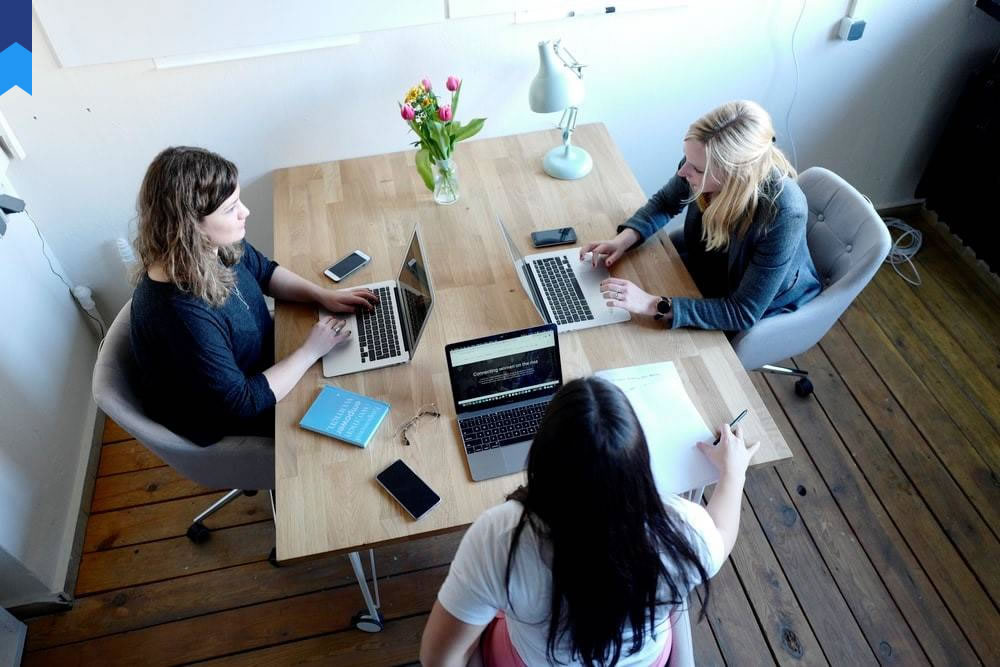
Conquer Algorithmic Complexity: Five Strategic Design & Analysis Approaches
Introduction: The design and analysis of algorithms is a cornerstone of computer science, impacting everything from search engines to medical diagnostics. However, the inherent complexity of many problems can be daunting. This article presents five strategic approaches to conquer algorithmic complexity, moving beyond basic overviews and delving into practical, innovative techniques. We'll explore strategies that challenge conventional wisdom, offering unexpected angles to tackle efficiency and scalability challenges.
Mastering Divide and Conquer
Divide and conquer is a classic algorithmic paradigm that breaks down a problem into smaller, self-similar subproblems, solves them recursively, and then combines the solutions to obtain the overall solution. This approach is particularly effective for problems that exhibit inherent recursive structure, such as sorting and searching. A prime example is merge sort, which recursively divides an unsorted list into smaller sublists until each sublist contains only one element. These single-element sublists are inherently sorted. The algorithm then repeatedly merges these sublists to produce larger sorted sublists until a single sorted list is obtained. The efficiency stems from the logarithmic reduction in problem size with each recursive step, leading to a time complexity of O(n log n). Another powerful application is the fast Fourier transform (FFT), crucial in signal processing and image analysis, demonstrating the power of divide and conquer in tackling complex data transformations.
Case Study 1: Netflix uses divide and conquer in its recommendation algorithm. The massive dataset of user preferences is broken down into smaller, manageable chunks to process recommendations efficiently. Case Study 2: In computational biology, divide and conquer is used for sequence alignment, where long DNA sequences are broken down for comparison, improving processing time drastically.
The strategy's efficacy hinges on the efficient design of the "divide" and "combine" steps. Inefficient division or combination can negate the benefits of the recursive approach. The choice of subproblem size also impacts performance significantly. Understanding these nuances is crucial for optimal results.
Furthermore, divide and conquer algorithms can be challenging to implement and debug, particularly for complex problems. The recursive nature can lead to stack overflow errors if not carefully handled. Careful attention to base cases and recursive calls is paramount. Finally, the overhead of dividing and combining subproblems might outweigh the gains for smaller problem instances.
The choice of subproblem size also impacts performance. Too small, and the overhead dominates; too large, and the benefits of recursion are lost. Optimal subproblem sizing often requires careful analysis and experimentation. For instance, in merge sort, the choice of splitting the list into two roughly equal halves is critical for maintaining the O(n log n) time complexity.
Dynamic Programming: Optimizing Overlap
Dynamic programming tackles problems by breaking them into overlapping subproblems, solving each subproblem only once, and storing the solutions to avoid redundant computations. This approach is particularly effective for optimization problems where the optimal solution can be constructed from optimal solutions to subproblems. Consider the classic knapsack problem, where the goal is to maximize the value of items placed in a knapsack with a weight constraint. Dynamic programming allows us to efficiently explore the space of possible combinations without redundant calculations, leading to a significantly faster solution than brute-force approaches.
Case Study 1: In route planning applications, like Google Maps, dynamic programming efficiently calculates the shortest paths between locations by breaking the route into smaller segments and reusing previously computed shortest paths between intermediate points. Case Study 2: Bioinformatics employs dynamic programming extensively for sequence alignment, where it efficiently finds the optimal alignment of two or more biological sequences.
The core of dynamic programming lies in identifying the overlapping subproblems and constructing a recursive relation that defines the optimal solution in terms of the optimal solutions to subproblems. Efficient storage mechanisms, like memoization or tabulation, are crucial for avoiding redundant calculations.
While powerful, dynamic programming can be memory-intensive, especially for problems with a large number of subproblems. The space complexity can be significant, and careful consideration of memory usage is necessary. Moreover, formulating the recursive relation can be challenging for complex problems, requiring a deep understanding of the problem's structure. Incorrect formulation leads to incorrect results, emphasizing the importance of careful problem analysis.
The design and implementation of dynamic programming algorithms often involve trade-offs between time and space complexity. For example, tabulation can lead to lower time complexity but higher space complexity compared to memoization. Selecting the appropriate approach requires careful consideration of the problem's specific constraints and resources.
Greedy Algorithms: Local Optimality
Greedy algorithms make locally optimal choices at each step, hoping to find a global optimum. They are often simpler and faster than dynamic programming or divide and conquer, but they don't guarantee the best solution. Consider Dijkstra's algorithm for finding the shortest path in a graph. At each step, it selects the node with the smallest distance from the source, extending the path greedily. While not always optimal for all graph types, Dijkstra's algorithm provides a very efficient solution for many practical scenarios. Another example is Huffman coding, used for data compression. It builds a binary tree greedily, assigning shorter codes to more frequent symbols, resulting in efficient compression.
Case Study 1: In network routing protocols, greedy algorithms are employed to select the best path for data packets, aiming for the quickest route. Case Study 2: In scheduling problems, greedy algorithms are often used to assign tasks to processors, prioritizing those with the shortest execution times.
Greedy algorithms are appealing due to their simplicity and efficiency. Their linear time complexity makes them attractive for large datasets. However, their primary drawback is that they don't always find the optimal solution. This lack of optimality is a significant limitation, especially when the global optimum is crucial.
The effectiveness of a greedy algorithm depends heavily on the problem's structure and the choice of greedy criterion. A poorly chosen criterion can lead to suboptimal, or even completely incorrect, solutions. Careful analysis of the problem and a thorough understanding of the greedy strategy are crucial for successful application. Moreover, proving the correctness or finding the limitations of a greedy algorithm can be mathematically challenging.
When applying greedy algorithms, it's crucial to analyze the problem's properties and carefully select the greedy choice. Incorrect choice leads to suboptimal results. It's essential to understand the trade-off between simplicity and optimality before employing this approach. Rigorous testing and validation are essential to ensure reliability.
Backtracking: Exploring Solution Space
Backtracking systematically explores the solution space by building candidate solutions incrementally. If a partial solution leads to an infeasible or non-optimal solution, it backtracks to a previous state and tries a different choice. The N-Queens problem, where the goal is to place N chess queens on an N×N chessboard such that no two queens attack each other, is a classic example where backtracking provides an efficient solution. Similarly, finding all permutations of a set can be efficiently solved using backtracking. This approach exhaustively explores all possibilities within a defined structure.
Case Study 1: Constraint satisfaction problems, commonly used in artificial intelligence, employ backtracking to find solutions that satisfy a set of constraints. Case Study 2: In graph theory, finding Hamiltonian cycles (cycles that visit each vertex exactly once) often utilizes backtracking.
Backtracking algorithms are relatively straightforward to implement, often relying on recursive functions to explore the solution space. However, their efficiency can be significantly impacted by the size of the search space. For problems with a large solution space, backtracking can become computationally expensive. Careful design and optimization techniques are crucial to manage this complexity.
The performance of backtracking is heavily dependent on the order in which candidate solutions are explored. Strategic ordering, such as using heuristics to guide the search, can dramatically improve performance. Furthermore, effective pruning techniques can significantly reduce the number of explored solutions, avoiding unnecessary computation. Overly aggressive pruning, however, could risk missing valid solutions.
In summary, backtracking provides a systematic way to explore the solution space, suitable for problems where the solution space is well-defined. However, its computational expense for large problems necessitates careful design and optimization strategies, including smart ordering and effective pruning techniques. The choice of exploration order and pruning rules significantly impact algorithm efficiency.
Branch and Bound: Pruning the Search Tree
Branch and bound is a sophisticated technique for solving optimization problems by systematically exploring a search tree while pruning branches that are guaranteed not to contain the optimal solution. It combines the systematic exploration of backtracking with intelligent pruning based on bounds on the optimal solution. The traveling salesman problem, where the goal is to find the shortest route that visits all cities exactly once, often employs branch and bound to find optimal or near-optimal solutions. Similarly, integer programming problems frequently utilize branch and bound to efficiently search the feasible region.
Case Study 1: In operations research, branch and bound is used to optimize resource allocation, efficiently searching the solution space. Case Study 2: In scheduling problems, branch and bound helps find optimal schedules, pruning infeasible options early.
Branch and bound's effectiveness lies in its ability to efficiently prune the search tree. The key is to develop tight bounds that accurately estimate the potential cost of exploring a branch. Well-designed bounds dramatically reduce the number of nodes explored, leading to significant performance gains. The choice of bounding technique significantly impacts efficiency.
The implementation of branch and bound algorithms can be complex, requiring careful design of the branching strategy and the bounding functions. Moreover, the efficiency heavily relies on the effectiveness of the bounding functions. Weak bounds result in less pruning and reduced performance gains. Conversely, too-strong bounds could be computationally expensive to compute, negating any benefits from reduced search.
Branch and bound offers a powerful technique for solving optimization problems, particularly those with large search spaces. However, the effectiveness depends on the quality of the bounding functions and the chosen branching strategy. The balance between computational cost for tighter bounds and reduced search space requires careful consideration and tuning.
Conclusion: Mastering algorithmic complexity requires a diverse toolkit. This article has explored five powerful strategic approaches: divide and conquer, dynamic programming, greedy algorithms, backtracking, and branch and bound. Each approach offers unique advantages and limitations, suitable for different problem types and contexts. Selecting the right approach necessitates careful consideration of the problem's characteristics, computational resources, and the trade-off between optimality and efficiency. The ongoing evolution of algorithms and computing power will continue to refine these strategies and pave the way for innovative solutions to increasingly complex challenges.