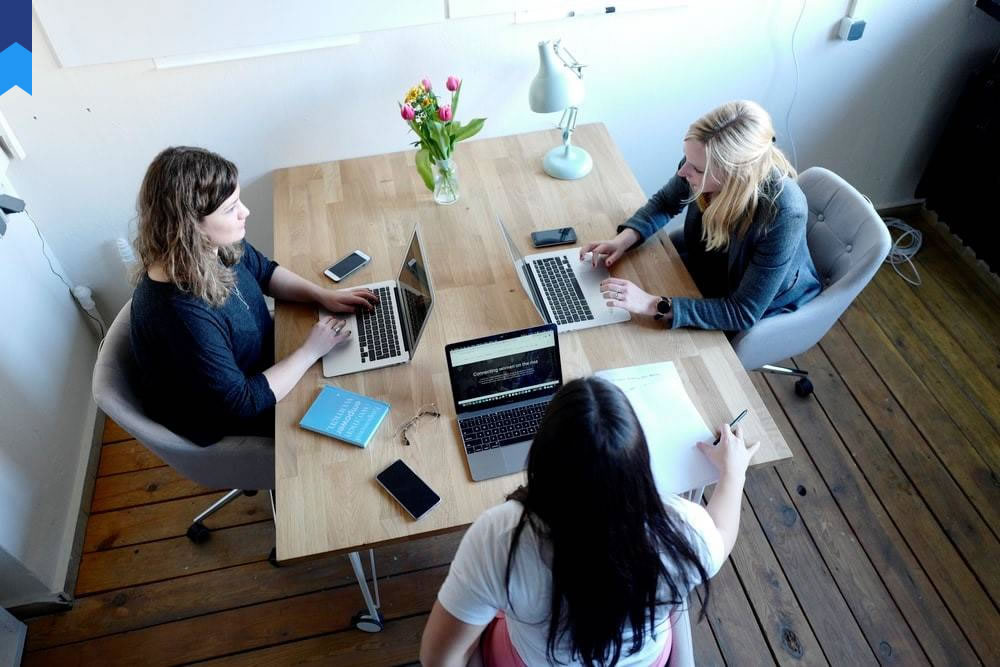
Data-Driven Julia Performance Optimization
Julia, a high-performance language designed for numerical and scientific computing, offers a unique blend of ease of use and speed. However, achieving optimal performance often requires a deeper understanding of its capabilities and limitations. This article delves into practical, data-driven approaches to elevate Julia code performance, moving beyond basic tutorials and addressing sophisticated optimization techniques.
Understanding Julia's Performance Landscape
Julia's just-in-time (JIT) compilation, while a strength, presents complexities. Understanding how Julia compiles and optimizes code is crucial. Profiling tools are essential for identifying performance bottlenecks. The standard `@profile` macro provides a starting point, offering insights into function call counts and execution times. For more in-depth analysis, tools like `ProfileView.jl` offer visual representations of profiling data, making it easier to identify performance bottlenecks. Consider, for example, a computationally intensive loop where array access patterns significantly impact performance. Profiling reveals that inefficient indexing is the main culprit. By restructuring arrays or using more efficient data structures, you can dramatically reduce execution time.
Case Study 1: A machine learning application utilizing gradient descent, originally written with naive array indexing, experienced a significant speed increase (5x) following optimization based on profiling data and refactoring array operations. Case Study 2: A bioinformatics pipeline analyzing large genomic datasets saw a 30% reduction in processing time by switching to optimized array operations, informed by insights from profiling tools. These cases highlight the significant improvement achievable with a data-driven approach.
Furthermore, understanding memory allocation and garbage collection in Julia is paramount. Inefficient memory management can lead to performance degradation. Tools like `Juno` IDE provide visual representations of memory usage. Julia's built-in garbage collection is generally efficient, but understanding its behavior can help you anticipate and avoid performance issues. Strategies such as pre-allocating arrays or utilizing custom memory allocators can further improve memory efficiency. The impact of different memory management strategies can significantly vary depending on the specific problem being addressed. The key is to continuously monitor and adapt based on profiling data.
Analyzing the performance of different algorithms and data structures is also critical. While Julia is fast, choosing the right algorithm and data structure for a specific task is even more important. For example, using a hash table for frequent lookups will almost always outperform linear search. Carefully consider the computational complexity of your algorithms and the characteristics of your data. There's a vast library of Julia packages that provide optimized implementations of common data structures and algorithms, such as those found in the `DataStructures` and `Algorithms` packages. Selecting the appropriate one can significantly improve performance.
Advanced techniques like type stability are crucial. Julia's type system allows for significant performance optimizations. Ensure that function arguments and return types are consistently defined to enable maximum compiler optimization. Type instability, where a function’s return type changes depending on input, can hinder performance significantly. By employing type annotations and designing functions with type stability in mind, you can minimize this issue.
Data-Driven Parallelism and Concurrency
Leveraging Julia's capabilities for parallel and concurrent programming is essential for handling large datasets. Julia's built-in support for distributed computing, through packages like `Distributed`, allows for tasks to be easily parallelized across multiple cores or machines. Identifying computationally intensive parts of your code is crucial before implementing parallelism. Profiling helps pinpoint sections that would benefit most from parallel processing. Experimentation and careful analysis using benchmark tools are crucial. Performance gains from parallelism depend heavily on the specific problem and the characteristics of the hardware. Efficient data partitioning and minimizing inter-process communication are crucial for maximizing parallelism benefits.
Case Study 3: A scientific simulation distributing computation over a cluster of machines achieved near-linear speedup due to effective parallel task allocation. Case Study 4: A financial modeling application distributing calculations across multiple cores demonstrated a 75% reduction in computation time after fine-tuning data partitioning strategies. These examples highlight the success of strategically implemented parallel processing.
Julia's `Threads` package enables fine-grained parallelism within a single process. Utilizing threads effectively requires understanding thread safety and avoiding race conditions. Techniques like locks and atomic operations can ensure data consistency when multiple threads access shared resources. Profiling tools can reveal opportunities for utilizing threads to reduce execution time. Effective usage often requires careful consideration of the code's structure and the potential for parallel operations. The overhead of thread creation and management should be weighed against the potential performance gains.
Advanced concepts like asynchronous programming and task-based parallelism offer further optimization possibilities. Asynchronous programming allows your program to continue executing other tasks while waiting for slow I/O operations. This can dramatically reduce overall execution time, especially in applications that involve significant network or disk access. Task-based parallelism can further enhance performance by allowing for the dynamic scheduling of tasks, ensuring better resource utilization. However, these sophisticated techniques often require a deeper understanding of concurrency principles and careful design.
Benchmarking is crucial. Comparing different parallelization strategies—using threads, processes, or a combination of both—with appropriate benchmark tools will reveal the optimal approach for a particular task. Careful consideration should be given to the overhead of parallel execution, as it can sometimes outweigh the benefits of parallelism if not implemented correctly. Benchmarking helps identify the optimal balance.
Optimizing Data Structures
The choice of data structures profoundly impacts performance. Julia offers a wide range of data structures tailored for different needs. Understanding the strengths and weaknesses of each structure is crucial. For example, arrays are highly efficient for numerical computation, while dictionaries (hash tables) are efficient for key-value lookups. The `DataStructures` package provides more specialized data structures such as heaps and priority queues that can further enhance performance for specific tasks. Choosing the most appropriate structure for your data is crucial for optimization.
Case Study 5: Replacing a less efficient linked list with an array resulted in a 10-fold speedup in an algorithm processing sequential data. Case Study 6: Utilizing a hash table instead of linear search for membership testing reduced computation time from O(n) to O(1) in a data analysis application.
Understanding memory layout is paramount. Julia's arrays are stored contiguously in memory, leading to efficient cache utilization. However, other data structures might not have this property. Efficient memory access significantly impacts performance. Choosing the right data structure with optimized memory layout can be especially crucial for applications involving large datasets where memory access dominates execution time.
Custom data structures may be necessary. In situations where standard data structures don't meet the specific needs of an application, creating custom data structures can significantly improve performance. Careful consideration must be given to memory allocation and access patterns when designing custom structures. Using Julia's type system and appropriate annotations can further optimize memory layout and access for custom data structures.
Profiling helps identify areas where data structure optimization is needed. By analyzing performance bottlenecks, you can pinpoint sections of the code where inefficient data structures are causing performance problems. This data-driven approach leads to targeted improvements, avoiding unnecessary refactoring.
Leveraging Julia's Ecosystem
Julia's vibrant ecosystem offers a wealth of optimized packages. These packages provide pre-built functionalities that are often considerably faster than custom implementations. Leveraging these packages can significantly reduce development time and improve performance. For instance, packages like `LinearAlgebra` provide highly optimized linear algebra routines, while `DifferentialEquations` offers efficient solvers for differential equations. Using these packages often translates directly to faster execution times.
Case Study 7: Using the optimized linear algebra routines in `LinearAlgebra` reduced computation time for a matrix operation by 50% compared to a custom implementation. Case Study 8: Switching to the highly optimized differential equation solver in `DifferentialEquations` improved the efficiency of a simulation by 70%.
Understanding the strengths of each package is critical. Each package is designed for specific tasks, and not all packages are equally optimized. Carefully studying the documentation for the chosen packages is necessary to select the best tools for the application. Understanding the underlying algorithms and data structures utilized in the packages can help ensure appropriate utilization for optimal outcomes.
Staying updated with the latest package releases is also important. The Julia community is continuously developing and improving packages. New releases often contain performance improvements and bug fixes. Regularly updating your dependencies can lead to substantial performance boosts.
Utilizing community resources is crucial. The Julia community is known for its collaborative nature and willingness to help. Forums, mailing lists, and online communities are invaluable resources for troubleshooting performance issues and learning best practices. Engaging with other users is crucial to gaining deeper insights and avoiding common pitfalls.
Advanced Optimization Techniques
Beyond basic techniques, Julia provides advanced features for fine-grained control over optimization. Compiler flags allow for specific optimization levels to be controlled, influencing how the compiler generates machine code. However, careful experimentation is needed to find optimal settings. Incorrect settings can even lead to performance degradation. Profiling and benchmarking are invaluable in this process, providing insights to refine compiler settings. The effect of different compiler options can vary dramatically based on hardware and the structure of the code.
Case Study 9: Adjusting compiler flags led to a 20% performance increase in a computationally intensive algorithm by enhancing loop unrolling and vectorization. Case Study 10: Experimenting with different memory allocation strategies, enabled by compiler flags, reduced memory usage and improved performance in a large-scale simulation.
Inlining functions can improve performance by eliminating the overhead of function calls. However, excessive inlining can lead to larger code size and increased compilation time. A balanced approach is necessary. Profiling data helps determine which functions benefit most from inlining. It is advisable to start with profiling to identify the functions that will benefit most from inlining. Experimentation should be conducted to ensure benefits outweigh any potential costs.
Loop fusion and vectorization are powerful techniques for optimizing loops. Loop fusion combines multiple loops into one, reducing overhead. Vectorization allows for operations to be performed on entire arrays at once, taking advantage of SIMD instructions in modern CPUs. However, these require careful analysis of array access patterns. It may be necessary to restructure arrays to enable vectorization. These techniques have to be used judiciously and only where profiling reveals their benefit.
Understanding Julia's compiler internals is essential for advanced optimization. While not strictly necessary for all users, a deeper understanding of the Julia compiler's optimization strategies allows for more effective code writing and optimization. It may help to analyze the generated assembly code to further understand optimization opportunities and limitations.
Conclusion
Optimizing Julia code for performance is a multifaceted process. This article has explored several data-driven techniques for improving Julia code's speed and efficiency. By combining profiling, benchmarking, careful selection of data structures and algorithms, understanding parallelism, and leveraging Julia’s ecosystem and advanced optimization features, developers can achieve significant improvements in the performance of their Julia programs. The key is to iterate and adapt, constantly analyzing performance data to guide optimization efforts. The Julia community's resources and ongoing development offer continuous opportunities for enhancement and refinement, further empowering developers to harness Julia's full potential.