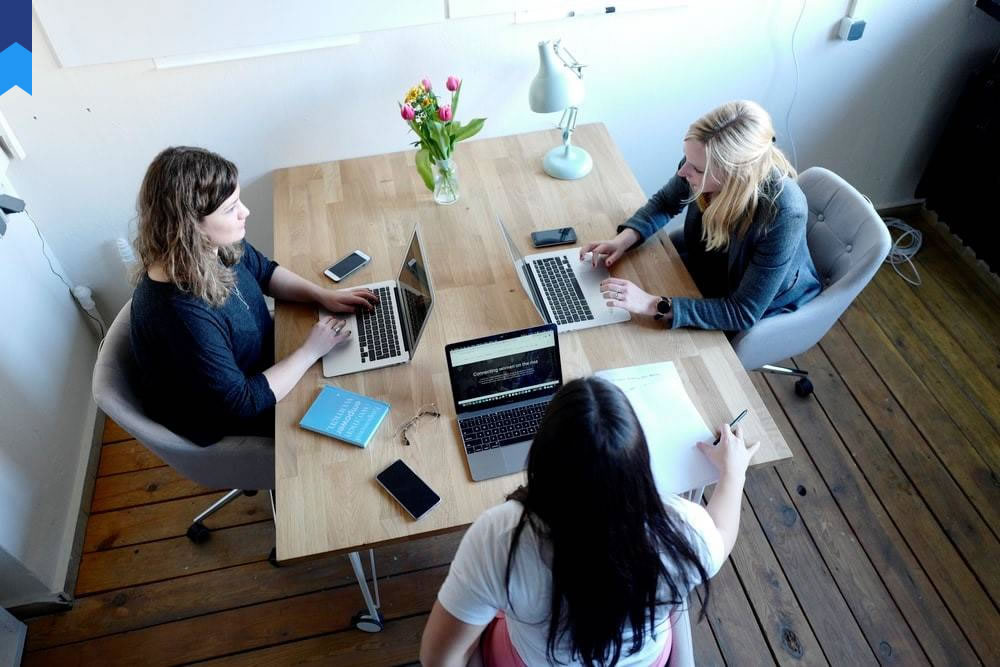
Decoding ASP.NET MVC: Beyond The Basics
ASP.NET MVC has been a cornerstone of web development for many years, empowering developers to build robust and scalable applications. However, mastering its nuances requires moving beyond elementary tutorials. This article delves into advanced techniques and innovative approaches, equipping you with the skills to tackle complex challenges and create truly exceptional web experiences.
Advanced Routing Techniques
Routing in ASP.NET MVC is far more than just mapping URLs to controllers and actions. Understanding advanced routing configurations opens up possibilities for creating clean, SEO-friendly URLs and improving the overall architecture of your application. Consider the use of attribute routing, which allows you to define routes directly within your controller actions using attributes, promoting code clarity and maintainability. For example, instead of complex route definitions in the `RouteConfig.cs` file, you can specify routes within controllers using attributes like [Route("products/{id}")]
, making it intuitive and easier to manage. Furthermore, exploring route constraints allows developers to enforce specific patterns in URLs, adding validation and control to the routing process. This enhanced control is crucial for building applications with multiple modules or complex URLs.
Case Study 1: A large e-commerce platform used attribute routing to manage their complex product catalog, resulting in simpler route definitions and improved maintainability. This made future modifications and additions much simpler and faster.
Case Study 2: A travel booking website implemented custom route constraints to validate flight codes, ensuring the application handled incorrect entries elegantly. This improved the user experience and data integrity significantly.
Beyond attribute routing and constraints, consider using named routes to increase code readability and maintainability. Named routes help avoid hardcoding route values directly into the code, improving both the code's quality and its flexibility to change. Implementing a robust routing strategy is critical to building scalable and manageable applications. The strategic use of custom route handlers and area routing allows for a well-organized and efficient routing structure within a large application, allowing for more manageable sections of the codebase.
Furthermore, exploring the use of route prefixes allows you to group related routes together, improving the organization and maintainability of large applications. Finally, using route data tokens permits the extraction of information directly from the route for use within the controller action, enhancing the potential for dynamic routing.
Mastering Dependency Injection
Dependency Injection (DI) is a cornerstone of modern software development, promoting loose coupling, testability, and maintainability. In ASP.NET MVC, DI is seamlessly integrated, allowing for a more structured and manageable application architecture. Understanding how to utilize DI effectively empowers developers to write cleaner, more testable code. The built-in DI container in ASP.NET MVC simplifies the process, allowing for effortless registration of dependencies within the application. By registering interfaces and their implementations using appropriate methods, developers can effectively decouple their components. This approach simplifies unit testing by allowing the substitution of mock dependencies, leading to more reliable and maintainable code. For example, consider a scenario where you have a class dependent on a database connection. Instead of creating the database connection directly within the class, use DI to inject an interface representing the database connection. This allows you to easily switch to a mock database connection during testing.
Case Study 1: A large banking application implemented DI to decouple its data access layer from the business logic. This made unit testing much more manageable, leading to increased software reliability and reduced bug counts.
Case Study 2: An e-commerce platform implemented DI for its logging mechanisms. This allowed them to switch between different logging providers (file, database, etc.) without modifying the core application logic.
Beyond basic DI implementation, explore advanced concepts such as constructor injection, property injection, and method injection, choosing the most suitable approach based on the specific requirements. Understanding the lifecycle of dependencies and how the container manages their instantiation is vital. Effectively using dependency injection helps to develop more robust and maintainable applications by reducing dependencies and improving testability. This also reduces the overall coupling of the application's various components, which simplifies future modifications and enhancements. DI helps avoid tight coupling between different modules and classes within the application.
Consider integrating a third-party DI container for more advanced features and customization. Mastering dependency injection is a significant step towards improving code quality, testability, and maintainability in ASP.NET MVC applications.
Handling Asynchronous Operations
In today's web development landscape, asynchronous operations are crucial for building responsive and performant applications. ASP.NET MVC provides robust support for asynchronous programming through the use of async and await keywords. Leveraging these features allows developers to handle long-running operations without blocking the main thread, preserving application responsiveness and improving overall performance. By employing asynchronous programming, developers avoid blocking the main thread and maintain application responsiveness. This is particularly critical when dealing with I/O-bound operations, such as database queries or web service calls. For instance, a scenario involving a file upload can be handled asynchronously without hindering the user interface.
Case Study 1: A social media platform adopted asynchronous programming for its real-time messaging feature, improving the user experience by preventing delays.
Case Study 2: An online payment gateway incorporated asynchronous operations for processing transactions, leading to a significant improvement in transaction throughput.
Going beyond basic asynchronous controllers, consider asynchronous actions within custom action filters and model binders to enhance application performance. Efficiently handling exceptions in asynchronous code is crucial for creating robust applications. By implementing appropriate error handling strategies, developers ensure seamless application behavior. Employing tasks and continuations offers even more control over asynchronous operations. Furthermore, consider the use of asynchronous unit testing to fully test the asynchronous parts of the application.
Understanding the nuances of asynchronous programming is vital for building high-performance, responsive ASP.NET MVC applications. Mastering the intricacies of asynchronous operations is a key step in improving the application’s overall efficiency, responsiveness, and scalability.
Optimizing Performance
Optimizing ASP.NET MVC applications for performance is crucial for providing a smooth user experience and ensuring scalability. Numerous strategies can significantly improve an application's speed and efficiency. Caching frequently accessed data using techniques like output caching and data caching can dramatically reduce database load and improve response times. Employing effective caching strategies minimizes unnecessary database calls, resulting in improved application performance. Utilizing various caching mechanisms allows developers to selectively cache specific data based on criteria such as age and frequency of access, ensuring that only the necessary data is cached.
Case Study 1: A news website implemented output caching for its static content, significantly reducing server load and improving page load times.
Case Study 2: An e-commerce platform used data caching for product information, resulting in faster response times for product page requests.
Beyond caching, consider database optimization techniques, such as indexing and query optimization, to minimize database access times. Efficient database interaction is crucial in enhancing application performance. Properly indexing database tables significantly speeds up data retrieval. Analyzing and optimizing database queries helps reduce the time taken to retrieve data. Employing techniques like connection pooling, managing database connections effectively, avoids unnecessary resource consumption.
Furthermore, optimizing application code for efficiency through techniques such as code profiling and code refactoring, streamlines application logic and improves execution speed. Profiling helps to identify performance bottlenecks in the code. Refactoring assists in improving code readability and execution efficiency. Regularly assessing the application’s performance and identifying areas for improvement through techniques like load testing, is essential for ensuring long-term efficiency. This allows developers to make proactive adjustments, improving the application's ability to handle increased traffic volumes.
Securing Your Application
Security is paramount in modern web development. ASP.NET MVC provides built-in features to enhance application security, but understanding and implementing best practices is crucial for protecting your application and user data. Implementing robust authentication and authorization mechanisms is crucial for controlling access to sensitive information. Using techniques like role-based authorization helps manage access privileges effectively. Applying the principle of least privilege, granting only necessary permissions, safeguards against unauthorized actions.
Case Study 1: A financial institution implemented multi-factor authentication to enhance the security of its online banking platform.
Case Study 2: An e-commerce website used HTTPS to encrypt data transmitted between the client and server.
Beyond the basics, explore techniques like input validation and sanitization to prevent injection attacks. Thoroughly validating user inputs before processing them safeguards against potential vulnerabilities. Sanitizing user inputs removes harmful characters, reducing the risk of attacks. Implementing robust error handling helps in preventing the disclosure of sensitive information during error conditions. Using parameterized queries prevents SQL injection attacks. Regular security audits and penetration testing expose potential vulnerabilities and ensure a secure application.
Understanding and addressing common vulnerabilities such as cross-site scripting (XSS) and cross-site request forgery (CSRF) is vital for preventing malicious attacks. Implementing appropriate countermeasures for these vulnerabilities enhances application security. Staying updated with the latest security best practices is crucial in protecting against emerging threats. Employing a comprehensive security strategy, addressing both authentication, authorization, and input validation, provides multi-layered protection against various types of attacks.
Conclusion
Mastering ASP.NET MVC involves more than just understanding the basics. By delving into advanced techniques such as optimizing performance, leveraging asynchronous operations, implementing robust security measures, and understanding dependency injection and routing, developers can build truly exceptional web applications. This article has explored several advanced concepts, providing a solid foundation for creating sophisticated and efficient applications. Continuous learning and adapting to the latest advancements are key to building robust, scalable, and secure web applications in the ever-evolving landscape of web development.
The future of ASP.NET MVC hinges on developers embracing these advanced techniques and staying abreast of emerging trends and best practices within the technology ecosystem. By incorporating these advanced concepts into their development workflows, developers can create web applications that are not just functional, but also performant, secure, and maintainable.