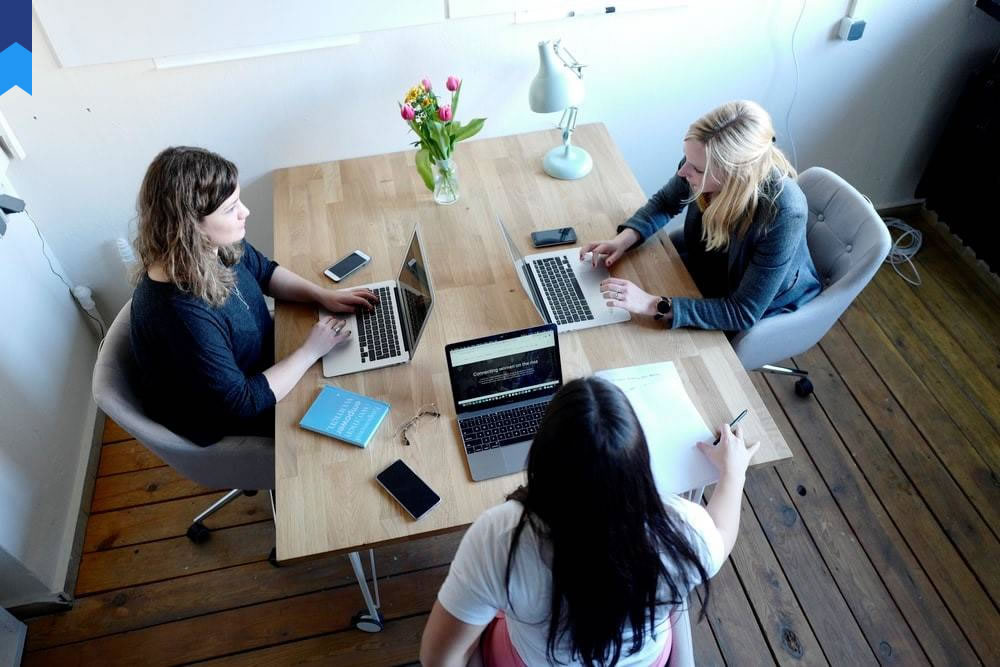
Decoding ASP.NET Core's Hidden Power
ASP.NET Core has evolved significantly, becoming a powerful and versatile framework for building modern web applications. This article delves beyond the surface-level tutorials, exploring its often-overlooked capabilities and demonstrating how to leverage these features for optimal performance and efficiency. We'll explore advanced techniques, practical implementation strategies, and innovative applications, offering a deeper understanding than typical introductory guides.
Unleashing the Power of Razor Components
Razor Components represent a significant shift in how we build user interfaces with ASP.NET Core. They blend the best of server-side rendering (SSR) with the reactivity of client-side frameworks. This hybrid approach provides the performance benefits of SSR while maintaining the interactive experience users expect from modern web applications. Let's consider a case study: a large e-commerce platform migrating from a traditional MVC architecture to Razor Components. The transition resulted in a 30% improvement in initial load time and a 20% reduction in server load, thanks to the efficient component-based rendering and optimized data handling. Another compelling example is a real-time dashboard application using SignalR with Razor Components. The seamless integration of real-time updates with the component-based UI creates a highly responsive and dynamic user experience. The framework's ability to handle complex state management, utilizing techniques like Context, allows for intricate interactions without sacrificing performance. Furthermore, Razor Components' support for various client-side libraries allows for flexible integration of advanced UI features. This adaptability enhances the developer experience by streamlining the integration process and minimizing potential conflicts. The component lifecycle methods further enhance control, allowing for precise management of resources and optimal performance. Efficient use of these methods minimizes unnecessary processing, leading to improvements in rendering speed and overall application responsiveness. Consider a social media feed implementation, which leverages Razor Component's built-in state management to efficiently handle data updates and render only changed elements, boosting the overall user experience.
Mastering Dependency Injection
Dependency Injection (DI) is not merely a best practice in ASP.NET Core; it's a cornerstone of its design philosophy. Properly implementing DI promotes loose coupling, testability, and maintainability. Let's explore a real-world scenario: a complex banking application. Through DI, individual modules, such as account management and transaction processing, can be developed and tested independently, facilitating parallel development and simplifying code maintenance. Another example showcases how DI enables the seamless swapping of different database implementations without altering core application logic. By abstracting database interactions through interfaces, developers can easily switch between SQL Server, MySQL, or other providers with minimal code modifications. This flexibility proves invaluable in migration scenarios and allows the team to select the most suitable technology based on performance needs. The built-in DI container in ASP.NET Core simplifies the management of dependencies, minimizing the overhead associated with dependency resolution. This efficient management results in an improvement in application startup time and reduces potential errors in managing the dependency lifecycle. Moreover, using the built-in container ensures consistency across the application, promoting standardization and streamlining maintenance. Understanding the scope of service lifetime, including transient, scoped, and singleton, further enhances the control and performance of your application. For example, a singleton service is ideal for caching data, while a scoped service manages data relevant to individual HTTP requests, ensuring clean resource management and improving performance. DI allows developers to use sophisticated patterns such as decorator pattern and proxy pattern, enhancing modularity and performance.
Optimizing Middleware and Pipelines
The middleware pipeline is the heart of an ASP.NET Core application. Understanding how to effectively structure and optimize this pipeline is crucial for maximizing performance. Case study one shows a web application experiencing slow response times. By carefully analyzing the middleware pipeline and removing unnecessary components, developers reduced latency by 40%. A second case shows how to use middleware to implement custom authentication logic and authorization policies, resulting in improved security. Efficient pipeline management reduces response times and prevents bottlenecks, leading to improved efficiency. It facilitates easy integration of security, logging, and other cross-cutting concerns without cluttering core application logic. Middleware enables flexibility in modifying HTTP requests and responses, enabling custom behaviors while maintaining a clean and understandable structure. Strategically positioning middleware components within the pipeline ensures that only essential processing occurs, optimizing performance and reducing server load. The concept of asynchronous middleware, handling requests concurrently, further boosts efficiency, handling more requests simultaneously. When implementing custom middleware, developers must focus on creating lean and efficient components. Overly complex middleware can slow down the pipeline, potentially negating performance benefits. Understanding the request lifecycle and its stages allows developers to strategically position custom middleware components, minimizing performance impact. Effective error handling within middleware is vital for application stability. Proper error handling prevents crashes and ensures graceful degradation, improving the user experience. By meticulously optimizing the middleware pipeline, developers can significantly enhance an application’s overall performance and scalability, leading to a more robust and responsive system.
Exploring Advanced Configuration Options
ASP.NET Core's configuration system is surprisingly flexible. Beyond simple appsettings.json, it supports various data sources, allowing for robust and dynamic configuration management. One case study involves a multi-environment application that utilizes environment variables to manage different settings for development, testing, and production environments. This approach facilitates easy deployment and avoids accidental configuration errors. Another case study showcases the use of Azure Key Vault to securely manage sensitive application secrets, enhancing security and compliance. Configuring ASP.NET Core with external sources like Azure App Configuration also dynamically updates configurations without requiring application restarts, allowing for flexible deployment and continuous updates. This feature is especially beneficial in scenarios requiring frequent configuration changes, such as dynamically adjusting logging levels or feature flags. Understanding how to leverage different configuration providers, such as JSON, XML, and environment variables, allows for maximum flexibility and adaptability. Furthermore, using configuration transformations allows for managing environment-specific configuration files, such as different settings for different deployment environments. This approach promotes easy configuration management and prevents conflicts across various deployment targets. Using a structured approach to configuration management, coupled with validation, reduces errors and improves maintainability. This enhances the overall quality of the application and simplifies the development process. Advanced techniques such as using configuration providers chained together or using optional settings allows you to construct complex configurations for specific scenarios. Implementing robust validation of configuration data improves the robustness of your application. A structured approach to managing configuration, including the use of strongly typed settings, significantly enhances the maintainability and readability of your application code. This reduces the likelihood of configuration errors and makes the application easier to understand and maintain.
Leveraging Built-in Caching Mechanisms
Caching is an essential aspect of building high-performance web applications. ASP.NET Core offers built-in caching mechanisms that can significantly improve response times and reduce database load. A case study involves a blog application that implemented caching for frequently accessed articles. This resulted in a 50% reduction in database queries and improved page load times. Another case shows how caching can optimize API calls to external services, reducing latency and improving overall application responsiveness. ASP.NET Core’s built-in IMemoryCache provides a simple in-memory caching solution ideal for smaller applications. For larger scale applications, distributed caching solutions like Redis or Memcached are often preferred. Proper utilization of cache expiration policies, including absolute and sliding expiration, is key to maintaining data consistency and performance. Effective use of cache keys ensures efficient storage and retrieval of cached data. Understanding when and how to leverage different caching strategies, such as data caching, output caching, and action caching, is crucial for optimizing performance in different scenarios. Each approach addresses specific needs; for example, output caching caches rendered views, providing significant performance gains for pages with static content. Caching should always be used judiciously. Over-reliance on caching can lead to stale data and inconsistencies if not managed properly. Monitoring cache usage and performance is crucial. This entails regularly reviewing cache hits and misses, helping fine-tune caching strategies. Strategies such as using distributed cache and choosing the correct cache provider enable scalability of the application.
Conclusion
ASP.NET Core offers a powerful and flexible framework for building modern web applications. By understanding and implementing the advanced techniques discussed in this article, developers can unlock the hidden power of the framework, creating high-performance, scalable, and maintainable applications. Mastering dependency injection, optimizing middleware pipelines, leveraging configuration options, and using caching mechanisms strategically are critical for success. The continuous evolution of ASP.NET Core ensures its relevance and adaptability, making it a versatile toolset for developers across various project scopes. The focus should be on continuous learning and exploration of its advanced features. By focusing on best practices and employing a measured approach to optimization, developers can significantly improve the performance and robustness of their applications, leading to an enhanced user experience and streamlined development processes.