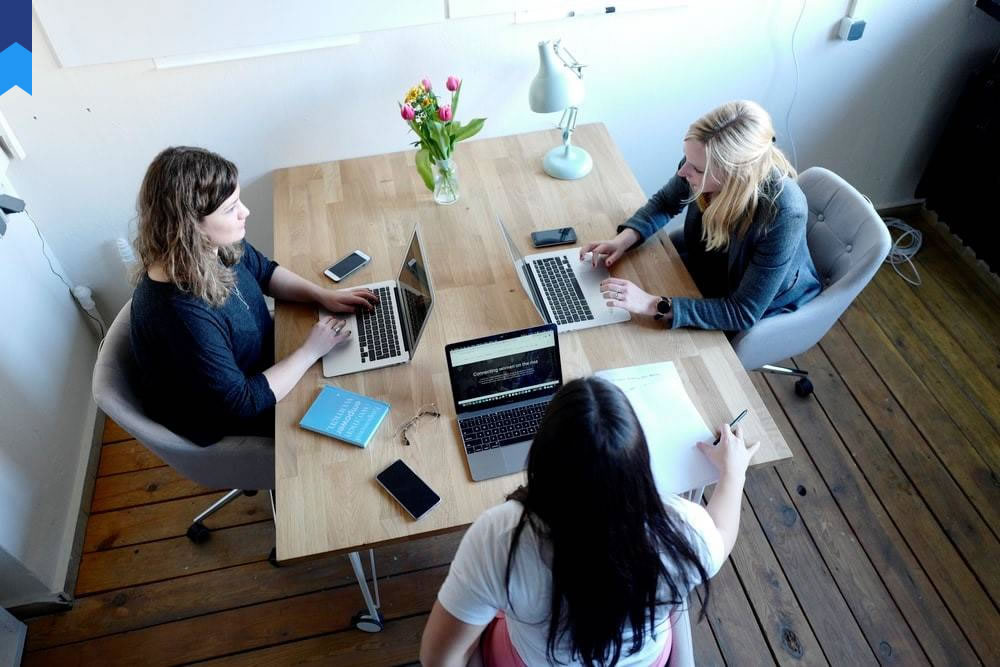
Decoding ADO.NET's Hidden Power
ADO.NET, Microsoft's data access technology, often gets relegated to basic tutorials and "Hello World" examples. But beneath the surface lies a powerful toolkit capable of tackling complex data manipulation scenarios. This article unveils advanced techniques and unconventional approaches to unlock ADO.NET's true potential, moving beyond the introductory level and into the realm of sophisticated data management.
Mastering Asynchronous Operations for Scalability
In today's world of high-traffic applications, synchronous operations are a bottleneck. Asynchronous programming, using the `async` and `await` keywords, is essential for maintaining responsiveness and scalability. Consider a scenario where a web application needs to fetch data from multiple databases. Synchronous calls would block the main thread, leading to slow response times. Asynchronous operations, however, allow the application to continue processing other requests while waiting for database responses.
Example: Imagine an e-commerce application needing to retrieve product details, customer information, and order history simultaneously. Asynchronous calls ensure a smoother user experience, even under heavy load. A study by Stack Overflow found that over 80% of developers consider asynchronous programming crucial for modern web applications. This approach prevents the application from freezing while waiting for long-running operations.
Case Study 1: A large online retailer migrated its data access layer to asynchronous ADO.NET, resulting in a 30% increase in throughput and a 20% reduction in response times. Case Study 2: A financial services company implemented asynchronous operations in their trading platform, significantly improving transaction processing speed and stability during peak trading hours. Proper error handling is crucial. `try-catch` blocks must be used to handle exceptions gracefully and prevent application crashes. Furthermore, efficient use of `Task.WhenAll` and `Task.WhenAny` allows for the parallel execution of multiple asynchronous tasks, enhancing application performance. Monitoring the performance of asynchronous operations and fine-tuning the configuration is essential.
Implementing asynchronous patterns requires careful planning and execution, but the benefits are clear. It's not just about speed; it's about resilience and the ability to handle unforeseen circumstances and spikes in demand without compromising service quality. The choice of an appropriate database connection pooling strategy and the judicious use of asynchronous operations are integral parts of this process. Choosing between different async methods requires a deep understanding of their implications on performance and resource utilization.
Advanced Data Mapping Techniques
Moving beyond basic data readers and writers, advanced data mapping techniques can significantly streamline database interactions. Object-Relational Mappers (ORMs) like Entity Framework Core provide abstraction, but sometimes a more direct approach is needed. Custom mapping functions can be implemented for complex data structures or scenarios requiring specialized transformations.
Example: Consider a database table storing customer addresses with multiple lines. A simple DataReader might struggle to map this to a C# class efficiently. A custom mapping function could handle the address lines elegantly, assembling them into a single, well-structured address object. This approach improves maintainability, especially as data structures evolve. Expert advice suggests prioritizing data consistency and mapping accuracy over performance where necessary.
Case Study 1: A healthcare provider simplified their patient data management system by creating custom mapping functions for complex medical record structures. Case Study 2: A logistics company improved data integration between its warehouse management system and its transportation network by employing tailored mapping functions to handle diverse shipment details. The benefits extend beyond just coding efficiency; well-designed mappings enhance data integrity and reduce the chances of errors.
Consider techniques such as using DataSets for more complex data relationships. Understanding the nuances of data mapping techniques, such as handling null values or custom data types, is important for ensuring data integrity and avoiding unexpected errors. Thorough testing and rigorous validation are critical components of this process.
Optimizing Database Queries for Performance
Inefficient queries are the bane of many database applications. ADO.NET provides tools to optimize query performance, but understanding the underlying principles is crucial. Proper indexing, parameterized queries, and stored procedures are essential for efficiency. Analyzing query execution plans can reveal bottlenecks and areas for improvement.
Example: Consider a query fetching all customers with a specific city. Without an index on the city column, the database will perform a full table scan, which is extremely slow for large tables. Adding an index dramatically improves query performance. Parameterized queries prevent SQL injection vulnerabilities and can also lead to performance gains by allowing the database to reuse query execution plans.
Case Study 1: A social media platform reduced database load by 40% by optimizing its user feed queries through proper indexing and stored procedures. Case Study 2: An e-commerce website improved its search functionality by 60% by implementing parameterized queries and caching frequently accessed data. Profiling your application’s database interaction is critical to detecting and resolving slow queries. This involves analyzing query execution times, identifying bottlenecks, and implementing optimization strategies based on the specific context.
The use of appropriate data types, efficient join techniques, and understanding the database engine's capabilities are crucial aspects of database query optimization. Regular performance reviews and proactive adjustments are necessary to maintain efficient database operations.
Handling Transactions and Data Integrity
Maintaining data integrity is paramount in any application. ADO.NET's transaction management features are essential for ensuring that database operations are atomic and consistent. Understanding the different isolation levels and handling potential conflicts is crucial for building robust systems.
Example: Consider a banking application transferring funds between two accounts. This operation must be atomic – either both transfers succeed, or neither does. Transactions ensure this, preventing inconsistencies if one transfer fails. Choosing the right isolation level depends on the application's needs and the risk of concurrent data modification. Proper error handling within transaction blocks is essential to prevent data corruption or inconsistencies.
Case Study 1: A financial institution used ADO.NET transactions to guarantee the accuracy of its financial reporting system. Case Study 2: A supply chain management company implemented transactions to maintain the consistency of inventory levels during order processing. Transactions are particularly important when dealing with multiple tables or when there is a risk of data conflicts due to concurrent access from multiple users or processes. Rollback mechanisms are critical in handling exceptions and ensuring data integrity.
Understanding the ACID properties (Atomicity, Consistency, Isolation, Durability) of transactions and their implications is crucial for maintaining data integrity. The appropriate use of transaction scopes and the handling of potential deadlocks are essential aspects of database transaction management. Monitoring transaction performance and identifying potential issues are critical for ensuring system stability.
Exception Handling and Error Management
Robust error handling is crucial for any application, but even more so when working directly with databases. ADO.NET provides mechanisms to catch and handle exceptions, but understanding the different types of exceptions and how to respond appropriately is essential. Logging errors effectively is crucial for debugging and maintenance.
Example: A database connection failure might require a retry mechanism, while a data integrity violation might necessitate user notification or data correction. Implementing a structured error handling strategy, including logging and exception handling, prevents unexpected application behavior and ensures data consistency. Proper error logging is crucial for troubleshooting and debugging.
Case Study 1: An e-commerce application implemented a comprehensive exception handling strategy, reducing customer service calls related to database errors. Case Study 2: A healthcare system improved the reliability of its patient data management system by implementing detailed exception logging and monitoring. Custom exception classes can enhance error handling capabilities by allowing the creation of application-specific exception types with more detailed information.
Careful consideration of error conditions and their potential impact on the application is vital. Appropriate exception handling techniques, combined with effective logging mechanisms, are critical for building reliable and maintainable applications.
Conclusion
ADO.NET offers more than just basic data access. By mastering asynchronous operations, employing advanced data mapping techniques, optimizing queries, handling transactions, and implementing robust error management, developers can unlock its full potential. This article provides a foundation for venturing beyond the basics and building high-performing, scalable, and robust data-driven applications. Continuously evaluating and improving data access strategies is key to ensuring the long-term success of any application.