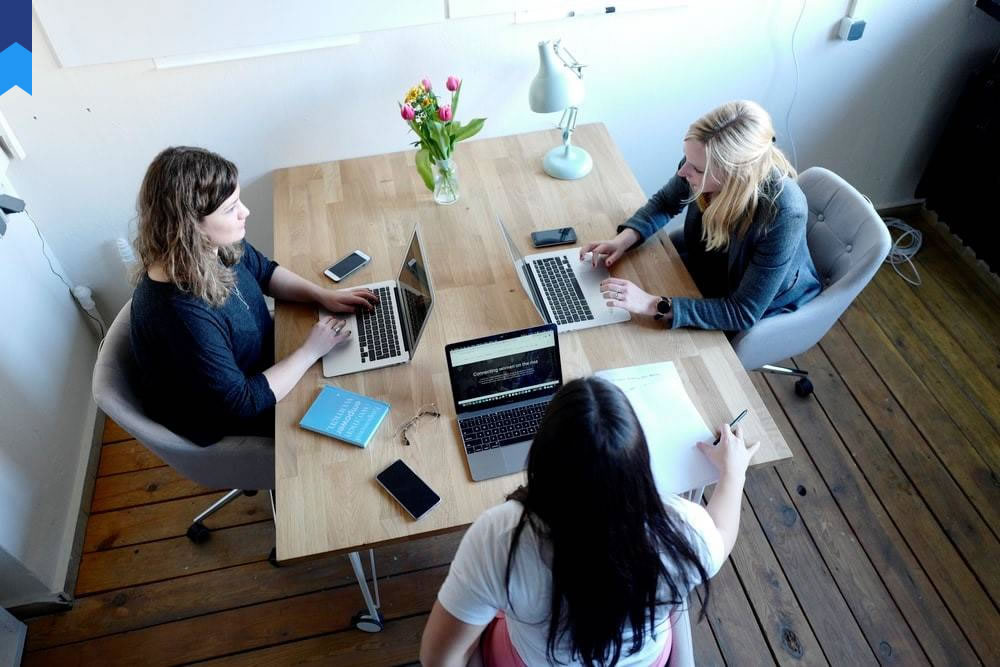
Breaking Free From Common Programming Paradigm Mistakes
Introduction: The world of programming is vast and ever-evolving. While countless resources exist to teach the fundamentals, many programmers struggle with ingrained, often subtle, mistakes that hinder efficiency, scalability, and maintainability. This article dives deep into common programming paradigm pitfalls, offering practical solutions and strategies for crafting cleaner, more robust code. We'll explore prevalent errors in different paradigms, illustrating how understanding these issues can significantly improve your programming prowess.
Imperative Programming's Hidden Traps
Imperative programming, focusing on "how" to achieve a result, is prone to certain challenges. One frequent issue is overly complex state management. As applications grow, tracking and managing the program's state becomes increasingly difficult, leading to bugs and maintenance nightmares. Consider a scenario involving multiple threads accessing shared resources without proper synchronization mechanisms. This can lead to race conditions and unpredictable behavior, resulting in data corruption or program crashes. For instance, a banking application's concurrent transaction processing requires rigorous state management to prevent inconsistencies in account balances. A well-known example of state management challenges is the development of early video games where managing the game state across multiple levels led to significant complexity and debugging challenges.
Another common pitfall is tightly coupled code. When modules are tightly intertwined, changes in one area necessitate widespread modifications, increasing development time and the risk of introducing new bugs. For example, a monolithic application with tightly integrated modules will prove challenging to modify, and the cascading impact of a single change can be enormous. Using a layered architecture or implementing design patterns like the Strategy pattern can improve the decoupling, making the code more adaptable and maintainable. One case study demonstrates the pitfalls of tight coupling. A large e-commerce platform found that changes in its payment gateway required significant modifications across multiple modules, leading to delays and a costly redesign.
Furthermore, the overuse of global variables can lead to unexpected side effects and make code difficult to understand and debug. Global variables create dependencies that are not always readily apparent, making code harder to reason about and maintain. A common illustration of this is when a function unexpectedly modifies a global variable, leading to issues in other parts of the application that rely on the original value. This problem is compounded in larger projects where multiple developers might unintentionally modify global variables. One case study shows how the overuse of global variables in a scientific simulation software led to frequent crashes and data inconsistencies due to unexpected interactions. Best practice here involves minimizing global variables, passing data explicitly between functions and using dependency injection.
Finally, neglecting error handling can result in application crashes and unexpected behavior. Imperative programs should always include robust error handling mechanisms to prevent crashes and unexpected behaviors, including logging, and exception handling. For example, a system that interacts with external services should include error handling to address potential network issues or service outages. Ignoring this can lead to the application crashing or producing unreliable results. One real-world case study demonstrates the severe impact of inadequate error handling: An airline reservation system that failed to handle network connectivity errors resulted in widespread booking failures, leading to significant financial losses and customer dissatisfaction.
Object-Oriented Programming's Common Mistakes
Object-oriented programming (OOP), while offering modularity and reusability, presents its own challenges. One prevalent mistake is designing overly complex class hierarchies. Deep inheritance hierarchies, while seemingly promoting code reuse, often lead to brittle and difficult-to-understand code. For example, having too many layers in your inheritance structures leads to difficult maintenance, testing and debugging. This problem can be significantly mitigated by employing composition instead of inheritance. For example, a car might have an engine, a transmission, and a steering system as separate objects rather than inheriting them.
Another frequent issue is the misuse of inheritance. Using inheritance solely for code reuse can lead to a tight coupling between classes, making future modifications challenging. For example, if class A inherits from class B, changes in class B will directly affect class A. A common example is trying to force inheritance relationships when there is no "is-a" relationship between objects. Favor composition to avoid such issues. One case study from the gaming industry highlights how poorly planned inheritance led to a lengthy and costly refactoring process during the development of a 3D game.
Furthermore, neglecting SOLID principles is a major source of problems. Failing to adhere to principles like the Single Responsibility Principle leads to large, unwieldy classes that are difficult to maintain and understand. Each class should ideally have a singular well-defined purpose. For example, a class responsible for user authentication, database interaction, and email sending violates the Single Responsibility Principle. Separating these functionalities into distinct classes significantly improves code organization and maintainability. One example is a banking software system where a class responsible for managing user accounts, transaction processing, and risk assessment violated the Single Responsibility Principle. Refactoring resulted in significant improvement in maintainability.
Finally, ignoring design patterns can lead to repetitive code and inefficient structures. Well-established design patterns provide proven solutions to common software development problems. Ignoring these established patterns can lead to repetitive code and inefficient structures. For example, using the Singleton pattern appropriately helps manage the creation and access to objects. The Observer pattern effectively handles notifications and updates to data. Utilizing these patterns can streamline development and enhance the quality of code. One industry case study showcased how the adoption of design patterns in a large-scale data processing system significantly improved its scalability and performance.
Functional Programming's Subtle Pitfalls
Functional programming, which emphasizes immutability and pure functions, can also present unique challenges. One common mistake is overlooking the importance of immutability. Modifying mutable data structures can lead to unexpected side effects and make debugging difficult. For example, unintentionally modifying a shared list within a function can cause unexpected results in other parts of the application. Using immutable data structures in functional programming can drastically minimize issues caused by accidental modifications. This is also critical for concurrency, as it avoids the need for complex synchronization mechanisms.
Another common issue in functional programming is the overuse of higher-order functions without careful consideration. While higher-order functions offer powerful abstraction mechanisms, their overuse can lead to code that is difficult to understand and debug. For example, deeply nested functions can make it challenging to trace the execution flow and identify potential errors. Maintaining clarity by limiting the nesting depth improves code readability and maintains ease of debugging. A case study in the finance industry revealed how poorly structured higher-order function usage in a risk modeling application hampered debugging efforts, causing significant delays in project completion.
Furthermore, neglecting error handling in functional code is a significant issue. While functional approaches often use techniques like monads to handle errors, ignoring this aspect can lead to unexpected program crashes. Robust error handling is crucial to ensure the reliability of functional programs. The use of error handling techniques, such as Try-Catch blocks in languages that support them, ensures a better user experience and less vulnerability to crashes. One case study from a large-scale machine learning project showed how overlooking error handling in functional components led to system failures during crucial model training phases.
Finally, a lack of understanding of lazy evaluation can lead to performance issues and unexpected behavior. Lazy evaluation, where expressions are evaluated only when their values are needed, can offer performance advantages. However, a poor understanding of how this mechanism works can result in unexpected behavior or performance problems. Overusing lazy evaluation in cases where immediate evaluation is necessary can impact performance. For example, if the result of a lazy evaluation is needed in real-time, the performance of the program can suffer significantly. A study of a large-scale data analysis project demonstrated how inefficient use of lazy evaluation led to slow processing speeds and user frustration.
Concurrency and Parallelism Challenges
Developing concurrent and parallel applications introduces complexities beyond those found in single-threaded programming. One frequent error is neglecting proper synchronization mechanisms when multiple threads access shared resources. Without proper synchronization, race conditions and data corruption can result. For example, two threads simultaneously updating a shared counter without locking mechanisms can lead to inaccurate results. Synchronization mechanisms like mutexes, semaphores, and monitors are critical tools that prevent these issues. A case study from a high-frequency trading platform highlights how a lack of proper synchronization led to significant financial losses due to data corruption.
Another common mistake is handling deadlocks. Deadlocks occur when two or more threads are blocked indefinitely, waiting for each other to release resources. Deadlocks can halt the entire application. Proper resource management and deadlock detection strategies are needed. For example, implementing proper locking order and using timeout mechanisms for resource acquisition can reduce the risk of deadlocks. In a database system, deadlocks involving transactions accessing shared data can lead to transaction rollbacks and overall performance degradation. A real-world case study from a financial institution shows the financial consequences of deadlocks.
Moreover, poorly designed thread pools can lead to performance bottlenecks or resource starvation. Thread pools allow efficient management of threads, but improper configuration can cause issues. For example, using a thread pool with too few threads can limit concurrency, while a pool with too many threads can consume excessive resources. Efficient thread pool management involves balancing the number of threads with system resources. A case study from a large-scale web server demonstrates how inefficient thread pool configuration led to performance bottlenecks and significant user delays.
Finally, ignoring thread safety when designing classes and methods can lead to unpredictable behavior. When multiple threads interact with non-thread-safe objects, data corruption or unexpected behavior can occur. Thread safety ensures that objects can be accessed concurrently without issues. Careful consideration of data structures and access methods is vital. A case study from a software development company shows how the lack of thread safety in a shared data structure led to numerous bugs that were difficult to detect and reproduce.
Database Interaction Pitfalls
Effective database interaction is critical for many applications. One frequent error is inefficient query design. Poorly written SQL queries can lead to significant performance problems. Optimizing queries through indexing, proper use of joins, and avoiding unnecessary operations is crucial. For example, using SELECT * instead of specifying the required columns can dramatically increase the processing time. A case study involving a large e-commerce database demonstrates how inefficient queries resulted in slow page load times and user dissatisfaction.
Another common mistake is inadequate data validation. Failing to validate data before inserting it into the database can lead to data inconsistencies and integrity issues. Implementing robust data validation on the application side as well as potentially at the database level is crucial. For example, validating the data type and length of fields helps to prevent common data integrity issues. A case study of a hospital management system illustrates how inadequate data validation led to incorrect patient records and medical errors.
Furthermore, ignoring transaction management can lead to data inconsistencies. Transactions ensure atomicity, consistency, isolation, and durability (ACID properties) of database operations. Failing to use transactions properly can result in partial updates or other data anomalies. For instance, in a banking application, a transfer between accounts should be managed as a single transaction to maintain data consistency. A case study involving an online banking platform demonstrates how insufficient transaction handling led to inconsistent account balances and financial errors.
Finally, neglecting database security can leave the application vulnerable to attacks. Protecting the database from unauthorized access and malicious attacks is critical. Using strong passwords, encryption, and access control mechanisms is vital. For example, implementing role-based access control ensures that only authorized users have the necessary permissions to access sensitive data. A case study from a financial services firm shows how the failure to implement adequate database security measures led to a data breach and significant financial losses.
Conclusion: Mastering programming paradigms requires not only understanding their theoretical concepts but also actively avoiding common pitfalls. By recognizing these frequent mistakes—in imperative, object-oriented, functional programming, concurrency and database interaction—programmers can significantly improve their code quality, efficiency, and maintainability. The focus should always be on writing clean, robust, and easily understandable code, regardless of the paradigm used. This requires consistent attention to detail, thorough testing, and a willingness to learn from mistakes.