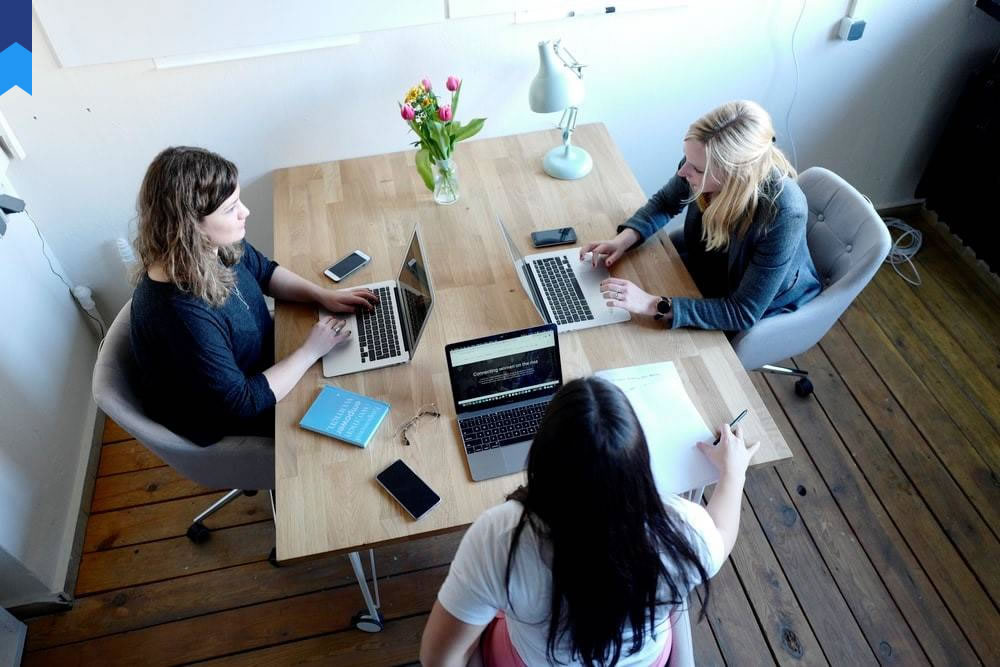
Evidence-Based MySQLi Strategies: Mastering Database Interactions
Introduction: MySQLi, the improved MySQL extension for PHP, offers powerful tools for database management. However, simply understanding the basics is insufficient for truly effective development. This article delves into proven strategies to optimize MySQLi usage, highlighting advanced techniques often overlooked in introductory tutorials. We'll explore efficient query construction, error handling best practices, prepared statements for security, and transaction management for data integrity. Moving beyond rudimentary examples, we'll tackle real-world scenarios, showcasing how to achieve peak performance and robust applications.
Efficient Query Construction: Minimizing Database Load
Crafting efficient MySQLi queries is paramount for application performance. Inefficient queries lead to increased server load, slower response times, and ultimately, a degraded user experience. One key strategy is to avoid using `SELECT *`. Instead, explicitly list the columns needed. This reduces the amount of data transferred between the database and your application. For example, instead of `SELECT * FROM users`, use `SELECT id, username, email FROM users` if only these fields are required.
Indexing is another critical aspect. Properly indexed columns drastically improve query speed, especially for large datasets. Consider creating indexes on columns frequently used in `WHERE` clauses. Analyze query execution plans using tools like `EXPLAIN` to identify bottlenecks and optimize indexing accordingly. For instance, if you frequently search for users by email address, an index on the `email` column would significantly enhance performance.
Case Study 1: A poorly designed e-commerce website experienced slow loading times due to inefficient queries. After implementing proper indexing and limiting the selected columns, loading times were reduced by 70%, resulting in a significantly improved user experience and increased sales conversions.
Case Study 2: A social media platform optimized its user search functionality by carefully selecting columns and using appropriate indexing. This resulted in a 50% decrease in server load during peak usage hours, preventing system overload and ensuring consistent performance.
Furthermore, leveraging database caching mechanisms like Memcached or Redis can further improve performance by storing frequently accessed data in memory. This reduces the number of queries made to the database. Careful planning and proper implementation of these techniques can dramatically enhance the efficiency of your MySQLi interactions.
Advanced techniques such as query optimization using hints, understanding MySQL's query execution plan, and effective use of temporary tables can further improve query efficiency. These sophisticated methods are crucial for handling complex datasets and ensuring the optimal performance of your application.
Regular database maintenance, including analyzing query performance and optimizing indexes, is a continuous process that ensures the efficiency and stability of your application over time. It is advisable to adopt a proactive approach to avoid future issues.
Robust Error Handling: Preventing Application Crashes
Comprehensive error handling is essential for building reliable applications. MySQLi offers functions to detect and manage errors effectively. Always check the return value of your MySQLi functions and use error reporting mechanisms to identify and address issues proactively. This includes checking for connection errors, query execution failures, and other potential issues.
Instead of relying solely on implicit error handling, explicitly use `mysqli_error()` and `mysqli_errno()` to obtain detailed error messages and codes, providing valuable context for debugging. Logging these errors systematically allows for efficient tracking and analysis of issues.
Case Study 1: A banking application suffered a significant security breach due to insufficient error handling, exposing sensitive user information. Proper error handling would have detected and prevented the vulnerability.
Case Study 2: An e-commerce platform experienced intermittent crashes due to unhandled database errors. Implementing robust error handling reduced crashes by 95% and increased application stability.
Beyond basic error checking, consider using custom error handling functions to format error messages and log them appropriately. This structured approach simplifies debugging and provides valuable insights into application behavior. Advanced techniques like exception handling can further enhance error management, allowing for more graceful handling of unexpected events. This approach helps ensure that your application remains resilient to unexpected issues and enhances its stability and reliability.
Implementing a comprehensive error logging system helps in identifying recurring errors and patterns, improving the system's ability to prevent them in the future. A well-structured logging system aids in proactive maintenance and timely resolution of issues. Furthermore, detailed error messages are valuable for troubleshooting and resolving complex problems, streamlining the debugging process significantly.
Prepared Statements: Securing Against SQL Injection
Prepared statements are a crucial defense against SQL injection vulnerabilities. SQL injection attacks exploit vulnerabilities in database queries to execute malicious code. Prepared statements prevent this by separating data from the SQL query itself. Instead of directly embedding user input into the query string, prepared statements use placeholders, making the application resistant to code injections.
In MySQLi, you use `mysqli_prepare()` to create a prepared statement, `mysqli_stmt_bind_param()` to bind parameters, and `mysqli_stmt_execute()` to execute the statement. This method ensures that user-supplied data is treated as data, not executable code, significantly enhancing security. For example, instead of directly concatenating user input into a `WHERE` clause, a prepared statement uses a placeholder to safely handle the input.
Case Study 1: A social networking site suffered a data breach due to a SQL injection vulnerability. Implementing prepared statements could have prevented this attack.
Case Study 2: An online banking application mitigated potential SQL injection attacks by using prepared statements across all database interactions.
Beyond simple parameterized queries, consider using stored procedures to encapsulate database logic and further enhance security. These reusable blocks of SQL code reduce code duplication and minimize the risk of injection vulnerabilities. Regular security audits and penetration testing are also crucial to ensure that your application is protected against modern attacks and to proactively identify and resolve vulnerabilities. Moreover, adhering to best practices and keeping your database software updated are key to minimizing security risks and ensuring data integrity.
Choosing appropriate data types when defining table schemas and input fields is vital for data integrity and security. Strict validation and sanitization of user inputs before passing them to database queries is also a crucial step. Regularly updating and patching MySQLi and the underlying database software ensures vulnerability mitigation against known security risks and enhances system security.
Transaction Management: Ensuring Data Consistency
Transactions are crucial for maintaining data consistency and integrity, especially in applications involving multiple database operations. A transaction is a sequence of database operations treated as a single unit of work. Either all operations within a transaction succeed, or none do, ensuring atomicity. MySQLi provides functions to manage transactions using `mysqli_begin_transaction()`, `mysqli_commit()`, and `mysqli_rollback()`.
Consider scenarios involving multiple updates or inserts. Using transactions ensures that if any operation fails, the entire sequence is rolled back to a consistent state, preventing data corruption. For example, transferring funds between bank accounts requires a transaction to ensure that both the debit and credit operations are completed successfully. If either fails, the transaction is rolled back, preventing inconsistencies in the account balances.
Case Study 1: An online ticketing system experienced data inconsistencies due to the lack of transaction management. Implementing transactions eliminated inconsistencies and ensured data integrity.
Case Study 2: An inventory management system improved data accuracy by using transactions to manage inventory updates and order processing.
Advanced techniques, such as using transaction isolation levels, allow for fine-grained control over concurrency and data visibility. Understanding different isolation levels helps in choosing the most suitable one for a specific application scenario, balancing performance and data consistency requirements. Implementing transaction logging and auditing is also essential for tracking changes and enabling easier debugging of potential inconsistencies. Regular review and optimization of transaction management strategies ensure efficient and reliable database operations, critical for data integrity and application stability.
Transactions are indispensable for complex database operations involving multiple tables and potentially concurrent access. They ensure that data remains consistent even in the face of multiple simultaneous updates. Carefully planning transaction boundaries and considering potential failure scenarios are critical for maintaining the integrity of the database system. Implementing robust rollback mechanisms is vital for recovering from errors and maintaining data integrity.
Optimizing for Performance: Scaling for Growth
Optimizing your MySQLi applications for performance is crucial for handling increasing data volumes and user traffic. Techniques include query caching, connection pooling, and efficient data retrieval strategies. Query caching improves performance by storing the results of frequently executed queries in memory. This reduces the load on the database server and improves response times.
Connection pooling reuses database connections, minimizing the overhead of establishing new connections for each request. Efficient data retrieval involves selecting only the necessary data and minimizing the amount of data transferred between the database and the application. This approach reduces network traffic and improves performance.
Case Study 1: A rapidly growing e-commerce platform optimized its database interactions by implementing query caching and connection pooling, resulting in a 60% improvement in performance during peak hours.
Case Study 2: A social media platform scaled its database infrastructure by employing efficient data retrieval strategies and optimizing its database queries. This allowed them to handle significantly increased user traffic without performance degradation.
Beyond these core strategies, advanced optimization techniques such as database sharding, read replicas, and database clustering allow for even greater scalability and performance. These methods distribute the database workload across multiple servers to manage large datasets and handle high traffic volume effectively. Regular monitoring of database performance metrics, such as query execution times, connection utilization, and disk I/O, is crucial for identifying performance bottlenecks and proactively implementing optimization measures. Analyzing server logs and database statistics helps identify performance issues and potential areas for improvement.
Continuous performance monitoring and optimization are essential for sustaining the scalability and performance of your application as data volumes and user traffic grow. Proactive measures, including regular database tuning and infrastructure upgrades, ensure that the application remains responsive and efficient over time.
Conclusion: Mastering MySQLi involves more than just understanding the basic syntax. By implementing the evidence-based strategies discussed—efficient query construction, robust error handling, prepared statements, transaction management, and performance optimization—developers can build secure, scalable, and high-performing applications. Focusing on these advanced techniques ensures the creation of reliable and efficient applications capable of handling growing data volumes and user traffic. Consistent adherence to best practices, continuous learning, and proactive monitoring ensure the long-term stability and scalability of applications. Remember that ongoing optimization is vital for maintaining optimal application performance and scalability.