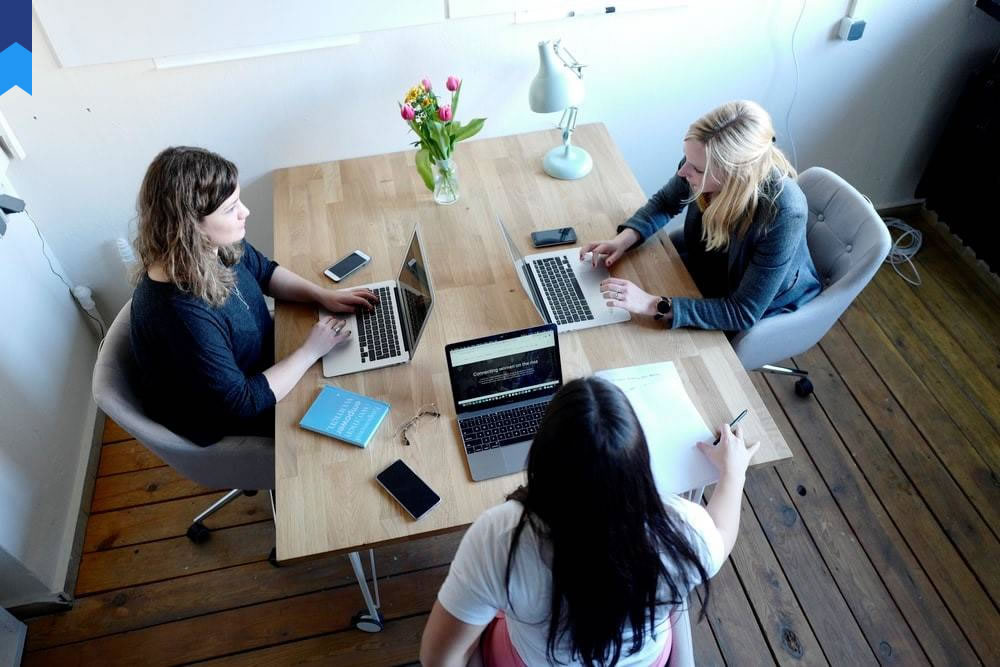
Evidence-Based ASP.NET Core Strategies For Scalable Web Applications
Introduction: ASP.NET Core, Microsoft's open-source framework, empowers developers to build robust and scalable web applications. This article delves into specific, evidence-based strategies to leverage ASP.NET Core's capabilities effectively. We'll explore architectural choices, performance optimization techniques, and best practices supported by real-world examples and industry data, showing how to move beyond basic implementations and unlock the true potential of this powerful framework. This approach emphasizes practical application and demonstrable results rather than theoretical overviews.
Microservices Architecture for Enhanced Scalability
Adopting a microservices architecture is crucial for building scalable ASP.NET Core applications. Instead of a monolithic application, you break down your system into smaller, independent services. Each service focuses on a specific business function, promoting modularity and independent deployment. This approach increases resilience; if one service fails, the others continue to operate. For instance, an e-commerce platform can have separate services for user accounts, product catalogs, and order processing. This allows each service to scale independently based on its specific needs, optimizing resource utilization and reducing overall costs. Consider the case of Netflix, a prime example of successful microservices adoption. Their transition to a microservices architecture allowed them to handle massive traffic spikes during peak viewing times. Another compelling case study comes from Amazon, where independent teams can iterate and deploy updates to their respective services without disrupting the entire platform. The flexibility and efficiency gained through this approach is invaluable. Properly designed API gateways handle communication between services, ensuring loose coupling and enhancing maintainability. This architectural approach allows for easy scaling of individual services to handle increased load. Monitoring tools are vital in a microservices architecture to track performance and identify potential bottlenecks across various services. A robust logging strategy allows for efficient troubleshooting and incident management. Careful consideration of data consistency across multiple services is also crucial. Consistent hashing techniques aid in distributing load effectively, avoiding service overload and ensuring responsiveness even under heavy traffic conditions. By carefully orchestrating communication through message queues or event buses, asynchronous operations are facilitated, improving overall responsiveness and scalability. The added benefit of continuous integration and continuous delivery (CI/CD) simplifies the deployment process and helps maintain consistent code quality across independent services.
Leveraging Caching Strategies for Optimal Performance
Caching is a fundamental performance optimization technique in ASP.NET Core applications. Caching mechanisms reduce database load by storing frequently accessed data in memory. Different caching strategies exist, depending on the specific needs of your application. For instance, using in-memory caching like `MemoryCache` for short-lived data provides immediate speed benefits. Distributed caching systems such as Redis are suitable for large-scale applications where data needs to be shared across multiple servers. Properly implementing output caching helps reduce server-side processing by storing rendered HTML pages. Implementing a robust caching strategy considers aspects such as cache expiration policies, cache invalidation techniques, and data consistency. A properly implemented caching strategy can dramatically improve the response times of your web application. Consider the case of a news website; caching frequently accessed articles reduces database load and improves response times during traffic surges. Imagine a banking application where crucial account data is cached to provide almost instantaneous response times to user requests. The importance of appropriate cache eviction policies cannot be overstated. Least Recently Used (LRU) or Least Frequently Used (LFU) algorithms ensure that the most relevant data is prioritized in the cache, preventing performance degradation due to cache overflow. Data consistency across multiple cache levels must be managed effectively, avoiding data discrepancies and ensuring data integrity. Properly structuring your cache keys is also essential for efficient data retrieval and cache management. An effective caching strategy requires careful consideration of various factors. Choosing the right caching technology, configuring eviction policies, and setting appropriate expiration times are crucial aspects. Regularly monitoring and tuning your cache to optimize performance is essential for maintaining responsiveness, especially in high-traffic scenarios. An effective caching strategy results in a significant boost to the scalability and performance of your web application.
Asynchronous Programming for Improved Responsiveness
Asynchronous programming is crucial for building responsive ASP.NET Core applications. Asynchronous operations allow your application to continue processing other requests without blocking on long-running tasks. This is particularly important in scenarios involving database operations, external API calls, or file I/O. Using the `async` and `await` keywords allows you to write asynchronous code that is easier to read and maintain. Consider an e-commerce application where processing an order might involve database interactions, external payment gateway calls, and inventory updates. With asynchronous programming, these operations can be handled concurrently, without blocking the user interface and improving overall responsiveness. Similarly, a social media application handling user requests, processing images and videos, and interacting with external APIs will greatly benefit from the responsiveness provided by asynchronous programming. The asynchronous approach ensures that the user is not kept waiting while the application performs background tasks. Proper error handling within asynchronous methods is essential for preventing unhandled exceptions. Proper utilization of tasks and asynchronous programming requires understanding of task cancellation and proper handling of exceptions during asynchronous operations. A well-structured asynchronous design prevents blocking scenarios and ensures responsiveness in high-concurrency environments. Monitoring tools aid in identifying potential bottlenecks and measuring the efficiency of asynchronous operations. Employing asynchronous programming throughout your application architecture will significantly improve the responsiveness and user experience of your application. Using asynchronous techniques dramatically reduces response times compared to synchronous operations. Optimizing asynchronous code often involves careful management of tasks and avoiding unnecessary context switching, ensuring efficient resource utilization.
Implementing Effective Logging and Monitoring
Robust logging and monitoring are critical aspects of building scalable and maintainable ASP.NET Core applications. Effective logging provides insights into application behavior, helps identify errors, and facilitates debugging. Using logging frameworks like Serilog or NLog allows structured logging, facilitating efficient log analysis and monitoring. Centralized logging systems like Elasticsearch, Fluentd, and Kibana (EFK stack) aggregate logs from various sources, aiding in comprehensive monitoring and analysis. Real-time monitoring dashboards provide immediate insights into application performance, allowing for proactive identification and resolution of issues. Application insights and performance monitoring tools provide valuable data about response times, error rates, and resource utilization. By proactively tracking key metrics, teams can quickly address bottlenecks, improving application performance and scalability. For instance, monitoring CPU usage, memory consumption, and database connection pool size are crucial aspects in identifying areas for optimization. Consider the case of a banking application where real-time monitoring of transaction processing times is critical for ensuring operational efficiency. A social media platform will similarly benefit from thorough monitoring and logging of user activity and system performance. Effective monitoring and logging are essential to proactively manage issues that could potentially impact scalability. Implementing appropriate alerts for critical events enables timely intervention and reduces potential downtime. A properly designed logging and monitoring strategy enhances the maintainability and overall reliability of your ASP.NET Core application. Regular log analysis reveals patterns and trends, informing future development decisions and helping prevent recurring issues. This data-driven approach helps in proactive maintenance, improving both performance and user experience.
Security Best Practices for Secure Applications
Security is paramount in building scalable ASP.NET Core applications. Implementing robust security practices throughout the development lifecycle is crucial to protecting sensitive data and preventing vulnerabilities. ASP.NET Core provides built-in security features that can be enhanced through additional security measures. Input validation and sanitization prevent injection attacks, ensuring that user-provided data cannot compromise the application. Proper authentication and authorization mechanisms are crucial for controlling access to application resources. Using industry-standard encryption techniques for sensitive data protects against unauthorized access. Regular security audits and penetration testing identify and address potential vulnerabilities before they can be exploited. Consider an e-commerce platform where protecting sensitive customer data, payment information, and order details is essential. Similarly, a healthcare application will have stringent security requirements to protect patient privacy and confidentiality. Implementing a zero-trust security model reduces the attack surface by verifying each access request regardless of network location. Regular updates and patching ensure that the application benefits from the latest security fixes. The principles of least privilege ensure that users and processes only have access to the resources necessary for their tasks. Strong password policies and multi-factor authentication add additional layers of security, protecting against unauthorized access. Security awareness training for developers and other stakeholders helps ensure a security-conscious mindset across the organization. By embedding security best practices throughout the entire development process, organizations can reduce their attack surface significantly, fostering a strong security posture for their applications. Regular vulnerability assessments provide a continuous security improvement cycle, addressing any new or emerging threats promptly and efficiently. Maintaining strong security protocols helps build user trust and protect against potential data breaches.
Conclusion: Building truly scalable and performant ASP.NET Core applications requires a strategic approach incorporating multiple well-defined practices. This article provided a detailed exploration of these strategies, emphasizing practical application and leveraging evidence-based best practices. By implementing the strategies highlighted – including adopting a microservices architecture, leveraging effective caching, utilizing asynchronous programming, establishing robust logging and monitoring, and implementing rigorous security measures – developers can confidently create applications capable of handling substantial growth and maintaining high levels of performance and security.