworks, such as Hardhat and Truffle, offer valuable tools for streamlining the testing process and identifying potential issues before deployment. However, relying solely on automated testing is insufficient. Manual testing is equally crucial to ensure that all functionalities work as intended.
Unit tests focus on individual components of the smart contract, while integration tests assess the interaction between multiple components. System tests examine the overall functioning of the application. Employing a multi-faceted testing approach combining unit, integration, and system tests is critical for comprehensive testing. Failing to incorporate different levels of testing can result in overlooking significant issues. Using various testing strategies helps uncover a broader range of bugs and improves the quality of the application.
Debugging smart contracts can be more challenging than debugging traditional applications due to the limitations of the EVM. Utilizing debugging tools and techniques is crucial for pinpointing and resolving issues efficiently. These tools allow developers to step through the execution of smart contracts, inspect variables, and identify the root cause of errors. Advanced debugging strategies, such as using Remix’s debugger or employing logging mechanisms, can significantly enhance the debugging process. Utilizing debuggers and logging mechanisms in tandem improves effectiveness.
The importance of thorough documentation cannot be overstated. Well-documented code is easier to understand, maintain, and debug. Comprehensive documentation makes it easier for other developers to contribute or understand the codebase. Without proper documentation, the maintainability of the codebase is significantly impaired, causing higher costs in terms of maintenance and updates. Adhering to best practices in documentation significantly improves long-term maintainability and collaboration.
Conclusion
Ethereum development presents unique challenges that demand meticulous attention to detail and a commitment to best practices. By avoiding common mistakes related to gas optimization, security, scalability, design patterns, and testing, developers can significantly improve the reliability, security, and scalability of their dApps. Proactive approaches, rigorous testing, and a deep understanding of Ethereum's underlying architecture are critical for building successful and sustainable applications on this transformative platform. The ongoing evolution of the Ethereum ecosystem underscores the need for continuous learning and adaptation to the latest advancements and security best practices. The adoption of industry best practices and the continuous improvement of development methodologies will be instrumental in unlocking the full potential of this revolutionary technology.