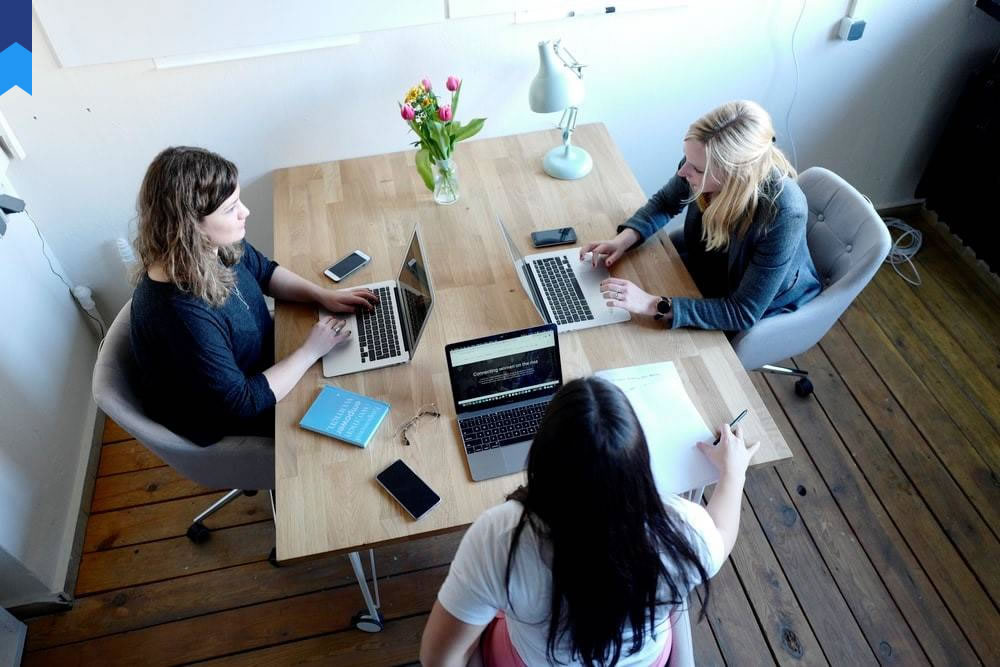
Conquer Automata Theory's Challenges With These Proven Strategies
Automata theory, a cornerstone of computer science, often presents significant hurdles for students and practitioners alike. This article delves into practical, innovative strategies to overcome common challenges, moving beyond basic overviews and exploring advanced techniques.
Understanding Finite Automata: From Theory to Practice
Finite automata (FA), the simplest model of computation, are frequently misunderstood. Many struggle to visualize their operation and apply them to real-world problems. One key challenge is translating formal definitions into practical implementations. Consider the problem of designing an FA to recognize valid email addresses. The seemingly simple task requires careful consideration of various rules, including the presence of an "@" symbol, a domain name, and acceptable character sets. A common mistake is neglecting edge cases, leading to incorrect acceptance or rejection of inputs. For instance, failing to account for multiple periods in the domain name could result in an inaccurate FA.
Another crucial aspect is the minimization of finite automata. A large, unminimized FA is difficult to understand and implement efficiently. Algorithms like the Hopcroft minimization algorithm offer a systematic way to reduce the number of states, improving readability and performance. This algorithm, while conceptually straightforward, requires meticulous attention to detail during implementation. For example, a subtle error in partitioning states can lead to an incorrect minimized automaton, resulting in flawed recognition capabilities. To illustrate this point, let’s consider an FA designed for recognizing binary strings divisible by three. The initial unminimized FA might contain many more states than necessary. Applying the Hopcroft algorithm would efficiently reduce this to a minimum-state equivalent, enhancing its usability and efficiency.
Practical application of FA extends to lexical analysis in compilers, pattern matching in text editors, and network protocol validation. Case study one: a compiler for a newly designed programming language utilizes FA to recognize keywords, identifiers, and operators, making the process faster and more efficient. Case study two: a network device uses a FA to filter malicious traffic by recognizing specific patterns in network packets. Effective use of FA in these scenarios is crucial. A poorly designed FA can negatively impact the performance and reliability of the entire system, emphasizing the need for precise understanding and efficient implementation. Furthermore, the transition table representation of an FA needs to be carefully constructed to avoid errors. One common error is forgetting transitions, causing the system to reject legitimate inputs. The careful and thorough use of minimization techniques becomes indispensable, thus the need to learn and apply the algorithm.
Finally, converting regular expressions into equivalent FAs is a common task in many applications. Understanding the relationship between these two formalisms is essential, and tools like regular expression to FA converters can ease the process. However, understanding the underlying algorithms is critical to troubleshooting and optimizing the conversion process. A mismatch between the intended regular expression and its resulting FA will lead to unexpected errors.
Context-Free Grammars and Parsing Techniques
Context-free grammars (CFGs) form the backbone of many programming language compilers and natural language processing systems. The challenge lies not only in understanding their formal definitions but also in applying parsing techniques to generate parse trees from input strings. Top-down parsing methods, such as recursive descent parsing, involve systematically constructing a parse tree by expanding non-terminal symbols based on the grammar rules. This often demands efficient management of the call stack and careful consideration of ambiguous grammar rules. A common pitfall is handling left recursion, which can lead to infinite loops. A well-defined grammar free of left recursion is critical for efficient and deterministic parsing. Case study one: Consider the design of a compiler for a functional programming language. Efficient parsing of function calls and nested expressions is pivotal for the compiler’s effectiveness. A well-designed grammar and parsing techniques are needed for this. Case study two: In natural language processing, CFGs are employed to analyze sentence structure. A grammar reflecting the complex grammatical rules of a language is critical for accurate parsing. A challenge is dealing with ambiguous grammars where multiple parse trees are possible for a single sentence.
Bottom-up parsing methods, such as LR(k) parsing, offer an alternative approach by directly constructing the parse tree from the input string. This requires careful construction of parsing tables and understanding of the state transitions. Incorrectly constructed parsing tables can lead to errors. Another challenge is understanding the different LR(k) variants and their associated limitations. The correct choice of LR(k) parser depends on the complexity of the grammar. Case study one: A compiler for an object-oriented language utilizes LR parsing for syntax analysis. Its efficiency is dependent on grammar design, parser table generation, and handling of errors. Case study two: In natural language processing systems, efficient parsing of complex sentences can benefit from employing bottom-up methods. However, difficulties may arise in dealing with highly ambiguous grammars that require extensive backtracking.
Ambiguity in CFGs often leads to multiple parse trees for a single string. Techniques like disambiguating rules are required to resolve this issue, but this adds complexity. Similarly, the design of efficient parsing algorithms is crucial for optimizing compiler performance, especially with complex grammar rules and longer strings. The choice of a suitable parser, whether recursive descent, LR, or LL, heavily depends on the characteristics of the specific grammar, with each having its own strengths and limitations in handling various grammars. Choosing the wrong parser can greatly impair the parser's performance and efficiency. Furthermore, error handling is another critical aspect of parsing. The parser needs to gracefully handle invalid input strings, providing informative error messages to assist in debugging.
Finally, the conversion of CFGs to other forms like Chomsky normal form is beneficial for certain parsing algorithms. Although seemingly a theoretical exercise, this step significantly impacts the efficiency and correctness of parsing. Efficient parsing necessitates a thorough understanding of these concepts and their impact on system performance.
Turing Machines and Undecidability
Turing machines (TMs) represent a powerful model of computation, capable of simulating any algorithm. However, the inherent complexity of TMs introduces unique challenges. One significant hurdle is understanding the concept of undecidability. The halting problem, for example, demonstrates the limitations of computation: there is no algorithm that can determine whether an arbitrary TM will halt on a given input. This fundamental concept can be difficult to grasp and requires careful consideration of the TM's behavior. Case study one: Verifying the correctness of software through formal methods involves developing TMs to check code properties. However, due to undecidability, this is not possible for all cases. Case study two: In theoretical computer science, research into undecidable problems pushes the limits of what algorithms can achieve. The pursuit of solutions to these problems deepens our understanding of computational limits.
Another area of complexity stems from designing TMs for specific tasks. Creating an efficient TM for a given problem necessitates a detailed understanding of the problem's computational requirements. A poorly designed TM can be inefficient, leading to significant performance issues. For example, a naive TM for multiplication could take exponentially longer than a more optimized version. Therefore, efficient algorithm design is as crucial in TMs as in any other computational model. Case study one: A TM used to simulate a sorting algorithm should be designed with efficiency in mind to minimize computational overhead. Case study two: A TM designed to search a database needs to account for various search strategies to optimize search time. Poorly optimized TMs can significantly hamper performance.
Furthermore, visualizing the execution of a TM can be challenging. The step-by-step operation of a TM often involves numerous state transitions and tape movements, making it difficult to trace the execution flow. Efficient tools and techniques are essential for visualizing and debugging TMs. For instance, simulators that provide visual representations of the tape and state transitions can simplify the process. Case study one: Debugging a TM designed for a complex algorithm requires careful monitoring of its execution, using tools to trace the state changes and tape movements. Case study two: Teaching students automata theory frequently uses visualization tools to clarify how TMs operate.
Finally, understanding the relationship between TMs and other computational models, such as recursive functions and lambda calculus, is crucial for a holistic perspective of computation theory. This equivalence underscores the power of TMs as a universal model of computation, but also highlights the limitations of any computational model in handling undecidable problems. A comprehensive understanding of TM limitations is essential for approaching computational problems effectively and realistically.
Pushdown Automata and Context-Free Languages
Pushdown automata (PDAs) extend the capabilities of finite automata by incorporating a stack. This stack allows PDAs to recognize context-free languages, which are more expressive than regular languages. However, designing and understanding PDAs can be more challenging than working with FAs. One common difficulty involves managing the stack effectively, ensuring that the PDA transitions correctly based on the input and stack contents. Incorrect stack manipulation can lead to incorrect acceptance or rejection of strings. Case study one: A parser for a programming language might use a PDA to manage the scope of variables. A poorly designed PDA could lead to scoping errors. Case study two: A compiler for a language with recursive function calls utilizes a PDA to handle function calls and returns. Efficient stack management is crucial to its function.
Another key aspect is understanding the relationship between PDAs and CFGs. PDAs are formally equivalent to CFGs, meaning they recognize the same class of languages. However, converting a CFG to an equivalent PDA or vice-versa requires a solid understanding of both formalisms and the transformation procedures. A common pitfall is misunderstanding how the stack is used in the PDA to reflect the derivation steps in the CFG. Case study one: Converting a CFG representing the grammar of a programming language to a PDA for parsing allows for a more efficient and practical implementation. Case study two: Analyzing the properties of a language described by a CFG by constructing its corresponding PDA can provide insights into its complexity.
Efficient PDA design is essential for applications that require recognizing context-free languages. For example, a PDA used for parsing arithmetic expressions needs to handle operator precedence and associativity correctly to produce the correct parse tree. Poor PDA design can lead to errors in the generated parse tree, ultimately impacting the correctness of the application. Case study one: Compiler construction for programming languages heavily relies on PDAs for parsing, and efficiency in PDA design directly correlates with the compiler's performance. Case study two: Natural language processing uses PDAs to parse sentences, and optimizing these PDAs leads to faster and more efficient language processing.
Finally, understanding the limitations of PDAs is equally important. While PDAs can handle context-free languages, they cannot handle more complex languages. Therefore, it’s crucial to understand the classes of languages and the appropriate automata for each class. This knowledge prevents trying to use a PDA for problems beyond its capabilities. This is also crucial for selecting the correct automata based on the language's properties and its application.
Advanced Topics and Future Trends
Beyond the fundamental concepts, several advanced topics in automata theory continue to shape the field. One area is the study of complexity classes and their relationship to different automata models. Understanding the time and space complexity of different algorithms implemented using automata models is crucial for optimizing performance. For example, determining the complexity of algorithms involving Turing machines requires careful consideration of tape movements and state transitions. Case study one: Analyzing the efficiency of algorithms for deciding the emptiness problem for context-free languages helps in optimizing parser design. Case study two: Research into complexity classes provides theoretical bounds on the solvability of computational problems.
Another significant area is the application of automata theory to verification and model checking. Formal verification techniques leverage automata models to check the correctness of software and hardware systems. These techniques are invaluable for ensuring the reliability and safety of critical systems. However, the complexity of these verification processes can be computationally expensive, necessitating efficient algorithms and data structures. Case study one: Model checking is employed extensively in the design of hardware systems, verifying the correctness of circuits and logic designs. Case study two: Formal verification methods are used in software engineering to analyze code and identify potential errors.
Furthermore, the intersection of automata theory with machine learning is an emerging area. Automata models can be used in the design of learning algorithms, allowing for the creation of systems that can learn and adapt to new data. The challenge lies in developing efficient learning algorithms that effectively integrate automata models into the learning process. Case study one: Reinforcement learning algorithms can benefit from automata models to represent the state space and transition dynamics. Case study two: Automata models can be used in natural language processing to build language models that can learn and adapt to new data.
Finally, ongoing research focuses on the development of new automata models and algorithms for tackling increasingly complex problems. For instance, exploring probabilistic automata or quantum automata expands the scope of computational models. These models offer novel approaches to solving complex problems that are intractable using traditional automata models. The field continues to evolve, promising new insights and innovations for years to come.
Conclusion
Overcoming the challenges of automata theory requires a blend of theoretical understanding and practical application. This article has explored several key areas, providing practical strategies and examples to navigate these complexities. From the foundational concepts of finite automata to the more advanced topics of Turing machines and model checking, a robust grasp of these principles is essential for success in computer science. By understanding the limitations and capabilities of various automata models, and by mastering the techniques for designing and implementing efficient automata-based algorithms, students and practitioners alike can effectively address the challenges presented by this crucial field. The continued exploration of advanced topics and their applications will undoubtedly shape future advancements in computer science, reinforcing the enduring importance of automata theory.