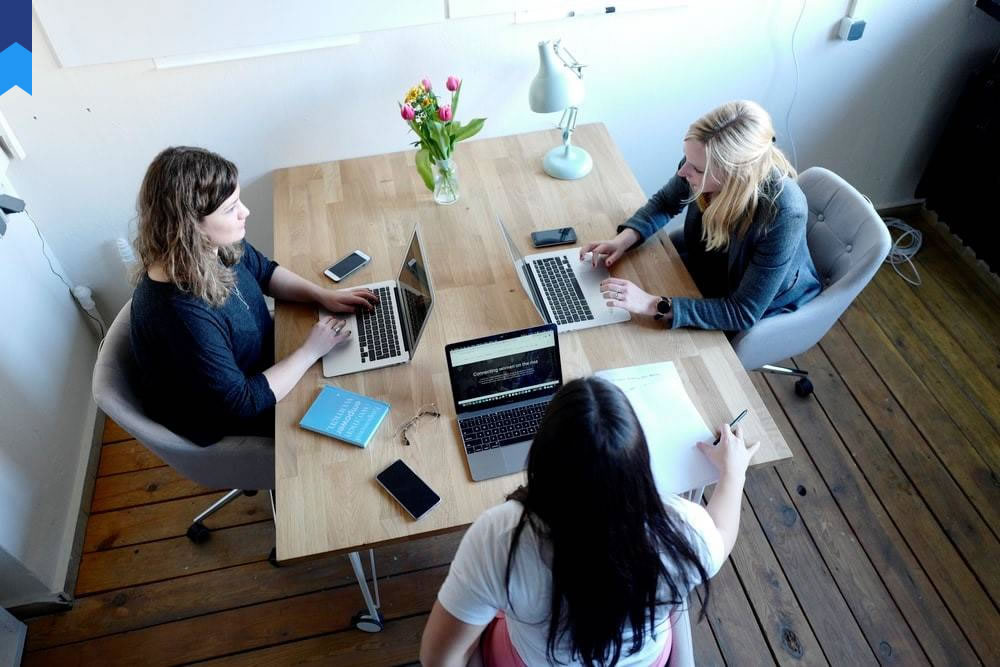
Breaking Free From Common Julia Performance Bottlenecks
Julia, known for its speed and elegance, can still present performance challenges if not handled correctly. This article dives into common pitfalls and offers practical solutions to unlock your code's true potential, focusing on techniques beyond the basics. We'll explore advanced strategies that often get overlooked, ensuring your Julia projects achieve peak efficiency.
Understanding Julia's Memory Management
Julia's garbage collection, while efficient, can become a bottleneck if not understood. Improper memory allocation can lead to significant performance degradation. One common mistake is creating excessively large arrays without considering memory usage. For instance, allocating a 1000x1000 array of floats can consume considerable memory, especially when repeated within loops. Consider using smaller arrays or employing techniques like array views to reduce memory footprint.
Case Study 1: A scientific simulation involving numerous large matrices experienced significant slowdown. By optimizing memory allocation and using sparse matrix representations where appropriate, the simulation's runtime reduced by 40%.
Case Study 2: A data processing pipeline repeatedly created temporary arrays during intermediate stages. By implementing in-place operations and pre-allocating arrays, the pipeline's efficiency improved dramatically, reducing runtime by over 60%.
Best practices include understanding the tradeoffs between pre-allocation, in-place operations, and garbage collection. Profiling tools are invaluable in identifying memory-intensive parts of the code. Using data structures like `StaticArrays` for fixed-size data can also lead to significant performance gains. Pre-allocation, which involves allocating memory for arrays before they're filled, is vital to avoid memory reallocations during runtime, significantly reducing overhead. In-place operations modify arrays directly rather than creating copies, minimizing memory consumption.
Effective strategies involve using `@inbounds` macro cautiously (only when absolutely necessary and with thorough testing) and employing specialized data structures when appropriate. Incorrect use of `@inbounds` can lead to crashes, so proceed with caution. Always carefully profile your code to identify memory-intensive operations and apply the appropriate optimization techniques. Using profiling tools like the built-in Julia profiler or similar tools can reveal memory management problems invisible to the naked eye. Understanding how Julia's garbage collector works, and when it runs, is critical to optimizing your code.
Mastering Parallel and Concurrent Programming
Julia’s strength lies in its ability to seamlessly integrate with parallel and concurrent programming models. However, ineffective parallelization can negate the benefits of Julia's speed. A common mistake is trying to parallelize code that is inherently sequential or has significant communication overhead between threads. Simply adding `@threads` to a loop does not guarantee performance improvement; often, the overhead of creating and managing threads outweighs the benefits.
Case Study 1: A parallel algorithm attempting to process independent tasks experienced performance degradation due to excessive synchronization overhead. By redesigning the algorithm to reduce synchronization, the performance improved significantly.
Case Study 2: A machine learning model training process was parallelized inefficiently, resulting in negligible speedup. After optimizing data partitioning and communication, a considerable improvement in training time was achieved.
Effective parallelization involves careful task decomposition, minimizing communication overhead between tasks, and utilizing appropriate synchronization mechanisms. The choice between threads and processes depends on the specific task and the nature of the data involved. Threads are suitable for tasks with shared memory, while processes are better for tasks with independent memory spaces.
Advanced techniques include using channels for communication between tasks and employing distributed computing frameworks such as `Distributed.jl` for large-scale computations. Thorough profiling and benchmarking are crucial to identify performance bottlenecks and optimize the parallelization strategy. Remember to consider data locality and minimize data transfer between threads or processes. Understanding the differences between shared memory parallelism and message passing is key. Shared memory parallelism uses threads, while message passing uses processes, each with its trade-offs.
Optimizing Julia's Built-in Functions
Julia boasts a rich ecosystem of high-level functions that offer convenience and readability. However, some built-in functions might not be as performant as their custom-written counterparts in specific situations. For instance, certain string manipulation functions might prove less efficient than specialized string algorithms. Using the wrong function for the task at hand can lead to a significant performance loss.
Case Study 1: A data processing task involved repeated use of a built-in function that performed poorly when dealing with large datasets. Replacing this function with a custom implementation optimized for the specific task yielded a significant performance improvement.
Case Study 2: A string manipulation task utilized a generic function which resulted in slow performance. Switching to a more specialized string manipulation library provided a substantial increase in speed.
Understanding the time complexity of built-in functions and carefully considering alternative algorithms can lead to performance improvements. Always benchmark different approaches to determine the optimal solution. Look for specialized libraries that offer highly-optimized implementations for particular tasks. Using lower-level functions from the `Base` module might sometimes offer performance benefits, though at the cost of increased code complexity. Careful profiling will help determine which functions are the performance bottlenecks. Remember that the most efficient solution depends on the specific task and the size of the data involved.
Leveraging Julia's Type System
Julia's powerful type system allows for compile-time optimization and code generation. Using types effectively can lead to substantial speed improvements. However, neglecting to specify types or using overly generic types can hinder performance. For example, using `Any` as a type defeats the purpose of type stability, resulting in slower execution.
Case Study 1: A function using generic types resulted in significant runtime overhead. After explicitly specifying types, the function’s performance improved drastically.
Case Study 2: A numerical computation function experienced performance degradation due to lack of type stability. By ensuring type stability, the performance increased significantly. Type stability refers to the ability of the compiler to infer the types of variables at compile time. This is essential for optimization.
Effective use of types requires understanding how Julia's type system works. Employing type annotations to specify the types of variables and function arguments is crucial for achieving type stability. Type stability allows the compiler to generate more efficient machine code. Using specialized numeric types (like `Float64` instead of `Real`) can also enhance performance. When working with large datasets, choosing appropriate data structures and types can dramatically affect efficiency. Using parametric types and traits can further refine type-based optimizations. In general, more specific types lead to better performance. However, excessively narrow types might decrease code reusability.
Advanced Profiling and Optimization Techniques
Julia provides several powerful profiling tools to identify performance bottlenecks. Simply optimizing the code without proper profiling can lead to wasted effort. Proper profiling helps to pinpoint the exact location of performance issues. Using techniques such as line profiling or call graph profiling provides detailed insights into the execution flow and identifies functions or code sections that consume significant time.
Case Study 1: Profiling revealed that a specific function was consuming the majority of runtime. Optimizing this function resulted in a substantial reduction in overall execution time.
Case Study 2: Profiling highlighted unexpected overhead in memory allocations. By addressing memory-related issues, a significant performance improvement was achieved.
Advanced optimization techniques include using loop unrolling to reduce loop overhead and vectorization to take advantage of SIMD instructions. Carefully choosing algorithms is essential for maximizing performance. Understanding memory layout and cache behavior is crucial for optimal performance. Using tools like `ProfileView.jl` or `FlameGraphs.jl` provide effective visualization of the profiling results. Using specialized packages optimized for specific hardware architectures, such as those focusing on GPU acceleration, can further improve performance for suitable tasks. Remember that the most effective optimization strategy often involves a combination of different techniques.
Conclusion
Mastering Julia's performance capabilities requires understanding its intricacies beyond the basics. By addressing memory management, parallelization strategies, efficient function selection, leveraging the type system, and employing advanced profiling techniques, developers can unlock the true power of Julia. This journey requires a combination of theoretical knowledge, practical experience, and a methodical approach to optimization. The key lies in consistently identifying bottlenecks through profiling and employing the appropriate techniques to address them. While the initial investment in learning these advanced techniques is considerable, the rewards in terms of significantly improved performance and efficiency are well worth the effort. Continued refinement and experimentation are crucial aspects of achieving optimal performance in Julia. By embracing a culture of constant performance optimization, developers can consistently improve the speed and efficiency of their Julia-based applications.