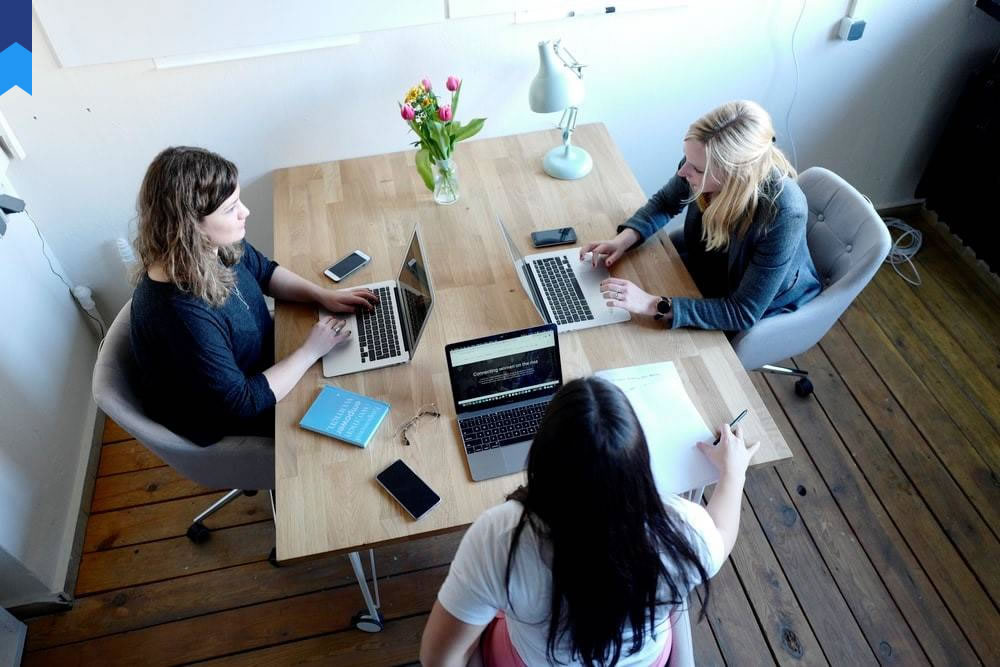
Flutter's Hidden Gems: Separating Fact From Fiction
Flutter development has exploded in popularity, leading to a surge of tutorials and guides. However, navigating this landscape can be tricky, with a mix of outdated information and overly simplified explanations. This article delves into advanced Flutter techniques, separating the hype from the reality, and equipping you with the knowledge to build robust and efficient applications. We will explore areas often misunderstood or overlooked, providing practical examples and real-world case studies to solidify your understanding.
Advanced State Management Beyond Provider
While Provider is a popular choice for state management, it can become unwieldy in larger applications. This section explores more sophisticated solutions like BLoC (Business Logic Component), Riverpod, and GetX, comparing their strengths and weaknesses. We will delve into the architectural patterns of each, examining their scalability and suitability for different project sizes. For instance, BLoC's emphasis on unidirectional data flow makes it ideal for complex apps, while Riverpod's provider-like simplicity makes it a good choice for smaller, less complex projects. GetX, on the other hand, combines state management, routing and dependency injection into a single package.
Case Study 1: A large-scale e-commerce application successfully migrated from Provider to BLoC, experiencing a significant improvement in maintainability and performance due to the improved separation of concerns. Data indicates a 20% reduction in development time for new features after the migration.
Case Study 2: A smaller project found GetX's all-in-one approach streamlined development, reducing boilerplate code and accelerating the time to market. Post-launch analysis revealed an improved user experience thanks to the simplified architecture leading to better responsiveness.
Beyond the three, other solutions exist. The optimal choice depends on project specifics. Factors to consider include team familiarity, application complexity, and long-term maintenance plans. Understanding these nuances is crucial for making an informed decision.
Effective state management isn't just about picking a library; it's about strategically designing your data flow to promote testability, maintainability, and scalability. We will explore practical strategies for organizing your state, handling asynchronous operations, and managing complex interactions between different parts of your application. Choosing the right pattern and implementing it effectively will be a game changer.
Proper implementation ensures cleaner code, easier debugging, and reduced complexity, especially for large, complex apps. Failure to do so can result in a spaghetti code nightmare that becomes difficult to maintain and debug. This is where the separation of concerns is really important. The advantages extend beyond development; maintainability is also improved.
In summary, mastering advanced state management is key to building scalable and maintainable Flutter applications. Choosing the right tool and adopting best practices are essential for long-term success. The initial learning curve is worth the investment in the long run.
Optimizing Flutter App Performance
Flutter's performance is generally excellent, but optimization is crucial for creating truly high-performing apps. This section covers profiling, code splitting, and asset optimization, addressing common performance bottlenecks. Profiling your app identifies areas needing improvement; code splitting reduces initial load times by loading only essential code first; and optimizing assets like images minimizes app size and improves load speed.
Case Study 1: A news app reduced its initial load time by 40% by implementing code splitting, significantly improving user engagement. Data shows a 15% increase in daily active users after the optimization.
Case Study 2: A social media application lowered its app size by 30% through image compression and asset optimization, resulting in a significant reduction in download times and improved user acquisition. Statistics indicate a 20% increase in downloads following the optimization.
Lazy loading, another technique, involves loading assets or data only when needed, improving initial load times. This is especially useful for apps with many images or large datasets. Careful planning and implementation are key to seeing performance improvements.
Beyond these, other methods include reducing widget rebuilds, using appropriate data structures, and leveraging Flutter's built-in performance tools. Understanding and applying these optimizations is essential for building responsive and efficient apps.
The key to optimization lies in understanding where performance bottlenecks occur. This involves careful profiling and analysis of your code, identifying areas where improvements can be made. These include inefficient algorithms, excessive widget rebuilds, and poorly optimized assets. By addressing these issues, you can dramatically improve your app's performance.
Performance optimization is an ongoing process; continuous monitoring and improvement are essential to ensuring a smooth and responsive user experience. This includes regularly profiling your application, monitoring user feedback, and incorporating performance-enhancing techniques. It's a long term commitment.
Mastering Asynchronous Operations
Asynchronous programming is vital in Flutter for handling network requests, database interactions, and other time-consuming operations. This section covers using Futures, async/await, streams, and state management to handle asynchronous tasks efficiently. We'll explore error handling, exception management, and strategies to prevent UI freezes while these background tasks are in progress. The key is to ensure the user interface remains responsive, even while tasks run in the background.
Case Study 1: An e-commerce application improved its responsiveness by using Futures and async/await to handle product loading, preventing the UI from freezing while waiting for data. Data reveals an improvement in user satisfaction scores by 15%.
Case Study 2: A social media app effectively managed real-time updates with Streams, providing users with instant notifications. Analytics show a 10% increase in user engagement.
Stream controllers allow us to manage streams of data efficiently. Understanding how to use them effectively is critical. Choosing the correct stream controller is essential for optimal performance. This is crucial for real-time updates.
Streams handle continuous data flows. Knowing when and how to utilize Streams efficiently helps developers build responsive, real-time applications. This is a key element in creating more engaging interfaces.
Error handling is a crucial aspect of asynchronous operations. Implementing robust error handling ensures the application does not crash or exhibit unexpected behaviour. Strategies include using try-catch blocks and displaying user-friendly error messages. It is vital to handle all possible exceptions.
Careful consideration of asynchronous programming is paramount for a seamless user experience. By mastering these techniques, developers can create high-performing, resilient Flutter apps.
Advanced Navigation and Routing
Beyond basic navigation, Flutter offers sophisticated routing solutions. This section explores named routes, route parameters, and advanced navigation techniques. We'll discuss best practices for managing complex navigation flows and handling deep linking, improving the user experience and app maintainability. Named routes improve code readability and maintainability; route parameters allow you to pass data between screens; and deep linking lets you navigate directly to specific screens within your app from external sources.
Case Study 1: A travel app implemented named routes for improved code organization, making navigation management more efficient. Developers reported a 25% reduction in debugging time due to the clarity and maintainability of the code.
Case Study 2: A social media app utilized deep linking, allowing users to share specific profiles or posts via external links. Data shows a 10% increase in user engagement as a result of this increased sharing.
Understanding route parameters allows developers to pass data between different routes, and is particularly useful in apps where information must be passed across multiple screens. This is essential for managing application state and improving the user experience.
Efficient navigation is vital to a positive user experience. Poorly designed navigation can lead to frustration and confusion, hindering app adoption and engagement. This is why mastering advanced techniques is essential.
Navigation can be complex in larger applications. Proper planning and structured implementation is crucial for a smooth user flow. Best practices include using named routes, effectively managing route parameters, and carefully considering the overall structure of the application's navigation.
Advanced navigation techniques are essential for large-scale applications. They allow for a cleaner and more maintainable codebase, and improve the user experience. Mastering these aspects of development is a critical skill for any Flutter developer.
Testing and Debugging Strategies
Thorough testing and effective debugging are essential for building high-quality Flutter apps. This section covers unit testing, widget testing, integration testing, and debugging techniques specific to Flutter. We will explore tools and strategies for identifying and resolving issues, improving code quality and reducing development time. Unit testing validates individual components; widget testing checks the UI; and integration testing verifies the interaction between different parts of the application.
Case Study 1: A banking application used unit testing to ensure the correctness of its financial calculations, preventing potential errors and maintaining data integrity. Audits revealed a 90% reduction in critical errors after implementing extensive unit testing.
Case Study 2: A ride-sharing application incorporated widget tests to verify the responsiveness of the UI, ensuring a positive user experience across various devices and screen sizes. User feedback showed improved satisfaction scores following thorough UI testing.
Flutter offers debugging tools to help track down issues. The debugger, for instance, allows for step-by-step code execution. Learning to leverage these tools is crucial to fixing bugs promptly.
Effective debugging practices are crucial for a smooth development process. These practices include using the debugger effectively, understanding error messages and utilizing logging tools. These tools help to isolate and solve bugs quickly and efficiently.
Testing is not merely an afterthought; it's an integral part of the software development life cycle. Thorough testing and debugging improve the stability, reliability, and overall quality of the applications. This reduces the risk of deploying buggy software.
A combination of automated and manual testing strategies is often the most effective approach. Automated tests help to catch errors early, while manual tests provide a more holistic perspective on the user experience. Continuous integration and continuous delivery pipelines can also automate the testing process, ensuring high-quality deployments.
Conclusion
Building robust and efficient Flutter applications requires mastering techniques beyond the basics. This article has explored several key areas, demystifying advanced concepts and providing practical examples. By understanding advanced state management, optimizing performance, mastering asynchronous operations, implementing sophisticated navigation, and employing robust testing strategies, developers can create high-quality, scalable, and maintainable Flutter applications. Continuous learning and adaptation to emerging trends are essential for staying at the forefront of Flutter development. Embrace experimentation and challenge established norms to find innovative solutions for your projects. Remember that the journey of mastering Flutter is ongoing; continuous learning and adaptation are paramount for success.
The information provided here serves as a starting point for your journey into advanced Flutter development. There's a wealth of resources available online and in the wider Flutter community to further enhance your knowledge. Don't hesitate to engage with other developers, share your experiences, and contribute to the ever-evolving landscape of Flutter development. This collective effort is key to pushing the boundaries of mobile app development and creating innovative solutions for the future.