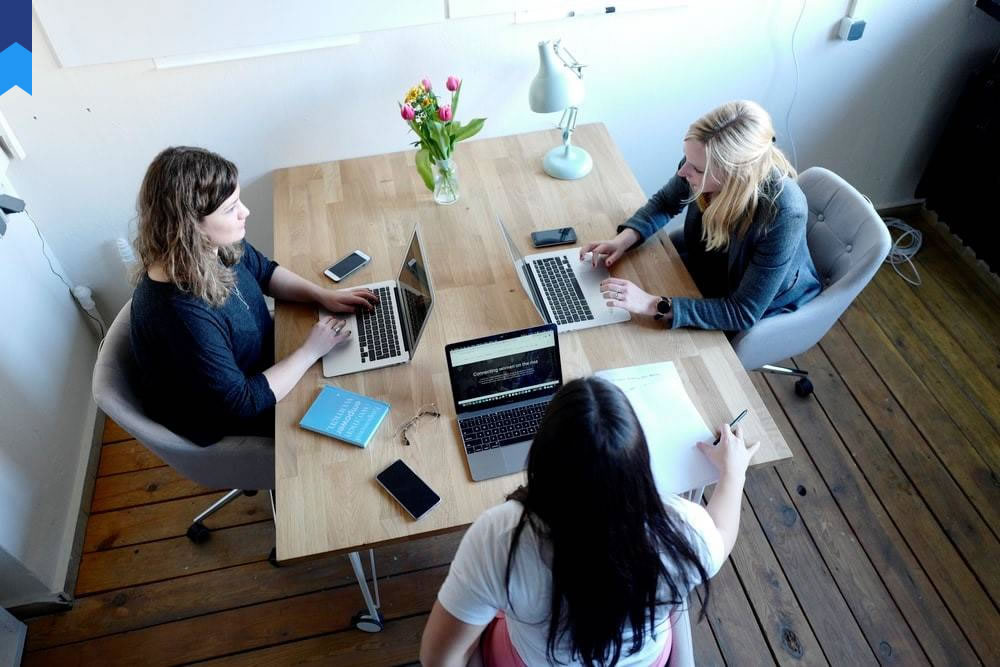
Breaking Free From Common SQL Query Mistakes
SQL, the cornerstone of relational database management, often presents challenges even for experienced developers. This article delves into common pitfalls in SQL query writing, providing practical solutions and advanced techniques to optimize performance and accuracy. We'll move beyond basic tutorials, focusing on the nuanced errors that can significantly impact database efficiency and data integrity.
Understanding Implicit Data Type Conversions
Implicit data type conversions are a frequent source of SQL errors. When comparing or operating on columns with different data types, SQL might attempt an automatic conversion, leading to unexpected results or errors. For instance, comparing a string column to a numeric column could yield incorrect comparisons, especially with leading zeros or non-numeric characters in the string. Always explicitly cast data types to avoid ambiguous behavior.
Case Study 1: Imagine a table with a 'product_code' (VARCHAR) and 'quantity' (INT) column. A query like `WHERE product_code = '00123' AND quantity > '10'` might fail or return unintended results due to string-integer comparison. Explicit casting (`WHERE CAST(product_code AS INT) = 123 AND quantity > 10`) would rectify this.
Case Study 2: A poorly designed database schema might have a column intended to store ages as a VARCHAR. Attempts to perform arithmetic on this column without casting to numeric type will fail. Explicitly casting the age column to INT before calculation is crucial for accurate results.
Explicit type casting not only improves query accuracy but also enhances readability and maintainability. By clearly specifying the intended data type for each operation, developers can anticipate and prevent subtle errors arising from implicit conversions.
The importance of explicit type casting cannot be overstated. It enhances code clarity, avoids ambiguity and protects against unexpected behavior stemming from implicit type coercion. By explicitly defining the expected data type in all operations, developers ensure consistency and reliability in their SQL queries. This best practice should be diligently followed in all database interactions. Furthermore, static code analysis tools can help identify potential implicit type conversion issues before they lead to runtime errors. Adopting a robust approach to data type management is essential for building scalable and maintainable database applications.
Proper data type handling is integral to data integrity and efficient query execution. Overlooking data type compatibility can lead to unexpected results, performance bottlenecks and maintainability issues. Employing best practices, including explicit casting and schema design that prioritizes type consistency, forms the foundation of robust and reliable SQL applications.
The Perils of Inefficient JOINs
JOIN operations are fundamental to relational databases, but inefficient JOINs can cripple performance. Using incorrect JOIN types or failing to optimize JOIN conditions can lead to significant slowdowns, particularly in large datasets. For example, a full table scan in a poorly constructed JOIN can be incredibly resource-intensive. Understanding the different JOIN types (INNER, LEFT, RIGHT, FULL OUTER) and selecting the appropriate one for your query is critical.
Case Study 1: A company managing customer orders might use a `LEFT JOIN` between the 'orders' and 'customers' tables to display all orders, including those with missing customer information. An incorrect `INNER JOIN` would exclude such orders.
Case Study 2: In an e-commerce application, joining products, categories, and inventory tables might involve multiple JOINs. Optimizing the order of JOINs and adding appropriate indexes can dramatically reduce execution time. Poorly ordered joins can exponentially increase query execution time.
Efficient JOINs require careful consideration of indexing strategies. Indexes accelerate data retrieval by allowing the database to quickly locate matching rows. Creating indexes on frequently joined columns significantly improves JOIN performance. The choice of index type (B-tree, hash, etc.) depends on the specific query pattern and data characteristics.
Beyond index selection, analyzing query plans provides crucial insights into query efficiency. Database systems offer tools to examine query execution plans, identifying bottlenecks and areas for optimization. By understanding the steps involved in query execution, developers can fine-tune their JOINs for optimal performance. This might involve rewriting queries to avoid unnecessary table scans or Cartesian products.
Database optimization is a continuous process that necessitates regular monitoring and analysis. Tools that visualize query execution plans offer critical feedback for identifying and eliminating performance bottlenecks. By continuously assessing and refining query performance, developers maintain optimal database efficiency.
Failing to Utilize Indexes Effectively
Indexes are crucial for efficient data retrieval. Without proper indexing, SQL queries may resort to full table scans, resulting in significantly slower performance. A carefully chosen indexing strategy can dramatically speed up queries, particularly those involving WHERE clauses, ORDER BY clauses, and JOINs.
Case Study 1: Consider a table storing millions of customer records. A query to find customers based on their location would be drastically slower without an index on the location column. Adding an index on 'location' allows the database to quickly pinpoint relevant records without scanning the entire table.
Case Study 2: In an e-commerce system, indexing the 'product_id' and 'category_id' columns would significantly improve the performance of queries retrieving products by category. This avoids the need to scan every product record.
The decision of which columns to index depends heavily on the query patterns in the application. Analyzing query logs helps identify commonly used search criteria, guiding the choice of indexed columns. Over-indexing, however, can also hurt performance. Too many indexes can slow down data modification operations (inserts, updates, deletes) as the database must update all affected indexes.
Understanding index types and their respective trade-offs is crucial. Different index types (B-tree, hash, full-text, etc.) offer varying performance characteristics depending on data types and query patterns. The choice of index type should align with specific application requirements and query characteristics. The proper use of indexes improves query speeds, reducing database load times and enhancing the overall user experience.
Neglecting Error Handling and Transactions
Robust error handling and transaction management are essential for data integrity and application reliability. Ignoring these aspects can lead to data corruption, inconsistencies, and application crashes. Using try-catch blocks or similar mechanisms allows for graceful handling of potential errors, preventing application failures and preserving data consistency.
Case Study 1: Consider a banking application where multiple transactions update account balances. Failure to manage transactions appropriately could lead to inconsistencies in the balances if one transaction fails midway. Transactions safeguard data against partial updates.
Case Study 2: An e-commerce site processing orders should employ transactions to ensure that the order, payment, and inventory updates happen atomically. If any part fails, the entire transaction rolls back, maintaining data consistency. This prevents partial updates leading to discrepancies.
Transactions provide ACID (Atomicity, Consistency, Isolation, Durability) properties, guaranteeing data reliability and integrity. Atomicity ensures that all operations within a transaction either complete successfully or fail entirely. Consistency ensures that the database remains in a valid state before and after a transaction.
Proper error handling is equally important to prevent unexpected application behavior. Comprehensive error handling mechanisms enable applications to gracefully handle unexpected situations, preventing unexpected termination and data inconsistencies. Strategies such as try-catch blocks or custom error handling routines improve application robustness and resilience.
Choosing the correct level of transaction isolation is also crucial. Different isolation levels (read uncommitted, read committed, repeatable read, serializable) control concurrency and prevent data inconsistencies. Careful selection of the isolation level is necessary to balance performance with data integrity requirements.
Overlooking SQL Optimization Techniques
SQL queries can be optimized in numerous ways to improve performance. Simple changes in query structure, the use of appropriate aggregate functions, and the avoidance of unnecessary computations can yield significant performance gains. Techniques include optimizing subqueries, minimizing data returned, and utilizing appropriate indexing.
Case Study 1: Using correlated subqueries can often be less efficient than using joins or set-based operations. Rewriting a query to use joins will result in a more optimal execution plan.
Case Study 2: Selecting only necessary columns rather than using SELECT * minimizes the amount of data transferred and processed. This simple optimization leads to faster query execution.
Careful analysis of the query execution plan (often available through database-specific tools) can identify potential bottlenecks. Tools like EXPLAIN PLAN in Oracle or similar features in other database systems help analyze query execution, leading to targeted optimizations. Examining the execution plan provides a detailed view of how the database processes the query, revealing potential areas for improvement.
Regularly reviewing and optimizing queries is crucial to maintaining database performance, especially as data volumes grow. Periodic performance testing and query analysis allows for the identification and rectification of performance bottlenecks. This prevents slowdowns and ensures efficient database operations.
Database optimization is an ongoing process. Regular monitoring, performance testing, and query analysis are essential for maintaining optimal database performance and scalability. Proactive optimization prevents slowdowns and ensures efficient operation as data volumes grow over time. Ignoring SQL optimization best practices can significantly affect system response times. Prioritizing optimization strategies ensures high-performance databases supporting efficient operations.
Conclusion
Mastering SQL requires more than just understanding basic syntax. Addressing these common pitfalls—implicit data type conversions, inefficient joins, ineffective indexing, poor error handling and transaction management, and overlooking optimization techniques—is crucial for building robust and high-performing database applications. By diligently applying the principles and techniques outlined in this article, developers can significantly improve the efficiency, reliability, and scalability of their SQL-based systems.
The journey to becoming a proficient SQL developer involves continuous learning and the adoption of best practices. By proactively addressing these challenges and embracing optimization strategies, developers ensure their applications remain efficient, reliable and easily maintainable as data volumes grow and application complexity increases. The long-term benefits far outweigh the initial investment in mastering these critical aspects of SQL development.