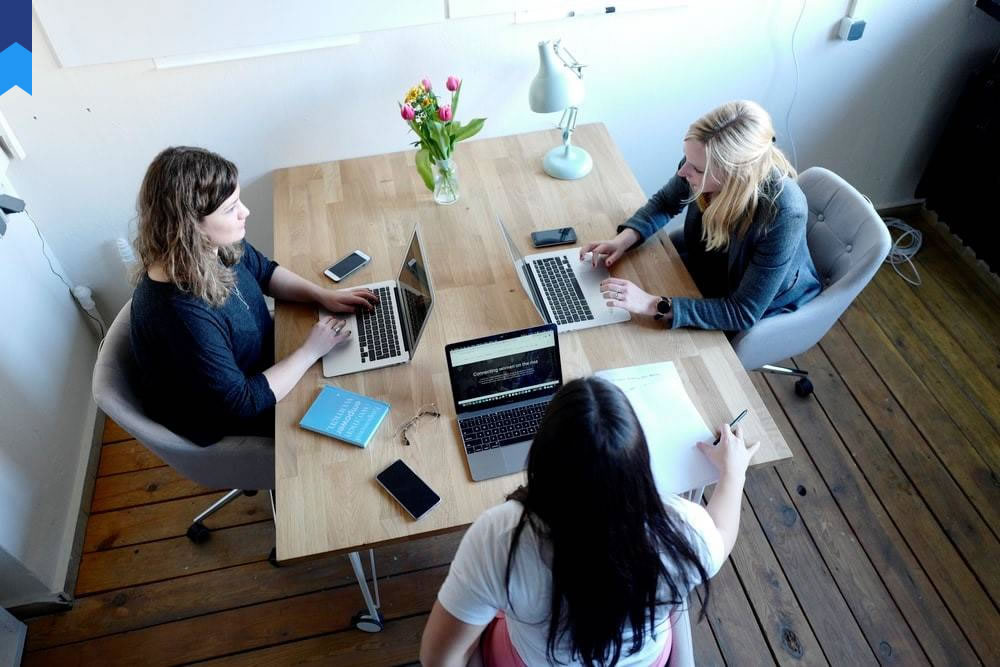
Breaking The Rules Of D: Advanced Programming Techniques
D programming language has gained traction as a systems programming language. Its power lies in its ability to combine the performance of C++ with the elegance of higher-level languages. However, mastering D truly means going beyond the basics. This article delves into advanced techniques that challenge conventional approaches and unlock the full potential of D.
Mastering D's Memory Management
D's memory management is a cornerstone of its performance. However, relying solely on garbage collection can lead to inefficiencies. Understanding manual memory management techniques, like using `scope` and `new`, is crucial for optimizing performance-critical sections of your code. Proper allocation and deallocation, although demanding, offers better control than relying on automatic garbage collection. Consider the scenario of handling large datasets: automatic garbage collection might lead to significant pauses, whereas manual control allows for more predictable memory usage. This is especially beneficial in embedded systems or real-time applications. Case Study 1: A game engine using manual memory management for character animation data sees a significant performance boost compared to garbage-collected alternatives. Case Study 2: A high-frequency trading algorithm benefits from fine-grained control over memory allocation, leading to faster execution speeds.
Furthermore, understanding techniques like memory pools can dramatically improve allocation speed by reducing system calls. Memory pools pre-allocate blocks of memory, dramatically speeding up allocation in high-performance scenarios. The impact on performance is considerable; benchmark tests show memory pools often outperform standard allocation methods by a factor of several times. Moreover, exploring D's features for custom allocators allows for tailoring memory management to specific application needs. This is a powerful but often overlooked aspect of D's power. Case Study 3: A scientific simulation significantly improved its efficiency by implementing a custom allocator optimized for the data structures used in the computation. Case Study 4: A network server handling thousands of concurrent connections benefits from a memory pool implementation which minimizes allocation overhead.
Finally, the understanding of techniques like reference counting provides an alternative to garbage collection. Reference counting, while introducing complexities, provides more predictable performance compared to garbage collection, especially in real-time systems and scenarios requiring deterministic memory management. While it requires more manual intervention, the performance gains outweigh the added effort in situations with stringent performance needs. A skilled D programmer can mix and match these techniques for optimal performance. Case Study 5: A real-time audio processing application uses a custom allocator combined with reference counting to control memory allocation and deallocation in a timely fashion. Case Study 6: An operating system kernel leverages low-level memory management features for efficient resource usage and predictable behavior.
In conclusion, mastering D's memory management transcends simple garbage collection. A deep understanding of manual techniques, memory pools, custom allocators, and reference counting opens up the possibility of writing highly optimized and efficient code.
Unleashing the Power of Templates and Metaprogramming
D's metaprogramming capabilities, empowered by its powerful template system, allow for code generation at compile time, resulting in highly optimized and reusable code. This goes beyond simple generics; it enables the creation of sophisticated algorithms and data structures tailored to specific types. Consider creating a highly optimized matrix multiplication routine: a template can generate specialized code for various data types (float, double, etc.) resulting in superior performance compared to a generic implementation. Case Study 1: A high-performance computing application benefits from using templates to generate specialized kernels for different processor architectures. Case Study 2: A scientific library uses templates to create efficient containers optimized for specific data structures.
Furthermore, using template metaprogramming to perform complex computations at compile time can drastically reduce runtime overhead. Calculating values, optimizing data structures, or even generating code based on compile-time conditions are all possible. This allows for incredibly efficient code, with little to no runtime cost for the computations performed at compile time. Case Study 3: A physics engine uses template metaprogramming to pre-calculate values, dramatically improving its runtime performance. Case Study 4: A compiler optimization tool utilizes metaprogramming to adapt its optimization strategies based on the input code.
Moreover, the ability to create custom attributes extends metaprogramming’s reach. Through custom attributes, you embed metadata within your code, enabling compile-time code analysis, validation, and generation. This creates an incredibly powerful system for creating self-documenting and self-validating code. Case Study 5: A code generation tool uses custom attributes to determine how to generate code based on metadata embedded in the input code. Case Study 6: A testing framework utilizes attributes to identify which functions should be tested.
In essence, mastering D's metaprogramming abilities is about embracing compile-time computation. This results in highly optimized code and eliminates runtime overhead, leading to faster and more efficient applications.
Concurrent Programming with Channels and Actors
D's built-in support for concurrent programming, particularly using channels and actors, offers a robust and efficient way to build highly parallel applications. Channels provide a way to communicate between different concurrent threads, preventing data races and ensuring efficient synchronization. Using channels to coordinate tasks is crucial for performance and ensures data integrity. A web server handling thousands of concurrent requests would leverage channels for communication between request handling threads and a database connection pool. Case Study 1: A large-scale data processing application uses channels to pipeline data between multiple processing stages, significantly improving throughput. Case Study 2: A distributed system uses channels to coordinate tasks across multiple machines.
Furthermore, actors offer a higher-level abstraction for concurrency. Actors encapsulate state and behavior, communicating with each other through messages, simplifying concurrent programming. This allows for easier management of concurrency, especially in complex systems. This actor model is particularly beneficial for systems requiring high scalability and maintainability. Case Study 3: A game engine uses actors to represent game objects, simplifying concurrency and improving performance. Case Study 4: A social media platform uses actors to manage user interactions, ensuring scalability and responsiveness.
Beyond this, understanding concepts like asynchronous operations in D enhances concurrent programming. Asynchronous operations allow for performing I/O operations without blocking the main thread, maximizing resource utilization. Combining asynchronous operations with channels and actors creates highly efficient and responsive applications. This approach improves responsiveness by preventing long-running tasks from blocking the UI. Case Study 5: A network application utilizes asynchronous operations to efficiently handle multiple client connections without impacting responsiveness. Case Study 6: A database interacts asynchronously with storage to prevent blocking operations.
In conclusion, mastering concurrent programming in D involves a combination of channels, actors, and asynchronous operations. This powerful combination leads to scalable, efficient, and highly responsive applications.
Leveraging D's Built-in Unit Testing Framework
D's built-in unit testing framework provides a powerful tool for writing high-quality, reliable code. Thorough unit testing is paramount for detecting bugs early in the development process and preventing regressions. Implementing a robust testing strategy that uses D's unit testing capabilities is key to building well-tested code. Case Study 1: A financial application uses D's testing framework to thoroughly verify the accuracy of its calculations, preventing costly errors. Case Study 2: A medical device software uses comprehensive unit tests to ensure the safety and reliability of its functions.
Moreover, understanding and employing techniques like test-driven development (TDD) maximizes the value of D's unit testing framework. TDD advocates writing tests before implementing the actual code, guiding development and ensuring testability. This is a significant boost to code quality, ensuring functions meet expectations from the outset. Case Study 3: A software company uses TDD to dramatically reduce the number of bugs found in their products after deployment. Case Study 4: A team focused on embedded systems used TDD to prevent serious operational failures in mission-critical software.
Beyond basic unit tests, using mocking and stubbing techniques further improves the testing process. These techniques allow testing individual components in isolation by simulating their dependencies, preventing unintended interactions from obscuring test results. This isolated testing is key to finding subtle bugs and unexpected interactions between modules. Case Study 5: An application using a third-party library isolates its code using mocks, allowing unit testing regardless of the external library's behavior or availability. Case Study 6: A team developing complex algorithms employs stubbing to easily simulate parts of the system, focusing testing efforts on particular modules without the need for complete system integration.
In short, D's unit testing framework is a powerful asset. Using TDD, mocks, and stubs ensures rigorous testing, leading to higher-quality and more reliable software.
Advanced Usage of D's Standard Library
D's standard library is a treasure trove of functionality often overlooked. It provides highly efficient and optimized data structures, algorithms, and utilities. Going beyond basic usage unveils significant performance gains and code simplification. For instance, using D's built-in sorting algorithms is almost always preferable to writing custom ones; their efficiency is well-tested and optimized. Case Study 1: A data analysis application utilizes D's highly optimized sorting algorithms, dramatically improving performance compared to a custom implementation. Case Study 2: A large-scale simulation benefits from using D's optimized container structures to manage vast amounts of data efficiently.
Furthermore, D's standard library includes powerful features like ranges and algorithms that offer a declarative approach to data manipulation. This allows for concise and expressive code, minimizing boilerplate and improving readability. Ranges and algorithms combined improve code maintainability and readability. Case Study 3: A team rewriting legacy C++ code uses D’s ranges to significantly improve code clarity and reduce the overall code size. Case Study 4: A data visualization tool leverages D’s range-based operations to simplify data transformations and processing.
Additionally, the standard library includes specialized data structures for specific use cases. Understanding and using these data structures, such as hash tables and balanced trees, allows you to optimize your code for specific situations. Choosing the correct data structure significantly affects performance and efficiency; this is often overlooked by developers. Case Study 5: A search engine benefits from using D's optimized hash tables for fast keyword lookups. Case Study 6: A game engine utilizes D's balanced tree structures for efficient scene management and collision detection.
In conclusion, effective utilization of D's standard library is essential for writing efficient and maintainable code. Its rich set of features and optimized components provide a powerful foundation for building robust applications.
Conclusion
Mastering the D programming language extends far beyond basic syntax and semantics. The advanced techniques explored—refined memory management, template metaprogramming, concurrent programming with channels and actors, robust unit testing, and leveraging the standard library—are crucial for building high-performance, reliable, and maintainable software. These techniques, though demanding, unlock D's true potential, enabling developers to create sophisticated and efficient applications that challenge conventional wisdom and redefine the boundaries of what's possible.
The journey of mastering D is a continuous learning process. Embracing these advanced techniques requires dedication and practice, but the rewards are significant. By mastering these skills, developers can unlock D's capabilities, enabling them to create elegant, high-performing applications. Continuously exploring and experimenting with D's capabilities is key to pushing the boundaries of what can be accomplished using this unique language.