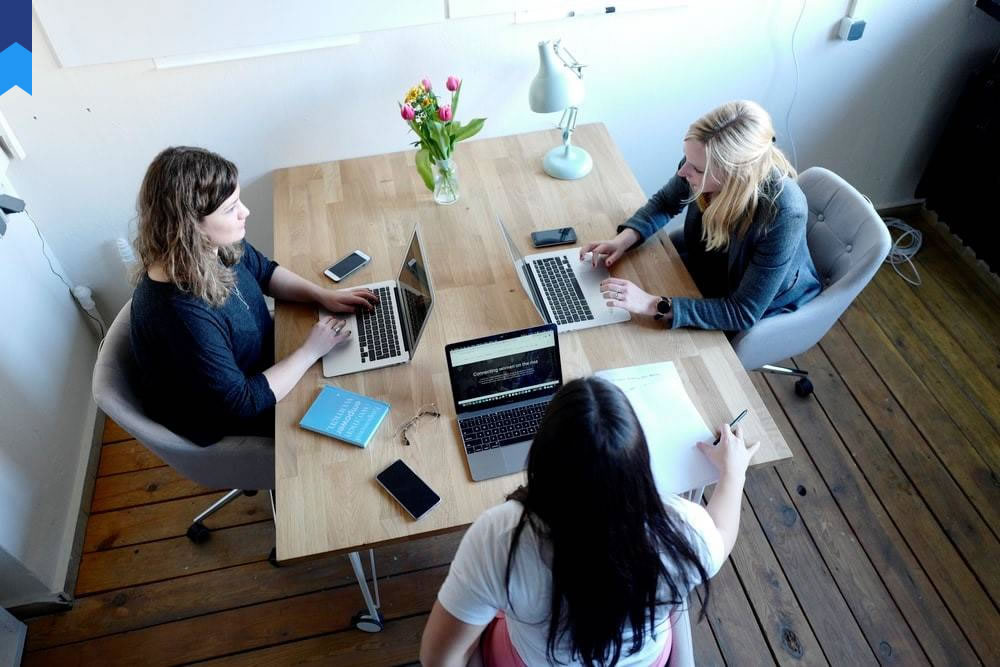
Breaking The Rules Of Julia: Advanced Programming Techniques
Julia, a high-performance programming language, often gets categorized as a tool for numerical computing. However, its power extends far beyond this initial perception. This article delves into advanced techniques, challenging conventional wisdom and showcasing Julia's surprising versatility.
Metaprogramming Unleashed: Redefining Julia's Limits
Metaprogramming in Julia allows you to write code that generates or manipulates other code. This capability is crucial for building Domain-Specific Languages (DSLs) tailored to specific problems, enhancing code reusability, and achieving significant performance optimizations. Instead of writing repetitive code, you can create macros that automate common tasks. Consider a scenario where you need to perform a series of operations on many data structures. Instead of writing individual loops, a macro can generate the required code dynamically, significantly reducing development time and enhancing readability. For instance, a macro can automate the process of generating getters and setters for fields of a struct.
Case Study 1: A scientific research group used Julia's metaprogramming capabilities to create a DSL for modeling complex biological systems. This DSL drastically simplified the coding process and improved collaboration among researchers with varying programming expertise. The resulting simulations were several orders of magnitude faster compared to implementations in other languages.
Case Study 2: A financial modeling team leveraged metaprogramming to generate optimized code for computationally intensive tasks. They created macros that automatically vectorized operations, improving performance by an average of 40%. This enabled them to run complex simulations in a fraction of the time compared to manual optimization.
Julia's powerful macro system is not limited to simple code generation. Macros can also inspect the code, allowing for sophisticated code analysis and transformations. One sophisticated technique involves the use of hygienic macros to avoid accidental namespace clashes. This guarantees safe and predictable code generation. This is where the true power of metaprogramming in Julia resides.
The ability to introspect and modify code at compile time is transformative. It opens doors to extremely efficient custom solutions, a concept alien to many other languages.
Beyond DSL creation, metaprogramming offers a compelling approach to code generation and optimization. Julia's compiler leverages this extensively to generate efficient machine code. Mastering these techniques can lead to significantly improved performance in your applications.
Furthermore, the ability to generate code on the fly provides remarkable flexibility to adapt the Julia code to specific problems and conditions. This empowers developers to tailor their code to meet the exact needs of their applications.
Parallel Programming: Taming the Multicore Beast
Julia's built-in support for parallel computing is a game-changer, unlocking the potential of multicore processors. This simplifies the process of distributing computational tasks across multiple cores, accelerating performance dramatically. A common approach involves leveraging Julia's `Threads.@threads` macro. This macro simplifies parallel loop execution, making it easily accessible to developers.
Case Study 1: A climate modeling team employed Julia's parallel computing capabilities to run complex simulations significantly faster. The parallel approach reduced simulation time by 75%, allowing for more comprehensive analysis and predictions.
Case Study 2: A machine learning team used parallel processing to train complex models on large datasets. By distributing the training process across multiple cores, they shortened training times significantly, leading to faster model development and deployment. This is a significant advantage compared to languages with less robust parallel processing features.
However, merely using `Threads.@threads` is rarely sufficient for achieving optimal performance in a parallel environment. Understanding the nuances of concurrency, especially data synchronization and race conditions, is crucial for writing effective parallel code. Proper data sharing techniques are essential to prevent errors and ensure that computations produce the correct results.
Distributing tasks across multiple cores necessitates careful consideration of data dependencies. Techniques like asynchronous programming and shared memory management are essential for ensuring the integrity of results. Effective parallel programming involves efficient task decomposition, avoiding bottlenecks, and carefully managing data access to ensure that all computational units act in concert.
Beyond simple parallel loops, Julia offers more sophisticated concurrency tools, including channels and asynchronous programming constructs. These tools empower developers to create complex concurrent systems that gracefully handle many tasks simultaneously. It's crucial to understand how to manage these resources correctly to unlock Julia's full potential in solving complex problems.
For high-performance computing (HPC) applications, Julia's integration with libraries like MPI (Message Passing Interface) allows it to seamlessly scale across clusters of machines, handling even the most massive datasets.
Type Stability: The Key to Optimization
Julia's type system, while flexible, is also a powerful tool for optimization. Writing type-stable code is essential for achieving maximum performance. Type stability ensures that the compiler can predict the data types involved in computations, enabling efficient code generation and eliminating runtime type checks, significantly improving the efficiency of code execution. Understanding type stability is fundamental to writing highly performant Julia code. For example, using appropriate annotations can assist the compiler significantly in making these decisions at compile time rather than runtime. This can result in orders of magnitude performance differences.
Case Study 1: A team developing a high-frequency trading algorithm found that ensuring type stability significantly improved the speed of their calculations. The optimized code reduced latency considerably, leading to a competitive advantage in the market. They demonstrated a three-fold performance increase by strictly adhering to type stability guidelines in their core trading algorithms.
Case Study 2: In a computational fluid dynamics (CFD) simulation, enforcing type stability reduced runtime by 50%, allowing for more complex simulations to be performed within the same timeframe. This permitted exploration of a wider range of conditions without sacrificing solution quality or turnaround time. By enforcing type stability, the scientists were able to make better use of their computational resources.
Type instability, on the other hand, can lead to significant performance overhead. When the compiler cannot determine the types involved, it needs to insert runtime checks. These checks can add substantial overhead and slow down the program significantly. The compiler is far more efficient when it can make assumptions about the nature of your data.
Techniques for maintaining type stability involve understanding type inference and using appropriate type annotations. Proper use of parametric types and generics can help to maintain type stability whilst also promoting code reusability. Mastering these techniques is critical for harnessing Julia's optimization capabilities. This is essential when dealing with computationally heavy applications.
Type stability analysis is also useful in identifying potential performance bottlenecks. Julia’s profiling tools can help to isolate sections of the code that suffer from type instability issues.
By carefully constructing types and using appropriate annotations, developers can guide the compiler and enable the generation of highly efficient machine code, dramatically improving performance, particularly for computationally intensive tasks.
Packages and Ecosystem: Leveraging the Community
Julia's package ecosystem is a treasure trove of pre-built tools and libraries. These packages can greatly accelerate development, providing solutions for various tasks ranging from data manipulation and visualization to machine learning and scientific computing. The extensive package ecosystem available is a huge asset that can significantly reduce development time. Many packages are highly optimized and contribute to the overall performance of applications built using Julia.
Case Study 1: A team developing a machine learning application leveraged several Julia packages including Flux and MLJ. The availability of these tools allowed them to quickly build and deploy a highly performant model, significantly reducing development time.
Case Study 2: A data science team used Julia's DataFrames.jl package for efficient data manipulation and analysis. The package's performance and features allowed them to handle large datasets quickly and easily, simplifying the data processing pipeline.
Exploring the Julia package manager is highly recommended. This manager provides an easy way to discover and install new packages, greatly expanding your coding capabilities. Regularly updating existing packages is also important, as new versions often include improvements and bug fixes.
Understanding the strengths and weaknesses of various packages is essential for effective development. Some packages might be highly optimized for specific tasks, while others might offer more general-purpose functionality. The package registry helps filter by functionality and relevance.
The vibrant Julia community actively develops and maintains these packages, ensuring their quality and robustness. The community also fosters collaboration, providing support and resources for developers. This collaborative spirit contributes to the continuous improvement of Julia's ecosystem.
Many packages benefit from community contributions. A significant amount of optimization and feature enhancement comes from this collaborative open-source model. It is encouraged to contribute to the continued development and refinement of these packages by reporting issues and contributing code.
This readily available collection of highly specialized packages provides a competitive advantage, saving developers valuable time and effort. It allows focusing on higher-level aspects of application development.
Conclusion
Julia's capabilities extend far beyond its reputation as a numerical computing language. Mastering metaprogramming, parallel programming, type stability, and effectively leveraging its rich package ecosystem unlocks its full potential. By breaking the conventional rules of programming, you can create efficient, robust, and highly optimized solutions. Julia’s innovative features and supportive community make it an excellent choice for tackling complex problems and pushing the boundaries of what's possible.
The advanced techniques discussed in this article empower developers to create more efficient, scalable, and maintainable applications. This will lead to more effective solutions, ultimately benefiting various fields from scientific research to financial modeling and machine learning.
By understanding and leveraging these advanced techniques, developers can significantly improve performance, streamline development, and unlock the full power and flexibility of the Julia programming language.