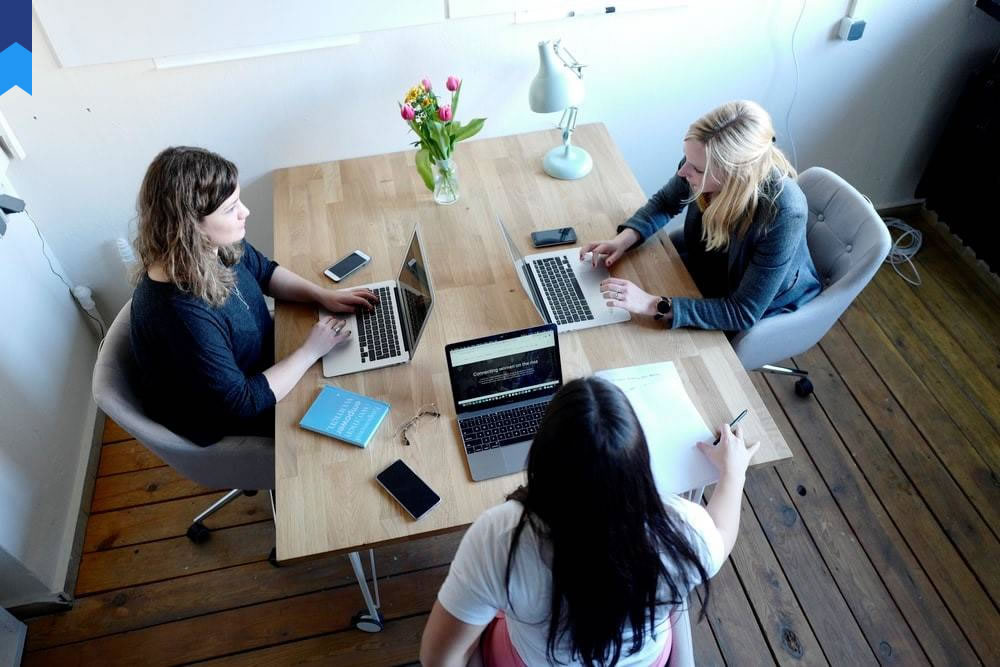
Decoding PySpark's Hidden Power: Advanced How-Tos
PySpark, the powerful distributed computing framework, often hides its true potential behind basic tutorials. This article delves into the sophisticated techniques that transform PySpark from a tool for simple data manipulation into a high-performance engine for complex analytics. We'll explore advanced topics, challenging conventional approaches and revealing unexpected possibilities for maximizing efficiency and unlocking deeper insights.
Mastering PySpark's DataFrames: Beyond the Basics
DataFrames are the cornerstone of PySpark, but many users only scratch the surface of their capabilities. Beyond simple selections and aggregations, advanced techniques like window functions unlock powerful analytical potential. For instance, calculating rolling averages, ranking data within groups, and partitioning for parallel processing are crucial for performance optimization. Consider a case study where a telecom company uses window functions to analyze customer churn patterns over time, identifying trends and predicting future churn based on usage patterns and call duration. Another example involves a financial institution using PySpark to rank transactions based on risk scores, allowing for prioritized fraud detection. Mastering these techniques allows for complex data manipulation with enhanced speed and efficiency.
Furthermore, understanding the nuances of DataFrame operations is critical for efficient memory management. Techniques like caching, broadcasting, and using optimized data structures significantly impact performance. For example, caching frequently accessed DataFrames reduces the time spent on repeated computations. Broadcasting smaller datasets improves performance by avoiding network transfers. Choosing the right data structure, such as using a sparse representation for datasets with many missing values, optimizes memory usage.
Efficient data partitioning is another often-overlooked aspect. Choosing the right partitioning strategy (hash partitioning, range partitioning) dramatically impacts query performance. The choice depends heavily on the nature of the data and the queries being executed. Consider a case study where an e-commerce company improves query response times by 50% by using range partitioning on their product catalog, enabling quicker searches based on price ranges. Similarly, another example could feature a logistics firm improving route optimization analysis by using hash partitioning to distribute data evenly across cluster nodes. Proper partitioning ensures efficient parallel processing.
Finally, optimizing PySpark DataFrame operations requires understanding data serialization and deserialization. Efficient serialization methods minimize the overhead of data movement between nodes and the driver. Using formats like Parquet, known for its columnar storage, can reduce I/O operations and improve query speeds significantly, leading to considerable performance gains. A case study demonstrating this might include a large-scale data warehousing application where Parquet's efficiency results in a 30% reduction in query execution times compared to CSV or other less efficient formats. Another example highlights the value of leveraging optimized serialization libraries for custom data types and complex data structures within the PySpark environment.
Unlocking the Power of Spark SQL: Advanced Queries and Optimizations
Spark SQL, a powerful component of PySpark, extends beyond basic SQL queries. Advanced features like CTEs (Common Table Expressions), window functions, and subqueries enable complex data analysis. For example, CTEs make complex queries easier to read and manage, breaking them down into smaller, more manageable parts. A financial modeling firm uses CTEs to simplify a complex portfolio valuation calculation by separating data extraction, normalization, and calculation steps into different CTEs. Another use case would involve a supply chain management company using CTEs to streamline the tracking of goods in transit, effectively layering multiple queries for complex logistical analysis.
Furthermore, understanding query optimization techniques is essential for maximizing performance. Utilizing Spark's query optimizer to choose the optimal execution plan is crucial. This involves analyzing query plans, identifying bottlenecks, and employing strategies such as data partitioning, caching, and broadcasting. A retail analytics company improved query performance by 40% by optimizing join operations using broadcast joins for smaller datasets. Another company, a social media platform analyzing user engagement metrics, uses data skewness detection and repartitioning for balanced query execution.
Advanced indexing techniques can significantly improve query performance. Creating appropriate indexes in Spark can help accelerate data retrieval. This is especially crucial when dealing with very large datasets or frequent queries. For instance, a healthcare provider uses indexes to efficiently retrieve patient records for real-time medical alerts, improving response times. Another case study involves a search engine optimizing query performance by indexing search terms for faster retrieval of relevant search results.
Utilizing Spark SQL’s built-in functions and UDFs (User-Defined Functions) extends its capabilities. Spark SQL offers a vast library of built-in functions, while UDFs enable custom logic. A bioinformatics company leverages UDFs to process genomic data, implementing custom algorithms for sequence alignment and analysis. Another example shows a financial institution employing custom functions for calculating complex derivatives pricing models within their Spark SQL workflow. Combining these approaches creates flexible and powerful data processing pipelines.
Harnessing the Power of Machine Learning with MLlib
PySpark's MLlib library provides a comprehensive suite of machine learning algorithms. However, true mastery involves understanding model selection, hyperparameter tuning, and performance evaluation. For example, choosing the appropriate algorithm depends on the nature of the data and the problem being solved. A marketing company might use a logistic regression model for customer churn prediction, while a fraud detection system might utilize a random forest model. Another example features a recommendation system leveraging collaborative filtering for user-based movie recommendations.
Hyperparameter tuning, the process of optimizing algorithm parameters, is crucial for achieving optimal model performance. Techniques like grid search and randomized search can help find the best parameter settings. A banking institution significantly improved credit risk assessment accuracy by tuning the hyperparameters of a gradient boosting model using randomized search. Another case study showcases how a manufacturing company optimized their predictive maintenance model by carefully adjusting the parameters of a support vector machine.
Effective model evaluation is essential for assessing the performance of a machine learning model. Metrics such as accuracy, precision, recall, and F1-score provide valuable insights into model quality. A healthcare research organization used ROC curves to evaluate different classifiers for identifying patients at high risk of developing a particular disease. Another study involves evaluating recommendation systems using metrics like precision@k and recall@k to assess the effectiveness of personalized recommendations based on user behavior.
Finally, deploying and scaling MLlib models in a production environment requires careful planning. Techniques such as model serialization, version control, and monitoring are crucial for ensuring the reliability and scalability of the models. A large e-commerce company implemented a robust model deployment pipeline to ensure their recommendation models are updated regularly and perform reliably under high loads. Another case study emphasizes the importance of monitoring model performance over time and retraining models as necessary to maintain accuracy and adapt to evolving data patterns.
Streaming Data Processing with Structured Streaming
PySpark's Structured Streaming enables real-time processing of streaming data. This involves understanding concepts like micro-batch processing, continuous processing, and checkpointing. For example, a social media platform uses structured streaming to analyze trending topics in real-time. Another company, a financial trading firm, employs it for high-frequency data analysis to react immediately to market changes. These examples highlight the power of real-time processing capabilities within the PySpark ecosystem.
Efficiently handling late-arriving data is crucial in streaming applications. Strategies like watermarking help manage the arrival of data that is delayed compared to the stream's overall timeline. A logistics company handles late GPS updates using watermarking to ensure accurate tracking and location analysis. Similarly, another example highlights the use of watermarking in a weather forecasting system to account for delayed sensor readings.
State management is essential for many streaming applications. Using stateful operations allows for tracking data over time and maintaining context across micro-batches or continuous processing. An application monitoring customer sessions uses state management to track user behavior and engagement. Another use case involves tracking inventory levels in a warehouse using stateful operations to maintain accurate records even with intermittent data.
Optimizing Structured Streaming performance involves understanding resource allocation, data partitioning, and query optimization. For instance, adjusting cluster resources and partitioning data appropriately is crucial for handling high-volume streaming data. A large-scale sensor data processing application improves throughput by optimizing the partitioning strategy and resource allocation within its Structured Streaming workflow. Another example demonstrates the value of utilizing advanced query optimization techniques like Catalyst optimizer to enhance processing efficiency in high-velocity data streaming scenarios.
Advanced Performance Tuning and Optimization
Optimizing PySpark performance requires a multi-faceted approach. Understanding resource allocation, including the number of executors, cores per executor, and driver memory, is essential. For example, insufficient memory can lead to out-of-memory errors and slow processing. A data warehouse application enhanced performance by carefully tuning the cluster configuration to match the workload requirements. Similarly, a genomics data analysis application improved processing speed by adjusting executor resources based on the complexity of the bioinformatics algorithms being utilized.
Network configuration impacts PySpark's performance; optimizing network bandwidth and latency reduces data transfer overhead. A distributed machine learning application improved training times by optimizing network configurations between nodes. Similarly, another example shows how a real-time fraud detection system benefited from reduced network latency through optimized network infrastructure.
Data serialization and deserialization significantly impact performance. Using efficient serialization formats like Parquet reduces I/O operations and improves query speeds. A large-scale data processing application reduced query times by 50% by switching to Parquet from CSV. Another example illustrates the optimization achieved by carefully managing data serialization and deserialization in a high-volume data processing pipeline.
Finally, profiling and monitoring PySpark applications is critical for identifying performance bottlenecks. Tools such as Spark UI provide valuable insights into application performance. By analyzing execution plans and identifying slow operations, developers can target specific areas for optimization. A financial analytics company effectively utilized the Spark UI to pinpoint and resolve a slow join operation, significantly improving overall query performance. Similarly, another case study showcases how monitoring resource usage through the Spark UI helped identify memory leaks and address them, leading to enhanced application stability and efficiency. These practices are crucial for building robust, scalable, and high-performance PySpark applications.
Conclusion
Mastering PySpark extends far beyond basic usage. By delving into advanced techniques, developers can unlock the true potential of this powerful framework. This article explored several crucial areas, from optimizing DataFrames and Spark SQL queries to leveraging MLlib and Structured Streaming. Through understanding advanced optimization techniques and best practices, developers can build high-performance, scalable, and reliable PySpark applications, transforming raw data into valuable insights with unprecedented efficiency. The journey to PySpark mastery involves continuous learning and adapting to the ever-evolving landscape of big data technologies.