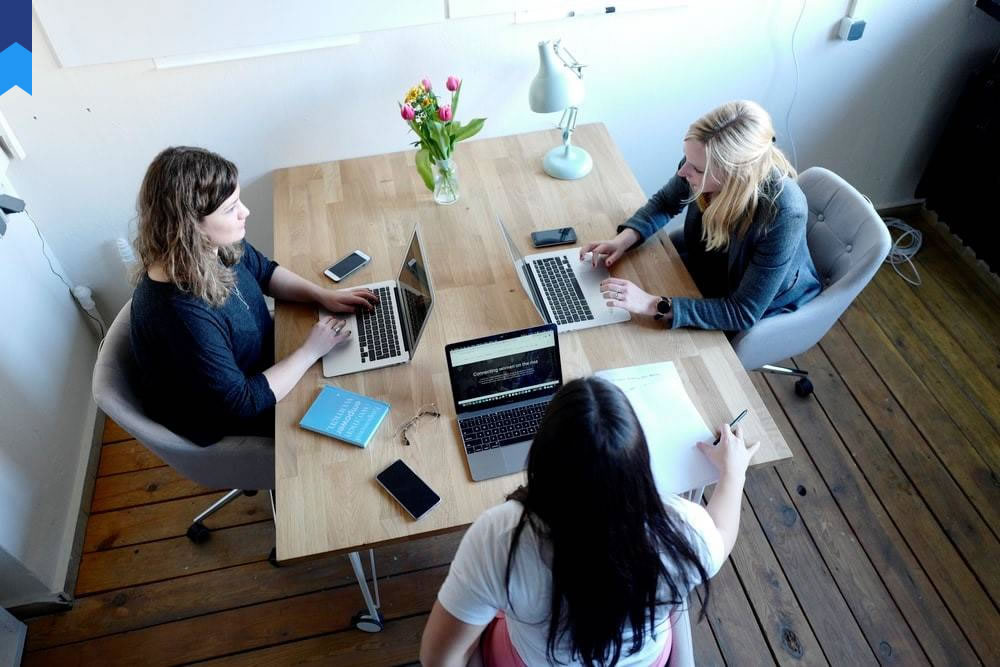
Breaking Free From Common PHP 7 Pitfalls
PHP 7, despite its maturity, still presents challenges for developers. This article delves into common mistakes, offering practical solutions and insightful strategies to elevate your PHP 7 coding skills. We'll move beyond basic tutorials, focusing on advanced techniques and problem-solving approaches that seasoned developers often overlook.
Understanding the Nuances of Object-Oriented Programming in PHP 7
Object-oriented programming (OOP) is central to modern PHP development. However, many developers struggle with its intricacies in PHP 7. Common pitfalls include misunderstanding inheritance, poorly designed class structures, and inefficient use of interfaces. A well-structured class hierarchy ensures maintainability and scalability. Consider the example of building an e-commerce system. A poorly designed structure might lead to code duplication and inconsistencies. A robust OOP approach will separate concerns clearly, for example, separating product management, order processing, and user accounts into distinct classes, each with its responsibilities. This enhances modularity, testing, and maintainability. Case Study 1: A poorly designed e-commerce system without proper OOP principles will lead to difficulties when adding new features or modifying existing functionalities. Code will become tightly coupled, increasing the risk of introducing bugs with every change. Case Study 2: Conversely, a well-designed system using inheritance, interfaces, and polymorphism will allow developers to easily adapt the system to new marketplaces, payment gateways, or product types. This adaptability is crucial for growth and competitiveness. Properly implementing traits enables code reuse, avoiding repetitive code and promoting consistency across different class structures. For instance, a logging trait could be incorporated into multiple classes for streamlined logging practices. The principle of single responsibility should guide the design. Each class should have one specific job. This reduces complexity and makes testing much easier. For instance, do not combine database interaction and business logic within a single class. Separate database operations into dedicated data access objects (DAOs) for better clarity and organization. Using abstract classes allows you to define a common interface without providing a concrete implementation. Subclasses then provide specific implementations. This promotes flexible designs and extensibility. Consider a payment gateway abstract class; subclasses can then implement specific payment processors (e.g., PayPal, Stripe).
Mastering Memory Management and Performance Optimization
PHP 7 introduced significant performance improvements, but efficient memory management remains crucial. Many performance bottlenecks stem from improper memory handling and inefficient algorithms. Using the appropriate data structures for tasks is crucial for performance. For instance, an array might be sufficient for simple tasks, but a more complex data structure like a hash table (implemented with an associative array) would be better for fast lookups. Analyzing memory usage using tools like Xdebug can pinpoint memory leaks or inefficient algorithms. Case Study 1: A poorly written loop that continuously allocates and deallocates memory can lead to noticeable slowdowns, especially when processing large datasets. Optimized loops, such as using iterators or generators, can significantly reduce memory consumption. Case Study 2: In a large-scale web application processing millions of user requests, using inappropriate data structures for caching frequently accessed data can dramatically affect performance. Utilizing efficient caching strategies (such as Redis or Memcached) alongside well-structured data structures is critical for handling high loads. Avoid memory leaks by ensuring proper object destruction. Use destructors (__destruct() method) to release resources when objects are no longer needed. Unnecessary object creation should be avoided to improve memory efficiency. For instance, if you create many small objects within a loop that are not stored, create them outside the loop. Utilizing PHP's built-in functions appropriately also helps. Sometimes, using custom-written functions is faster, but often built-in functions are more optimized and should be preferred.
Securing Your PHP 7 Applications: Best Practices
Security is paramount in any web application. PHP 7 offers enhanced security features, but developers must still implement robust security measures to protect against common vulnerabilities. Input validation is critical to prevent SQL injection and cross-site scripting (XSS) attacks. Never trust user input. Always sanitize and validate it before using it in database queries or displaying it on the page. Use parameterized queries (prepared statements) to prevent SQL injection. These queries separate data from the SQL code, preventing malicious code injection. Escape special characters in output to prevent XSS. HTML escaping is essential to render user input safely within HTML context. Proper error handling is crucial to prevent sensitive information from being revealed to attackers. Always avoid displaying detailed error messages to users. Use a robust logging system to track security-related events. Regularly update PHP, its extensions, and libraries to patch security vulnerabilities. Implement strong password policies, including mandatory complexity requirements and regular password changes. Case Study 1: A website failing to validate user input during a login process can be vulnerable to SQL injection attacks, potentially allowing attackers to gain unauthorized access to the database. Case Study 2: A forum application that does not properly escape user-submitted content in displayed posts is susceptible to XSS attacks, allowing attackers to inject malicious JavaScript code to steal user cookies or redirect users to harmful websites. Regular security audits can help identify vulnerabilities. Consider using tools such as static code analysis to detect potential security flaws. Employ output encoding to protect against vulnerabilities when interacting with external systems. Consider using security headers to enhance the security posture of the application. Use HTTPS to encrypt communication between clients and servers.
Efficient Database Interactions and Optimization Techniques
Database interactions are a frequent source of performance bottlenecks. Optimizing database queries, choosing the right database system, and efficiently handling database connections are vital for application performance. Optimize database queries to minimize execution time. Use indexes appropriately to speed up data retrieval. Avoid using SELECT *; retrieve only the necessary columns to reduce data transfer and improve performance. Choosing the right database system is dependent on the application's needs. For example, relational databases (like MySQL or PostgreSQL) are suitable for structured data, while NoSQL databases (like MongoDB) are better for unstructured or semi-structured data. Efficiently manage database connections to avoid overhead and resource depletion. Use connection pooling to reuse database connections, reducing the time and resources required to establish new connections. Case Study 1: An e-commerce site using inefficient queries to fetch product data will experience slow page loads, negatively impacting user experience. Optimizing database queries using proper indexing and carefully selecting the retrieved fields can lead to significantly faster responses. Case Study 2: A social media platform relying on a relational database for user relationships and posts may experience performance degradation as the data scales. Moving to a NoSQL database or adopting a hybrid approach can enhance performance in handling large volumes of user data. Use prepared statements to prevent SQL injection. These statements allow you to pre-compile queries, making them more efficient to execute. Transactions guarantee data integrity; multiple operations within a transaction are performed atomically. Caching frequently accessed data in memory or using a caching system can also significantly improve performance. Regularly analyze database performance using tools to identify bottlenecks and areas for optimization. Consider database normalization to minimize redundancy and maintain data integrity.
Leveraging Advanced PHP 7 Features for Enhanced Development
PHP 7 introduced several advanced features that can significantly enhance the development process. Using these features effectively can lead to cleaner, more maintainable code. Namespaces organize code into logical units, preventing naming conflicts. Anonymous functions (closures) provide flexibility and enhance code readability. Generators are efficient for handling large datasets without loading them into memory. Traits allow code reuse without the complexities of inheritance. Exceptions provide a structured way to handle errors and unexpected events. Case Study 1: In a large-scale application with numerous developers, namespaces help avoid naming collisions and maintain a more organized codebase. Case Study 2: Using generators to process large log files allows developers to process the data piece by piece, significantly reducing memory consumption. Efficiently using these features leads to more robust and manageable applications. Employing best practices from the beginning ensures scalability. Leveraging object-oriented principles enhances the overall architecture. Properly designing classes and interfaces facilitates modularity and code reuse. Using PSR standards promotes consistency across different projects. Regular code reviews are essential for catching errors and improving code quality. Using version control systems like Git is crucial for collaborative development.
Conclusion
Mastering PHP 7 requires a deep understanding of its intricacies and a commitment to best practices. By avoiding common pitfalls in object-oriented programming, memory management, security, database interactions, and by effectively utilizing advanced features, developers can create robust, scalable, and secure applications. Continuously learning and adapting to new techniques is essential for staying at the forefront of PHP development. This involves actively participating in the community, attending conferences, and engaging with online resources. A proactive approach to learning and a deep understanding of fundamental concepts will set developers on a successful path towards building high-quality PHP applications.